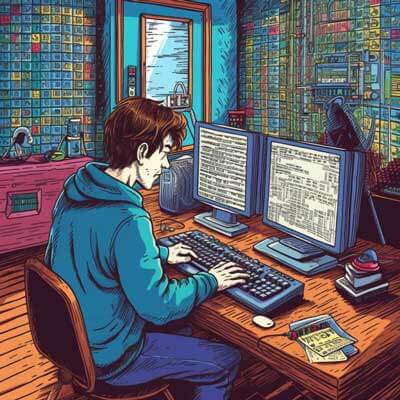
React Components
React is a JavaScript library for building user interfaces. At the core of React development are components, which are reusable, self-contained pieces of code that encapsulate a specific functionality or UI element. Components can be simple or complex, and they can be composed together to create larger, more sophisticated applications.
React components can be classified into two main types: functional components and class components.
Related Article: What Are The Benefits of Using ReactJS in Web Development?
Functional Components
Functional components are JavaScript functions that accept props (short for properties) as arguments and return React elements. They are stateless and can be written as plain JavaScript functions or arrow functions.
Here’s an example of a functional component that renders a simple “Hello, World!” message:
function HelloWorld() { return <div>Hello, World!</div>; }
Functional components are easy to write and understand, and they can be used in any React application. However, they don’t have access to React’s lifecycle methods or state management features.
Class Components
Class components are JavaScript classes that extend the React.Component class. They have access to React’s lifecycle methods and state management features, making them more useful and flexible than functional components.
Here’s an example of a class component that renders a simple “Hello, World!” message:
class HelloWorld extends React.Component { render() { return <div>Hello, World!</div>; } }
Class components can have state, which allows them to store and manage data that can change over time. They can also define lifecycle methods, such as componentDidMount and componentDidUpdate, which are called at specific points in the component’s lifecycle.
In general, functional components are recommended for simple UI elements or when you don’t need access to React’s lifecycle methods or state management features. Class components are recommended for more complex UI elements or when you need to manage state or use lifecycle methods.
Virtual DOM
The Virtual DOM is a concept in React that represents the current state of the user interface. It is a lightweight, in-memory representation of the actual DOM (Document Object Model) that React uses to efficiently update and render components.
The Virtual DOM works by creating a virtual representation of the actual DOM tree. When a component’s state or props change, React updates the Virtual DOM instead of the actual DOM. React then compares the updated Virtual DOM with the previous Virtual DOM and determines the minimal set of changes needed to update the actual DOM. This process is known as reconciliation.
Here’s an example of how the Virtual DOM works:
// Initial render const virtualDom = ( <div> <h1>Hello, World!</h1> <p>Welcome to React!</p> </div> ); ReactDOM.render(virtualDom, document.getElementById('root')); // Update const updatedVirtualDom = ( <div> <h1>Hello, React!</h1> <p>Welcome to the Virtual DOM!</p> </div> ); ReactDOM.render(updatedVirtualDom, document.getElementById('root'));
In this example, React updates the Virtual DOM based on the updatedVirtualDom, and then efficiently updates the actual DOM with the necessary changes. This process is much faster than directly manipulating the actual DOM for every change.
Related Article: The Mechanisms Behind ReactJS's High-Speed Performance
JSX
JSX is a syntax extension for JavaScript that allows you to write HTML-like code within your JavaScript code. It is a key feature of React and is used to define the structure and appearance of components.
JSX is not valid JavaScript, so it needs to be transformed into valid JavaScript before it can be executed. This transformation is typically done by Babel, a JavaScript compiler that supports JSX and other modern JavaScript features.
Here’s an example of JSX code:
const element = <h1>Hello, World!</h1>;
In this example, the JSX code <h1>Hello, World!</h1>
is transformed into the following JavaScript code:
const element = React.createElement('h1', null, 'Hello, World!');
JSX makes it easier to write and understand React components by providing a familiar HTML-like syntax. It allows you to mix JavaScript expressions and HTML-like elements, making it more expressive and concise.
Component Lifecycle
The React component lifecycle refers to the series of methods that are called at different stages of a component’s existence. These methods allow you to perform actions at specific points in a component’s lifecycle, such as when it is first mounted, updated, or unmounted.
The component lifecycle can be divided into three main phases: mounting, updating, and unmounting.
Mounting
The mounting phase occurs when a component is being created and inserted into the DOM for the first time. The following methods are called in order during the mounting phase:
– constructor: This is the first method called when a component is created. It is used to initialize the component’s state and bind event handlers.
– static getDerivedStateFromProps: This method is called before rendering and allows the component to update its state based on changes in props.
– render: This method is responsible for rendering the component’s JSX code or returning null if the component doesn’t need to render anything.
– componentDidMount: This method is called immediately after the component has been inserted into the DOM. It is commonly used to perform side effects, such as fetching data from an API or setting up event listeners.
