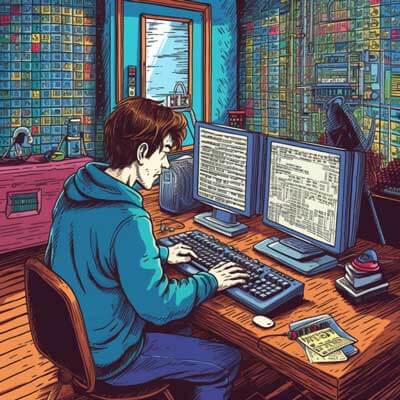
Table of Contents
Neural networks have revolutionized the field of machine learning, enabling us to solve complex problems such as image classification, natural language processing, and speech recognition. PyTorch, a popular deep learning framework, provides a useful and flexible platform for building neural networks. In this article, we will explore the fundamentals of neural networks and how to implement them in PyTorch.
Overview of Neural Networks
Neural networks are a class of machine learning models inspired by the structure and function of the human brain. They consist of interconnected layers of artificial neurons, known as nodes or units. These nodes receive inputs, apply a transformation to them, and produce an output. The connections between nodes are represented by weights, which determine the strength of the signal transmitted between them.
The basic building block of a neural network is the artificial neuron, also known as a perceptron. Each neuron takes a weighted sum of its inputs, applies an activation function to the sum, and produces an output. The activation function introduces non-linearity to the model, enabling it to learn complex patterns and make non-linear predictions.
Related Article: Practical Guide to PyTorch Model Deployment
Backpropagation in Neural Networks
Backpropagation is a key algorithm for training neural networks. It enables the network to learn from the difference between its predicted outputs and the actual outputs, and adjust its weights accordingly. The process involves calculating the gradient of the loss function with respect to the weights of the network, and using this gradient to update the weights through an optimization algorithm such as gradient descent.
The backpropagation algorithm works by propagating the error from the output layer back to the input layer, adjusting the weights of each layer along the way. This process is performed iteratively for a number of epochs until the network converges to a satisfactory solution.
Implementing Backpropagation in PyTorch
PyTorch provides a high-level API for building and training neural networks with ease. Let's take a look at a simple example of implementing backpropagation in PyTorch.
First, we need to define our neural network architecture using the torch.nn
module. This module provides various classes for building different types of layers, activation functions, and loss functions. For example, we can define a simple feedforward neural network with two hidden layers using the torch.nn.Sequential
class:
import torch import torch.nn as nn class NeuralNetwork(nn.Module): def __init__(self): super(NeuralNetwork, self).__init__() self.fc1 = nn.Linear(in_features=784, out_features=128) self.fc2 = nn.Linear(in_features=128, out_features=64) self.fc3 = nn.Linear(in_features=64, out_features=10) def forward(self, x): x = torch.flatten(x, 1) x = torch.relu(self.fc1(x)) x = torch.relu(self.fc2(x)) x = self.fc3(x) return x
In this example, we define a neural network with three fully connected layers. The forward
method defines the forward pass of the network, where the input x
is passed through each layer with a non-linear activation function applied.
To train the network, we need to define a loss function and an optimizer. PyTorch provides a wide range of loss functions and optimizers to choose from. For example, we can use the cross-entropy loss function and the stochastic gradient descent optimizer:
network = NeuralNetwork() criterion = nn.CrossEntropyLoss() optimizer = torch.optim.SGD(network.parameters(), lr=0.01)
Once we have defined our network architecture, loss function, and optimizer, we can start the training process. The general steps for training a neural network in PyTorch are as follows:
1. Initialize the network and optimizer.
2. Iterate over the training dataset.
3. Perform a forward pass to obtain the predicted outputs.
4. Calculate the loss between the predicted outputs and the ground truth labels.
5. Perform a backward pass (backpropagation) to compute the gradients.
6. Update the weights and biases of the network using the optimizer.
7. Repeat steps 3-6 for a number of epochs.
Here is an example of training the network on a dataset:
for epoch in range(num_epochs): for images, labels in train_loader: optimizer.zero_grad() outputs = network(images) loss = criterion(outputs, labels) loss.backward() optimizer.step()
This code snippet demonstrates the main training loop, where we iterate over the training dataset, perform a forward pass, calculate the loss, perform backpropagation, and update the weights using the optimizer. By repeating this process for a number of epochs, the network gradually learns to make accurate predictions.
Convolutional Neural Networks in PyTorch
Convolutional Neural Networks (CNNs) are a specialized type of neural network commonly used for image classification tasks. They are designed to automatically learn hierarchical representations of images by applying convolutional filters and pooling operations.
PyTorch provides a convenient way to build CNNs using the torch.nn
module. Let's see an example of implementing a simple CNN in PyTorch.
import torch import torch.nn as nn class ConvNet(nn.Module): def __init__(self): super(ConvNet, self).__init__() self.conv1 = nn.Conv2d(1, 32, kernel_size=3) self.conv2 = nn.Conv2d(32, 64, kernel_size=3) self.fc1 = nn.Linear(64 * 5 * 5, 128) self.fc2 = nn.Linear(128, 10) def forward(self, x): x = torch.relu(self.conv1(x)) x = torch.relu(self.conv2(x)) x = torch.flatten(x, 1) x = torch.relu(self.fc1(x)) x = self.fc2(x) return x
This example defines a CNN with two convolutional layers, followed by two fully connected layers. The forward
method defines the forward pass of the network, where the input x
is passed through the convolutional layers with a non-linear activation function applied, and then flattened and passed through the fully connected layers.
