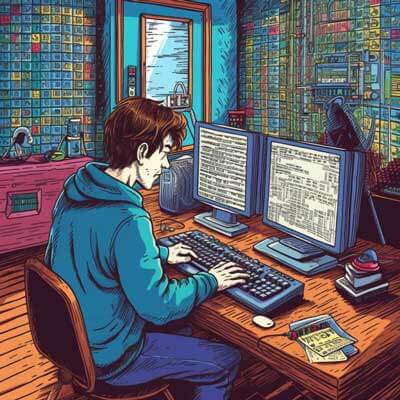
- Working Principles of ReactJS
- Benefits of ReactJS for Front-end Development
- Building a Full Application with ReactJS
- Understanding JSX in ReactJS
- Exploring the Role of Virtual DOM in ReactJS
- Creating Mobile Apps with ReactJS
- Handling Routing in a ReactJS Application
- Understanding Redux and its Relationship with ReactJS
- Popular Libraries and Frameworks Compatible with ReactJS
- Additional Resources
Working Principles of ReactJS
ReactJS is a JavaScript library that is used for building user interfaces. It follows the component-based architecture, where the UI is divided into reusable components. These components are then combined to create complex UIs.
ReactJS works on the principle of Virtual DOM (Document Object Model). The Virtual DOM is a lightweight copy of the actual DOM, which is a representation of the HTML structure of a web page. ReactJS uses the Virtual DOM to efficiently update only the necessary parts of the UI, rather than re-rendering the entire page.
When a component’s state or props change, ReactJS creates a new Virtual DOM representation of the component. It then compares this new Virtual DOM with the previous one to identify the minimal set of changes needed to update the actual DOM. This process is known as reconciliation.
The diffing algorithm used by ReactJS is highly efficient, allowing for fast and optimized updates to the UI. This makes ReactJS well-suited for building dynamic web applications.
Here’s an example of a simple ReactJS component:
import React from 'react'; class Counter extends React.Component { constructor(props) { super(props); this.state = { count: 0 }; } incrementCount() { this.setState({ count: this.state.count + 1 }); } render() { return ( <div> <h2>Counter: {this.state.count}</h2> <button onClick={() => this.incrementCount()}>Increment</button> </div> ); } } export default Counter;
In this example, we have a Counter
component that maintains a count state. The incrementCount
method is called when the button is clicked, updating the count state and triggering a re-render of the component.
Related Article: Enhancing React Applications with Third-Party Integrations
Benefits of ReactJS for Front-end Development
ReactJS offers several benefits for front-end development:
1. Component-based Architecture: ReactJS promotes the use of reusable components, which leads to modular and maintainable code. Components can be easily composed and combined to create complex UIs.
2. Virtual DOM: ReactJS’s Virtual DOM allows for efficient updates to the UI by only re-rendering the necessary parts. This results in better performance and a smoother user experience.
3. Declarative Syntax: ReactJS uses a declarative syntax, where developers describe what the UI should look like based on its current state. This makes it easier to understand and reason about the code.
4. One-way Data Flow: ReactJS follows a one-way data flow, where data is passed from parent components to child components as props. This makes it easier to track and manage data flow within an application.
5. Rich Ecosystem: ReactJS has a vibrant ecosystem with a wide range of libraries, tools, and community support. This makes it easier to find solutions to common problems and accelerates development.
Here’s an example of a ReactJS component that demonstrates some of these benefits:
import React from 'react'; const Greeting = ({ name }) => { return <h1>Hello, {name}!</h1>; }; const App = () => { return ( <div> <Greeting name="John" /> <Greeting name="Jane" /> </div> ); }; export default App;
In this example, we have a Greeting
component that accepts a name
prop and displays a personalized greeting. The App
component then renders two instances of the Greeting
component with different names. This demonstrates the reusability of components and the one-way data flow in ReactJS.
Building a Full Application with ReactJS
ReactJS can be used to build full applications, not just UI components. With the help of additional libraries and tools, ReactJS applications can handle routing, manage state, and interact with APIs.
To build a full application with ReactJS, you can follow these steps:
1. Project Setup: Set up a new ReactJS project using tools like Create React App or manually configure your build system.
2. Component Hierarchy: Design the component hierarchy of your application. Identify the main components that will represent different sections or pages of your application.
3. State Management: Decide on a state management solution for your application. ReactJS provides its own state management through component state, but for larger applications, you might consider using a library like Redux or MobX.
4. API Integration: If your application needs to interact with APIs, set up API integration using libraries like axios or fetch. Create service modules to encapsulate API calls and handle data manipulation.
5. Routing: Implement routing in your application using a library like React Router. Define routes for different sections or pages of your application and update the UI accordingly.
