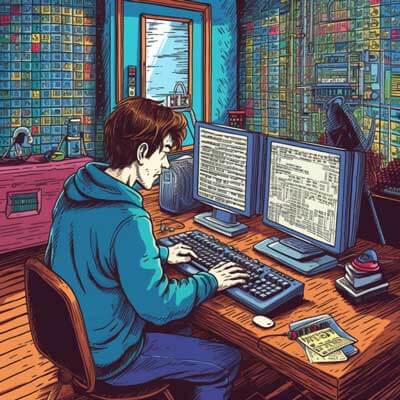
Table of Contents
Overview of Converting cURL Commands
Converting cURL commands to Python is a useful way to automate the process of making HTTP requests in Python applications. cURL is a command-line tool that allows users to send HTTP requests and receive responses from web servers. However, when it comes to writing production-level code, it is often more convenient to use a programming language like Python.
There are several reasons why developers prefer to convert cURL commands to Python. First, Python provides a more expressive and readable syntax compared to the command-line interface of cURL. Second, Python offers useful libraries and modules that simplify the process of making HTTP requests and handling responses. Lastly, by using Python, developers can easily integrate HTTP requests into their existing Python codebase.
In this article, we will explore two popular methods for converting cURL commands to Python: using the requests module and working with the urllib module. We will also cover important concepts such as handling HTTP headers and manipulating JSON data in Python.
Related Article: How to Solve a Key Error in Python
Using the requests module in Python
The requests module is a popular choice for making HTTP requests in Python. It provides a simple and intuitive API that allows developers to send HTTP requests and handle responses effortlessly. To use the requests module, you need to install it first using pip:
$ pip install requests
Once installed, you can import the module in your Python script:
import requests
Sending a GET request
To send a GET request using the requests module, you can use the get()
function. Simply pass the URL of the request as a parameter, and the function will return a Response object containing the server's response:
import requests response = requests.get('https://api.example.com/users')
You can then access the response's status code, headers, and content using the corresponding attributes:
print(response.status_code) # 200 print(response.headers) # {'Content-Type': 'application/json'} print(response.content) # b'{"message": "Hello, world!"}'
Sending a POST request
To send a POST request, you can use the post()
function and pass the URL and the data to be sent in the request body:
import requests data = {'name': 'John Doe', 'email': 'john.doe@example.com'} response = requests.post('https://api.example.com/users', data=data)
You can also send JSON data by passing a JSON object instead of a dictionary:
import requests import json data = {'name': 'John Doe', 'email': 'john.doe@example.com'} response = requests.post('https://api.example.com/users', json=json.dumps(data))
Related Article: How to Sort a Dictionary by Key in Python
Working with the urllib module in Python
The urllib module is another option for making HTTP requests in Python. It is part of the Python standard library, which means you don't need to install any additional packages. The urllib module provides several modules for working with URLs, including urllib.request for making HTTP requests.
To use the urllib module, you can import the urllib.request module in your Python script:
import urllib.request
Sending a GET request
To send a GET request using the urllib module, you can use the urlopen()
function. Simply pass the URL of the request as a parameter, and the function will return a file-like object that represents the server's response:
import urllib.request response = urllib.request.urlopen('https://api.example.com/users')
You can then access the response's status code, headers, and content using the corresponding methods:
print(response.status) # 200 print(response.getheaders()) # [('Content-Type', 'application/json')] print(response.read()) # b'{"message": "Hello, world!"}'
Sending a POST request
To send a POST request using the urllib module, you can use the urlopen()
function with a data parameter. The data should be encoded in bytes before being passed to the function:
import urllib.request import urllib.parse data = urllib.parse.urlencode({'name': 'John Doe', 'email': 'john.doe@example.com'}).encode('utf-8') response = urllib.request.urlopen('https://api.example.com/users', data=data)
You can also send JSON data by encoding it as bytes and setting the Content-Type header to application/json:
import urllib.request import json data = json.dumps({'name': 'John Doe', 'email': 'john.doe@example.com'}).encode('utf-8') req = urllib.request.Request('https://api.example.com/users', data=data) req.add_header('Content-Type', 'application/json') response = urllib.request.urlopen(req)
Handling HTTP headers in Python
HTTP headers contain additional information about the request or response. They can include details such as the content type, content length, and authentication credentials. When converting cURL commands to Python, it is important to handle HTTP headers correctly to ensure the request is properly authenticated and the response is correctly interpreted.
In Python, both the requests module and the urllib module provide methods for adding and accessing HTTP headers.
