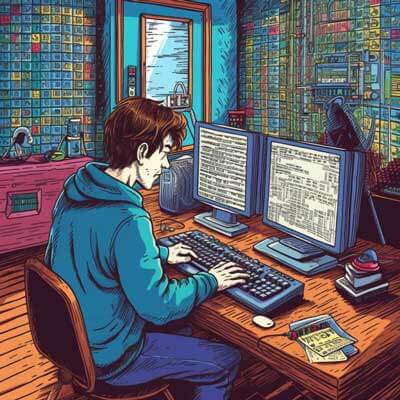
- Overview of Infinity in Python
- What is Math.inf
- Representing Infinity in Python
- Positive and Negative Infinity
- Checking for Infinity with Math.isinf()
- Evaluating Finite Numbers with Math.isfinite()
- Dividing by Zero
- Mathematical Operations with Infinity
- Handling Infinity in NumPy
- Implications of Using Infinity in Calculations
- Comparing Numbers with Infinity
- Output of Float(‘inf’)
Overview of Infinity in Python
Infinity represents an unbounded value that exceeds all finite numbers. In Python, it is treated as a floating-point number, allowing developers to use it in various mathematical operations. This capability is beneficial when working with mathematical models, simulations, or algorithms that require bounds or limits. For more on mathematical operations, check out our guide on Python math operations.
Related Article: How to Execute a Program or System Command in Python
What is Math.inf
The math module provides a special constant, math.inf
, which represents positive infinity. This can be used in calculations where a number can exceed any finite value. The math
module also provides various functions for mathematical operations, making it easier to handle cases involving infinity.
Example of how to use math.inf
:
import math positive_infinity = math.inf print(positive_infinity) # Output: inf
Representing Infinity in Python
Infinity in Python can be represented in multiple ways. The most common method is through the math.inf
constant. However, it can also be created using the floating-point representation:
positive_infinity = float('inf') negative_infinity = float('-inf') print(positive_infinity) # Output: inf print(negative_infinity) # Output: -inf
This representation allows for easy comparison and mathematical operations involving infinite values.
Positive and Negative Infinity
Python distinguishes between positive and negative infinity. Positive infinity represents values greater than any finite number, while negative infinity represents values less than any finite number. This distinction is crucial in mathematical contexts.
Example of both positive and negative infinity:
pos_inf = float('inf') neg_inf = float('-inf') print(pos_inf > 1000) # Output: True print(neg_inf < -1000) # Output: True
This ability to differentiate between the two types of infinity allows for more precise mathematical calculations. For example, when working with limits, understanding how to handle these values is essential. You can find more on handling values with hash maps.
Related Article: How to Use Python with Multiple Languages (Locale Guide)
Checking for Infinity with Math.isinf()
To check if a value is infinite, the math.isinf()
function can be employed. This function returns True
if the given value is either positive or negative infinity.
Example of using math.isinf()
:
import math value = float('inf') print(math.isinf(value)) # Output: True finite_value = 10 print(math.isinf(finite_value)) # Output: False
This function is useful in conditions where you need to validate if a number is infinite before performing further calculations.
Evaluating Finite Numbers with Math.isfinite()
Conversely, math.isfinite()
can be used to check if a number is a finite value. It returns True
for any real number except for positive and negative infinity, as well as NaN (Not a Number).
Example of using math.isfinite()
:
import math finite_value = 10 infinite_value = float('inf') print(math.isfinite(finite_value)) # Output: True print(math.isfinite(infinite_value)) # Output: False
This function helps in ensuring that calculations are performed only on finite numbers, preventing unwanted errors.
Dividing by Zero
In Python, attempting to divide by zero raises a ZeroDivisionError
. However, dividing a finite number by zero returns positive or negative infinity, depending on the sign of the numerator.
Example of dividing numbers:
positive_division = 1 / 0 # Raises ZeroDivisionError negative_division = -1 / 0 # Raises ZeroDivisionError # To illustrate infinity positive_infinity = 1.0 / float('inf') negative_infinity = -1.0 / float('inf') print(positive_infinity) # Output: 0.0 print(negative_infinity) # Output: -0.0
This behavior demonstrates how Python treats division by infinity as a special case.
Related Article: How to Suppress Python Warnings
Mathematical Operations with Infinity
Mathematical operations involving infinity follow specific rules. Adding or subtracting a finite number from infinity results in infinity. Multiplying or dividing infinity by a positive finite number yields infinity, while multiplying or dividing by zero results in NaN.
Examples of these operations:
import math inf = math.inf finite_value = 10 # Addition and subtraction print(inf + finite_value) # Output: inf print(inf - finite_value) # Output: inf # Multiplication print(inf * finite_value) # Output: inf print(inf / finite_value) # Output: inf # Division by zero print(finite_value / 0) # Raises ZeroDivisionError
These behaviors are essential in ensuring that mathematical models using infinity behave as expected.
Handling Infinity in NumPy
NumPy, a popular library for numerical computations, also has its representation of infinity. Similar to Python’s built-in capabilities, NumPy allows the creation of infinite values using numpy.inf
.
Example of using NumPy with infinity:
import numpy as np positive_inf = np.inf negative_inf = -np.inf print(positive_inf) # Output: inf print(negative_inf) # Output: -inf
NumPy provides various functions that can handle operations with infinite values, ensuring compatibility with mathematical operations. If you’re interested in manipulating data, you might find our filtering tutorial useful.
Implications of Using Infinity in Calculations
Using infinity in calculations can lead to unexpected results if not handled properly. For instance, operations that result in infinity can propagate through calculations, affecting final results. Awareness of how infinity interacts with mathematical operations is crucial for accurate computations.
Example of implications:
result = float('inf') + 1000 print(result) # Output: inf result = float('inf') - float('inf') print(result) # Output: nan (Not a Number)
These examples illustrate the importance of understanding the consequences of including infinity in mathematical models.
Related Article: How to Measure Elapsed Time in Python
Comparing Numbers with Infinity
Comparisons with infinity are intuitive. Positive infinity is always greater than any finite number, while negative infinity is always less than any finite number. This property allows for easy sorting and filtering of values.
Example of comparisons:
pos_inf = float('inf') neg_inf = float('-inf') finite_value = 100 print(pos_inf > finite_value) # Output: True print(neg_inf < finite_value) # Output: True print(pos_inf > neg_inf) # Output: True
Such comparisons can simplify logic in algorithms that require boundary conditions. For additional insights on data handling, refer to our guide on index access.
Output of Float(‘inf’)
The expression float('inf')
returns a floating-point representation of positive infinity. This is a convenient way to create an infinite value for use in calculations.
Example of using float('inf')
:
infinity = float('inf') print(infinity) # Output: inf
This function provides a clear and direct method for initializing infinite values, making it easy to incorporate into various applications and calculations.