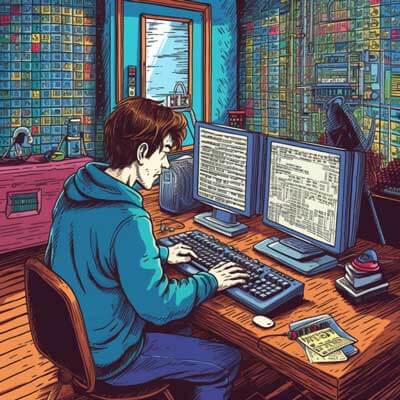
Table of Contents
Different ways to access the length of an array in this.state
In ReactJS, the this.state
object is commonly used to store and manage the state of a component. Oftentimes, this state may include arrays, and it can be useful to access the length of these arrays for various purposes. There are several different methods and techniques that can be used to access the length of an array in this.state
. In this section, we will explore some of these methods and provide examples of how to use them.
Related Article: Inserting Plain Text into an Array Using ReactJS
Using the .length property to access the array length in this.state
The simplest and most straightforward way to access the length of an array in this.state
is by using the .length
property. This property returns the number of elements in the array. Here's an example:
class MyComponent extends React.Component { constructor(props) { super(props); this.state = { myArray: ['apple', 'banana', 'orange'] }; } render() { const arrayLength = this.state.myArray.length; return ( <div> The length of the array is: {arrayLength} </div> ); } }
In this example, the myArray
property in the component's state is an array with three elements. We can access the length of this array by using this.state.myArray.length
. The value of arrayLength
will be 3, and it can be rendered in the component's JSX.
Using the Object.keys() method to access the array length in this.state
Another method to access the length of an array in this.state
is by using the Object.keys()
method. This method returns an array of the object's own enumerable property names, in this case, the keys of the state object. By passing the array in this.state
to Object.keys()
, we can retrieve an array of the keys and then access its length property. Here's an example:
class MyComponent extends React.Component { constructor(props) { super(props); this.state = { myArray: ['apple', 'banana', 'orange'] }; } render() { const arrayLength = Object.keys(this.state.myArray).length; return ( <div> The length of the array is: {arrayLength} </div> ); } }
In this example, we pass this.state.myArray
to Object.keys()
to retrieve an array of the keys. We can then access the length property of this array to get the length of the original array. The value of arrayLength
will be 3, the same as the previous example.
Using the Object.values() method to access the array length in this.state
Similar to Object.keys()
, the Object.values()
method can be used to access the length of an array in this.state
. This method returns an array of the object's own enumerable property values. By passing the array in this.state
to Object.values()
, we can retrieve an array of the values and then access its length property. Here's an example:
class MyComponent extends React.Component { constructor(props) { super(props); this.state = { myArray: ['apple', 'banana', 'orange'] }; } render() { const arrayLength = Object.values(this.state.myArray).length; return ( <div> The length of the array is: {arrayLength} </div> ); } }
In this example, we pass this.state.myArray
to Object.values()
to retrieve an array of the values. We can then access the length property of this array to get the length of the original array. The value of arrayLength
will be 3, the same as the previous examples.
Related Article: Comparing Reactivity in ReactJS and VueJS Variables
Using the Object.entries() method to access the array length in this.state
The Object.entries()
method can also be used to access the length of an array in this.state
. This method returns an array of the object's own enumerable string-keyed property [key, value] pairs. By passing the array in this.state
to Object.entries()
, we can retrieve an array of the entries and then access its length property. Here's an example:
class MyComponent extends React.Component { constructor(props) { super(props); this.state = { myArray: ['apple', 'banana', 'orange'] }; } render() { const arrayLength = Object.entries(this.state.myArray).length; return ( <div> The length of the array is: {arrayLength} </div> ); } }
In this example, we pass this.state.myArray
to Object.entries()
to retrieve an array of the entries. We can then access the length property of this array to get the length of the original array. The value of arrayLength
will be 3, the same as the previous examples.
Using the Array.from() method to access the array length in this.state
The Array.from()
method can be used to create a new array instance from an iterable object. By passing the array in this.state
to Array.from()
, we can create a new array and then access its length property. Here's an example:
class MyComponent extends React.Component { constructor(props) { super(props); this.state = { myArray: ['apple', 'banana', 'orange'] }; } render() { const newArray = Array.from(this.state.myArray); const arrayLength = newArray.length; return ( <div> The length of the array is: {arrayLength} </div> ); } }
In this example, we pass this.state.myArray
to Array.from()
to create a new array instance. We can then access the length property of this new array to get the length of the original array. The value of arrayLength
will be 3, the same as the previous examples.
Using the spread operator (...) to access the array length in this.state
The spread operator (...
) can be used to create a new array with the elements from another array. By spreading the array in this.state
, we can create a new array and then access its length property. Here's an example:
class MyComponent extends React.Component { constructor(props) { super(props); this.state = { myArray: ['apple', 'banana', 'orange'] }; } render() { const newArray = [...this.state.myArray]; const arrayLength = newArray.length; return ( <div> The length of the array is: {arrayLength} </div> ); } }
In this example, we spread this.state.myArray
using the spread operator (...
) to create a new array instance. We can then access the length property of this new array to get the length of the original array. The value of arrayLength
will be 3, the same as the previous examples.
Additional Resources
- Accessing Array Length in React