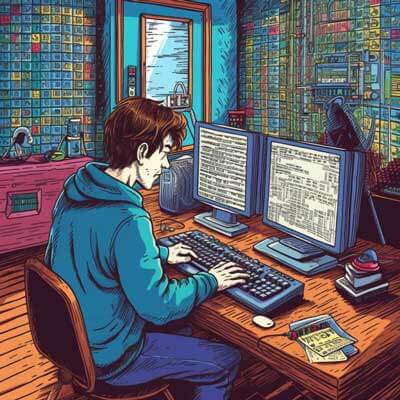
- Overview of Deno and NPM Modules
- Common Errors in Deno Module Imports
- What Causes the ‘Is Not a Function’ Error
- Resolving Deno Module Import Issues
- Correct Import Syntax for NPM Modules
- Differences Between Deno and Node.js
- Deno’s Handling of NPM Modules
- Debugging Deno Module Import Problems
- Checking for Undefined Deno Modules
- Limitations of NPM Modules in Deno
Overview of Deno and NPM Modules
Deno is a modern runtime for JavaScript and TypeScript, built on the V8 engine and developed by the creator of Node.js. Unlike Node.js, Deno is designed with security in mind, requiring explicit permissions for file, network, and environment access. This approach aims to provide a more secure environment for running JavaScript applications.
NPM (Node Package Manager) is the default package manager for Node.js, hosting a vast ecosystem of open-source libraries and modules. Developers frequently use NPM to install and manage packages in their Node.js projects. However, Deno introduces its own module system, which interacts with NPM modules differently.
When attempting to use NPM modules in Deno, issues can arise, particularly regarding import statements and module compatibility. Understanding how Deno handles NPM modules is crucial for a seamless development experience.
Related Article: How to Use Force and Legacy Peer Deps in Npm
Common Errors in Deno Module Imports
Errors often occur during module imports in Deno, especially when integrating third-party libraries from NPM. Common issues include:
1. Module Not Found: This error indicates that Deno cannot locate the specified module.
2. Not a Function: This error arises when trying to call a module or export that is not a function.
3. Undefined Exports: This occurs when the expected exports from a module are not defined or incorrectly referenced.
These errors can stem from incorrect import paths, misunderstanding how modules are exported, or issues related to Deno’s security model.
What Causes the ‘Is Not a Function’ Error
The ‘Is Not a Function’ error typically arises when attempting to call a module or export as a function when it is not. This can happen for several reasons:
1. Incorrect Import Syntax: Using the wrong import syntax can lead to improper references. For example, importing a default export as a named import will result in this error.
2. Module Structure: If the module does not export the expected function, trying to invoke it will trigger the error.
3. Compatibility Issues: Some NPM modules may rely on Node.js-specific features that Deno does not support, leading to unexpected behavior.
Understanding the structure of the module being imported is critical to avoiding this error.
Resolving Deno Module Import Issues
Resolving import issues in Deno involves several steps:
1. Verify Module Existence: Ensure the module is correctly installed and accessible. Use the following command to check for module availability:
deno run --allow-net https://cdn.skypack.dev/your-module-name
2. Check Import Path: Make sure the import path is correct. For example, if importing a specific function from a module, the syntax should be:
import { functionName } from "https://cdn.skypack.dev/your-module-name";
3. Inspect Exports: Review the module’s documentation or source code to confirm the available exports. This information will help determine the correct usage.
Related Article: How to manually install a PrimeVue component npm
Correct Import Syntax for NPM Modules
Using the correct import syntax for NPM modules is vital for successful integration into Deno applications. The syntax differs from that used in Node.js. Below are examples of correct import statements:
1. Default Export:
import express from "https://cdn.skypack.dev/express";
2. Named Exports:
import { Router } from "https://cdn.skypack.dev/express";
3. Importing Multiple Exports:
import { methodA, methodB } from "https://cdn.skypack.dev/your-module-name";
Ensuring that the import matches the module’s export structure prevents errors and allows for smooth functionality.
Differences Between Deno and Node.js
Deno and Node.js differ significantly in several areas:
1. Security Model: Deno requires explicit permission for file and network access, enhancing security by default.
2. Module System: Deno uses ES modules, while Node.js traditionally uses CommonJS modules. This difference affects how modules are imported and exported.
3. Built-in TypeScript Support: Deno has first-class TypeScript support built-in, allowing developers to write TypeScript directly without additional configuration.
4. Standard Library: Deno includes a standard library with modern APIs and utilities, while Node.js relies heavily on third-party packages.
Understanding these differences is essential when transitioning from Node.js to Deno.
Deno’s Handling of NPM Modules
Deno can import NPM modules, but it requires special care. Deno’s module system is designed to work with URLs, meaning NPM modules must be accessed through a CDN or converted to a supported format. One popular way to use NPM modules in Deno is by using Skypack or JSPM:
import { someFunction } from "https://cdn.skypack.dev/some-npm-package";
This approach allows Deno to load NPM modules as ES modules, aligning with its design principles. However, not all NPM packages are compatible with this method, particularly those relying on Node.js internals.
Related Article: How To Detect Programming Language In Npm Code
Debugging Deno Module Import Problems
Debugging import problems in Deno requires a systematic approach:
1. Console Logging: Use console logs to track the values of imported modules or functions. For example:
import { myFunction } from "https://cdn.skypack.dev/my-module"; console.log(myFunction); // Check if it's defined
2. Error Messages: Pay attention to error messages in the console. They often provide clues about what went wrong.
3. Documentation Review: Checking the module’s documentation can clarify usage and common issues.
Checking for Undefined Deno Modules
Before invoking a function or using a module, checking for undefined values can prevent runtime errors. This can be done using conditional statements:
import { myFunction } from "https://cdn.skypack.dev/my-module"; if (typeof myFunction === "function") { myFunction(); } else { console.error("myFunction is not defined or not a function"); }
This approach ensures that the function is available before attempting to call it, reducing the likelihood of encountering the ‘Is Not a Function’ error.
Limitations of NPM Modules in Deno
While Deno supports NPM modules, several limitations exist:
1. Node.js Dependencies: Some NPM modules depend on Node.js-specific APIs, which do not exist in Deno.
2. Compatibility Issues: Not all NPM modules are tested with Deno, leading to potential compatibility problems.
3. Limited Ecosystem: Deno’s ecosystem is smaller than that of Node.js, meaning fewer libraries are available.
Being aware of these limitations helps developers make informed decisions when considering using NPM modules within Deno applications.