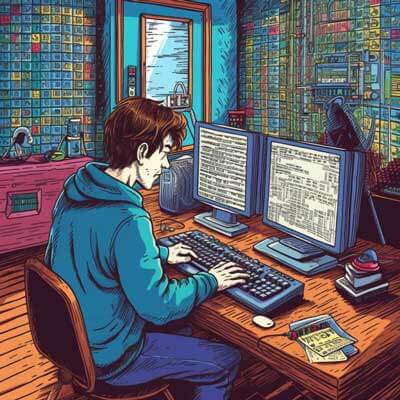
- Reactstrap Components
- Reactstrap Navbar
- Reactstrap Modal
- Reactstrap Form
- Reactstrap Grid
- Reactstrap Buttons
- Reactstrap Cards
- Reactstrap Installation
- Reactstrap Documentation
- How to Install and Use Reactstrap
- Examples of Reactstrap Components
- Example 1: Button
- Example 2: Card
- Finding Reactstrap Documentation
- Creating a Navbar with Reactstrap
- Implementing a Modal with Reactstrap
- Syntax for Creating Forms with Reactstrap
- Using the Grid System in Reactstrap
- Styling Buttons with Reactstrap
- Examples of Reactstrap Cards
- Differences between Reactstrap and Regular Bootstrap
- Additional Resources
Reactstrap Components
Reactstrap is a library that provides a set of Bootstrap 4 components that can be used with React. These components are pre-styled and ready to use, making it easy to create beautiful and responsive user interfaces in ReactJS. Some of the commonly used Reactstrap components include:
– Navbar: A navigation bar component that can be customized with various options such as positioning, color schemes, and responsive behavior.
– Modal: A pop-up window component that can be used to display additional information or capture user input.
– Form: A set of form components such as inputs, checkboxes, and selects that can be used to build interactive forms.
– Grid: A grid system that allows you to create responsive layouts by dividing the page into rows and columns.
– Buttons: A set of button components that can be customized with various styles and sizes.
– Cards: A component that can be used to display content in a card-like format with an image, title, and description.
Related Article: How to Build Forms in React
Reactstrap Navbar
The Reactstrap Navbar component is a versatile component that allows you to create navigation bars with ease. It provides various options for customization, such as positioning, color schemes, and responsive behavior.
Here’s an example of how to create a basic navbar using Reactstrap:
import React from 'react'; import { Navbar, NavbarBrand, Nav, NavItem, NavLink } from 'reactstrap'; const AppNavbar = () => { return ( <Navbar color="light" light expand="md"> <NavbarBrand href="/">My App</NavbarBrand> <Nav className="ml-auto" navbar> <NavItem> <NavLink href="/about">About</NavLink> </NavItem> <NavItem> <NavLink href="/contact">Contact</NavLink> </NavItem> </Nav> </Navbar> ); } export default AppNavbar;
In the above example, we import the necessary components from Reactstrap and use them to create a Navbar component. We set the color prop to “light” and the expand prop to “md” to make the navbar responsive. Inside the Navbar component, we have a NavbarBrand component for the logo or brand name and a Nav component with NavItem and NavLink components for the navigation links.
Reactstrap Modal
The Reactstrap Modal component is a pop-up window that can be used to display additional information or capture user input. It provides a simple and customizable way to create modals in ReactJS.
Here’s an example of how to create a basic modal using Reactstrap:
import React, { useState } from 'react'; import { Button, Modal, ModalHeader, ModalBody, ModalFooter } from 'reactstrap'; const ModalExample = () => { const [isOpen, setIsOpen] = useState(false); const toggleModal = () => { setIsOpen(!isOpen); } return ( <div> <Button color="primary" onClick={toggleModal}>Open Modal</Button> <Modal isOpen={isOpen} toggle={toggleModal}> <ModalHeader toggle={toggleModal}>Modal Title</ModalHeader> <ModalBody> Lorem ipsum dolor sit amet, consectetur adipiscing elit. Nullam id ligula ac nisi ullamcorper aliquam. </ModalBody> <ModalFooter> <Button color="secondary" onClick={toggleModal}>Close</Button> <Button color="primary">Save Changes</Button> </ModalFooter> </Modal> </div> ); } export default ModalExample;
In the above example, we use the useState hook to manage the state of the modal. The isOpen state variable determines whether the modal is open or closed. We use the toggleModal function to toggle the state of the modal when the button is clicked.
