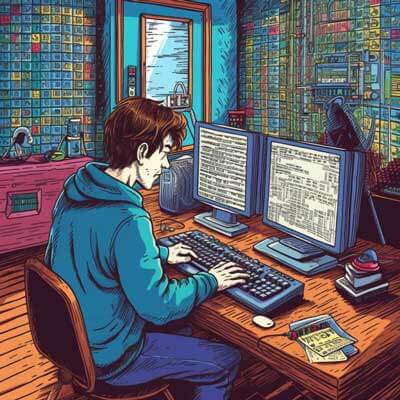
- Overview of GraphQL Error Handling
- Error Policies in GraphQL
- Setting Error Policies in Apollo Client
- Ignoring Errors in Apollo Client
- Handling Errors While Ignoring
- Accessing Partial Data with Ignored Errors
- Logging Ignored Errors
- Client-Side Errors in GraphQL
- Network Errors and Their Impact
- Error Propagation in GraphQL Servers
- Implications of Ignoring Errors
- Best Practices for Error Management
Overview of GraphQL Error Handling
Error handling in GraphQL is essential for building resilient applications. GraphQL allows clients to query data, and sometimes those queries can fail. Unlike traditional REST APIs that often return a simple error status code, GraphQL typically returns a structured error response alongside the data. This means that even if an error occurs, the client may still receive some valid data, making it crucial to understand how to manage these situations effectively.
When a GraphQL request fails, the server responds with an object that contains an “errors” array. Each object in this array provides details about the error, including a message and potentially additional information like locations or path pointers. This structured format makes it easier to identify what went wrong and to decide how to handle the error in the client application.
Related Article: Exploring Directus GraphQL
Error Policies in GraphQL
GraphQL defines a few common error scenarios, each requiring different handling strategies. The primary categories include:
1. Client Errors: These occur when the client sends an invalid query or request. For example, a query might be malformed or contain fields that don’t exist. Handling these errors usually involves notifying the user or logging the issue for further investigation.
2. Server Errors: These result from issues on the server side, such as database failures or server misconfiguration. In these cases, the client might still receive partial data, so handling requires both alerting users and ensuring data integrity.
3. Network Errors: These happen during the communication process, such as timeouts or connectivity issues. Clients must be prepared to retry requests or inform users about the inability to fetch data.
The strategy for handling these errors can vary widely depending on the application’s needs and the user experience desired.
Setting Error Policies in Apollo Client
Apollo Client provides built-in mechanisms for managing errors effectively. The ApolloClient
instance can be configured with error handling policies that dictate how to deal with errors returned from GraphQL server responses.
To set error policies, define the client with a custom link that includes error handling logic. For instance:
// file: client.js import { ApolloClient, InMemoryCache, HttpLink } from '@apollo/client'; const client = new ApolloClient({ link: new HttpLink({ uri: 'https://your-graphql-endpoint.com/graphql' }), cache: new InMemoryCache(), // Error handling policies can be added here });
Integrating error handling directly into the client helps streamline responses to common errors and maintain a smoother user experience.
Ignoring Errors in Apollo Client
In scenarios where an application might want to ignore certain errors, Apollo Client provides mechanisms that allow developers to specify which errors should be disregarded. This might be useful in cases where partial data is acceptable or when specific errors are expected and do not require user intervention.
To ignore specific errors, you can customize your query execution. For example:
// file: query.js import { gql, useQuery } from '@apollo/client'; const GET_DATA = gql` query GetData { items { id name } } `; const MyComponent = () => { const { data, error } = useQuery(GET_DATA, { onError: (error) => { if (error.graphQLErrors) { // Ignore specific errors return; } console.error(error); }, }); return <div>{JSON.stringify(data)}</div>; };
This approach allows developers to filter out errors they don’t want to act upon, enabling a more tailored error management strategy.
Related Article: How to Query Data in GraphQL
Handling Errors While Ignoring
Ignoring errors does not mean that they should be completely forgotten. It is still essential to implement a mechanism for logging or monitoring these ignored errors. This way, developers can keep track of issues that may need attention in the future.
One way to handle errors while ignoring them is to log them to an external service or console. Here’s how to implement this:
// file: logger.js const logError = (error) => { // Log to an external service or console console.error('Ignored error:', error); }; // file: query.js const MyComponent = () => { const { data, error } = useQuery(GET_DATA, { onError: (error) => { if (error.graphQLErrors) { logError(error); return; // Ignore the error } console.error(error); }, }); return <div>{JSON.stringify(data)}</div>; };
This practice ensures that even though certain errors are ignored in the UI, they are still tracked in the background, providing valuable insights into potential issues.
Accessing Partial Data with Ignored Errors
One of the advantages of GraphQL is its ability to return partial data along with error responses. When a query encounters an error, the server can still return any successfully retrieved data. This feature allows applications to remain functional even when some parts of the data cannot be loaded.
In order to access this partial data, you can use the data
object returned by Apollo Client. Here is an example illustrating this concept:
// file: query.js const MyComponent = () => { const { data, error } = useQuery(GET_DATA, { onError: (error) => { if (error.graphQLErrors) { logError(error); return; // Ignore the error } }, }); if (error) { return <div>Error occurred: {error.message}</div>; } return ( <div> <h1>Items List</h1> <ul> {data?.items?.map((item) => ( <li key={item.id}>{item.name}</li> ))} </ul> </div> ); };
This implementation gracefully handles the display of data even when an error has occurred, demonstrating the utility of GraphQL’s partial data feature.