6. Styling: Apply styles to your application using CSS or a CSS-in-JS solution like styled-components. Use CSS frameworks or design systems to speed up the styling process.
7. Testing: Write tests for your components and application logic using testing libraries like Jest and React Testing Library. Test different scenarios and edge cases to ensure the reliability of your application.
8. Deployment: Deploy your ReactJS application to a hosting platform like Netlify, Vercel, or AWS. Configure your build process to optimize and bundle your application for production.
Here’s an example of a full ReactJS application structure:
my-app/ README.md node_modules/ package.json public/ index.html favicon.ico src/ components/ Header.js Footer.js HomePage.js AboutPage.js services/ api.js App.js index.js styles.css utils.js
In this example, we have a basic file structure for a ReactJS application. The src
directory contains the main components, services for API integration, and other utility files. The public
directory contains the HTML template and other static assets. The components
directory contains the individual components that make up the application.
Understanding JSX in ReactJS
JSX is a syntax extension for JavaScript that allows you to write HTML-like code within JavaScript. It is a fundamental part of ReactJS and is used to define the structure and appearance of components.
JSX code is transpiled to plain JavaScript code by the Babel compiler, which is then executed by the JavaScript engine. This allows you to write expressive and declarative code in a familiar HTML-like syntax.
Here’s an example of JSX code:
import React from 'react'; const App = () => { return <h1>Hello, ReactJS!</h1>; }; export default App;
In this example, we have a simple functional component called App
that returns a JSX expression. The JSX expression <h1>Hello, ReactJS!</h1>
represents an HTML-like element that will be rendered as a heading with the text “Hello, ReactJS!”.
JSX supports embedding JavaScript expressions within curly braces {}
. This allows you to dynamically generate content and include variables or function calls within JSX elements.
Here’s an example of JSX code with embedded JavaScript expressions:
import React from 'react'; const App = () => { const name = 'John'; return <h1>Hello, {name}!</h1>; }; export default App;
In this example, the JSX expression <h1>Hello, {name}!</h1>
includes the JavaScript expression {name}
within curly braces. This will render the value of the name
variable within the heading element.
JSX also allows for the use of custom components as JSX elements. These custom components can be defined using either classes or functions.
Here’s an example of JSX code with a custom component:
import React from 'react'; const Button = ({ onClick, children }) => { return <button onClick={onClick}>{children}</button>; }; const App = () => { const handleClick = () => { console.log('Button clicked!'); }; return ( <div> <h1>Hello, ReactJS!</h1> <Button onClick={handleClick}>Click me</Button> </div> ); }; export default App;
In this example, we have a custom component called Button
that accepts an onClick
prop and children
prop. The Button
component renders a button element with the provided onClick
prop as the event handler and the children
prop as the button label.
The App
component then uses the Button
component as a JSX element, passing in the handleClick
function as the onClick
prop and the text “Click me” as the children
prop.
Related Article: The Mechanisms Behind ReactJS's High-Speed Performance
Exploring the Role of Virtual DOM in ReactJS
The Virtual DOM is a key concept in ReactJS that allows for efficient updates to the actual DOM. It is a lightweight copy of the actual DOM and serves as a representation of the HTML structure of a web page.
When a React component is rendered, it creates a corresponding Virtual DOM representation. This Virtual DOM is then compared to the previous Virtual DOM representation to identify the minimal set of changes needed to update the actual DOM.
The Virtual DOM reconciliation process in ReactJS involves three steps:
1. Render: When a component’s state or props change, ReactJS re-renders the component and creates a new Virtual DOM representation.
2. Diffing: ReactJS compares the new Virtual DOM representation with the previous one to identify the differences between them. This process is known as diffing.
3. Updating: ReactJS updates the actual DOM based on the identified differences. Only the necessary parts of the DOM are updated, resulting in optimized and efficient updates.
The use of Virtual DOM in ReactJS brings several benefits:
1. Performance: By only updating the necessary parts of the DOM, ReactJS minimizes the amount of work required to update the UI. This leads to better performance and a smoother user experience.
2. Efficiency: The diffing algorithm used by ReactJS is highly efficient, allowing for fast comparisons between Virtual DOM representations. This enables ReactJS to quickly identify the minimal set of changes needed to update the actual DOM.
3. Abstraction: The Virtual DOM acts as an abstraction layer between the developer and the actual DOM. Developers can work with a lightweight and simplified representation of the DOM, making it easier to reason about and manipulate the UI.
Here’s an example to illustrate the role of Virtual DOM in ReactJS:
import React from 'react'; class Counter extends React.Component { constructor(props) { super(props); this.state = { count: 0 }; } incrementCount() { this.setState({ count: this.state.count + 1 }); } render() { return ( <div> <h2>Counter: {this.state.count}</h2> <button onClick={() => this.incrementCount()}>Increment</button> </div> ); } } export default Counter;
In this example, we have a Counter
component that maintains a count state. The incrementCount
method is called when the button is clicked, updating the count state and triggering a re-render of the component.
