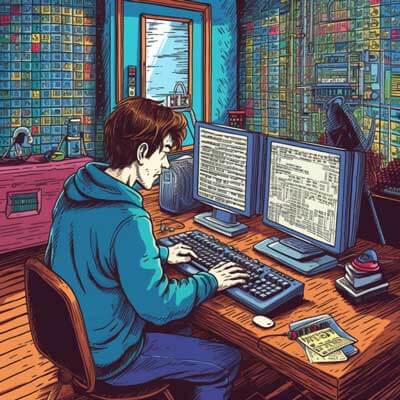
Table of Contents
Exploring Deep Learning Concepts
Deep learning is a subfield of machine learning that focuses on the development and application of artificial neural networks. These neural networks are inspired by the structure and function of the human brain and are capable of learning from large amounts of data to make accurate predictions or classifications.
Deep learning has gained significant traction in recent years due to its ability to handle complex tasks such as image and speech recognition, natural language processing, and autonomous driving. It has revolutionized many industries, including healthcare, finance, and technology.
At the core of deep learning are neural networks, which are composed of interconnected layers of artificial neurons called nodes. Each node takes in input data, applies a mathematical function to it, and produces an output. The output is then passed on to the next layer of nodes until a final output is generated.
Training a neural network involves feeding it with labeled training data and adjusting the weights and biases of the nodes to minimize the difference between the predicted output and the actual output. This process is known as backpropagation and is a key component of deep learning.
Related Article: Data Loading and Preprocessing in PyTorch
Machine Learning Frameworks
Machine learning frameworks provide the tools and libraries necessary to implement and train deep learning models efficiently. They offer a wide range of functionalities, including data preprocessing, model building, training, and evaluation.
Two popular machine learning frameworks in the deep learning community are PyTorch and TensorFlow. While both frameworks are widely used and have their strengths, they have different design philosophies and approaches to deep learning.
PyTorch, developed by Facebook's AI research lab, is a dynamic framework that emphasizes ease of use and flexibility. It allows developers to define and modify computational graphs on the fly, making it suitable for rapid prototyping and experimentation. PyTorch's dynamic nature also enables more intuitive debugging and easier integration with Python libraries.
TensorFlow, on the other hand, was developed by Google and is known for its static computational graph. It emphasizes scalability and production readiness, making it a popular choice for large-scale deployments. TensorFlow's static graph allows for optimization and distributed training, but it may require more effort to modify and experiment with the models.
The Importance of GPU Support
Deep learning models require significant computational power to train and infer accurately. Graphics Processing Units (GPUs) have become an essential tool in deep learning due to their ability to perform parallel computations efficiently. GPUs can process large amounts of data simultaneously, reducing training times and enabling the training of more complex models.
Both PyTorch and TensorFlow provide GPU support, allowing developers to leverage the power of GPUs to accelerate deep learning tasks. By utilizing GPUs, developers can significantly reduce training times and achieve faster inference speeds, making it feasible to train larger and more complex models.
Enabling GPU support in PyTorch and TensorFlow is relatively straightforward. Both frameworks provide APIs that allow developers to allocate and transfer data to the GPU and perform computations on it. By utilizing these APIs, developers can seamlessly integrate GPU acceleration into their deep learning workflows.
An In-depth Look at Autograd
Autograd, short for automatic differentiation, is a fundamental component of deep learning frameworks that allows for efficient computation of gradients. Gradients are essential for optimizing the parameters of a neural network during training.
In PyTorch, autograd is a key feature that enables automatic differentiation of computations. It keeps track of the operations performed on tensors and automatically computes the gradients of the operations with respect to the input tensors. This feature eliminates the need for manual computation of gradients, significantly simplifying the training process.
Let's take a look at a simple example to illustrate how autograd works in PyTorch:
import torchx = torch.tensor(2.0, requires_grad=True)y = x**2 + 2*x + 1y.backward()print(x.grad) # Output: 6.0
In this example, we define a tensor x
with requires_grad=True
, indicating that we want to compute gradients with respect to x
. We then perform some computations on x
to obtain the tensor y
. By calling y.backward()
, PyTorch automatically computes the gradients of y
with respect to x
using the chain rule. Finally, we can access the gradients of x
by accessing the grad
attribute of x
.