Inside the Modal component, we have a ModalHeader component for the title, a ModalBody component for the content, and a ModalFooter component for the buttons. The toggle prop is used to close the modal when the close button is clicked.
Reactstrap Form
The Reactstrap Form component provides a set of pre-styled form components that can be used to build interactive forms in ReactJS. These components include inputs, checkboxes, selects, and more.
Here’s an example of how to create a basic form using Reactstrap:
import React from 'react'; import { Form, FormGroup, Label, Input, Button } from 'reactstrap'; const MyForm = () => { return ( <Form> <FormGroup> <Label for="name">Name</Label> <Input type="text" name="name" id="name" placeholder="Enter your name" /> </FormGroup> <FormGroup> <Label for="email">Email</Label> <Input type="email" name="email" id="email" placeholder="Enter your email" /> </FormGroup> <FormGroup> <Label for="message">Message</Label> <Input type="textarea" name="message" id="message" placeholder="Enter your message" /> </FormGroup> <Button color="primary">Submit</Button> </Form> ); } export default MyForm;
In the above example, we import the necessary components from Reactstrap and use them to create a Form component. Inside the form, we use FormGroup components to group form elements together. Each FormGroup component contains a Label component for the form field label and an Input component for the form field itself. We can set various attributes on the Input component, such as type, name, id, and placeholder.
Finally, we have a Button component for the submit button.
Related Article: Handling Routing in React Apps with React Router
Reactstrap Grid
The Reactstrap Grid system allows you to create responsive layouts by dividing the page into rows and columns. It provides a flexible and easy-to-use way to create grid-based layouts in ReactJS.
Here’s an example of how to create a basic grid layout using Reactstrap:
import React from 'react'; import { Container, Row, Col } from 'reactstrap'; const MyGrid = () => { return ( <Container> <Row> <Col sm="6">Column 1</Col> <Col sm="6">Column 2</Col> </Row> </Container> ); } export default MyGrid;
In the above example, we import the necessary components from Reactstrap and use them to create a Container component. Inside the container, we have a Row component that represents a row of columns. Inside the Row component, we have two Col components that represent two columns.
We set the sm prop on the Col components to “6” to make each column take up half of the row on small screens. This ensures that the columns stack vertically on small screens.
Reactstrap Buttons
The Reactstrap Buttons component provides a set of pre-styled button components that can be customized with various styles and sizes. These components are easy to use and can be integrated into your ReactJS applications with ease.
Here’s an example of how to create a basic button using Reactstrap:
import React from 'react'; import { Button } from 'reactstrap'; const MyButton = () => { return ( <Button color="primary">Click me</Button> ); } export default MyButton;
In the above example, we import the Button component from Reactstrap and use it to create a button. We set the color prop to “primary” to give the button a primary color scheme.
Reactstrap Buttons also support additional props for customization, such as size, outline, and disabled.
Reactstrap Cards
The Reactstrap Cards component allows you to display content in a card-like format with an image, title, and description. Cards are a versatile component that can be used to display various types of content in a visually appealing way.
Here’s an example of how to create a basic card using Reactstrap:
import React from 'react'; import { Card, CardImg, CardText, CardBody, CardTitle, CardSubtitle, Button } from 'reactstrap'; const MyCard = () => { return ( <Card> <CardImg top width="100%" src="/path/to/image.jpg" alt="Card image cap" /> <CardBody> <CardTitle>Card Title</CardTitle> <CardSubtitle>Card Subtitle</CardSubtitle> <CardText>Lorem ipsum dolor sit amet, consectetur adipiscing elit.</CardText> <Button>Learn More</Button> </CardBody> </Card> ); } export default MyCard;
In the above example, we import the necessary components from Reactstrap and use them to create a Card component. Inside the Card component, we have a CardImg component for the image, a CardBody component for the card content, and various other components such as CardTitle, CardSubtitle, and CardText for the title, subtitle, and text respectively.
We can also add buttons or other components inside the CardBody component to provide additional functionality.
Related Article: Exploring Differences in Rendering Components in ReactJS
Reactstrap Installation
To start using Reactstrap in your ReactJS project, you need to install it first. Reactstrap can be installed using npm or yarn, depending on your preference.
