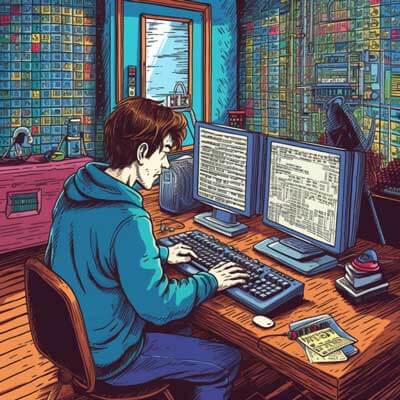
Table of Contents
In ReactJS, the render
method is responsible for rendering the component's output to the screen. It is called automatically by React when a component needs to be rendered or updated. Inside the render
method, you can define and use functions to enhance the rendering logic and make the code more readable and modular.
Here's an example of crafting a function within the render
method:
class Greeting extends React.Component { render() { const formatName = (name) => { return name.toUpperCase(); }; return <h1>Hello, {formatName(this.props.name)}!</h1>; } }
In this example, the Greeting
component defines a function called formatName
within the render
method. This function takes a name
parameter and returns the uppercase version of the name. The formatName
function is then used within the JSX expression to format the name prop.
Crafting functions within the render
method can be useful for encapsulating logic, reusing code, and improving code readability. However, it's important to keep in mind that defining functions within the render
method will create new function instances on each render, which may impact performance. If a function doesn't rely on any component state or props, it's generally recommended to define it outside the render
method.
What is a component in ReactJS?
In ReactJS, a component is a reusable piece of code that defines the structure and behavior of a specific part of a user interface. Components can be classified into two types: functional components and class components.
Functional components are JavaScript functions that return JSX (JavaScript XML) elements. They are simpler to write and understand, and they are often used for presentational purposes. Here's an example of a functional component:
function Welcome(props) { return <h1>Hello, {props.name}!</h1>; }
Class components, on the other hand, are ES6 classes that extend the React.Component class. They have a more complex syntax but offer additional features, such as lifecycle methods and the ability to maintain state. Here's an example of a class component:
class Welcome extends React.Component { render() { return <h1>Hello, {this.props.name}!</h1>; } }
Related Article: How to Set Up Your First ReactJS Project
What is the concept of state in ReactJS?
State is a fundamental concept in ReactJS that allows components to manage their internal data. It represents the current state of a component and determines how it should render and behave. State can be updated using the setState()
method, and when the state changes, React automatically re-renders the component to reflect the new state.
To use state in a class component, you need to initialize it in the constructor and access it using the this.state
object. Here's an example:
class Counter extends React.Component { constructor(props) { super(props); this.state = { count: 0 }; } render() { return ( <div> <p>Count: {this.state.count}</p> <button onClick={() => this.setState({ count: this.state.count + 1 })}> Increment </button> </div> ); } }
In the above example, the Counter
component has an initial state of count: 0
. When the button is clicked, the setState()
method is called to update the state and trigger a re-render.
What are props in ReactJS?
Props (short for properties) are a way to pass data from a parent component to its child components. They are read-only and cannot be modified by the child components. Props allow components to be configurable and reusable, as different instances of the same component can receive different props.
In functional components, props are passed as parameters to the function. Here's an example:
function Greeting(props) { return <h1>Hello, {props.name}!</h1>; }
In class components, props are accessed using the this.props
object. Here's an example:
class Greeting extends React.Component { render() { return <h1>Hello, {this.props.name}!</h1>; } }
To use the above components, you can pass props like this:
<Greeting name="John" />
In this example, the name
prop is set to "John", and the component will render "Hello, John!".
What is JSX in ReactJS?
JSX (JavaScript XML) is an extension to JavaScript that allows you to write HTML-like code within JavaScript. It is a syntax extension provided by ReactJS, and it is used to define the structure and content of React components.
JSX makes it easier to create and manipulate DOM elements in a declarative way. It allows you to embed JavaScript expressions within curly braces {}
to dynamically generate content. Here's an example:
function Welcome(props) { return <h1>Hello, {props.name}!</h1>; }
In this example, the JSX expression <h1>Hello, {props.name}!</h1>
is used to define the structure of the Welcome
component. The {props.name}
expression is a JavaScript expression that evaluates to the value of the name
prop.
JSX is not required to use ReactJS, but it is a recommended and widely adopted practice due to its readability and ease of use.
Related Article: Handling State Persistence in ReactJS After Refresh
What is the render method in ReactJS?
