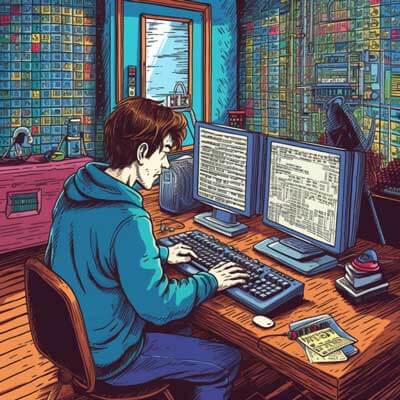
Table of Contents
ReactJS and VueJS are two popular JavaScript frameworks used for building dynamic user interfaces. Both frameworks provide tools and mechanisms for managing state and reactivity, allowing developers to create responsive and interactive web applications. However, there are some differences in how reactivity is handled in ReactJS and VueJS. In this article, we will compare the reactivity features of ReactJS and VueJS variables and explore their similarities and differences.
Difference between ReactJS State and VueJS Variables
While both ReactJS and VueJS provide mechanisms for managing state and reactivity, there are some differences between ReactJS state and VueJS variables.
In ReactJS, state is a JavaScript object that holds data that can change over time. State is managed within a component and can be updated using the setState
function, triggering a re-render of the component. ReactJS state is local to the component and cannot be accessed or modified from other components.
On the other hand, VueJS uses reactive variables that are defined in the data
property of a component. These variables are automatically made reactive by VueJS, meaning that any changes to these variables will trigger updates in the component's template. Unlike ReactJS state, VueJS variables can be accessed and modified from other components using the VueJS instance.
Another difference is how state and variables are updated. In ReactJS, state updates are performed by calling the setState
function with the new value of the state. ReactJS then compares the previous state with the new state and updates the component accordingly. In VueJS, variables are updated directly by assigning a new value to them. VueJS then detects the change and updates the component's template.
Related Article: Exploring Differences in Rendering Components in ReactJS
How ReactJS Manages State
ReactJS provides a built-in state management system that allows developers to manage and update the state of a component. State is an object that holds data that can be changed over time and triggers a re-render of the component when updated.
ReactJS manages state by using the useState
hook or the this.state
property in class components. The useState
hook is a function that returns an array with two elements: the current state value and a function to update the state. Here's an example of using the useState
hook:
import React, { useState } from 'react'; const Counter = () => { const [count, setCount] = useState(0); const increment = () => { setCount(count + 1); }; return ( <div> <p>Count: {count}</p> <button onClick={increment}>Increment</button> </div> ); }; export default Counter;
In this example, we define a functional component called Counter
. We use the useState
hook to define a state variable called count
and a function called setCount
to update the state. The initial value of count
is set to 0. We also define an increment
function that updates the count
state by incrementing it by 1 when the button is clicked. The count
state is then rendered inside a p
tag.
ReactJS manages state by internally storing the current state value and the function to update the state. When the state is updated using the update function, ReactJS compares the previous state with the new state and updates the component accordingly. This process is known as reconciliation and allows ReactJS to efficiently update the DOM.
What are ReactJS Hooks and How Do They Work?
ReactJS introduced hooks in version 16.8 as a way to use state and other React features without writing a class. Hooks allow developers to write reusable logic and stateful components in a more concise and functional way.
Hooks are functions that can be used inside functional components to access React features. The most commonly used hook is the useState
hook, which allows functional components to have state. Hooks are called inside the body of a functional component and cannot be called outside of a component or in a regular JavaScript function.
Hooks work by leveraging closures and the call order of the hooks inside a component. When a functional component is rendered, ReactJS calls the hooks in the same order every time, ensuring that the state is preserved between renders. This allows functional components to have state and other features that were previously only available in class components.
Here's an example of using the useState
hook to manage state in a functional component:
import React, { useState } from 'react'; const Example = () => { const [text, setText] = useState(''); const handleChange = (event) => { setText(event.target.value); }; return ( <div> <input type="text" value={text} onChange={handleChange} /> <p>Entered text: {text}</p> </div> ); }; export default Example;
In this example, we define a functional component called Example
. We use the useState
hook to define a state variable called text
and a function called setText
to update the state. The initial value of text
is set to an empty string. We also define a handleChange
function that updates the text
state whenever the user types in the input field. The text
state is then rendered inside a p
tag.
Hooks provide a way to reuse stateful logic between components, making it easier to manage complex state and state transitions. They also make functional components more useful and flexible, reducing the need for class components in many cases.
