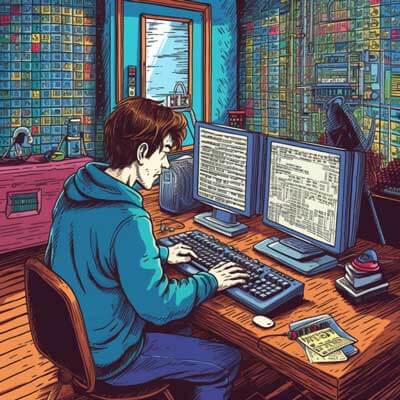
Table of Contents
ModelForms in Django
Django provides a useful feature called ModelForms, which allows you to automatically generate forms based on your Django models. With ModelForms, you can easily create a form that is tied to a specific model and automatically handles form rendering, field validation, and saving data back to the database.
To create a ModelForm, you need to define a class that inherits from the ModelForm
class provided by Django. In this class, you can specify the model that the form is based on, as well as any additional fields or customization you need.
Here's an example of how to create a basic ModelForm for a User
model:
from django import forms from django.contrib.auth.models import User class UserForm(forms.ModelForm): class Meta: model = User fields = ['username', 'email', 'password']
In this example, the UserForm
class is defined as a subclass of ModelForm
. The Meta
inner class specifies the model that the form is based on (User
), as well as the fields that should be included in the form (username
, email
, and password
).
With this ModelForm, you can now easily render the form in a template, validate user input, and save the form data back to the database.
Related Article: Advanced Django Views & URL Routing: Mixins and Decorators
Form Field Validation in Django
Django provides a rich set of built-in form field validation methods that you can use to ensure that user input meets your application's requirements. These validation methods are automatically called when you validate a form, and they raise validation errors if the input is invalid.
Here are a few examples of common form field validation methods in Django:
- clean_()
: This method is called for each field in the form and allows you to perform custom validation on a specific field. For example, you can check if a username is already taken or if an email address is valid.
- clean()
: This method is called after all the individual field validation methods have been called. It allows you to perform cross-field validation, such as checking if two fields have the same value or if a start date is before an end date.
- clean_()
: This method is called for each field in the form and allows you to perform custom validation on a specific field. For example, you can check if a username is already taken or if an email address is valid.
- clean()
: This method is called after all the individual field validation methods have been called. It allows you to perform cross-field validation, such as checking if two fields have the same value or if a start date is before an end date.
Here's an example of how to define custom validation methods in a Django form:
from django import forms class MyForm(forms.Form): def clean_username(self): username = self.cleaned_data.get('username') if username == 'admin': raise forms.ValidationError('Username cannot be "admin"') return username def clean(self): cleaned_data = super().clean() password = cleaned_data.get('password') confirm_password = cleaned_data.get('confirm_password') if password and confirm_password and password != confirm_password: raise forms.ValidationError('Passwords do not match')
In this example, the clean_username()
method checks if the username is set to "admin" and raises a ValidationError
if it is. The clean()
method checks if the password and confirm password fields match and raises a ValidationError
if they don't.
To display validation errors in a template, you can use the {{ form..errors }}
template variable, where is the name of the field you want to display errors for.
Formset Management in Django
Django provides a convenient way to work with multiple instances of a form in a single request through formsets. A formset is a collection of forms of the same type that can be easily managed and processed together.
To create a formset in Django, you need to use the formset_factory
function provided by the django.forms
module. This function takes a form class as input and returns a formset class that you can use to create instances of the form.
Here's an example of how to create a formset for a UserForm
:
from django import forms from django.forms import formset_factory class UserForm(forms.Form): username = forms.CharField() email = forms.EmailField() password = forms.CharField(widget=forms.PasswordInput()) UserFormSet = formset_factory(UserForm, extra=2)
In this example, the UserForm
class is defined as a regular form with three fields: username
, email
, and password
. The UserFormSet
is created using the formset_factory
function, which takes the UserForm
class as input and specifies the number of extra forms to display.