TensorFlow also provides automatic differentiation functionality through its Automatic Differentiation (tf.GradientTape) API. It allows developers to compute gradients with respect to variables in a computation graph. TensorFlow's automatic differentiation is similar to PyTorch's autograd and provides a convenient way to compute gradients without manual intervention.
Related Article: PyTorch Application in Natural Language Processing
Comparing PyTorch and TensorFlow for Natural Language Processing
Natural Language Processing (NLP) is a subfield of artificial intelligence that focuses on the interaction between computers and human language. It involves tasks such as language translation, sentiment analysis, and text generation. Deep learning has had a significant impact on NLP, enabling the development of more accurate and robust models.
Both PyTorch and TensorFlow provide useful tools and libraries for NLP tasks. However, they have different approaches and APIs for handling NLP-specific tasks.
PyTorch's flexibility and dynamic nature make it an excellent choice for NLP research and experimentation. It provides a wide range of tools and libraries, such as torchtext and transformers, that facilitate natural language processing tasks. PyTorch's dynamic computational graph allows for easy model customization and integration with other Python libraries commonly used in NLP.
TensorFlow, on the other hand, has a more extensive ecosystem and is well-suited for large-scale NLP deployments. It provides high-level APIs like TensorFlow Hub and TensorFlow Text that simplify common NLP tasks. TensorFlow's static computational graph allows for efficient computation and optimization, making it suitable for production-ready NLP models.
When choosing between PyTorch and TensorFlow for NLP, it's essential to consider the specific requirements of the project and the trade-offs between flexibility and scalability.
Key Differences in GPU Support Between PyTorch and TensorFlow
While both PyTorch and TensorFlow provide GPU support, there are some key differences in how they handle GPU acceleration.
PyTorch's GPU support is seamless and intuitive. Developers can easily move tensors to the GPU using the .to()
method and perform computations on them. PyTorch also supports multi-GPU training out of the box, allowing for efficient parallelization of computations across multiple GPUs.
TensorFlow, on the other hand, requires a bit more configuration for GPU support. Developers need to explicitly define and manage GPU devices using TensorFlow's tf.device()
context manager. TensorFlow also provides APIs for distributed training, allowing for the efficient utilization of multiple GPUs or even multiple machines.
Another difference is in how the two frameworks handle memory management on the GPU. PyTorch uses a caching memory allocator that can lead to more efficient memory usage, especially in cases where the memory requirements vary dynamically. TensorFlow, on the other hand, uses a static memory allocator that can be more efficient for models with fixed memory requirements.
When it comes to GPU support, both PyTorch and TensorFlow provide robust functionality. The choice between the two depends on the specific requirements of the project and the level of control and flexibility desired.
Analyzing PyTorch's Autograd Functionality vs TensorFlow's Automatic Differentiation
PyTorch's autograd and TensorFlow's automatic differentiation provide similar functionality for computing gradients. However, there are some differences in their implementation and usage.
PyTorch's autograd is based on a dynamic computational graph. It dynamically builds a graph of operations as they are executed and keeps track of the operations for gradient computation. This dynamic nature allows for easy model customization and debugging, as the graph can be modified on the fly. PyTorch's autograd also supports higher-order gradients, making it suitable for advanced optimization techniques.
TensorFlow's automatic differentiation, on the other hand, is based on a static computational graph. The graph needs to be defined upfront, and operations are added to the graph before execution. This static nature allows for efficient computation and optimization, especially in production-ready models. TensorFlow's automatic differentiation also supports distributed training, making it suitable for large-scale deployments.
Both autograd and automatic differentiation handle gradient computation efficiently and accurately. The choice between PyTorch and TensorFlow depends on the specific requirements of the project and the desired level of flexibility and control.
Additional Resources
- PyTorch vs. TensorFlow for Natural Language Processing