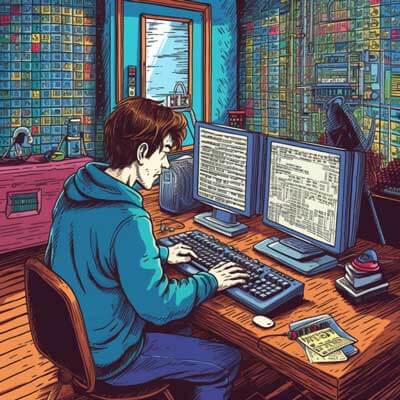
Table of Contents
Django Models: Purpose and Functionality
In Django, models represent the structure and behavior of data in a database. They define the fields and methods that a database table should have. Django follows the Object-Relational Mapping (ORM) pattern, which allows developers to interact with the database using high-level Python code instead of writing SQL queries directly.
To create a model in Django, you need to define a Python class that inherits from the django.db.models.Model
class. Each attribute of the class represents a field of the model, and the field types determine the type of data that can be stored in the database. Django provides a wide range of field types, such as CharField
, IntegerField
, DateField
, and ForeignKey
, among others.
Example 1: Creating a simple model with a few fields
from django.db import models class Customer(models.Model): first_name = models.CharField(max_length=100) last_name = models.CharField(max_length=100) email = models.EmailField(unique=True) date_of_birth = models.DateField()
In this example, we define a Customer
model with four fields: first_name
, last_name
, email
, and date_of_birth
. The CharField
and EmailField
represent string fields, while the DateField
represents a date field.
Example 2: Creating a model with a foreign key relationship
from django.db import models class Order(models.Model): customer = models.ForeignKey(Customer, on_delete=models.CASCADE) product_name = models.CharField(max_length=100) quantity = models.IntegerField() price = models.DecimalField(max_digits=5, decimal_places=2)
In this example, we define an Order
model that has a foreign key relationship with the Customer
model. The ForeignKey
field allows us to establish a one-to-many relationship between the Order
and Customer
models, where a customer can have multiple orders.
Django models also provide various methods and attributes to interact with the database. For example, you can use the objects
attribute to query the database and retrieve instances of a model. Additionally, you can define custom methods on the model class to perform specific operations related to the data.
To use a model in Django, you need to register it in the Django admin interface, which allows you to perform CRUD (Create, Read, Update, Delete) operations on the data. The Django admin interface provides a useful and customizable interface for managing the data in your application.
Related Article: Calculating Averages with Numpy in Python
Additional Resources
Related Article: Python Super Keyword Tutorial
- Django Admin Interface