To render the formset in a template, you can iterate over the formset and render each individual form:
{{ formset.management_form }} {% for form in formset %} {{ form.as_table }} {% endfor %}
In this template, the formset.management_form
template variable is used to include the hidden fields required for formset management. The {% for form in formset %}
loop is used to iterate over each form in the formset and render it using the form.as_table
template variable.
Inline Formsets in Django
Inline formsets are a variation of formsets that are specifically designed for working with related models in Django. They allow you to handle the creation, editing, and deletion of related model instances directly from a parent model's form.
To create an inline formset in Django, you need to use the inlineformset_factory
function provided by the django.forms
module. This function takes a parent model, a child model, and optionally a form class as input and returns an inline formset class that you can use to create instances of the form.
Here's an example of how to create an inline formset for a Book
model related to an Author
model:
from django import forms from django.forms import inlineformset_factory from .models import Author, Book class BookForm(forms.ModelForm): class Meta: model = Book fields = ['title', 'publication_date'] AuthorBookFormSet = inlineformset_factory(Author, Book, form=BookForm, extra=2)
In this example, the BookForm
class is defined as a ModelForm that is based on the Book
model and includes the title
and publication_date
fields. The AuthorBookFormSet
is created using the inlineformset_factory
function, which takes the Author
model, the Book
model, and the BookForm
class as input.
To render the inline formset in a template, you can use the same approach as with regular formsets:
{{ formset.management_form }} {% for form in formset %} {{ form.as_table }} {% endfor %}
Related Article: How to Match a Space in Regex Using Python
Dynamic Form Fields in Django Forms
In some cases, you may need to dynamically generate form fields in Django based on certain conditions or user input. Django provides several ways to achieve this, including using the extra
attribute, overriding the __init__
method, or using JavaScript to dynamically add and remove form fields.
One approach is to use the extra
attribute of a form class to specify the number of extra form fields to display. This can be useful when you want to allow users to add multiple instances of a form field dynamically.
Here's an example of how to use the extra
attribute to dynamically generate form fields in Django:
from django import forms class MyForm(forms.Form): name = forms.CharField() email = forms.EmailField() extra_fields = forms.CharField(required=False, widget=forms.TextInput()) def __init__(self, *args, **kwargs): extra_fields = kwargs.pop('extra', 0) super().__init__(*args, **kwargs) self.fields['extra_fields'].widget.attrs['class'] = 'extra-fields' for index in range(extra_fields): self.fields[f'extra_{index}'] = forms.CharField()
In this example, the MyForm
class defines three form fields: name
, email
, and extra_fields
. The extra_fields
field is a single text input field that allows users to enter additional information.
The __init__
method of the form class takes an optional extra
parameter, which specifies the number of extra form fields to display. It then dynamically adds these extra form fields to the form using a loop.
Custom Form Validation in Django
Django allows you to define custom form validation methods to perform complex validation logic that cannot be easily achieved with the built-in form field validation methods. These custom validation methods can be defined at both the form level and the field level.
To define a custom form validation method, you need to override the clean()
method of the form class. This method is called after all the individual field validation methods have been called and allows you to perform cross-field validation or any other custom validation logic.
Here's an example of how to define a custom form validation method in Django:
from django import forms class MyForm(forms.Form): field1 = forms.CharField() field2 = forms.CharField() def clean(self): cleaned_data = super().clean() field1_value = cleaned_data.get('field1') field2_value = cleaned_data.get('field2') if field1_value and field2_value and field1_value == field2_value: raise forms.ValidationError('Field 1 and Field 2 cannot have the same value')
In this example, the clean()
method checks if the values of field1
and field2
are the same and raises a ValidationError
if they are.
To display custom validation errors in a template, you can use the same approach as with regular form field validation errors:
{{ form.as_table }} {% if form.non_field_errors %} <ul class="errorlist"> {% for error in form.non_field_errors %} <li>{{ error }}</li> {% endfor %} </ul> {% endif %}
In this template, the form.non_field_errors
template variable is used to display any custom validation errors that are raised by the form.
