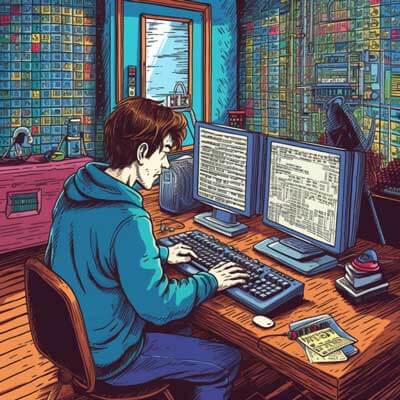
Table of Contents
How to implement localization in Rails?
Localization, also known as internationalization (I18n), is the process of adapting a software application to different languages, regions, and cultures. Rails provides robust support for localization, making it easy to implement in your application.
To implement localization in Rails, follow these steps:
Step 1: Set up your application to use I18n by configuring the default locale in the config/application.rb
file:
config.i18n.default_locale = :en
This sets the default locale to English. You can change it to any other supported language.
Step 2: Create locale files for each language you want to support. These files should be placed in the config/locales
directory. For example, to add support for French, create a file named fr.yml
:
fr: hello: "Bonjour"
Step 3: Use the t
helper method to translate your application's text. For example, in a view file:
<h1><%= t(:hello) %></h1>
This will display "Bonjour" when the locale is set to French.
Step 4: Set the locale for each request. You can do this by using a before_action callback in your controllers. For example:
class ApplicationController < ActionController::Base before_action :set_locale def set_locale I18n.locale = params[:locale] || I18n.default_locale end end
This code sets the locale based on the locale
parameter in the URL, if present. Otherwise, it falls back to the default locale.
Step 5: Provide language switchers to allow users to change the locale. This can be done by adding links to your views that specify the desired locale. For example:
<%= link_to "English", params.merge(locale: :en) %> <%= link_to "French", params.merge(locale: :fr) %>
These links will append the locale
parameter to the current URL, effectively changing the locale for the next request.
Related Article: Ruby on Rails Performance Tuning and Optimization
How does Rails handle different encodings like UTF-8 and ISO-8859-1?
Rails has built-in support for handling different encodings, including UTF-8 and ISO-8859-1. It automatically handles encoding conversions when necessary, making it easier to work with multilingual data.
When Rails receives a request, it automatically sets the encoding for the request body based on the Content-Type
header. For example, if the request specifies a Content-Type
of text/html; charset=UTF-8
, Rails will set the encoding to UTF-8.
Rails also handles encoding conversions when interacting with databases. By default, Rails uses UTF-8 as the database encoding, ensuring that data is stored and retrieved correctly.
When working with different encodings, it's important to be aware of potential issues and take appropriate steps to handle them. For example, if you're receiving data from an external source in a different encoding, you may need to convert it to the desired encoding before processing it.
Rails provides various methods for working with encodings. For example, the force_encoding
method can be used to explicitly set the encoding of a string. The encode
method can be used to convert a string from one encoding to another.
Here's an example that demonstrates how Rails handles different encodings:
# Assume we have a string in ISO-8859-1 encoding iso_8859_1_string = "Café" # Convert the string to UTF-8 encoding utf8_string = iso_8859_1_string.force_encoding("ISO-8859-1").encode("UTF-8") # Print the UTF-8 string puts utf8_string
In this example, the force_encoding
method is used to set the encoding of the string to ISO-8859-1. The encode
method is then used to convert the string to UTF-8. Finally, the UTF-8 string is printed, which should display "Café" correctly.
Rails' handling of different encodings simplifies working with multilingual data and ensures that data is stored, retrieved, and displayed correctly in your application.
What is the importance of character encoding in Rails?
Character encoding is a critical aspect of web development, including Rails applications. It determines how characters are represented and stored in a computer's memory and how they are displayed to users. Rails provides robust support for character encoding, and understanding its importance is crucial for developing internationalized applications.
Here are some reasons why character encoding is important in Rails:
1. Supporting Multiple Languages: Different languages require different character encodings. For example, English text can be represented using ASCII or UTF-8 encoding, while languages like Chinese or Arabic require more complex encodings like UTF-16. By using the appropriate encoding, Rails ensures that your application can handle and display text from various languages correctly.
2. Preventing Data Corruption: Using the wrong character encoding can lead to data corruption. If you store text in a database using one encoding and retrieve it using a different encoding, the text may be incorrectly interpreted or modified. Rails' support for character encoding ensures that data is stored and retrieved correctly, preventing data corruption and preserving data integrity.
