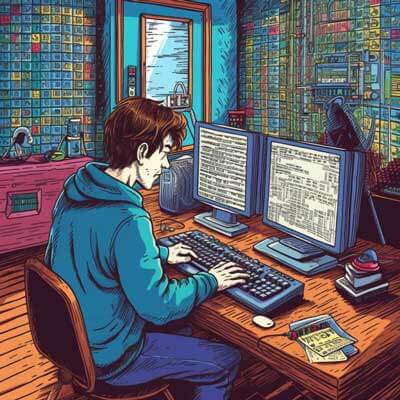
Introduction
The development of single-page applications (SPAs) has gained significant popularity in recent years. React.js and Vue.js are two widely-used frontend frameworks that enable developers to build dynamic and interactive user interfaces. However, these SPAs require efficient and reliable backends to handle data processing, authentication, and other backend functionalities. In this article, we will explore how to build efficient Gin backends for React.js and Vue.js SPAs. We will discuss the integration of Gin, a web framework written in Golang, with these frontend frameworks. Additionally, we will explore API design considerations and the utilization of HTMX for partial page updates without heavy JavaScript.
Related Article: Golang Tutorial for Backend Development
Building Efficient Gin Backends
Building efficient backends is crucial for the smooth functioning of SPAs. Gin, a lightweight web framework written in Golang, provides a useful and efficient solution for building backend APIs. It offers a wide range of features, including routing, middleware, and request handling, making it an ideal choice for building efficient backends for React.js and Vue.js SPAs.
Gin Installation
To get started with Gin, you need to install it in your Golang project. Open your terminal and run the following command:
go get -u github.com/gin-gonic/gin
This command will download and install Gin and its dependencies in your project.
Creating a Basic Gin Server
Once Gin is installed, you can create a basic server to handle HTTP requests. Create a new file called main.go
and add the following code:
package main import "github.com/gin-gonic/gin" func main() { r := gin.Default() r.GET("/", func(c *gin.Context) { c.JSON(200, gin.H{ "message": "Hello, World!", }) }) r.Run() // Run the server }
In this code, we import the gin
package and create a new Gin router using gin.Default()
. We define a route for the root URL (“/”) and respond with a JSON message. Finally, we start the server using r.Run()
.
To run the server, execute the following command in your terminal:
go run main.go
You should see the server running and listening on localhost:8080
. If you visit http://localhost:8080
in your browser, you will see the JSON message “Hello, World!”.
React.js Frontend Integration with Gin
React.js is a popular JavaScript library for building user interfaces. Integrating React.js with Gin allows us to create useful SPAs with efficient backends. In this section, we will explore how to integrate React.js with Gin and build a basic SPA.
Setting up a React.js Project
To get started, make sure you have Node.js and npm (Node Package Manager) installed on your machine. Open your terminal and run the following command to create a new React.js project:
npx create-react-app my-app
This command will create a new directory called my-app
with a basic React.js project structure.
Creating a React Component
Next, let’s create a simple React component. Open the src/App.js
file in your project and replace the existing code with the following:
import React from 'react'; function App() { return ( <div> <h1>Hello, React!</h1> </div> ); } export default App;
In this code, we define a functional component called App
that returns a div
element with an h1
heading.
Integrating React.js with Gin
To integrate React.js with Gin, we need to build the React.js project and serve the static files using Gin. Open the main.go
file in your Gin server project and update it with the following code:
package main import ( "net/http" "github.com/gin-gonic/gin" ) func main() { r := gin.Default() // Serve static files r.StaticFS("/static", http.Dir("my-app/build/static")) r.StaticFile("/", "my-app/build/index.html") r.Run() // Run the server }
In this code, we serve the static files generated by the React.js build process. The StaticFS
function is used to serve the static files in the my-app/build/static
directory, and the StaticFile
function is used to serve the index.html
file as the default route.
To run the server, execute the following command in your terminal:
go run main.go
You should see the server running and listening on localhost:8080
. If you visit http://localhost:8080
in your browser, you should see the React.js application running.
