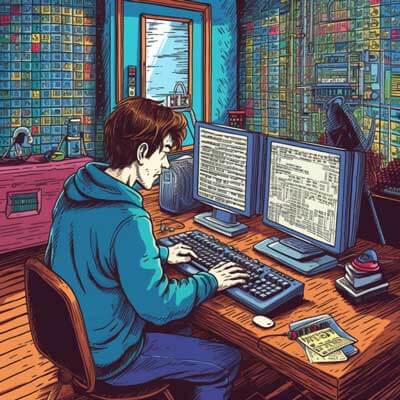
Table of Contents
In React, event handling works similarly to how it works in traditional JavaScript. You can attach event handlers to elements in your React components and define functions that will be executed when the events occur. React uses a synthetic event system, which means that the events are normalized across different browsers and have consistent behavior.
What is the onClick function in React?
The onClick function is a built-in event handler in React that is used to handle the click event on an element. It is commonly used to add interactivity to your components and allow users to interact with your application. When a click event occurs on an element, the onClick function is called, and you can define the logic that should be executed in response to the click event.
Related Article: Adding a Newline in the JSON Property Render with ReactJS
How do you write an onClick function in React?
To write an onClick function in React, you need to define a function that will be called when the click event occurs. This function can be defined as a regular JavaScript function or as an arrow function. You can then attach the onClick event handler to an element by using the onClick prop and passing the function as its value.
Here is an example of how to write an onClick function in a functional component:
import React from 'react'; function MyButton() { const handleClick = () => { console.log('Button Clicked!'); }; return <button onClick={handleClick}>Click Me</button>; }
And here is an example of how to write an onClick function in a class component:
import React from 'react'; class MyButton extends React.Component { handleClick() { console.log('Button Clicked!'); } render() { return <button onClick={this.handleClick}>Click Me</button>; } }
What is a React component?
A React component is a reusable piece of code that represents a part of the user interface. Components can be simple, representing a single element, or complex, representing a combination of elements and other components. React components can be classified into two types: functional components and class components.
How do you handle state in React?
In React, state is an object that is used to store and manage data that can change over time. State is typically used to represent the data that is specific to a component and needs to be updated and displayed dynamically. React provides the useState hook for functional components and the this.state property for class components to handle state.
Here is an example of how to handle state in a functional component using the useState hook:
import React, { useState } from 'react'; function Counter() { const [count, setCount] = useState(0); const increment = () => { setCount(count + 1); }; return ( <div> <p>Count: {count}</p> <button onClick={increment}>Increment</button> </div> ); }
And here is an example of how to handle state in a class component using this.state:
import React from 'react'; class Counter extends React.Component { constructor(props) { super(props); this.state = { count: 0 }; } increment() { this.setState({ count: this.state.count + 1 }); } render() { return ( <div> <p>Count: {this.state.count}</p> <button onClick={this.increment.bind(this)}>Increment</button> </div> ); } }
Related Article: Handling State Persistence in ReactJS After Refresh
What are props in React?
Props, short for properties, are a way to pass data from a parent component to a child component in React. Props are used to make components reusable and to customize their behavior and appearance. Props are passed to a component as attributes and can be accessed within the component using the props object.
Here is an example of how to pass props to a child component:
import React from 'react'; function Greeting(props) { return <h1>Hello, {props.name}!</h1>; } function App() { return <Greeting name="John" />; }
In this example, the Greeting component receives the name prop and displays a personalized greeting. The App component passes the name prop with the value "John" to the Greeting component.
What is JSX in React?
JSX is a syntax extension for JavaScript that allows you to write HTML-like code within your JavaScript code. It is a convenient way to define the structure and appearance of your React components. JSX is not valid JavaScript, but it can be transformed into JavaScript using a transpiler like Babel.
Here is an example of JSX code:
import React from 'react'; function Greeting() { return <h1>Hello, World!</h1>; }
In this example, the Greeting component returns JSX code that represents an HTML heading element with the text "Hello, World!".
What is a functional component in React?
A functional component in React is a component that is defined as a JavaScript function. Functional components are the simplest form of components in React and are used for rendering UI based on props and state. Functional components can be written as regular JavaScript functions or as arrow functions.
Here is an example of a functional component:
import React from 'react'; function Greeting(props) { return <h1>Hello, {props.name}!</h1>; }
In this example, the Greeting component is a functional component that receives the name prop and displays a personalized greeting.
What is a class component in React?
A class component in React is a component that is defined as a JavaScript class. Class components are used when you need to manage state and handle lifecycle methods in your component. Class components extend the React.Component class and have a render method that returns the component's UI.
Here is an example of a class component:
import React from 'react'; class Greeting extends React.Component { render() { return <h1>Hello, {this.props.name}!</h1>; } }
In this example, the Greeting component is a class component that receives the name prop and displays a personalized greeting.
Related Article: Sharing Variables Between Components in ReactJS
Code Snippet: Implementing an onClick function in a functional component
import React from 'react'; function MyButton() { const handleClick = () => { console.log('Button Clicked!'); }; return <button onClick={handleClick}>Click Me</button>; }
In this code snippet, we have a functional component called MyButton that renders a button element. The handleClick function is defined within the component and is called when the button is clicked. It logs a message to the console when the button is clicked.
Code Snippet: Implementing an onClick function in a class component
import React from 'react'; class MyButton extends React.Component { handleClick() { console.log('Button Clicked!'); } render() { return <button onClick={this.handleClick}>Click Me</button>; } }
In this code snippet, we have a class component called MyButton that extends the React.Component class. The handleClick method is defined within the component and is called when the button is clicked. It logs a message to the console when the button is clicked.
Code Snippet: Passing props to a child component
import React from 'react'; function Greeting(props) { return <h1>Hello, {props.name}!</h1>; } function App() { return <Greeting name="John" />; }
In this code snippet, we have a functional component called Greeting that receives the name prop and displays a personalized greeting. The App component renders the Greeting component and passes the name prop with the value "John".
Code Snippet: Using JSX syntax in React
import React from 'react'; function Greeting() { return <h1>Hello, World!</h1>; }
In this code snippet, we have a functional component called Greeting that returns JSX code. The JSX code represents an HTML heading element with the text "Hello, World!".
Related Article: How to Solve “_ is not defined” Errors in ReactJS
Advanced: Handling complex state in React
When dealing with complex state in React, it is common to use the useState hook or the this.state property to manage the state of individual variables or objects. However, as the complexity of the state increases, it may become difficult to manage and update the state in an efficient manner. In such cases, you can consider using the useReducer hook or implementing state management libraries like Redux.
Advanced: Optimizing performance in React components
To optimize performance in React components, you can follow best practices like using memoization, implementing shouldComponentUpdate or PureComponent for class components, and using React.memo for functional components. These techniques can help reduce unnecessary re-renders and improve the overall performance of your React application.
Troubleshooting: Common issues with onClick functions in React
Some common issues that you may encounter with onClick functions in React include not binding the event handler correctly, losing the context of this within a class component, and encountering issues with event propagation. To troubleshoot these issues, you can use techniques like binding the event handler in the constructor, using arrow functions, or using event.stopPropagation() to prevent event bubbling.
Troubleshooting: Debugging React components
When debugging React components, you can use tools like the React Developer Tools extension for your browser, the console.log statement to log values, and the debugger statement to set breakpoints and step through your code. These tools can help you inspect the component's state and props, track down issues, and understand the flow of your code.