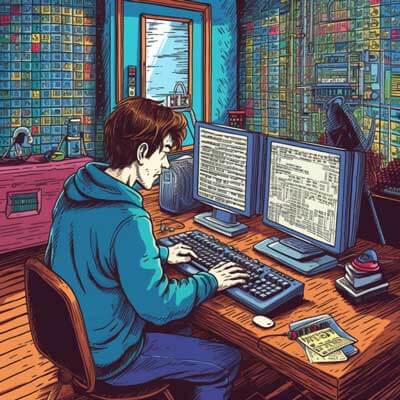
Table of Contents
In this article, we will explore various techniques and approaches for rendering newlines in ReactJS components when working with JSON properties. We will also discuss common challenges faced when handling newlines in JSON and provide best practices to ensure proper rendering and formatting of JSON data.
Common Challenges with Newlines in JSON Properties
When dealing with JSON properties, there are several challenges that developers may face when it comes to handling newlines. These challenges include:
1. JSON Parsing: JSON properties are represented as strings, and when parsing JSON data, special characters like newlines need to be properly escaped to ensure correct interpretation. Failure to escape newlines can lead to parsing errors and unexpected behavior.
2. Rendering in ReactJS: ReactJS is a popular JavaScript library for building user interfaces, and when rendering JSON data in React components, developers need to consider how newlines are handled. If newlines are not properly handled, the rendered content may not match the intended formatting.
3. User Input: In some cases, JSON properties may contain user-generated content, and users may intentionally or unintentionally include newlines in their input. Developers need to handle these newlines properly to avoid security vulnerabilities or formatting issues.
4. Cross-platform Compatibility: JSON data is often consumed by different platforms and devices, and each platform may have different newline conventions. Developers need to ensure that the JSON data is compatible across different platforms and that newlines are rendered consistently.
Related Article: ReactJS: How to Re-Render Post Localstorage Clearing
Techniques for Rendering Newlines in ReactJS Components
When it comes to rendering newlines in ReactJS components, there are several techniques that can be used. Let's explore some of the common approaches:
1. Using the newline character "\n": The most basic approach is to use the newline character "\n" within the JSON property value. This character represents a newline and can be rendered as a line break in HTML. For example:
const json = { message: "Hello\nWorld" }; function App() { return <div>{json.message}</div>; }
This will render the text "Hello" and "World" on separate lines.
2. Using the
HTML tag: Another approach is to use the <br>
HTML tag within the JSON property value. This tag represents a line break in HTML and can be used to force a newline. For example:
const json = { message: "Hello<br>World" }; function App() { return <div dangerouslySetInnerHTML={{ __html: json.message }} />; }
Note that the dangerouslySetInnerHTML
prop is used here to safely render the HTML content.
3. Using CSS styles: CSS can also be used to handle newlines in ReactJS components. By setting the white-space
CSS property to pre-line
or pre-wrap
, newlines within the JSON property value will be preserved and rendered as line breaks. For example:
const json = { message: "Hello\nWorld" }; function App() { return <div style={{ whiteSpace: "pre-wrap" }}>{json.message}</div>; }
This will render the text "Hello" and "World" on separate lines.
These are just a few techniques for rendering newlines in ReactJS components. The choice of technique depends on the specific requirements of your application and the desired rendering behavior.
Approaches for Adding Newlines to JSON Properties in ReactJS
When working with JSON properties in ReactJS, there are different approaches for adding newlines. Let's explore some of these approaches:
1. Using template literals: Template literals, also known as template strings, can be used to add newlines to a JSON property value. By using the backtick character to define the string and the newline character (\n) within the string, newlines can be easily added. For example:
const json = { message: `Hello World` }; function App() { return <div>{json.message}</div>; }
This will render the text "Hello" and "World" on separate lines.
2. Using string concatenation: Another approach is to use string concatenation to add newlines to a JSON property value. By using the plus (+) operator to concatenate strings and the newline character (\n) as a separate string, newlines can be added. For example:
const json = { message: "Hello" + "\n" + "World" }; function App() { return <div>{json.message}</div>; }
This will render the text "Hello" and "World" on separate lines.
These are just a few approaches for adding newlines to JSON properties in ReactJS. The choice of approach depends on the specific requirements of your application and the preferred coding style.
Exploring ReactJS Libraries for Handling Newlines in JSON
ReactJS has a vibrant ecosystem of libraries and tools that can help with handling newlines in JSON properties. Let's explore some of the popular libraries:
1. react-json-view: react-json-view is a useful JSON viewer and editor for ReactJS. It provides a user-friendly interface for visualizing and manipulating JSON data, including proper handling of newlines. It supports syntax highlighting, collapsible sections, and customizable themes. You can find more information and examples on the official documentation page: react-json-view
2. react-syntax-highlighter: react-syntax-highlighter is a syntax highlighting library for ReactJS. It supports various programming languages and data formats, including JSON. It can be used to render JSON with proper formatting, including newlines. You can find more information and examples on the official documentation page: react-syntax-highlighter
These are just a couple of examples of ReactJS libraries that can help with handling newlines in JSON properties. Depending on your specific use case, there may be other libraries that better suit your needs. It's always a good idea to explore the ReactJS ecosystem and choose the library that best fits your requirements.
Related Article: Implementing HTML Templates in ReactJS
Escaping Characters in JSON Properties for ReactJS Rendering
When working with JSON properties in ReactJS, it is important to properly escape special characters to ensure correct rendering. This is especially important when dealing with characters like quotes, backslashes, and newlines that have special meaning in JSON and JavaScript.