Differences between ReactJS Props and VueJS Variables
ReactJS and VueJS both provide mechanisms for passing data between components, but there are some differences between ReactJS props and VueJS variables.
In ReactJS, props are a way to pass data from a parent component to a child component. Props are read-only and cannot be modified by the child component. ReactJS components receive props as function parameters or by accessing the props
object. Here's an example:
import React from 'react'; const Greeting = (props) => { return ( <div> <p>Hello, {props.name}!</p> </div> ); }; export default Greeting;
In this example, we define a functional component called Greeting
that receives a prop called name
. The name
prop is then rendered inside a p
tag. The value of the name
prop is passed from the parent component when using the Greeting
component.
In VueJS, variables are defined in the data
property of a component and are automatically made reactive by VueJS. VueJS variables can be accessed and modified by the component itself as well as its child components. Here's an example:
<template> <div> <p>Hello, {{ name }}!</p> </div> </template> <script> export default { data() { return { name: 'John' }; } }; </script>
In this example, we define a VueJS component that has a data
property with a variable called name
. The name
variable is then rendered inside a p
tag using the double curly brace syntax. The name
variable can be accessed and modified within the component and its child components.
Another difference is how data is passed from a parent component to a child component. In ReactJS, data is passed as props when using the child component. In VueJS, data is accessed directly within the template of the child component using the variable defined in the parent component.
Overall, ReactJS props and VueJS variables serve similar purposes of passing data between components, but have some differences in how they are defined, accessed, and modified. Understanding these differences is important when working with ReactJS and VueJS components.
Related Article: How to Implement Custom CSS in ReactJS
ReactJS Component Lifecycle and How It Works
ReactJS components have a lifecycle that consists of different phases, allowing developers to hook into specific moments in a component's lifespan. The lifecycle methods provide control over when a component is rendered, updated, or destroyed.
The ReactJS component lifecycle can be divided into three main phases: mounting, updating, and unmounting.
Mounting Phase
The mounting phase occurs when a component is being initialized and inserted into the DOM for the first time. During this phase, the following methods are called:
- constructor()
: This is the first method called during the mounting phase. It is used to initialize the component's state and bind event handlers.
- static getDerivedStateFromProps()
: This method is called right before rendering the component and allows the component to update its state based on changes in props.
- render()
: This method is called to generate the JSX that will be rendered on the screen.
- componentDidMount()
: This method is called after the component has been rendered to the DOM. It is commonly used to fetch data from an API or set up event listeners.
Here's an example of a component with the mounting phase methods:
import React from 'react'; class App extends React.Component { constructor(props) { super(props); // Initialize state and bind event handlers } static getDerivedStateFromProps(nextProps, prevState) { // Update state based on props } render() { // Generate JSX } componentDidMount() { // Perform side effects after rendering } render() { return ( <div> <p>Hello, World!</p> </div> ); } } export default App;
In this example, the constructor
method is used to initialize the component's state and bind event handlers. The getDerivedStateFromProps
method is used to update the state based on changes in props. The render
method generates the JSX that will be rendered on the screen. Finally, the componentDidMount
method is used to perform any side effects after the component has been rendered.
Updating Phase
The updating phase occurs when a component is re-rendered due to changes in props or state. During this phase, the following methods are called:
- static getDerivedStateFromProps()
: This method is called before rendering the component and allows the component to update its state based on changes in props.
- shouldComponentUpdate()
: This method is called before re-rendering the component and allows the component to control whether the re-rendering should occur or not.
- render()
: This method is called to generate the JSX that will be rendered on the screen.
- getSnapshotBeforeUpdate()
: This method is called right before the changes from the virtual DOM have been applied to the actual DOM. It allows the component to capture information from the DOM before it is potentially changed.
- componentDidUpdate()
: This method is called after the component has been re-rendered. It is commonly used to perform side effects after a component update, such as fetching new data based on props or state changes.
Here's an example of a component with the updating phase methods:
import React from 'react'; class Counter extends React.Component { constructor(props) { super(props); // Initialize state and bind event handlers } static getDerivedStateFromProps(nextProps, prevState) { // Update state based on props } shouldComponentUpdate(nextProps, nextState) { // Control whether re-rendering should occur } getSnapshotBeforeUpdate(prevProps, prevState) { // Capture information from the DOM } componentDidUpdate(prevProps, prevState, snapshot) { // Perform side effects after re-rendering } render() { // Generate JSX } } export default Counter;
In this example, the getDerivedStateFromProps
method is used to update the state based on changes in props. The shouldComponentUpdate
method controls whether the re-rendering should occur or not. The render
method generates the JSX that will be rendered on the screen. The getSnapshotBeforeUpdate
method allows the component to capture information from the DOM before it is potentially changed. Finally, the componentDidUpdate
method is used to perform any side effects after the component has been re-rendered.
