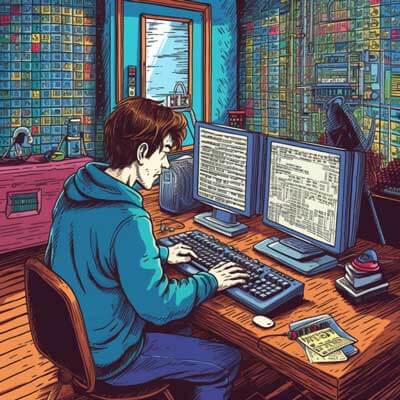
Table of Contents
Basics of Using Locale in Python
Related Article: How to Round Up a Number in Python
Understanding Locale in Python
Locale is an essential aspect of software development that deals with language, cultural conventions, and formatting preferences. In Python, the locale module provides functionalities to handle various locale-related tasks. It allows developers to format numbers, dates, times, currencies, and sort data based on different cultural conventions.
import locale # Set the locale to the user's default locale.setlocale(locale.LC_ALL, '') # Get the current locale current_locale = locale.getlocale() print(current_locale)
Formatting Numbers with Locale
The locale module enables you to format numbers according to specific cultural conventions. By using the format()
function along with the appropriate locale settings, you can achieve localized number formatting.
import locale # Set the locale to French (France) locale.setlocale(locale.LC_ALL, 'fr_FR') # Format a number using French number formatting rules formatted_number = locale.format_string("%.2f", 12345.6789) print(formatted_number)
Formatting Dates and Times with Locale
In addition to numbers, you can also format dates and times based on different local conventions. The strftime() function allows you to customize date and time representations using format codes.
import datetime import locale # Set the locale to German (Germany) locale.setlocale(locale.LC_ALL, 'de_DE') # Format the current date and time using German conventions formatted_datetime = datetime.datetime.now().strftime("%A, %d %B %Y - %H:%M") print(formatted_datetime)
Related Article: How to Add New Keys to a Python Dictionary
All Features of Python Locale
Numeric Formatting with Locale
The locale module provides various features for numeric formatting. You can format numbers as currency, percentages, or scientific notation.
import locale # Set the locale to the user's default locale.setlocale(locale.LC_ALL, '') # Format a number as currency formatted_currency = locale.currency(12345.67) print(formatted_currency) # Format a number as percentage formatted_percentage = locale.format_string("%.2f%%", 0.75) print(formatted_percentage) # Format a number in scientific notation formatted_scientific = locale.format_string("%.2e", 12345.67) print(formatted_scientific)
Date and Time Formatting with Locale
In addition to basic date and time formatting, the locale module allows you to format timestamps, weekdays, and months according to specific cultural conventions.
import datetime import locale # Set the locale to Spanish (Spain) locale.setlocale(locale.LC_ALL, 'es_ES') # Format a timestamp using Spanish conventions formatted_timestamp = datetime.datetime.now().strftime("%c") print(formatted_timestamp) # Get the full weekday name in Spanish for Monday (1) weekday_name = datetime.date(2022, 1, 31).strftime("%A") print(weekday_name) # Get the abbreviated month name in Spanish for February (2) month_name = datetime.date(2022, 2, 1).strftime("%b") print(month_name)
Use Cases for Python Locale
Related Article: How to Change Column Type in Pandas
Multi-Language Support in Web Applications
Python's locale module is often used in web applications that require multi-language support. By utilizing different locales based on user preferences or browser settings, developers can dynamically display content in various languages.
Internationalization of Financial Applications
Locale plays a crucial role in financial applications that deal with currency conversions, exchange rates, and formatting. Python's locale module allows developers to handle these aspects efficiently, ensuring accurate and localized financial information.
Best Practices for Utilizing Python Locale
Use Unicode Strings
When working with locales, it is essential to use Unicode strings to avoid encoding issues. By using Unicode strings throughout your codebase, you can ensure seamless compatibility with different languages and character sets.
Related Article: How To Fix The 'No Module Named Pip' Error
Avoid Hard-Coding Locale Settings
To make your code more flexible and adaptable, avoid hard-coding specific locale settings. Instead, consider retrieving the user's preferred locale from their profile or using browser headers to determine the appropriate settings dynamically.