Related Article: Sharing Variables Between Components in ReactJS
Updating
The updating phase occurs when a component’s state or props change. The following methods are called in order during the updating phase:
– static getDerivedStateFromProps: This method is also called during the updating phase, similar to the mounting phase. It allows the component to update its state based on changes in props.
– shouldComponentUpdate: This method is called before rendering and allows the component to decide whether to proceed with the update or not. It is commonly used to optimize performance by preventing unnecessary updates.
– render: This method is called again to update the component’s JSX code based on the updated state or props.
– componentDidUpdate: This method is called immediately after the component has been updated and re-rendered. It is commonly used to perform side effects based on the updated state or props.
Unmounting
The unmounting phase occurs when a component is being removed from the DOM. The following method is called during the unmounting phase:
– componentWillUnmount: This method is called immediately before the component is unmounted and destroyed. It is commonly used to clean up any resources or event listeners created during the component’s lifecycle.
The component lifecycle methods provide a way to hook into specific points of a component’s existence and perform actions accordingly. By using these methods effectively, you can control the behavior and functionality of your React components.
Functional Components vs Class Components
Functional components and class components are two types of components in React that serve different purposes and have different features.
Related Article: ReactJS: How to Re-Render Post Localstorage Clearing
Functional Components
Functional components, as the name suggests, are functions that take in props and return JSX code. They are simple and straightforward to write, and they don’t have access to React’s lifecycle methods or state management features.
Here’s an example of a functional component:
function Greeting(props) { return <div>Hello, {props.name}!</div>; }
Functional components are recommended for simple UI elements or when you don’t need access to React’s lifecycle methods or state management features. They are also easier to test and understand compared to class components.
Class Components
Class components are JavaScript classes that extend the React.Component class. They have access to React’s lifecycle methods and state management features, making them more useful and flexible than functional components.
Here’s an example of a class component:
class Greeting extends React.Component { render() { return <div>Hello, {this.props.name}!</div>; } }
Class components can have state, which allows them to store and manage data that can change over time. They can also define lifecycle methods, such as componentDidMount and componentDidUpdate, which are called at specific points in the component’s lifecycle.
Class components are recommended for more complex UI elements or when you need to manage state or use lifecycle methods. They provide more control and flexibility compared to functional components.
React Render Method
In React, the render method is a required method that every class component must have. It is responsible for rendering the component’s JSX code or returning null if the component doesn’t need to render anything.
The render method is called whenever the component needs to update or re-render, which typically occurs when the component’s state or props change.
Here’s an example of a class component with a render method:
class HelloWorld extends React.Component { render() { return <div>Hello, World!</div>; } }
In this example, the render method returns a JSX element <div>Hello, World!</div>
. This element will be rendered as HTML in the actual DOM.
The render method should be a pure function, meaning it should not modify component state or perform any side effects. It should only return the JSX code that represents the component’s UI.
Related Article: Inserting Plain Text into an Array Using ReactJS
React.createElement
React.createElement is a method provided by React that creates and returns a new React element. It is often used behind the scenes when writing JSX code, as JSX is transformed into React.createElement calls before it can be executed.
The syntax for React.createElement is as follows:
React.createElement(type, props, children);
– type: The type of the element to create. This can be a string representing an HTML tag (e.g., ‘div’), a React component, or a custom element.
– props: An object containing the properties or attributes to assign to the element.
– children: The children of the element, which can be other React elements or plain text.
Here’s an example of using React.createElement to create a simple element:
const element = React.createElement('h1', { className: 'title' }, 'Hello, World!');
In this example, React.createElement creates a new element of type ‘h1’ with the className ‘title’ and the text content ‘Hello, World!’.
React.createElement is useful when you need to create elements dynamically or programmatically, without using JSX. However, JSX is generally preferred for its readability and ease of use.
ReactDOM
ReactDOM is a package provided by React that enables you to render React components into the actual DOM. It provides methods for rendering, updating, and unmounting components.
To use ReactDOM, you need to import it into your JavaScript file:
import ReactDOM from 'react-dom';
Here are some of the key methods provided by ReactDOM:
– ReactDOM.render: This method is used to render a React component into the DOM. It takes two arguments: the React component to render and the DOM element to render it into.
– ReactDOM.hydrate: This method is similar to ReactDOM.render, but it is used for server-side rendering.
– ReactDOM.unmountComponentAtNode: This method is used to unmount a rendered React component from the DOM. It takes a DOM element as an argument.
Here’s an example of using ReactDOM.render to render a component into the DOM:
import React from 'react'; import ReactDOM from 'react-dom'; class HelloWorld extends React.Component { render() { return <div>Hello, World!</div>; } } ReactDOM.render(<HelloWorld />, document.getElementById('root'));
In this example, the HelloWorld component is rendered into the DOM, inside the element with the ID ‘root’.
ReactDOM is an essential part of React development and is used to bridge the gap between the Virtual DOM and the actual DOM, allowing you to create dynamic and interactive user interfaces.