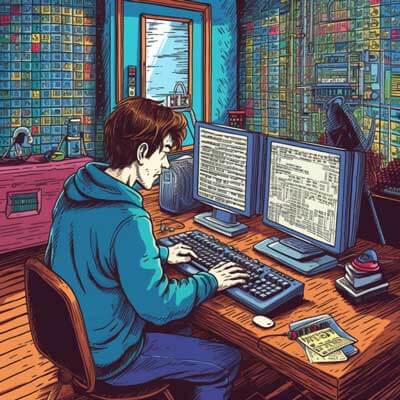
Table of Contents
Benefits of Using the Beego Framework
The Beego framework is a useful tool for building web applications in the Go programming language. It provides developers with a comprehensive set of features and tools to simplify the development process and improve productivity. Here are some of the key benefits of using the Beego framework:
Related Article: Deployment and Monitoring Strategies for Gin Apps
Rapid Development
Beego follows the Model-View-Controller (MVC) architectural pattern, which promotes the separation of concerns and allows for modular and reusable code. This pattern enables developers to quickly build and iterate on their applications, reducing development time and effort. The framework provides a set of built-in functions and utilities that simplify common tasks, such as routing, request handling, and database operations.
Scalability and Performance
Beego is designed to handle high traffic and large-scale applications. It leverages the power of the Go language, which is known for its excellent performance and concurrency capabilities. Beego applications can handle thousands of concurrent requests without sacrificing performance. Additionally, Beego provides support for caching, session management, and other performance optimization techniques to ensure optimal application performance.
Extensibility and Customization
Beego is highly extensible and allows developers to customize and extend its functionality to meet the specific needs of their applications. It provides a modular structure that enables developers to add or remove components as needed, allowing for flexibility and adaptability. Beego also supports the use of third-party libraries and packages, making it easy to integrate with existing systems or leverage external tools.
Related Article: Exploring Advanced Features of Gin in Golang
Community and Ecosystem
Beego has a vibrant and active community of developers who contribute to its development, offer support, and share their knowledge and experience. The framework has a rich ecosystem of plugins, modules, and extensions that can be used to enhance and extend the functionality of Beego applications. The community also provides extensive documentation, tutorials, and resources to help developers get started with Beego and solve common challenges.
MVC Pattern in Go
The Model-View-Controller (MVC) pattern is a software architectural pattern commonly used in web application development. It helps separate the concerns of data storage, user interface, and application logic, making the codebase more modular, maintainable, and scalable. In the context of Go and the Beego framework, the MVC pattern can be implemented as follows:
Model
The model represents the data and business logic of the application. It defines the structure and behavior of the data, as well as any operations or transformations that need to be performed on the data. In Go, the model typically consists of structs that define the data schema, and methods that operate on the data. Here's an example of a simple model in Go:
type User struct { ID int Username string Email string } func (u *User) Save() error { // Logic to save the user to the database return nil }
In this example, the User
struct represents a user object with properties like ID, Username, and Email. The Save
method is a behavior associated with the User model that performs the logic to save the user to a database.
View
The view is responsible for presenting the data to the user and handling user interactions. In the context of Beego, the view is typically implemented using templates. Beego provides a built-in template engine that supports the use of HTML templates with embedded Go code. Here's an example of a simple view template in Beego:
<html> <head> <title>Welcome</title> </head> <body> <h1>Welcome, {{ .Username }}!</h1> <p>Your email is {{ .Email }}.</p> </body> </html>
In this example, the view template is an HTML file that displays a welcome message along with the data from the User model.
Related Article: Handling Large Volumes of Data with Golang & Gin
Controller
The controller acts as the intermediary between the model and the view. It receives user input, updates the model accordingly, and renders the appropriate view. In Beego, controllers are implemented as structs that embed the beego.Controller
type and define methods to handle different requests. Here's an example of a simple controller in Beego:
type UserController struct { beego.Controller } func (c *UserController) Get() { user := User{ ID: 1, Username: "john", Email: "john@example.com", } c.Data["Username"] = user.Username c.Data["Email"] = user.Email c.TplName = "user/welcome.tpl" }
In this example, the UserController
struct embeds the beego.Controller
type and defines a Get
method to handle GET requests. Inside the Get
method, a User model is created, and its data is passed to the view template using the Data
field of the controller. Finally, the TplName
field is set to the path of the view template to render it.