Performance Considerations for Python Locale
Caching Locale Settings
In scenarios where locale settings do not change frequently, caching them can significantly improve performance. By storing frequently used locale settings in memory or database systems, you can minimize the overhead of retrieving and setting them repeatedly.
Avoid Overusing Dynamic Formatting
Dynamic formatting functions provided by the locale module can be resource-intensive. Avoid excessive use of dynamic formatting in performance-critical sections of your code to prevent unnecessary slowdowns.
Related Article: How to Convert String to Bytes in Python 3
Code Snippet Ideas: Formatting Dates and Times
import datetime import locale # Set the locale to French (France) locale.setlocale(locale.LC_ALL, 'fr_FR') # Format a date using French conventions formatted_date = datetime.date(2022, 12, 31).strftime("%A %d %B %Y") print(formatted_date) # Format a time using French conventions formatted_time = datetime.time(14, 30).strftime("%H:%M") print(formatted_time)
Code Snippet Ideas: Number Formatting and Localization
import locale # Set the locale to German (Germany) locale.setlocale(locale.LC_ALL, 'de_DE') # Format a large number with localized thousand separators formatted_number = locale.format_string("%d", 1234567890, grouping=True) print(formatted_number) # Parse a localized number string into an actual number localized_number_string = "1.234.567,89" parsed_number = locale.atof(localized_number_string) print(parsed_number)
Code Snippet Ideas: Currency Formatting and Exchange Rates
import locale # Set the locale to British English (United Kingdom) locale.setlocale(locale.LC_ALL, 'en_GB') # Format a currency value with the appropriate symbol formatted_currency = locale.currency(1234.56, grouping=True) print(formatted_currency) # Convert an amount from one currency to another based on exchange rates amount_in_euros = 1000.00 exchange_rate_usd_to_eur = 1.18 amount_in_usd = amount_in_euros * exchange_rate_usd_to_eur formatted_amount_in_usd = locale.currency(amount_in_usd, grouping=True) print(formatted_amount_in_usd)
Code Snippet Ideas: Sorting and Collation with Locale
import locale # Set the locale to Spanish (Spain) locale.setlocale(locale.LC_ALL, 'es_ES') fruits = ['manzana', 'naranja', 'plátano', 'limón'] sorted_fruits = sorted(fruits, key=locale.strxfrm) print(sorted_fruits)
Related Article: How To Merge Dictionaries In Python
Advanced Technique: Customizing Locale Settings in Python
Creating a Custom Locale Object
In Python, you can create custom locale objects with specific settings. This allows you to define and use unique locale configurations tailored to your application's requirements.
import locale # Create a custom locale object with specific number formatting custom_locale = locale.localeconv() custom_locale['thousands_sep'] = ' ' custom_locale['decimal_point'] = ',' # Set the custom locale as the current locale locale.setlocale(locale.LC_ALL, custom_locale) # Format a number using the custom locale settings formatted_number = locale.format_string("%d", 1234567890) print(formatted_number)
Advanced Technique: Handling Multiple Locales in a Single Application
Using Context Managers for Locale Switching
In scenarios where an application needs to support multiple locales simultaneously, context managers can be used to temporarily switch between different locales for specific sections of code.
import contextlib import locale @contextlib.contextmanager def temporary_locale(new_locale): original_locale = locale.setlocale(locale.LC_ALL) try: yield locale.setlocale(locale.LC_ALL, new_locale) finally: locale.setlocale(locale.LC_ALL, original_locale) # Example usage: with temporary_locale('fr_FR'): formatted_price = locale.currency(1234.56) print(formatted_price) with temporary_locale('de_DE'): formatted_price = locale.currency(1234.56) print(formatted_price)
Related Article: How to Use Matplotlib for Chinese Text in Python
Advanced Technique: Working with Time Zones in Python Locale
Converting Time Zones
Python provides modules like pytz for working with time zones. By combining the capabilities of the locale module with time zone conversion libraries, you can handle localization and time zone-related tasks effectively.
import datetime import pytz # Set the timezone to UTC timezone_utc = pytz.timezone('UTC') # Set the locale to French (France) locale.setlocale(locale.LC_ALL, 'fr_FR') # Get the current date and time in UTC utc_now = datetime.datetime.now(timezone_utc) # Format the UTC date and time using French conventions formatted_datetime = utc_now.strftime("%A %d %B %Y - %H:%M") print(formatted_datetime)