Unmounting Phase
The unmounting phase occurs when a component is being removed from the DOM. During this phase, the following method is called:
- componentWillUnmount()
: This method is called before the component is removed from the DOM. It is commonly used to clean up any resources or event listeners created in the componentDidMount
method.
Here's an example of a component with the unmounting phase method:
import React from 'react'; class App extends React.Component { componentDidMount() { // Set up event listeners or start timers } componentWillUnmount() { // Clean up resources or event listeners } render() { return ( <div> <p>Hello, World!</p> </div> ); } } export default App;
In this example, the componentDidMount
method is used to set up event listeners or start timers. The componentWillUnmount
method is used to clean up any resources or event listeners created in the componentDidMount
method.
The ReactJS component lifecycle provides developers with control over different phases of a component's lifespan. By using the lifecycle methods, developers can perform side effects, update state, and handle component updates in a controlled manner.
Related Article: Implementing Server Rendering with Ruby on Rails & ReactJS
How ReactJS Handles Events
ReactJS provides a simple and efficient way to handle events in components. Event handlers are functions that are called when a specific event occurs, such as a button click or input change.
In ReactJS, event handlers are attached to elements using the onEventName
syntax, where EventName
is the name of the event. Event handlers can be defined as methods in class components or as functions in functional components.
Here's an example of handling a button click event in a class component:
import React from 'react'; class Button extends React.Component { handleClick() { console.log('Button clicked'); } render() { return ( <button onClick={this.handleClick}>Click me</button> ); } } export default Button;
In this example, we define a class component called Button
that has a handleClick
method. The handleClick
method logs a message to the console when the button is clicked. The handleClick
method is then attached to the onClick
event of the button using the onClick
attribute.
Here's an example of handling a button click event in a functional component:
import React from 'react'; const Button = () => { const handleClick = () => { console.log('Button clicked'); }; return ( <button onClick={handleClick}>Click me</button> ); }; export default Button;
In this example, we define a functional component called Button
that uses the useState
hook to define a state variable called count
and a function called setCount
to update the state. We also define a handleClick
function that logs a message to the console when the button is clicked. The handleClick
function is then attached to the onClick
event of the button.
ReactJS event handling follows the standard DOM event handling model, but with some differences. ReactJS uses synthetic events, which are cross-browser compatible and have consistent behavior. ReactJS also automatically binds the event handler to the component instance, so you don't need to manually bind the this
context.
ReactJS Reconciliation and Rendering
ReactJS uses a process called reconciliation to update the DOM efficiently. Reconciliation is the process of comparing the previous virtual DOM tree with the new virtual DOM tree and determining the minimal set of changes needed to update the actual DOM.
When a component's state or props change, React creates a new virtual DOM tree by applying the changes to the previous virtual DOM tree. React then performs a diffing algorithm to compare the new virtual DOM tree with the previous one. The diffing algorithm determines which parts of the virtual DOM have changed and generates a list of updates needed to bring the actual DOM in sync with the new virtual DOM.
React uses the list of updates to perform the necessary changes to the actual DOM. React updates the DOM in a batched manner to minimize the number of updates and improve performance. React also takes advantage of the virtual DOM's ability to batch updates and perform them in an optimized way.
Reconciliation is an efficient way to update the DOM because React only updates the parts of the DOM that have changed. This reduces the number of DOM updates and improves performance, especially when dealing with complex and large applications.
React's reconciliation algorithm is optimized to perform updates in an efficient and predictable manner. It uses heuristics to determine the most optimal way to update the DOM and minimize the impact on performance. React also provides mechanisms like shouldComponentUpdate and PureComponent to further optimize the update process and avoid unnecessary re-renders.
In addition to reconciliation, React also provides mechanisms like the virtual DOM and diffing algorithm to further optimize rendering. The virtual DOM allows React to perform updates in a more efficient way by batching the changes and updating the actual DOM only when necessary. The diffing algorithm compares the new virtual DOM tree with the previous one and generates a list of updates needed to bring the actual DOM in sync with the new virtual DOM.
Overall, ReactJS reconciliation and rendering are key features that enable efficient updates and rendering of components. They play a crucial role in optimizing performance and providing a smooth user experience in ReactJS applications.