3. Handling Special Characters: Some characters have special meanings in HTML, JavaScript, or other contexts. For example, the <
and >
characters have special meanings in HTML tags, and the "
character has special meaning in JavaScript. Rails automatically escapes these characters to prevent security vulnerabilities and ensure that they are displayed correctly in the browser.
4. Search Engine Optimization (SEO): Character encoding can affect how search engines index and display your website. Using the correct encoding ensures that search engines can correctly interpret and display your content, improving your website's visibility in search results.
To summarize, character encoding is crucial for supporting multiple languages, preventing data corruption, handling special characters, and optimizing your Rails application for search engines. By understanding and using the appropriate character encoding, you can ensure that your application handles text correctly and provides a seamless experience for users across different languages and regions.
What are some strategies for multi-language SEO optimization in Rails?
Search Engine Optimization (SEO) is crucial for improving the visibility and ranking of your Rails application in search engine results. When it comes to multi-language applications, there are several strategies you can employ to optimize your application for SEO.
Here are some strategies for multi-language SEO optimization in Rails:
1. Use Language-Specific URLs: One effective strategy is to use language-specific URLs for different language versions of your website. For example, instead of using subdomains like en.example.com
and fr.example.com
, you can use language-specific paths like example.com/en
and example.com/fr
. This approach helps search engines understand that you have different language versions of your website and can improve the indexing and ranking of your content.
2. Use hreflang Tags: The hreflang
attribute is an HTML tag that helps search engines understand the language and regional targeting of each page on your website. In Rails, you can include this tag in the <head>
section of your layout file, dynamically setting the language and region based on the current locale. Here's an example:
<head> <%= tag(:link, rel: "alternate", hreflang: I18n.locale, href: url_for(locale: I18n.locale)) %> </head>
This code generates the appropriate hreflang
tag based on the current locale. Search engines can then use this information to serve the correct language version of your website in search results.
3. Add Language-Specific Metadata: Each language version of your website may have different metadata, such as titles and descriptions. In Rails, you can leverage the content_for
helper to dynamically set language-specific metadata in your views. For example:
<% content_for :title do %> <%= t(:homepage_title) %> <% end %>
This code sets the title of the page based on the current locale. You can do the same for other metadata tags, such as the description.
4. Submit Language-Specific Sitemaps: A sitemap is an XML file that lists the URLs of your website, helping search engines discover and crawl your content. For multi-language websites, it's recommended to submit language-specific sitemaps to search engines. In Rails, you can generate language-specific sitemaps using a gem like sitemap_generator
and submit them to search engines through their respective webmaster tools.
5. Implement Language Switching: Language switchers allow users to switch between different language versions of your website. Providing a language switcher can improve the user experience and help search engines understand the language options available. In Rails, you can implement language switching by adding links to different language versions of your website. For example:
<%= link_to "English", url_for(locale: :en) %> <%= link_to "French", url_for(locale: :fr) %>
These links allow users to switch between the English and French versions of your website.
Related Article: Ruby on Rails with Modular Rails, ActiveRecord & Testing
What are the benefits of internationalization in Rails?
Internationalization, also known as I18n, is the process of adapting a software application to different languages, regions, and cultures. Rails provides robust support for internationalization, and there are several benefits to implementing it in your application.
Here are some benefits of internationalization in Rails:
1. Expanded User Base: By supporting multiple languages, your application can reach a wider audience. Users from different language backgrounds can use your application comfortably, increasing its popularity and user base.
2. Improved User Experience: Internationalization enhances the user experience by presenting content in the user's preferred language. Users are more likely to engage with your application if they can understand the content and interact with it in their native language.
3. Increased Accessibility: Internationalization makes your application more accessible to users with different language preferences. It allows individuals who may have limited English proficiency or prefer other languages to use your application effectively.
4. Compliance with Legal Requirements: In some countries, there are legal requirements to provide applications in the local language. By internationalizing your Rails application, you can ensure compliance with these regulations and expand your market reach.
5. Simplified Localization: Rails provides a comprehensive framework for localization, making it easier to translate and adapt your application to different languages. The built-in support for translations and locale management simplifies the process of adding new languages and maintaining translations.
6. Easier Maintenance: By separating content from code through the use of translation files, Rails makes it easier to maintain and update your application's translations. Changes or updates to translations can be made without modifying the application's codebase.
7. Search Engine Optimization (SEO): Internationalizing your Rails application can improve its ranking in search engine results. By providing language-specific versions of your content and using language-specific URLs, metadata, and hreflang tags, you can optimize your application for search engines and increase its visibility in different language markets.
