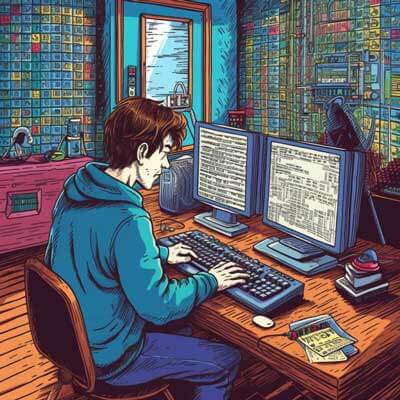
Scrolling to the top of a page using JavaScript is a common task in web development. There are several ways to achieve this, and in this answer, we will explore two possible solutions.
Solution 1: Using the scrollTo() Method
One straightforward way to scroll to the top of a page is by using the scrollTo()
method. This method allows you to scroll to a specific coordinate on the page. To scroll to the top, we need to set the x and y coordinates to 0.
Here’s an example of how you can implement this:
function scrollToTop() { window.scrollTo(0, 0); }
In the above code snippet, we define a function called scrollToTop()
that utilizes the scrollTo()
method to scroll to the top of the page. By setting the x and y coordinates to 0, we ensure that the page scrolls to the top-left corner.
You can trigger this function in various ways, such as attaching it to a button’s click event or calling it programmatically. For example, to scroll to the top when a button with the ID “scrollButton” is clicked, you can use the following code:
document.getElementById("scrollButton").addEventListener("click", scrollToTop);
Related Article: nvm (Node Version Manager): Install Guide & Cheat Sheet
Solution 2: Using the scrollTop Property
Another approach to scrolling to the top of a page is by manipulating the scrollTop
property of the document’s body or the documentElement
(HTML element).
Here’s an example of how you can use the scrollTop
property to achieve this:
function scrollToTop() { document.documentElement.scrollTop = 0; // For modern browsers document.body.scrollTop = 0; // For older browsers }
In the code snippet above, we define the scrollToTop()
function, which sets the scrollTop
property of both the document.documentElement
and document.body
elements to 0. This ensures compatibility with both modern and older browsers.
Similar to the previous solution, you can trigger this function using an event listener or by calling it programmatically.
Best Practices and Considerations
When implementing scroll-to-top functionality, it’s important to consider accessibility and user experience. Here are some best practices to keep in mind:
1. Provide a visual cue: When scrolling to the top, it’s helpful to provide users with a visual cue, such as a smooth scroll animation or a scroll-to-top button, to indicate that the action has been triggered.
2. Accessibility considerations: Ensure that the scroll-to-top functionality is accessible to all users. This includes providing keyboard navigation support and ensuring that the functionality is compatible with screen readers.
3. Smooth scrolling animation: To enhance the user experience, consider implementing a smooth scroll animation when scrolling to the top. This can be achieved using CSS transitions or JavaScript libraries that provide smooth scrolling functionality.
4. Performance considerations: When implementing scroll-to-top functionality, be mindful of performance implications, especially if the page contains a large amount of content. Consider using techniques like debouncing or throttling to optimize the scroll event handling.
5. Cross-browser compatibility: Test your scroll-to-top implementation across different browsers to ensure consistent behavior. Some older browsers may require specific workarounds to achieve proper scrolling.
Overall, scrolling to the top of a page using JavaScript is a relatively simple task that can greatly improve the user experience of your web application. By following best practices and considering accessibility, you can ensure that your implementation is user-friendly and inclusive.
For more information on scrolling and related techniques, you can refer to the following resources:
– [MDN Web Docs: Window.scrollTo()](https://developer.mozilla.org/en-US/docs/Web/API/Window/scrollTo)
– [MDN Web Docs: Element.scrollTop](https://developer.mozilla.org/en-US/docs/Web/API/Element/scrollTop)
Related Article: How to Use the forEach Loop with JavaScript