Related Article: Creating Custom Datasets and Dataloaders in PyTorch
Advantages of Using Convolutional Neural Networks
Convolutional Neural Networks offer several advantages for image classification tasks:
1. Parameter sharing: CNNs exploit the spatial correlation in images by sharing weights across different regions of the input. This reduces the number of parameters and enables the network to learn more efficiently.
2. Translation invariance: CNNs are able to recognize objects in images regardless of their position, thanks to the use of convolutional filters and pooling operations.
3. Hierarchical representations: CNNs learn to extract hierarchical representations of images, capturing low-level features such as edges and textures, and gradually building up to higher-level features such as objects and scenes.
4. Robust to variations: CNNs are robust to variations in scale, rotation, and translation, making them suitable for real-world applications where the input images may have different orientations or sizes.
Recurrent Neural Networks in PyTorch
Recurrent Neural Networks (RNNs) are a class of neural networks particularly suited for sequential data, such as time series or natural language. They have the ability to process inputs of arbitrary length and maintain an internal state, which allows them to capture temporal dependencies and context.
PyTorch provides a simple way to build RNNs using the torch.nn
module. Let's take a look at an example of implementing a simple RNN in PyTorch.
import torch import torch.nn as nn class RNN(nn.Module): def __init__(self, input_size, hidden_size, output_size): super(RNN, self).__init__() self.hidden_size = hidden_size self.rnn = nn.RNN(input_size, hidden_size, batch_first=True) self.fc = nn.Linear(hidden_size, output_size) def forward(self, x): h0 = torch.zeros(1, x.size(0), self.hidden_size).to(x.device) out, _ = self.rnn(x, h0) out = self.fc(out[:, -1, :]) return out
In this example, we define an RNN with a single recurrent layer. The forward
method defines the forward pass of the network, where the input x
is passed through the recurrent layer and the output is passed through a fully connected layer.
RNNs are widely used in natural language processing tasks such as text generation, sentiment analysis, and machine translation. They are also used in time series analysis tasks such as stock market prediction and speech recognition.
Implementing Activation Functions
Activation functions play a crucial role in neural networks by introducing non-linearity to the model. They help the network to learn complex patterns and make non-linear predictions.
PyTorch provides a wide range of activation functions in the torch.nn
module. Here are some commonly used activation functions and their implementations in PyTorch:
- ReLU (Rectified Linear Unit):
torch.relu(x)
- Sigmoid:
torch.sigmoid(x)
- Tanh (Hyperbolic Tangent):
torch.tanh(x)
- Softmax:
torch.softmax(x, dim=1)
These activation functions can be applied to the output of each layer in the neural network to introduce non-linearity and improve the network's ability to learn complex patterns.
Activation Functions in Neural Networks
Activation functions are an essential component of neural networks. They introduce non-linearity to the model, allowing it to learn complex patterns and make non-linear predictions.
Here are some key activation functions commonly used in neural networks:
- ReLU (Rectified Linear Unit): The ReLU function returns the input if it is positive, and zero otherwise. It is widely used in deep learning models due to its simplicity and effectiveness in handling the vanishing gradient problem.
- Sigmoid: The sigmoid function maps the input to a value between 0 and 1. It is often used in binary classification tasks where the output represents the probability of the input belonging to a certain class.
- Tanh (Hyperbolic Tangent): The tanh function maps the input to a value between -1 and 1. It is similar to the sigmoid function but has a steeper gradient, making it more effective in capturing non-linear patterns.
- Softmax: The softmax function is used in multi-class classification tasks to convert the output of the model into a probability distribution over the classes. It ensures that the predicted probabilities sum up to 1.
Choosing the right activation function depends on the nature of the problem and the characteristics of the data. Experimentation and empirical evaluation are often necessary to determine the most suitable activation function for a given task.
Related Article: Comparing PyTorch and TensorFlow
Gradient Descent in Neural Networks
Gradient descent is a popular optimization algorithm used to train neural networks. It iteratively updates the weights of the network in the direction of the steepest descent of the loss function.
The basic idea behind gradient descent is to calculate the gradient of the loss function with respect to the weights of the network, and adjust the weights by taking a small step in the opposite direction of the gradient. This process is repeated for a number of iterations until the network converges to a satisfactory solution.
There are different variants of gradient descent, including batch gradient descent, mini-batch gradient descent, and stochastic gradient descent. Batch gradient descent calculates the gradient using the entire training dataset, while mini-batch gradient descent and stochastic gradient descent use a subset or a single training example, respectively.
PyTorch provides a convenient way to perform gradient descent using the torch.optim
module. It offers various optimization algorithms such as stochastic gradient descent (SGD), Adam, and RMSprop. These optimizers handle the computation of gradients and the updating of weights automatically, allowing us to focus on defining the network architecture and the training process.
Additional Resources
- Implementing Backpropagation in PyTorch