Here’s how to install Reactstrap using npm:
1. Open your terminal or command prompt.
2. Navigate to your project directory.
3. Run the following command to install Reactstrap and its dependencies:
npm install reactstrap bootstrap
4. Once the installation is complete, you can import the required components from Reactstrap and start using them in your project.
Here’s how to install Reactstrap using yarn:
1. Open your terminal or command prompt.
2. Navigate to your project directory.
3. Run the following command to install Reactstrap and its dependencies:
yarn add reactstrap bootstrap
4. Once the installation is complete, you can import the required components from Reactstrap and start using them in your project.
Reactstrap Documentation
Reactstrap provides comprehensive documentation that covers all the available components and their usage. The documentation includes detailed examples, code snippets, and explanations of each component’s props and usage.
You can find the Reactstrap documentation at the following URL: Reactstrap Documentation
The documentation is divided into sections, each focusing on a specific component or feature. You can navigate through the documentation to find the component or feature you are interested in and learn more about its usage.
The Reactstrap documentation also includes a live code editor that allows you to experiment with the components and see the results in real-time.
How to Install and Use Reactstrap
To install and use Reactstrap in your ReactJS project, follow these steps:
1. Make sure you have Node.js and npm (Node Package Manager) installed on your system.
2. Create a new React project or navigate to an existing React project directory in your terminal or command prompt.
3. Install Reactstrap and Bootstrap by running the following command:
npm install reactstrap bootstrap
4. Once the installation is complete, you can start using Reactstrap components in your project.
5. Import the desired Reactstrap components into your React component file. For example, to use the Button component, add the following line at the top of your file:
import { Button } from 'reactstrap';
6. You can now use the imported component in your JSX code. For example, to render a primary button, you can use the following code:
<Button color="primary">Click me</Button>
7. Customize the component by passing different props. Reactstrap components have various props that allow you to customize their appearance and behavior. Refer to the Reactstrap documentation for a complete list of props for each component.
8. Save your changes and run your React app to see the Reactstrap component in action.
Related Article: How To Pass Parameters to Components in ReactJS & TypeScript
Examples of Reactstrap Components
Here are a few examples of Reactstrap components and their usage:
Example 1: Button
import React from 'react'; import { Button } from 'reactstrap'; const MyButton = () => { return ( <Button color="primary">Click me</Button> ); } export default MyButton;
In this example, we import the Button component from Reactstrap and use it to render a primary button with the label “Click me”.
Example 2: Card
import React from 'react'; import { Card, CardImg, CardText, CardBody, CardTitle, CardSubtitle, Button } from 'reactstrap'; const MyCard = () => { return ( <Card> <CardImg top width="100%" src="/path/to/image.jpg" alt="Card image cap" /> <CardBody> <CardTitle>Card Title</CardTitle> <CardSubtitle>Card Subtitle</CardSubtitle> <CardText>Lorem ipsum dolor sit amet, consectetur adipiscing elit.</CardText> <Button>Learn More</Button> </CardBody> </Card> ); } export default MyCard;
In this example, we import the necessary components from Reactstrap and use them to render a card with an image, title, subtitle, text, and a button.
Related Article: Extracting URL Parameters in Your ReactJS Component
Finding Reactstrap Documentation
The Reactstrap documentation is a valuable resource that provides detailed information about each component, including examples, code snippets, and explanations of each component’s props and usage.
To find the Reactstrap documentation:
1. Open your web browser and go to the following URL: Reactstrap Documentation
2. Once you are on the Reactstrap documentation page, you can navigate through the different sections to find the component or feature you are interested in.
3. Each component section provides an overview of the component’s purpose and usage, along with examples and code snippets.
4. You can also use the search bar at the top of the page to search for specific keywords or component names.
5. The Reactstrap documentation also includes a live code editor that allows you to experiment with the components and see the results in real-time.
6. Take advantage of the documentation to learn about the various Reactstrap components and how to use them effectively in your ReactJS projects.
Creating a Navbar with Reactstrap
The Reactstrap Navbar component allows you to create a navigation bar with ease. It provides various options for customization, such as positioning, color schemes, and responsive behavior.