Form Widgets in Django
Django provides a wide variety of form widgets that you can use to customize the appearance and behavior of form fields. Form widgets are responsible for rendering HTML form elements and handling user input.
Some common form widgets provided by Django include:
- TextInput
: Renders a single-line text input field.
- Textarea
: Renders a multi-line textarea input field.
- CheckboxInput
: Renders a checkbox input field.
- Select
: Renders a dropdown select input field.
- RadioSelect
: Renders a group of radio buttons.
- DateInput
: Renders a date input field.
You can specify the widget to use for a form field by setting the widget
attribute of the field. For example:
from django import forms class MyForm(forms.Form): name = forms.CharField(widget=forms.TextInput(attrs={'class': 'my-class'})) email = forms.EmailField(widget=forms.EmailInput()) comments = forms.CharField(widget=forms.Textarea())
In this example, the name
field uses a TextInput
widget with a custom CSS class, the email
field uses an EmailInput
widget, and the comments
field uses a Textarea
widget.
You can also create custom form widgets by subclassing the Widget
class provided by Django. This allows you to define your own rendering and handling logic for form fields.
Form Field Types in Django
Django provides a wide range of form field types that you can use to represent different types of data in your forms. These field types handle validation, rendering, and cleaning of form input for their respective data types.
Some common form field types provided by Django include:
- CharField
: Represents a single-line text input field.
- EmailField
: Represents an email input field.
- IntegerField
: Represents an integer input field.
- FloatField
: Represents a floating-point input field.
- DateField
: Represents a date input field.
- TimeField
: Represents a time input field.
You can specify the field type to use for a form field by setting the field's class attribute. For example:
from django import forms class MyForm(forms.Form): name = forms.CharField() email = forms.EmailField() age = forms.IntegerField() weight = forms.FloatField() date_of_birth = forms.DateField() time_of_birth = forms.TimeField()
In this example, the name
field uses a CharField
, the email
field uses an EmailField
, the age
field uses an IntegerField
, the weight
field uses a FloatField
, the date_of_birth
field uses a DateField
, and the time_of_birth
field uses a TimeField
.
Related Article: How to Export a Python Data Frame to SQL Files
Form Processing in Django
Once a form is submitted by a user, Django provides a set of methods and APIs to handle the processing of form data. This includes validating the form, saving the form data to the database, and performing any additional processing or actions based on the form data.
To process a form in Django, you typically follow these steps:
1. Create an instance of the form class, passing in the request data as a parameter.
2. Call the is_valid()
method on the form instance to perform form validation.
3. Access the cleaned form data using the cleaned_data
attribute of the form instance.
4. Perform any additional processing or actions based on the form data.
5. Save the form data to the database if needed.
Here's an example of how to process a form in Django:
from django.shortcuts import render from .forms import MyForm def my_view(request): if request.method == 'POST': form = MyForm(request.POST) if form.is_valid(): # Process the form data name = form.cleaned_data['name'] email = form.cleaned_data['email'] # Perform additional processing or actions # Save the form data to the database form.save() else: form = MyForm() return render(request, 'my-template.html', {'form': form})
In this example, the my_view
function handles the processing of a form. If the request method is POST, it creates an instance of the MyForm
class using the request data and checks if the form is valid. If the form is valid, it accesses the cleaned form data using the cleaned_data
attribute and performs any additional processing or actions. Finally, it saves the form data to the database using the save()
method.
Form Submission in Django
Django provides several ways to handle form submission and perform actions based on the submitted form data. This includes redirecting to a different page, rendering a different template, or displaying success or error messages to the user.
To handle form submission in Django, you typically follow these steps:
1. Create an instance of the form class, passing in the request data as a parameter.
2. Call the is_valid()
method on the form instance to perform form validation.
3. Access the cleaned form data using the cleaned_data
attribute of the form instance.