Related Article: Creating Random Strings with Letters & Digits in Python
Using the requests module
To add headers to a request using the requests module, you can pass a dictionary of headers to the request functions:
import requests headers = {'Authorization': 'Bearer my_token', 'Content-Type': 'application/json'} response = requests.get('https://api.example.com/users', headers=headers)
You can also access the response headers using the headers
attribute of the response object:
print(response.headers['Content-Type']) # application/json
Using the urllib module
To add headers to a request using the urllib module, you can create a Request
object and add headers using the add_header()
method:
import urllib.request req = urllib.request.Request('https://api.example.com/users') req.add_header('Authorization', 'Bearer my_token') req.add_header('Content-Type', 'application/json') response = urllib.request.urlopen(req)
You can access the response headers using the getheader()
method of the response object:
print(response.getheader('Content-Type')) # application/json
Manipulating JSON data in Python
JSON (JavaScript Object Notation) is a popular data interchange format that is widely used in web APIs and web services. When working with cURL commands, it is common to encounter JSON data in the response. In Python, there are built-in methods and libraries that make it easy to manipulate JSON data.
Using the requests module
The requests module provides a convenient json()
method that can be used to parse JSON data from the response:
import requests response = requests.get('https://api.example.com/users') data = response.json() print(data['name']) # John Doe
You can also send JSON data in the request body using the json
parameter:
import requests data = {'name': 'John Doe', 'email': 'john.doe@example.com'} response = requests.post('https://api.example.com/users', json=data)
Related Article: How To Create Pandas Dataframe From Variables - Valueerror
Using the urllib module
To manipulate JSON data using the urllib module, you need to use the json
module from the Python standard library. The json
module provides methods for parsing and serializing JSON data:
import urllib.request import json response = urllib.request.urlopen('https://api.example.com/users') data = json.load(response) print(data['name']) # John Doe
Similarly, you can send JSON data in the request body using the json
module:
import urllib.request import json data = {'name': 'John Doe', 'email': 'john.doe@example.com'} req = urllib.request.Request('https://api.example.com/users', data=json.dumps(data).encode('utf-8')) req.add_header('Content-Type', 'application/json') response = urllib.request.urlopen(req)
Converting cURL Commands to Python
Now that we have covered the basics of using the requests module and the urllib module in Python, let's see how we can convert cURL commands to Python code.
The first step in converting a cURL command to Python is to identify the HTTP method (GET, POST, PUT, DELETE) and the URL. Once you have that information, you can choose the appropriate method from the requests module or the urllib module.
Next, you need to consider any additional options or flags in the cURL command. These options can include headers, data parameters, and authentication credentials. You can add headers using the headers
parameter in the requests module or by creating a Request
object in the urllib module and adding headers using the add_header()
method.
If the cURL command includes data parameters, you can pass them as a dictionary or a JSON object, depending on the requirements of the API. The requests module allows you to pass data parameters directly using the data
or json
parameter, while the urllib module requires you to encode the data as bytes and set the Content-Type header to application/json.
Common uses of the requests module in Python
The requests module is widely used in Python for making HTTP requests due to its simplicity and ease of use. Here are some common use cases for the requests module:
- Consuming web APIs: The requests module allows developers to easily interact with web APIs and retrieve data from external services.
- Testing web applications: With the requests module, developers can simulate user actions and test the functionality of web applications.
- Web scraping: The requests module is often used in combination with other libraries like BeautifulSoup to scrape data from websites.
- File uploads: The requests module provides convenient methods for uploading files to web servers.
- Authentication: The requests module supports various authentication schemes, making it easy to authenticate with web services.
Sending HTTP headers in a Python request
HTTP headers provide additional information about the request or response. In Python, you can send HTTP headers in a request using the requests module or the urllib module.
To send headers using the requests module, you can pass a dictionary of headers to the request functions:
import requests headers = {'Authorization': 'Bearer my_token', 'Content-Type': 'application/json'} response = requests.get('https://api.example.com/users', headers=headers)
To send headers using the urllib module, you can create a Request
object and add headers using the add_header()
method:
import urllib.request req = urllib.request.Request('https://api.example.com/users') req.add_header('Authorization', 'Bearer my_token') req.add_header('Content-Type', 'application/json') response = urllib.request.urlopen(req)
In both cases, you can access the response headers using the appropriate attribute or method of the response object.
Related Article: How To Handle Ambiguous Truth Value In Python Series
Additional Resources
- Common uses of the requests module in Python