Error Handling in Go Applications
Effective error handling is essential in any application to ensure proper functioning and provide meaningful feedback to users. In Go, error handling is straightforward and encourages explicit handling of errors. Here are some best practices for error handling in Go applications:
Returning Errors
In Go, functions that can potentially encounter errors typically return an error value as the last return value. By convention, if the function completes successfully, it returns nil
as the error value. If an error occurs, the function should return an error value that provides information about the error. Here's an example of a function that returns an error:
func divide(a, b int) (int, error) { if b == 0 { return 0, fmt.Errorf("cannot divide by zero") } return a / b, nil }
In this example, the divide
function takes two integers as input and returns their division. If the second input is zero, the function returns an error with a custom error message using the fmt.Errorf
function.
Checking Errors
When calling a function that returns an error, it's important to check the error value to handle any potential errors. This can be done using an if
statement or the :=
shorthand notation. Here's an example of checking an error:
result, err := divide(10, 2) if err != nil { log.Fatal(err) } fmt.Println(result)
In this example, the divide
function is called, and the returned result and error values are assigned to the result
and err
variables. If the error is not nil
, indicating an error occurred, the program logs the error and exits. Otherwise, it prints the result.
Related Article: Optimizing and Benchmarking Beego ORM in Golang
Error Wrapping and Context
In more complex applications, it's often necessary to provide additional context or wrap errors to provide more meaningful information to the caller. Go provides the errors
package and the fmt.Errorf
function for creating custom error values. Here's an example of wrapping errors:
func openFile(filename string) (*File, error) { f, err := os.Open(filename) if err != nil { return nil, fmt.Errorf("failed to open file: %w", err) } return f, nil }
In this example, the openFile
function attempts to open a file. If an error occurs, it wraps the original error with additional context using the %w
verb in the fmt.Errorf
function.
Logging Options in Go
Logging is an essential aspect of any application, as it helps developers understand what's happening within the application and troubleshoot issues. In Go, there are several options for logging, ranging from the built-in log
package to more feature-rich third-party libraries. Here are some logging options in Go:
Using the log
Package
Go's standard library provides the log
package, which offers a simple logging interface. It provides functions like Print
, Printf
, and Println
for logging messages with different levels of severity. Here's an example of using the log
package:
package main import ( "log" ) func main() { log.Println("This is a log message") log.Printf("This is a formatted log message: %s", "hello") }
In this example, the log
package is imported, and the Println
and Printf
functions are used to log messages to the console.
Using the logrus
Library
logrus
is a popular third-party logging library for Go that provides a more feature-rich and flexible logging solution. It supports different log levels, log formatting, hooks, and more. Here's an example of using logrus
:
package main import ( "github.com/sirupsen/logrus" ) func main() { log := logrus.New() log.SetFormatter(&logrus.TextFormatter{}) log.SetLevel(logrus.DebugLevel) log.Debug("This is a debug log message") log.Info("This is an info log message") log.Warn("This is a warning log message") log.Error("This is an error log message") }
In this example, the logrus
package is imported, and a new logger instance is created using logrus.New()
. The logger's formatter is set to logrus.TextFormatter
, and the log level is set to logrus.DebugLevel
. The logger is then used to log messages at different log levels.
Related Article: Golang Tutorial for Backend Development
Best Practices for Unit Testing in Go
Unit testing is an integral part of software development, as it helps ensure the correctness and reliability of code. In Go, the standard library provides a testing package that makes it easy to write and run unit tests. Here are some best practices for unit testing in Go:
Write Focused and Independent Tests
Each unit test should focus on testing a specific functionality or behavior of the code. Tests should be independent of each other, meaning that the outcome of one test should not affect the outcome of another test. This ensures that each test accurately reflects the behavior of the code being tested and makes it easier to identify and fix issues when tests fail. Here's an example of a focused and independent test in Go:
func TestAdd(t *testing.T) { result := add(2, 3) expected := 5 if result != expected { t.Errorf("add(2, 3) = %d; expected %d", result, expected) } }
In this example, the TestAdd
function tests the add
function by providing two input values and comparing the result to the expected output. If the result does not match the expected value, the test fails and an error message is logged.
Use Table-Driven Tests
Table-driven tests are a technique where test cases are defined in a table-like structure, allowing for easy addition and modification of test cases. This approach reduces code duplication and makes it easier to understand the test cases being executed. Here's an example of a table-driven test in Go:
func TestMultiply(t *testing.T) { testCases := []struct { a int b int expected int }{ {2, 3, 6}, {0, 5, 0}, {-1, 4, -4}, } for _, tc := range testCases { result := multiply(tc.a, tc.b) if result != tc.expected { t.Errorf("multiply(%d, %d) = %d; expected %d", tc.a, tc.b, result, tc.expected) } } }
In this example, the TestMultiply
function defines a table of test cases, each with input values and an expected output. The test function then iterates over the test cases, executes the multiply
function with the input values, and compares the result to the expected output.
