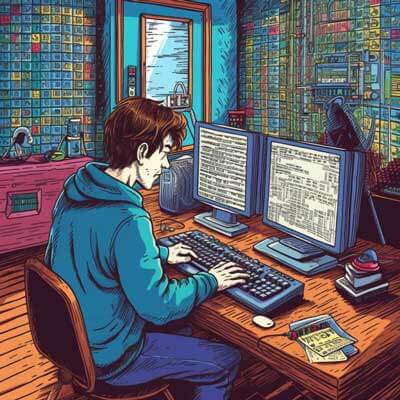
The setTimeout
function in jQuery allows you to execute a function after a specified amount of time has passed. This can be useful for delaying the execution of code, creating animations, or implementing timeout functionality in your web applications. In this guide, we will walk through the steps of using the setTimeout
function in jQuery.
Syntax
The syntax for using the setTimeout
function in jQuery is as follows:
setTimeout(function, delay);
– The function
parameter is the function that you want to execute after the specified delay.
– The delay
parameter is the amount of time, in milliseconds, to wait before executing the function.
Related Article: nvm (Node Version Manager): Install Guide & Cheat Sheet
Examples
Here are a few examples that demonstrate how to use the setTimeout
function in different scenarios:
Example 1: Delayed Execution
setTimeout(function() { console.log("Hello, world!"); }, 2000);
In this example, the function console.log("Hello, world!")
will be executed after a delay of 2000 milliseconds (or 2 seconds). This can be useful for delaying the execution of a specific task or animation.
Example 2: Repeated Execution
var count = 0; function incrementCount() { count++; console.log("Count: " + count); if (count < 5) { setTimeout(incrementCount, 1000); } } setTimeout(incrementCount, 1000);
In this example, the function incrementCount
is called every 1 second. It increments a counter variable count
and logs the current value to the console. The function will continue executing until the value of count
reaches 5.
Best Practices
When using the setTimeout
function in jQuery, it is important to keep the following best practices in mind:
1. Use meaningful function names: Choose descriptive names for the functions that you pass to setTimeout
. This makes your code more readable and maintainable.
2. Clear timeouts when necessary: If you no longer need a timeout to execute, it is a good practice to clear it using the clearTimeout
function. This helps prevent unnecessary function calls and improves performance.
var timeoutId = setTimeout(function() { console.log("This will not be executed"); }, 5000); // Clear the timeout clearTimeout(timeoutId);
3. Use anonymous functions sparingly: While it is common to use anonymous functions with setTimeout
, it can make your code harder to read and debug. Consider defining your functions separately and passing them as arguments instead.
4. Use the requestAnimationFrame
function for animations: For smooth and efficient animations, consider using the requestAnimationFrame
function instead of setTimeout
. It synchronizes with the browser’s rendering and reduces jank.
function animate() { // Animation logic here if (condition) { requestAnimationFrame(animate); } } requestAnimationFrame(animate);
Related Article: How to Use the forEach Loop with JavaScript