When the render
method is called, ReactJS creates a new Virtual DOM representation of the Counter
component. It then compares this new Virtual DOM with the previous one to identify the minimal set of changes needed to update the actual DOM.
In this case, ReactJS would identify that the text content of the h2
element needs to be updated with the new count value. Only that specific part of the DOM would be updated, resulting in an optimized and efficient update.
Creating Mobile Apps with ReactJS
ReactJS can be used to create mobile apps using React Native, a framework based on ReactJS for building native mobile applications for iOS and Android platforms.
React Native allows developers to write mobile apps using a combination of JavaScript and native code. It provides a set of components and APIs that are specific to each platform, allowing for a native look and feel.
To create a mobile app with React Native, you can follow these steps:
1. Environment Setup: Set up your development environment by installing Node.js, React Native CLI, and a mobile development SDK like Xcode for iOS or Android Studio for Android.
2. Project Initialization: Create a new React Native project using the React Native CLI. This will generate the necessary files and dependencies for your project.
3. Component Development: Design and develop the components for your mobile app using React Native’s component system. Use components like View
, Text
, Image
, and others to build the UI.
4. API Integration: If your mobile app needs to interact with APIs, set up API integration using libraries like axios or fetch. Create service modules to encapsulate API calls and handle data manipulation.
5. Styling: Apply styles to your mobile app using CSS-like stylesheets. React Native provides a StyleSheet API for defining styles that are specific to mobile platforms.
6. Navigation: Implement navigation in your mobile app using a navigation library like React Navigation. Define screens and navigation routes to enable users to navigate between different sections of your app.
7. Testing: Test your mobile app using tools like the React Native Testing Library. Write unit tests and integration tests to ensure the reliability of your app.
8. Build and Deployment: Build your mobile app for iOS and Android platforms using the respective build tools. Deploy your app to the app stores or distribute it using Over-The-Air (OTA) updates.
Here’s an example of a simple mobile app built with React Native:
import React from 'react'; import { View, Text, Button } from 'react-native'; const App = () => { const handleClick = () => { console.log('Button clicked!'); }; return ( <View> <Text>Hello, React Native!</Text> <Button title="Click me" onPress={handleClick} /> </View> ); }; export default App;
In this example, we have a simple mobile app that displays a text message and a button. When the button is clicked, the handleClick
function is called, logging a message to the console.
React Native provides a set of components like View
, Text
, and Button
that have styling and behavior specific to mobile platforms. These components allow you to build mobile UIs that look and feel native to each platform.
Handling Routing in a ReactJS Application
Routing is an essential part of many web applications, allowing users to navigate between different sections or pages. ReactJS provides several libraries and approaches for handling routing in a ReactJS application.
One popular routing library for ReactJS is React Router. React Router provides a declarative way to define routes and handle navigation within a ReactJS application.
To handle routing in a ReactJS application using React Router, you can follow these steps:
1. Installation: Install React Router using npm or yarn.
npm install react-router-dom
2. Router Setup: Wrap your application with the BrowserRouter
component from React Router. This sets up the routing context for your application.
import React from 'react'; import { BrowserRouter as Router } from 'react-router-dom'; const App = () => { return ( <Router> {/* Your application components */} </Router> ); }; export default App;
3. Route Definition: Use the Route
component from React Router to define routes within your application. Each route should have a path
prop that defines the URL path for that route and a component
prop that specifies the component to render for that route.
import React from 'react'; import { Route } from 'react-router-dom'; const Home = () => { return <h1>Home Page</h1>; }; const About = () => { return <h1>About Page</h1>; }; const App = () => { return ( <Router> <Route path="/" component={Home} /> <Route path="/about" component={About} /> </Router> ); }; export default App;
4. Navigation: Use the Link
component from React Router to create links to different routes within your application. The to
prop of the Link
component specifies the target route.
import React from 'react'; import { Link } from 'react-router-dom'; const Navigation = () => { return ( <nav> <ul> <li> <Link to="/">Home</Link> </li> <li> <Link to="/about">About</Link> </li> </ul> </nav> ); }; const App = () => { return ( <Router> <Navigation /> <Route path="/" component={Home} /> <Route path="/about" component={About} /> </Router> ); }; export default App;
5. Nested Routes: React Router supports nested routes, allowing you to define routes within routes. This is useful for creating complex application structures with nested components.
import React from 'react'; import { Route } from 'react-router-dom'; const UserProfile = () => { return <h1>User Profile</h1>; }; const UserPosts = () => { return <h1>User Posts</h1>; }; const User = () => { return ( <div> <h1>User Page</h1> <Route path="/user/profile" component={UserProfile} /> <Route path="/user/posts" component={UserPosts} /> </div> ); }; const App = () => { return ( <Router> <Route path="/user" component={User} /> </Router> ); }; export default App;
React Router provides many other features, such as route parameters, route guarding, and programmatic navigation. Refer to the React Router documentation for more information on these advanced features.