Logging Ignored Errors
Logging is a critical part of error management. By logging ignored errors, developers can maintain visibility into issues that may otherwise go unnoticed. This practice helps in identifying trends or recurring problems that could affect the user experience.
To implement logging for ignored errors, consider using a dedicated logging service, such as Sentry or LogRocket, which can provide insights into the application’s health. Below is an example of how to integrate a logging service:
// file: logger.js import * as Sentry from '@sentry/browser'; const logError = (error) => { Sentry.captureException(error); }; // file: query.js const MyComponent = () => { const { data, error } = useQuery(GET_DATA, { onError: (error) => { if (error.graphQLErrors) { logError(error); // Log the ignored error return; // Ignore the error } console.error(error); }, }); if (error) { return <div>Error occurred: {error.message}</div>; } return ( <div> <h1>Items List</h1> <ul> {data?.items?.map((item) => ( <li key={item.id}>{item.name}</li> ))} </ul> </div> ); };
This approach ensures that while users may not see certain errors, developers can still access and analyze the underlying issues, enhancing overall application reliability.
Related Article: How to Get Started with GraphQL Basics
Client-Side Errors in GraphQL
Client-side errors arise when there is an issue with the request made by the client. These errors are often caused by malformed queries, incorrect variable types, or other issues that violate the GraphQL schema. Handling client-side errors is crucial, as they indicate problems with how the client interacts with the GraphQL API.
For instance, if a query includes a field that does not exist, the server will return an error. Proper handling of these errors should provide users with clear feedback, allowing them to correct their queries or input.
An example of handling client-side errors effectively is as follows:
// file: query.js const MyComponent = () => { const { data, error } = useQuery(GET_DATA, { onError: (error) => { if (error.networkError) { console.error('Network error occurred:', error.networkError); } else if (error.graphQLErrors) { console.error('GraphQL errors:', error.graphQLErrors); } }, }); return ( <div> {error && <div>Error: {error.message}</div>} <h1>Items List</h1> <ul> {data?.items?.map((item) => ( <li key={item.id}>{item.name}</li> ))} </ul> </div> ); };
In this example, different types of errors are logged separately, providing clarity on what went wrong.
Network Errors and Their Impact
Network errors occur when there are issues communicating with the GraphQL server. These may include timeouts, DNS failures, or other connectivity problems. Network errors are significant as they can prevent data from being fetched entirely, resulting in a poor user experience.
Handling network errors often requires implementing retry logic or displaying user-friendly messages that inform users of the connection issue. Here is an example of how to manage network errors:
// file: query.js const MyComponent = () => { const { data, error } = useQuery(GET_DATA, { onError: (error) => { if (error.networkError) { alert('Network error: Please check your internet connection.'); } }, }); return ( <div> {error && <div>Error: {error.message}</div>} <h1>Items List</h1> <ul> {data?.items?.map((item) => ( <li key={item.id}>{item.name}</li> ))} </ul> </div> ); };
The implementation of user notifications can enhance the user experience by providing actionable feedback during network issues.
Error Propagation in GraphQL Servers
Error propagation in GraphQL servers refers to how errors encountered during query execution are communicated back to the client. GraphQL’s error handling mechanism ensures that even if some parts of the query succeed while others fail, the server returns a structured response that indicates which parts of the query were successful and which were not.
Developers can customize error propagation behavior based on their application’s requirements. For example, specific errors can be transformed or enriched before being sent to the client. This is achieved through middleware or error handling functions within the server’s implementation.
An example of customizing error propagation in a GraphQL server might look like this:
// file: server.js const { ApolloServer, gql } = require('apollo-server'); const typeDefs = gql` type Item { id: ID! name: String! } type Query { items: [Item] } `; const resolvers = { Query: { items: async () => { throw new Error('Something went wrong while fetching items'); }, }, }; const server = new ApolloServer({ typeDefs, resolvers, formatError: (error) => { // Customize error response return { message: error.message, code: 'INTERNAL_SERVER_ERROR', }; }, }); server.listen().then(({ url }) => { console.log(`🚀 Server ready at ${url}`); });
In this example, errors thrown during the resolution of the items
query are formatted before being sent back to the client, allowing for a more controlled error message structure.
Related Article: Step by Step Process: Passing Enum in GraphQL Query
Implications of Ignoring Errors
Ignoring errors in an application can lead to significant implications if not managed properly. When errors are disregarded, the application may fail to respond accurately to user actions, leading to confusion or a lack of critical information. Furthermore, unaddressed errors can accumulate and create larger issues over time, potentially affecting performance and user experience.
The decision to ignore specific errors should be made carefully. It is crucial to ensure that the ignored errors do not compromise the integrity of the application or mislead users. Developers should establish clear criteria for what constitutes an ignorable error and implement robust logging mechanisms to track these occurrences.
Best Practices for Error Management
Effective error management in GraphQL applications requires a mix of strategies. Here are some best practices to consider:
1. Categorize Errors: Differentiate between client, server, and network errors for appropriate handling.
2. Implement Logging: Use logging services to track ignored errors, providing insights into the application’s health.
3. Provide User Feedback: Inform users about errors in a user-friendly manner, enhancing their experience.
4. Utilize Partial Data: Take advantage of GraphQL’s ability to return partial data, ensuring the application remains responsive even during errors.
5. Test Error Scenarios: Regularly test how your application handles various error scenarios to uncover potential weaknesses.
6. Custom Error Formatting: Format errors on the server side to provide clearer messages to clients, improving clarity.