Vue.js API Design using Gin
Vue.js is another popular frontend framework that can be integrated with Gin to build efficient SPAs. In this section, we will explore how to design APIs for Vue.js apps using Gin.
Setting up a Vue.js Project
To get started, make sure you have Node.js and npm installed on your machine. Open your terminal and run the following command to create a new Vue.js project:
vue create my-app
This command will create a new directory called my-app
with a basic Vue.js project structure.
Creating a Vue Component
Next, let’s create a simple Vue component. Open the src/App.vue
file in your project and replace the existing code with the following:
<template> <div> <h1>Hello, Vue!</h1> </div> </template> <script> export default { name: 'App', } </script>
In this code, we define a Vue component called App
with a template that contains an h1
heading.
Designing APIs with Gin
To design APIs for Vue.js apps using Gin, we can define routes and handlers in our Gin server. Open the main.go
file in your Gin server project and update it with the following code:
package main import ( "github.com/gin-gonic/gin" ) func main() { r := gin.Default() r.GET("/api/hello", func(c *gin.Context) { c.JSON(200, gin.H{ "message": "Hello, Vue!", }) }) r.Run() // Run the server }
In this code, we define a route /api/hello
and respond with a JSON message “Hello, Vue!”. This API can be consumed by our Vue.js app to fetch data from the backend.
To run the server, execute the following command in your terminal:
go run main.go
You should see the server running and listening on localhost:8080
. If you visit http://localhost:8080/api/hello
in your browser, you should see the JSON message “Hello, Vue!”.
Related Article: Exploring Advanced Features of Gin in Golang
HTMX Integration with Gin for Partial Page Updates
HTMX is a JavaScript library that allows for partial page updates without heavy JavaScript. By integrating HTMX with Gin, we can enhance the user experience of our SPAs by dynamically updating parts of the page without reloading the entire page. In this section, we will explore how to integrate HTMX with Gin for partial page updates.
Installing HTMX
To get started, we need to include the HTMX library in our frontend project. Open your terminal and run the following command to install HTMX using npm:
npm install htmx
This command will download and install HTMX in your project.
Performing Partial Page Updates with HTMX
To perform partial page updates with HTMX, we can define routes and handlers in our Gin server that respond with HTML snippets. Open the main.go
file in your Gin server project and update it with the following code:
package main import ( "github.com/gin-gonic/gin" ) func main() { r := gin.Default() r.GET("/profile", func(c *gin.Context) { c.HTML(200, "profile.html", gin.H{ "name": "John Doe", "age": 30, }) }) r.Run() // Run the server }
In this code, we define a route /profile
and respond with an HTML template file called profile.html
. We pass some data to the template, such as the name and age of a user.
Create a new file called templates/profile.html
in your Gin server project and add the following code:
<div> <h1>Welcome, {{ .name }}!</h1> <p>You are {{ .age }} years old.</p> </div>
In this HTML template, we use Go’s template syntax to render the dynamic data passed from the Gin handler.
To run the server, execute the following command in your terminal:
go run main.go
You should see the server running and listening on localhost:8080
. If you visit http://localhost:8080/profile
in your browser, you should see the HTML template rendered with the dynamic data.
Now, we can use HTMX in our frontend project to perform partial page updates. Open the src/App.vue
file in your Vue.js project and update it with the following code:
<template> <div> <h1>Hello, Vue!</h1> <div hx-get="/profile" hx-target="#profile"> <button>Load Profile</button> </div> <div id="profile"></div> </div> </template> <script> export default { name: 'App', } </script>
In this code, we use the hx-get
attribute on a div
element to specify the route /profile
to be fetched using an HTMX request. The fetched HTML is then inserted into the #profile
element.
To test the partial page update, start both the Gin server and the Vue.js development server. Visit http://localhost:3000
in your browser and click the “Load Profile” button. You should see the profile HTML rendered in the #profile
element without reloading the entire page.
Additional Resources
– Go by Example
– Understanding REST APIs
– How REST APIs Work