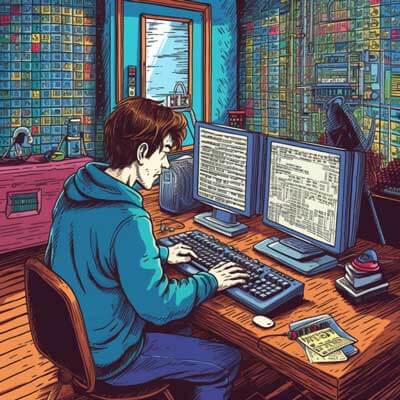
Table of Contents
Using Index for Substring Comparison
One way to compare substrings in Python is by using the index method. This method returns the starting index of the first occurrence of a substring within a string. If the substring is not found, it returns -1.
Here's an example that demonstrates how to use the index method for substring comparison:
string = "Hello, World!" substring = "Hello" if string.index(substring) != -1: print("Substring found!") else: print("Substring not found!")
In this example, the index method is used to check if the substring "Hello" is present in the string "Hello, World!". If the index is not -1, it means the substring is found and the corresponding message is printed.
Related Article: How To Set Environment Variables In Python
Using Slice for Substring Comparison
Another approach to compare substrings in Python is by using the slice notation. The slice notation allows you to extract a portion of a string based on its indices.
Here's an example that demonstrates how to use slice notation for substring comparison:
string = "Hello, World!" substring = "Hello" if substring in string[:len(substring)]: print("Substring found!") else: print("Substring not found!")
In this example, the slice notation string[:len(substring)]
is used to extract the first len(substring)
characters from the string. The in
operator is then used to check if the substring is present in the extracted portion.
Comparing Substrings with Substring Function
Python provides a built-in function called substring()
that can be used to compare substrings. The substring()
function takes two parameters: the string to search in, and the substring to search for. It returns True
if the substring is found, and False
otherwise.
Here's an example that demonstrates how to use the substring()
function for substring comparison:
string = "Hello, World!" substring = "Hello" if substring(string, substring): print("Substring found!") else: print("Substring not found!")
In this example, the substring()
function is used to check if the substring "Hello" is present in the string "Hello, World!". If the function returns True
, it means the substring is found and the corresponding message is printed.
Comparing Substrings with Substring Method
In addition to the substring()
function, Python also provides a substring()
method that can be called directly on a string object. The substring()
method works in a similar way to the function, taking a substring as an argument and returning True
if it is found, and False
otherwise.
Here's an example that demonstrates how to use the substring()
method for substring comparison:
string = "Hello, World!" substring = "Hello" if string.substring(substring): print("Substring found!") else: print("Substring not found!")
In this example, the substring()
method is called on the string object string
to check if the substring "Hello" is present. If the method returns True
, it means the substring is found and the corresponding message is printed.
Related Article: How to Use Python Pip Install on MacOS
Code Snippet: Comparing Substrings in Python
Here's a code snippet that demonstrates different approaches to comparing substrings in Python:
def compare_substrings(string, substring): if string.index(substring) != -1: print("Using index: Substring found!") else: print("Using index: Substring not found!") if substring in string[:len(substring)]: print("Using slice: Substring found!") else: print("Using slice: Substring not found!") if substring(string, substring): print("Using function: Substring found!") else: print("Using function: Substring not found!") if string.substring(substring): print("Using method: Substring found!") else: print("Using method: Substring not found!") string = "Hello, World!" substring = "Hello" compare_substrings(string, substring)
In this code snippet, a function compare_substrings()
is defined to compare substrings using different methods discussed earlier. The function takes a string and a substring as parameters, and calls the respective methods to check if the substring is present.
Examples of Substring Comparison in Python
Let's explore a few examples of comparing substrings in Python to understand how these methods can be applied in practice.
Example 1: Checking if a URL contains a specific domain
url = "https://www.example.com/path/to/resource" domain = "example.com" if domain in url: print("URL contains the domain!") else: print("URL does not contain the domain!")
In this example, we check if the given URL contains the domain "example.com" by using the in
operator. If the domain is found in the URL, the corresponding message is printed.
Example 2: Validating user input
username = input("Enter your username: ") if len(username) >= 3 and len(username) <= 10: print("Username is valid!") else: print("Username is invalid!")
In this example, we validate the user input for a username by checking if its length is between 3 and 10 characters using the len()
function. If the username meets the criteria, the corresponding message is printed.
Example 3: Searching for a specific word in a sentence
sentence = "The quick brown fox jumps over the lazy dog." word = "fox" if word in sentence: print("Word found in the sentence!") else: print("Word not found in the sentence!")
In this example, we search for the word "fox" in a given sentence using the in
operator. If the word is found, the corresponding message is printed.
Additional Resources
- String Comparison in Python (compare() and casefold())