To escape characters in JSON properties, you can use the backslash (\) character followed by the character you want to escape. For example, to include a double quote within a JSON property value, you can escape it like this:
const json = { message: "He said, \"Hello World!\"" }; function App() { return <div>{json.message}</div>; }
This will render the text "He said, "Hello World!"".
Similarly, to include a backslash within a JSON property value, you can escape it like this:
const json = { message: "This is a backslash: \\" }; function App() { return <div>{json.message}</div>; }
This will render the text "This is a backslash: \".
When it comes to newlines, you can escape them using the newline character (\n) within the JSON property value. For example:
const json = { message: "Hello\nWorld" }; function App() { return <div>{json.message}</div>; }
This will render the text "Hello" and "World" on separate lines.
Understanding the JavaScript Escape Sequence for Newlines in JSON
In JavaScript, the escape sequence for a newline character is \n
. When working with JSON properties in ReactJS, you can use this escape sequence to include newlines in a JSON property value.
The escape sequence \n
represents a newline character and is used to create a line break within a string. For example:
const json = { message: "Hello\nWorld" }; function App() { return <div>{json.message}</div>; }
This will render the text "Hello" and "World" on separate lines.
It is important to note that the escape sequence \n
is specific to JavaScript and JSON, and other programming languages may have different escape sequences for newlines. When working with JSON data in a non-JavaScript context, it is important to consult the documentation or specifications of that particular language to determine the appropriate escape sequence for newlines.
Best Practices for Handling Newlines in JSON Properties in ReactJS
When handling newlines in JSON properties in ReactJS, it is important to follow best practices to ensure proper rendering and maintainability of your code. Here are some best practices to consider:
1. Consistent Formatting: Maintain consistent formatting of JSON properties across your codebase. This includes using consistent newline characters and indentation. Consistent formatting improves readability and makes it easier to understand and maintain the code.
2. Proper Escaping: Always properly escape special characters, including newlines, within JSON properties. This ensures that the content is rendered correctly and avoids parsing errors.
3. Use React Components: Break down complex JSON structures into smaller, reusable React components. This improves code organization and makes it easier to handle newlines and other formatting requirements.
4. Test with Realistic Data: Test your React components with realistic JSON data that contains newlines. This helps identify any rendering issues and ensures that the components handle newlines correctly in different scenarios.
5. Documentation and Comments: Document the handling of newlines in JSON properties within your codebase. This makes it easier for other developers to understand and maintain the code in the future.
Advanced Techniques for Handling Newlines in JSON Properties
In addition to the basic techniques discussed earlier, there are advanced techniques that can be used to handle newlines in JSON properties in ReactJS. These techniques provide more flexibility and control over the rendering of newlines. Let's explore some of these advanced techniques:
1. Using CSS Flexbox: CSS Flexbox is a useful layout system that can be used to handle newlines in JSON properties. By applying flexbox properties to the container element, you can control how the child elements are laid out, including the wrapping behavior. This allows you to create complex layouts with newlines and other formatting requirements. Here's an example:
const json = { message: "Hello\nWorld" }; function App() { return ( <div style={{ display: "flex", flexDirection: "column" }}> {json.message.split("\n").map((line, index) => ( <div key={index}>{line}</div> ))} </div> ); }
This will render the text "Hello" and "World" on separate lines using flexbox.
2. Using Regular Expressions: Regular expressions can be used to manipulate and format JSON properties that contain newlines. By using regular expression matching and replacement, you can add or remove newlines as needed. Here's an example:
const json = { message: "Hello World" }; function App() { const formattedMessage = json.message.replace(/World/g, "World\n"); return <div>{formattedMessage}</div>; }
This will render the text "Hello" and "World" on separate lines by adding a newline after "World".
These are just a couple of advanced techniques for handling newlines in JSON properties in ReactJS. Depending on your specific requirements, you may need to explore other techniques or combine multiple techniques to achieve the desired rendering and formatting behavior.
Related Article: How to Set Up Your First ReactJS Project
Examples and Code Snippets for Rendering Newlines in ReactJS
Let's now look at some examples and code snippets for rendering newlines in ReactJS components.
Example 1: Using the newline character "\n"
const json = { message: "Hello\nWorld" }; function App() { return <div>{json.message}</div>; }
This will render the text "Hello" and "World" on separate lines.
Example 2: Using the
HTML tag
const json = { message: "Hello<br>World" }; function App() { return <div dangerouslySetInnerHTML={{ __html: json.message }} />; }
Note that the dangerouslySetInnerHTML
prop is used here to safely render the HTML content.
Example 3: Using CSS styles
const json = { message: "Hello\nWorld" }; function App() { return <div style={{ whiteSpace: "pre-wrap" }}>{json.message}</div>; }
This will render the text "Hello" and "World" on separate lines.
These examples demonstrate different techniques for rendering newlines in ReactJS components. Depending on your specific requirements, you can choose the technique that best suits your needs.
A Step-by-Step Tutorial for Adding Newlines to JSON Properties in ReactJS
In this step-by-step tutorial, we will walk through the process of adding newlines to JSON properties in ReactJS. We will use a simple example to demonstrate the techniques discussed earlier.
Step 1: Create a new ReactJS project using Create React App:
npx create-react-app json-newlines-tutorial
Step 2: Navigate to the project directory:
cd json-newlines-tutorial
Step 3: Open the src/App.js
file in your favorite code editor.
Step 4: Replace the existing code in App.js
with the following code:
import React from 'react'; const json = { message: "Hello\nWorld" }; function App() { return <div>{json.message}</div>; } export default App;
This code defines a JSON object with a property message
that contains a newline character. The App
component renders the message
property within a div
element.
Step 5: Save the file and start the development server:
npm start
Step 6: Open your web browser and navigate to http://localhost:3000
. You should see the text "Hello" and "World" rendered on separate lines.