Here’s an example of how to create a basic navbar using Reactstrap:
import React from 'react'; import { Navbar, NavbarBrand, Nav, NavItem, NavLink } from 'reactstrap'; const AppNavbar = () => { return ( <Navbar color="light" light expand="md"> <NavbarBrand href="/">My App</NavbarBrand> <Nav className="ml-auto" navbar> <NavItem> <NavLink href="/about">About</NavLink> </NavItem> <NavItem> <NavLink href="/contact">Contact</NavLink> </NavItem> </Nav> </Navbar> ); } export default AppNavbar;
In this example, we import the necessary components from Reactstrap and use them to create a Navbar component. We set the color prop to “light” and the expand prop to “md” to make the navbar responsive.
Inside the Navbar component, we have a NavbarBrand component for the logo or brand name and a Nav component with NavItem and NavLink components for the navigation links.
You can customize the navbar further by adding more NavItem and NavLink components or by adding additional props to the Navbar component, such as fixed or sticky positioning.
Implementing a Modal with Reactstrap
The Reactstrap Modal component is a pop-up window that can be used to display additional information or capture user input. It provides a simple and customizable way to create modals in ReactJS.
Here’s an example of how to create a basic modal using Reactstrap:
import React, { useState } from 'react'; import { Button, Modal, ModalHeader, ModalBody, ModalFooter } from 'reactstrap'; const ModalExample = () => { const [isOpen, setIsOpen] = useState(false); const toggleModal = () => { setIsOpen(!isOpen); } return ( <div> <Button color="primary" onClick={toggleModal}>Open Modal</Button> <Modal isOpen={isOpen} toggle={toggleModal}> <ModalHeader toggle={toggleModal}>Modal Title</ModalHeader> <ModalBody> Lorem ipsum dolor sit amet, consectetur adipiscing elit. Nullam id ligula ac nisi ullamcorper aliquam. </ModalBody> <ModalFooter> <Button color="secondary" onClick={toggleModal}>Close</Button> <Button color="primary">Save Changes</Button> </ModalFooter> </Modal> </div> ); } export default ModalExample;
In this example, we use the useState hook to manage the state of the modal. The isOpen state variable determines whether the modal is open or closed. We use the toggleModal function to toggle the state of the modal when the button is clicked.
Inside the Modal component, we have a ModalHeader component for the title, a ModalBody component for the content, and a ModalFooter component for the buttons. The toggle prop is used to close the modal when the close button is clicked.
You can customize the modal further by adding more components or props, such as size, backdrop, or keyboard behavior.
Related Article: Implementing onclick Events in Child Components with ReactJS
Syntax for Creating Forms with Reactstrap
The Reactstrap Form component provides a set of pre-styled form components that can be used to build interactive forms in ReactJS. These components include inputs, checkboxes, selects, and more.
Here’s an example of how to create a basic form using Reactstrap:
import React from 'react'; import { Form, FormGroup, Label, Input, Button } from 'reactstrap'; const MyForm = () => { return ( <Form> <FormGroup> <Label for="name">Name</Label> <Input type="text" name="name" id="name" placeholder="Enter your name" /> </FormGroup> <FormGroup> <Label for="email">Email</Label> <Input type="email" name="email" id="email" placeholder="Enter your email" /> </FormGroup> <FormGroup> <Label for="message">Message</Label> <Input type="textarea" name="message" id="message" placeholder="Enter your message" /> </FormGroup> <Button color="primary">Submit</Button> </Form> ); } export default MyForm;
In this example, we import the necessary components from Reactstrap and use them to create a Form component. Inside the form, we use FormGroup components to group form elements together. Each FormGroup component contains a Label component for the form field label and an Input component for the form field itself.
We can set various attributes on the Input component, such as type, name, id, and placeholder.
Finally, we have a Button component for the submit button.
You can customize the form further by adding more form elements or by adding additional props to the Form component, such as inline or horizontal layout.
Using the Grid System in Reactstrap
The Reactstrap Grid system allows you to create responsive layouts by dividing the page into rows and columns. It provides a flexible and easy-to-use way to create grid-based layouts in ReactJS.
