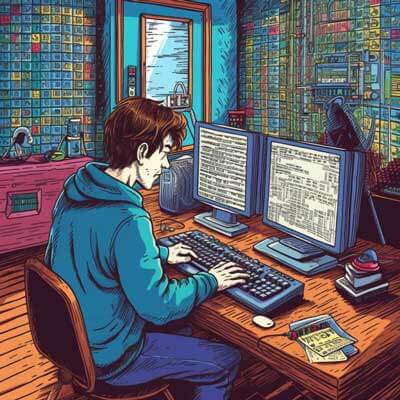
Using Hash Maps in Python
A hash map, also known as a hash table or dictionary, is a data structure that allows for efficient storage and retrieval of key-value pairs. In Python, hash maps can be implemented using the built-in dict
class. Here, we will explore how to use hash maps in Python and cover best practices and examples.
Related Article: How To Convert a Dictionary To JSON In Python
Creating a Hash Map
To create a hash map in Python, you can simply initialize an empty dictionary using curly braces {}
or by using the dict()
constructor. Here are a few examples:
# Method 1: Using curly braces my_hash_map = {} # Method 2: Using the dict() constructor my_hash_map = dict()
Adding and Retrieving Elements
To add elements to a hash map, you can use the square bracket notation. The key is enclosed in square brackets, followed by the assignment operator =
, and then the corresponding value. Here’s an example:
my_hash_map = {} my_hash_map['name'] = 'John' my_hash_map['age'] = 30
To retrieve values from a hash map, you can use the same square bracket notation and provide the key. If the key exists, the corresponding value will be returned. If the key does not exist, a KeyError
will be raised. Here’s an example:
my_hash_map = {'name': 'John', 'age': 30} print(my_hash_map['name']) # Output: John print(my_hash_map['gender']) # Raises KeyError: 'gender'
Checking if a Key Exists
To check if a key exists in a hash map, you can use the in
keyword. It returns a boolean value indicating whether the key exists in the hash map or not. Here’s an example:
my_hash_map = {'name': 'John', 'age': 30} print('name' in my_hash_map) # Output: True print('gender' in my_hash_map) # Output: False
Related Article: How to Sort a Dictionary by Key in Python
Updating and Deleting Elements
To update the value of an existing key in a hash map, you can use the same square bracket notation and provide the key. If the key exists, the value will be updated. If the key does not exist, a new key-value pair will be added. Here’s an example:
my_hash_map = {'name': 'John', 'age': 30} my_hash_map['age'] = 31 # Update value my_hash_map['gender'] = 'Male' # Add new key-value pair
To delete a key-value pair from a hash map, you can use the del
keyword and provide the key. If the key exists, the corresponding key-value pair will be removed. If the key does not exist, a KeyError
will be raised. Here’s an example:
my_hash_map = {'name': 'John', 'age': 30} del my_hash_map['age']
Iterating Over a Hash Map
To iterate over the keys or values of a hash map, you can use the keys()
and values()
methods, respectively. These methods return iterable objects that can be used in a loop or converted to a list using the list()
constructor. Here are a few examples:
my_hash_map = {'name': 'John', 'age': 30} # Iterate over keys for key in my_hash_map.keys(): print(key) # Iterate over values for value in my_hash_map.values(): print(value) # Convert keys to a list keys_list = list(my_hash_map.keys()) # Convert values to a list values_list = list(my_hash_map.values())
Best Practices
– Choose meaningful and descriptive keys for your hash map. This will make your code more readable and maintainable.
– Be cautious when using mutable objects as keys, such as lists or dictionaries. Mutable objects can be modified, which may lead to unexpected behavior in the hash map.
– Take advantage of the built-in methods and functions provided by Python’s dict
class, such as get()
, pop()
, and items()
. These methods can simplify your code and improve performance in certain scenarios.