Related Article: How to Implement onClick Functions in ReactJS
Understanding Redux and its Relationship with ReactJS
Redux is a state management library for JavaScript applications, including ReactJS applications. It provides a predictable state container that helps manage the state of an application in a centralized manner.
ReactJS and Redux work well together, as Redux can be used as the state management solution for ReactJS applications. Redux follows a unidirectional data flow pattern, which aligns with ReactJS’s one-way data flow.
To use Redux with ReactJS, you can follow these steps:
1. Installation: Install Redux using npm or yarn.
npm install redux react-redux
2. Store Setup: Create a Redux store, which holds the state of your application. The store is created using the createStore
function from Redux, passing in a reducer function that specifies how the state should be updated.
import { createStore } from 'redux'; const initialState = { count: 0, }; const reducer = (state = initialState, action) => { switch (action.type) { case 'INCREMENT': return { ...state, count: state.count + 1 }; case 'DECREMENT': return { ...state, count: state.count - 1 }; default: return state; } }; const store = createStore(reducer);
3. Provider Component: Wrap your ReactJS application with the Provider
component from the react-redux
library. This makes the Redux store available to all components in your application.
import React from 'react'; import { Provider } from 'react-redux'; const App = () => { return ( <Provider store={store}> {/* Your application components */} </Provider> ); }; export default App;
4. Connect Function: Use the connect
function from react-redux
to connect your components to the Redux store. This allows your components to access the state and dispatch actions to update the state.
import React from 'react'; import { connect } from 'react-redux'; const Counter = ({ count, increment, decrement }) => { return ( <div> <h2>Counter: {count}</h2> <button onClick={increment}>Increment</button> <button onClick={decrement}>Decrement</button> </div> ); }; const mapStateToProps = (state) => { return { count: state.count, }; }; const mapDispatchToProps = (dispatch) => { return { increment: () => dispatch({ type: 'INCREMENT' }), decrement: () => dispatch({ type: 'DECREMENT' }), }; }; export default connect(mapStateToProps, mapDispatchToProps)(Counter);
In this example, we have a Counter
component that displays a count value from the Redux store and provides buttons to increment and decrement the count. The mapStateToProps
function maps the count value from the store’s state to the component’s props, while the mapDispatchToProps
function maps the increment and decrement actions to the component’s props.
The connect
function then connects the Counter
component to the Redux store, allowing it to access the state and dispatch actions.
Redux provides additional features such as middleware, selectors, and asynchronous actions. Refer to the Redux documentation for more information on these advanced features.
Popular Libraries and Frameworks Compatible with ReactJS
ReactJS has a rich ecosystem of libraries and frameworks that are compatible and often used alongside it. These libraries and frameworks provide additional functionality and enhance the development experience of ReactJS applications.
Some popular libraries and frameworks compatible with ReactJS are:
1. React Router: React Router is a routing library for ReactJS applications. It provides a declarative way to handle navigation and define routes within a ReactJS application.
2. Redux: Redux is a state management library for JavaScript applications, including ReactJS applications. It provides a predictable state container that helps manage the state of an application in a centralized manner.
3. Axios: Axios is a popular JavaScript library for making HTTP requests. It provides an easy-to-use API for interacting with APIs and handling data in ReactJS applications.
4. Styled-components: Styled-components is a CSS-in-JS library for ReactJS. It allows you to write CSS code as JavaScript components, making it easier to manage styles and create dynamic stylesheets.
5. Formik: Formik is a form library for ReactJS applications. It simplifies form handling, validation, and submission, providing a better user experience for form interactions.
6. Material-UI: Material-UI is a popular UI component library for ReactJS. It provides a set of pre-designed, customizable components that follow the Material Design guidelines.
7. Jest: Jest is a JavaScript testing framework that is widely used for testing ReactJS applications. It provides a simple and useful API for writing tests and running test suites.
8. React Native: React Native is a framework based on ReactJS for building native mobile applications. It allows you to write mobile apps using a combination of JavaScript and native code, targeting iOS and Android platforms.
These are just a few examples of the many libraries and frameworks compatible with ReactJS. The ReactJS ecosystem is constantly evolving, and new libraries and frameworks are being developed to enhance the development experience and provide solutions to common problems.
Additional Resources
– Technologies to Learn Alongside React
– React State Management Tutorial – How React Handles State