Overall, internationalization in Rails offers numerous benefits, including an expanded user base, improved user experience, increased accessibility, compliance with legal requirements, simplified localization, easier maintenance, and improved SEO. By implementing internationalization in your Rails application, you can create a more inclusive and globally accessible software product.
How to handle translation in Rails?
Translation is a crucial aspect of internationalization in Rails. It allows you to provide content in different languages and adapt your application to different locales. Rails provides a useful translation framework that simplifies the process of handling translations.
Here's how you can handle translation in Rails:
Step 1: Set up your application to use the translation framework by configuring the default locale in the config/application.rb
file:
config.i18n.default_locale = :en
Step 2: Create translation files for each language you want to support. These files should be placed in the config/locales
directory. For example, to add support for French, create a file named fr.yml
:
fr: hello: "Bonjour"
This file contains translations for the French locale. Add translations for other languages as needed.
Step 3: Use the t
helper method to translate text in your application. For example, in a view file:
<h1><%= t(:hello) %></h1>
This code translates the :hello
key based on the current locale. If the locale is set to French, it will display "Bonjour".
Step 4: Provide translations for dynamic content using interpolation. You can pass variables to the translation method and use them in the translated text. For example:
<%= t(:welcome_message, name: current_user.name) %>
In this example, the :welcome_message
key contains a greeting that includes the user's name. The name
variable is passed as an option to the translation method, allowing it to be dynamically inserted into the translated text.
Step 5: Handle pluralization by using the pluralize
option in your translation files. For example:
en: apples: one: "One apple" other: "%{count} apples"
In this example, the apples
key has two translations: one for the singular form (one
) and one for the plural form (other
). The %{count}
variable can be used to dynamically insert the count into the translated text.
Step 6: Organize your translations using nested keys. This allows you to group related translations together. For example:
en: greetings: hello: "Hello" goodbye: "Goodbye"
In this example, the greetings
key contains translations for different greetings. This can help keep your translation files organized and easier to maintain.
What are some best practices for internationalizing a Rails application?
Internationalizing a Rails application involves adapting it to different languages, regions, and cultures. It requires careful planning and implementation to ensure a seamless experience for users across different locales. Here are some best practices for internationalizing a Rails application:
1. Plan for Internationalization from the Start: Consider internationalization requirements early in the development process. This includes designing your application to support multiple languages, regions, and cultures. By planning ahead, you can avoid potential pitfalls and make the internationalization process smoother.
2. Separate Content from Code: Use translation files to separate content from code. This allows you to manage translations independently of the application's codebase, making it easier to update and maintain translations. Rails' built-in support for translation files simplifies this process.
3. Follow Localization Standards: Adhere to localization standards and guidelines. This includes using standard language and region codes, formatting dates, times, and numbers correctly for each locale, and respecting cultural norms and preferences. Rails provides helpers and methods to assist with these tasks.
4. Test with Different Locales: Test your application with different locales to ensure that it works correctly in each language and region. Pay attention to text expansion and contraction, as translated text may have different lengths than the original. Adequate testing helps uncover issues and ensures a seamless experience for users.
5. Provide Language-Specific Support: Consider language-specific requirements and features, such as right-to-left (RTL) text support, font selection, and localized input validation. Adapting your application to these language-specific needs improves usability and user experience.
6. Handle Time Zones: Consider time zone differences when dealing with date and time data. Use Rails' time zone support to ensure that dates and times are displayed correctly for each locale. This helps prevent confusion and ensures that time-related functionality works as expected.
7. Consider Cultural Differences: Be mindful of cultural differences and sensitivities. Avoid assumptions and stereotypes that may be incorrect or offensive in different cultures. Provide options for users to customize their experience based on their cultural preferences, such as date format or measurement units.
8. Stay Up-to-Date with Translation Changes: Keep your translations up-to-date as your application evolves. As you add or modify content, ensure that the translations remain accurate and contextually relevant. Encourage community contributions for translations to maintain the highest quality and coverage.
9. Optimize Performance: Consider the performance implications of internationalization. Caching translations, minimizing database queries, and optimizing asset delivery can help improve the performance of your internationalized application.
10. Continuously Monitor and Improve: Monitor user feedback, analytics, and metrics to identify areas for improvement. Actively seek feedback from users in different locales to address any issues or gaps in your internationalization efforts. Regularly update your translations and enhance the internationalization features of your application.
Additional Resources
- Internationalization and Localization in Ruby on Rails