Here’s an example of how to create a basic grid layout using Reactstrap:
import React from 'react'; import { Container, Row, Col } from 'reactstrap'; const MyGrid = () => { return ( <Container> <Row> <Col sm="6">Column 1</Col> <Col sm="6">Column 2</Col> </Row> </Container> ); } export default MyGrid;
In this example, we import the necessary components from Reactstrap and use them to create a Container component. Inside the container, we have a Row component that represents a row of columns. Inside the Row component, we have two Col components that represent two columns.
We set the sm prop on the Col components to “6” to make each column take up half of the row on small screens. This ensures that the columns stack vertically on small screens.
You can customize the grid further by adding more rows and columns or by adding additional props to the Container, Row, or Col components, such as gutter spacing or column ordering.
Styling Buttons with Reactstrap
The Reactstrap Buttons component provides a set of pre-styled button components that can be customized with various styles and sizes. These components are easy to use and can be integrated into your ReactJS applications with ease.
Here’s an example of how to create a basic button using Reactstrap:
import React from 'react'; import { Button } from 'reactstrap'; const MyButton = () => { return ( <Button color="primary">Click me</Button> ); } export default MyButton;
In this example, we import the Button component from Reactstrap and use it to create a button. We set the color prop to “primary” to give the button a primary color scheme.
Reactstrap Buttons also support additional props for customization, such as size, outline, and disabled.
You can customize the button further by adding additional props or by using CSS classes or custom styles.
Related Article: How to Add Navbar Components for Different Pages in ReactJS
Examples of Reactstrap Cards
The Reactstrap Cards component allows you to display content in a card-like format with an image, title, and description. Cards are a versatile component that can be used to display various types of content in a visually appealing way.
Here’s an example of how to create a basic card using Reactstrap:
import React from 'react'; import { Card, CardImg, CardText, CardBody, CardTitle, CardSubtitle, Button } from 'reactstrap'; const MyCard = () => { return ( <Card> <CardImg top width="100%" src="/path/to/image.jpg" alt="Card image cap" /> <CardBody> <CardTitle>Card Title</CardTitle> <CardSubtitle>Card Subtitle</CardSubtitle> <CardText>Lorem ipsum dolor sit amet, consectetur adipiscing elit.</CardText> <Button>Learn More</Button> </CardBody> </Card> ); } export default MyCard;
In this example, we import the necessary components from Reactstrap and use them to create a Card component. Inside the Card component, we have a CardImg component for the image, a CardBody component for the card content, and various other components such as CardTitle, CardSubtitle, and CardText for the title, subtitle, and text respectively.
We can also add buttons or other components inside the CardBody component to provide additional functionality.
You can customize the card further by adding additional components or by using CSS classes or custom styles.
Differences between Reactstrap and Regular Bootstrap
Reactstrap is a library that provides a set of Bootstrap 4 components that can be used with React. While Reactstrap is based on Bootstrap, there are some key differences between the two.
1. Syntax: Reactstrap uses JSX syntax, which is a combination of JavaScript and HTML, to define components and their props. Regular Bootstrap uses HTML and CSS classes to define components and their styles.
2. React Integration: Reactstrap is specifically designed to work with ReactJS, making it easy to integrate Bootstrap components into React-based applications. Regular Bootstrap can be used with any web framework or even with plain HTML and JavaScript.
3. Componentization: Reactstrap components are modular and can be easily reused and composed to build complex user interfaces. Regular Bootstrap components are not as modular and often require additional customization using CSS classes.
4. State Management: Reactstrap components can easily manage their own state using React’s state and lifecycle hooks. Regular Bootstrap components do not have built-in state management and rely on JavaScript frameworks or libraries for state management.
5. Customization: Reactstrap components can be easily customized using props and CSS-in-JS libraries. Regular Bootstrap components can be customized using CSS classes and custom stylesheets.
6. Performance: Reactstrap components are optimized for performance and use React’s virtual DOM to efficiently update the UI. Regular Bootstrap components may not be as performant when used in complex and dynamic applications.
It’s important to note that while Reactstrap provides a convenient way to use Bootstrap components in React, it may not cover all the features and functionality of regular Bootstrap. If you need to use specific Bootstrap features that are not available in Reactstrap, you may need to use regular Bootstrap alongside Reactstrap or find alternative solutions.
Additional Resources
– Reactstrap – Easy to use React Bootstrap 4 components
– Reactstrap – GitHub Repository
– Styled Components – CSS in JS