4. Perform any additional processing or actions based on the form data.
5. Redirect to a different page or render a different template based on the outcome of the form processing.
Here's an example of how to handle form submission in Django:
from django.shortcuts import render, redirect from .forms import MyForm def my_view(request): if request.method == 'POST': form = MyForm(request.POST) if form.is_valid(): # Process the form data name = form.cleaned_data['name'] email = form.cleaned_data['email'] # Perform additional processing or actions # Redirect to a different page or render a different template return redirect('success-page') else: form = MyForm() return render(request, 'my-template.html', {'form': form})
In this example, if the request method is POST, it creates an instance of the MyForm
class using the request data and checks if the form is valid. If the form is valid, it accesses the cleaned form data using the cleaned_data
attribute and performs any additional processing or actions. Finally, it redirects to a different page or renders a different template based on the outcome of the form processing.
Code Snippet - Dynamic Generation of Django Forms:
from django import forms class MyForm(forms.Form): def __init__(self, *args, **kwargs): extra_fields = kwargs.pop('extra', 0) super().__init__(*args, **kwargs) self.fields['extra_fields'] = forms.CharField() for index in range(extra_fields): self.fields[f'extra_{index}'] = forms.CharField()
Code Snippet - Working with Formsets in Django:
from django import forms from django.forms import formset_factory class MyForm(forms.Form): name = forms.CharField() MyFormSet = formset_factory(MyForm, extra=2)
Code Snippet - Using Inline Formsets in Django:
from django import forms from django.forms import inlineformset_factory from .models import Author, Book class BookForm(forms.ModelForm): class Meta: model = Book fields = ['title', 'publication_date'] AuthorBookFormSet = inlineformset_factory(Author, Book, form=BookForm, extra=2)
Code Snippet - Adding Dynamic Form Fields in Django:
from django import forms class MyForm(forms.Form): name = forms.CharField() email = forms.EmailField() extra_fields = forms.CharField(required=False, widget=forms.TextInput()) def __init__(self, *args, **kwargs): extra_fields = kwargs.pop('extra', 0) super().__init__(*args, **kwargs) self.fields['extra_fields'].widget.attrs['class'] = 'extra-fields' for index in range(extra_fields): self.fields[f'extra_{index}'] = forms.CharField()
Code Snippet - Custom Validation on Django Forms:
from django import forms class MyForm(forms.Form): field1 = forms.CharField() field2 = forms.CharField() def clean(self): cleaned_data = super().clean() field1_value = cleaned_data.get('field1') field2_value = cleaned_data.get('field2') if field1_value and field2_value and field1_value == field2_value: raise forms.ValidationError('Field 1 and Field 2 cannot have the same value')
Code Snippet - Customizing Form Widgets in Django:
from django import forms class MyForm(forms.Form): name = forms.CharField(widget=forms.TextInput(attrs={'class': 'my-class'})) email = forms.EmailField(widget=forms.EmailInput()) comments = forms.CharField(widget=forms.Textarea())
Code Snippet - Exploring Different Form Field Types in Django:
from django import forms class MyForm(forms.Form): name = forms.CharField() email = forms.EmailField() age = forms.IntegerField() weight = forms.FloatField() date_of_birth = forms.DateField() time_of_birth = forms.TimeField()
Code Snippet - Processing Form Submissions in Django:
from django.shortcuts import render from .forms import MyForm def my_view(request): if request.method == 'POST': form = MyForm(request.POST) if form.is_valid(): # Process the form data name = form.cleaned_data['name'] email = form.cleaned_data['email'] # Perform additional processing or actions # Save the form data to the database form.save() else: form = MyForm() return render(request, 'my-template.html', {'form': form})
Code Snippet - Handling Form Submission and Performing Actions in Django:
from django.shortcuts import render, redirect from .forms import MyForm def my_view(request): if request.method == 'POST': form = MyForm(request.POST) if form.is_valid(): # Process the form data name = form.cleaned_data['name'] email = form.cleaned_data['email'] # Perform additional processing or actions # Redirect to a different page or render a different template return redirect('success-page') else: form = MyForm() return render(request, 'my-template.html', {'form': form})
Additional Resources
- Django documentation - Working with forms