Mock Dependencies
When writing unit tests, it's often necessary to isolate the code being tested from its dependencies to ensure that the tests are focused and do not rely on external factors. In Go, this can be achieved by using interfaces and creating mock implementations of dependencies. Here's an example of using interfaces and mocks in a unit test:
type Database interface { Save(data []byte) error } type MockDatabase struct { CalledSave bool } func (m *MockDatabase) Save(data []byte) error { m.CalledSave = true return nil } func TestSaveData(t *testing.T) { db := &MockDatabase{} err := saveData(db, []byte("test data")) if err != nil { t.Errorf("saveData returned an unexpected error: %v", err) } if !db.CalledSave { t.Error("saveData did not call Save on the database") } }
In this example, the Database
interface defines the behavior of a database dependency, and the MockDatabase
struct implements that interface. The TestSaveData
function creates a mock instance of the database, calls the saveData
function with the mock database, and verifies that the Save
method of the database is called.
Related Article: Beego Integration with Bootstrap, Elasticsearch & Databases
Testing Strategies for Beego Applications
When it comes to testing Beego applications, there are several strategies and techniques that can be employed to ensure comprehensive test coverage and reliable application behavior. Here are some testing strategies for Beego applications:
Controller Testing
Controllers play a crucial role in Beego applications as they handle the routing and processing of requests. Testing controllers allows you to verify that the correct actions are taken based on specific requests and that the responses are generated as expected. To test controllers in Beego, you can use the beego.TestBeegoInit
function to initialize the Beego application and the httptest
package to simulate requests. Here's an example of testing a controller in Beego:
func TestUserController_Get(t *testing.T) { ctrl := &UserController{} r, _ := http.NewRequest("GET", "/user", nil) w := httptest.NewRecorder() beego.BeeApp.Handlers.ServeHTTP(w, r) if w.Code != http.StatusOK { t.Errorf("expected status code %d, got %d", http.StatusOK, w.Code) } expectedBody := "Welcome, john!" if w.Body.String() != expectedBody { t.Errorf("expected body '%s', got '%s'", expectedBody, w.Body.String()) } }
In this example, the TestUserController_Get
function tests the Get
method of the UserController
by simulating a GET request to the /user
endpoint. The response is then checked to ensure that the status code and body match the expected values.
Model Testing
Models in Beego applications are responsible for interacting with the data and performing business logic. Testing models allows you to verify that the data is correctly stored and retrieved and that any associated business logic functions as expected. To test models in Beego, you can create test cases that exercise the model's methods and validate the expected outcomes. Here's an example of testing a model in Beego:
func TestUser_Save(t *testing.T) { user := &User{ ID: 1, Username: "john", Email: "john@example.com", } err := user.Save() if err != nil { t.Errorf("expected no error, got %v", err) } // Verify that the user is saved correctly // ... }
In this example, the TestUser_Save
function tests the Save
method of the User
model by creating a test user instance and calling the Save
method. The test then verifies that no error occurs during the save operation and can further validate the outcome by checking the database or performing other assertions.
Organizing MVC in a Beego Application
Organizing the Model-View-Controller (MVC) components in a Beego application is crucial for maintainability and readability. By following best practices for organizing MVC components, you can improve code reusability, modularity, and overall project structure. Here are some best practices for organizing MVC in a Beego application:
Related Article: Exploring Advanced Features of Beego in Golang
Separate Files for Models, Views, and Controllers
To keep the codebase organized, it's recommended to separate the models, views, and controllers into their respective files or packages. This makes it easier to locate and work with specific components and promotes code reusability. Here's an example of a directory structure for a Beego application:
. ├── main.go ├── models │ └── user.go ├── views │ └── user │ └── welcome.tpl └── controllers └── user.go
In this example, the models are stored in the models
directory, views in the views
directory, and controllers in the controllers
directory. Each component has its own file or package, allowing for easy navigation and organization.
Use Subdirectories for Related Components
In larger applications, it's common to have multiple models, views, and controllers. To avoid cluttering the root directory, it's a good practice to use subdirectories to group related components. For example, you can create a users
subdirectory to store all components related to user management. Here's an example:
. ├── main.go ├── models │ ├── user.go │ └── post.go ├── views │ ├── user │ │ └── welcome.tpl │ └── post │ └── index.tpl └── controllers ├── user.go └── post.go
In this example, the users
subdirectory contains the user-related components, including the user model, user view templates, and user controller. This approach helps keep the codebase organized and makes it easier to manage and maintain.