The render()
method is a mandatory method in ReactJS components. It defines what should be rendered to the screen when the component is mounted or updated.
In functional components, the render()
method is replaced by the function itself. Here's an example:
function Greeting(props) { return <h1>Hello, {props.name}!</h1>; }
In class components, the render()
method must be defined explicitly. It should return a JSX element or null. Here's an example:
class Greeting extends React.Component { render() { return <h1>Hello, {this.props.name}!</h1>; } }
In both examples, the render()
method defines the structure of the component's output. It can contain JSX elements, JavaScript expressions, and even other React components.
The render()
method is automatically called by React when a component needs to be rendered or updated. It is responsible for generating the virtual DOM representation of the component, which is then used to update the actual DOM.
What is a functional component in ReactJS?
A functional component in ReactJS is a JavaScript function that returns JSX elements. It is the simplest form of a React component and is primarily used for presentational purposes.
Functional components are stateless, meaning they do not have their own internal state. They receive data via props and render it to the screen. They are also more lightweight and performant compared to class components.
Here's an example of a functional component:
function Greeting(props) { return <h1>Hello, {props.name}!</h1>; }
In this example, the Greeting
component receives a name
prop and renders it within an <h1>
element.
Functional components can also receive multiple props and render complex JSX structures. They are ideal for components that don't require internal state or complex lifecycle methods.
What is a class component in ReactJS?
A class component in ReactJS is an ES6 class that extends the React.Component
class. It provides additional features compared to functional components, such as the ability to maintain internal state and use lifecycle methods.
Class components have a more complex syntax but offer more flexibility and control. They are used when a component needs to manage its own state, handle user interactions, or perform complex logic.
Here's an example of a class component:
class Counter extends React.Component { constructor(props) { super(props); this.state = { count: 0 }; } render() { return ( <div> <p>Count: {this.state.count}</p> <button onClick={() => this.setState({ count: this.state.count + 1 })}> Increment </button> </div> ); } }
In this example, the Counter
component maintains an internal state count
and renders it within a <p>
element. The onClick
event handler updates the state when the button is clicked.
Class components can have additional lifecycle methods, such as componentDidMount()
and componentDidUpdate()
, which allow for more fine-grained control over the component's behavior.
How to use the useState hook in ReactJS?
The useState
hook is a built-in hook in ReactJS that allows functional components to manage state. It provides an alternative to using class components and the setState()
method.
To use the useState
hook, you need to import it from the react
module:
import React, { useState } from 'react';
Once imported, you can use the useState
hook inside a functional component. It takes an initial state value as its argument and returns an array with two elements: the current state value and a function to update the state.
Here's an example of using the useState
hook:
import React, { useState } from 'react'; function Counter() { const [count, setCount] = useState(0); return ( <div> <p>Count: {count}</p> <button onClick={() => setCount(count + 1)}>Increment</button> </div> ); }
In this example, the Counter
component uses the useState
hook to initialize the state variable count
to 0. The setCount
function returned by the useState
hook is used to update the state when the button is clicked.
The useState
hook can be used multiple times within a single component to manage different state variables.
Related Article: How to Add Navbar Components for Different Pages in ReactJS
How to use the useEffect hook in ReactJS?
The useEffect
hook is a built-in hook in ReactJS that allows functional components to perform side effects, such as fetching data, subscribing to events, or manipulating the DOM. It replaces lifecycle methods like componentDidMount
and componentDidUpdate
in class components.
To use the useEffect
hook, you need to import it from the react
module:
import React, { useEffect } from 'react';
Once imported, you can use the useEffect
hook inside a functional component. It takes a function as its first argument, which will be executed after the component has rendered. The function can also return a cleanup function to handle any necessary cleanup before the component is unmounted.
Here's an example of using the useEffect
hook:
import React, { useState, useEffect } from 'react'; function Timer() { const [time, setTime] = useState(0); useEffect(() => { const interval = setInterval(() => { setTime((prevTime) => prevTime + 1); }, 1000); return () => { clearInterval(interval); }; }, []); return <p>Time: {time}</p>; }
In this example, the Timer
component uses the useEffect
hook to start a timer that increments the time
state variable every second. The useEffect
hook is called after the component has rendered for the first time, thanks to the empty dependency array []
. It returns a cleanup function that clears the interval when the component is unmounted.
The useEffect
hook can also take an optional second argument, an array of dependencies. If any of the dependencies change between renders, the effect function will be re-executed. This allows you to control when the effect should be triggered.
Additional Resources
- ReactJS - Wikipedia