Follow Naming Conventions
Consistent naming conventions can greatly improve the readability and maintainability of the codebase. When organizing MVC components in a Beego application, it's a good practice to follow naming conventions for files, packages, and struct names. For example, use singular or plural names for models, use lowercase names for views and controllers, and use descriptive names that reflect the purpose of the component. Here's an example:
. ├── main.go ├── models │ ├── user.go │ └── post.go ├── views │ ├── user │ │ └── welcome.tpl │ └── post │ └── index.tpl └── controllers ├── user.go └── post.go
In this example, the file and package names follow the naming conventions: the models are named using the singular form (user.go
, post.go
), the views are named using lowercase names (user
, post
), and the controllers are named using lowercase names (user.go
, post.go
).
Customizing Response Handling in Beego
In Beego, customizing the response handling allows developers to control how the application responds to different requests and situations. Beego provides various options for customizing the response, such as setting custom HTTP headers, handling errors, and providing custom error pages. Here are some examples of customizing response handling in Beego:
Related Article: Implementing Real-time Features with Gin & Golang
Setting Custom HTTP Headers
Beego allows you to set custom HTTP headers in the response to provide additional information or control certain behaviors. This can be useful for setting security-related headers, caching headers, or custom headers specific to your application. Here's an example of setting a custom HTTP header in Beego:
func (c *MainController) Get() { c.Ctx.ResponseWriter.Header().Set("X-Custom-Header", "Hello") c.Ctx.WriteString("Hello, World!") }
In this example, the Get
method of the MainController
sets a custom HTTP header named X-Custom-Header
with the value "Hello"
. The response body is then written using the WriteString
method of the Context
object.
Error Handling and Custom Error Pages
Beego provides built-in error handling mechanisms that allow you to handle different types of errors and provide custom error pages. You can define error handlers for specific HTTP status codes, handle panics, or implement a custom error controller to handle all errors. Here's an example of handling errors and providing custom error pages in Beego:
beego.ErrorController(&controllers.ErrorController{}) func (ec *ErrorController) Error404() { ec.Data["content"] = "Page not found" ec.TplName = "errors/404.tpl" } func (ec *ErrorController) Error500() { ec.Data["content"] = "Internal server error" ec.TplName = "errors/500.tpl" }
In this example, the ErrorController
is defined to handle errors. The Error404
method handles the HTTP 404 Not Found error and sets the appropriate template to render a custom 404 error page. Similarly, the Error500
method handles the HTTP 500 Internal Server Error and sets the template to render a custom 500 error page.
Examples of Beego Applications
To demonstrate the usage of Beego and its various features, here are two examples of Beego applications:
Example 1: Simple Blogging Platform
This example showcases a simple blogging platform built with Beego. It includes features such as user registration, login, creating and editing blog posts, and viewing blog posts. The application follows the MVC pattern, with models for users and blog posts, controllers for handling user actions, and views for rendering templates. Here's an overview of the directory structure for this example:
. ├── main.go ├── models │ ├── user.go │ └── post.go ├── views │ ├── user │ │ ├── login.tpl │ │ └── register.tpl │ └── post │ ├── create.tpl │ ├── edit.tpl │ └── view.tpl └── controllers ├── user.go └── post.go
In this example, the models
directory contains the user and post models, the views
directory contains the templates for user-related actions and post-related actions, and the controllers
directory contains the controllers for handling user actions and post actions.
Related Article: Real-Time Communication with Beego and WebSockets
Example 2: E-commerce Platform
This example showcases an e-commerce platform built with Beego. It includes features such as product listing, product details, shopping cart management, and order processing. The application follows the MVC pattern, with models for products, orders, and users, controllers for handling user actions and order processing, and views for rendering templates. Here's an overview of the directory structure for this example:
. ├── main.go ├── models │ ├── product.go │ ├── order.go │ └── user.go ├── views │ ├── product │ │ ├── list.tpl │ │ └── details.tpl │ ├── cart │ │ ├── view.tpl │ │ └── checkout.tpl │ └── order │ ├── confirm.tpl │ └── success.tpl └── controllers ├── product.go ├── cart.go ├── order.go └── user.go
In this example, the models
directory contains the product, order, and user models, the views
directory contains the templates for product listing and details, cart management, and order processing, and the controllers
directory contains the controllers for handling user actions, cart management, order processing, and product listing.
These examples demonstrate how Beego can be used to build different types of applications and highlight the organization and structure of MVC components in a Beego application.