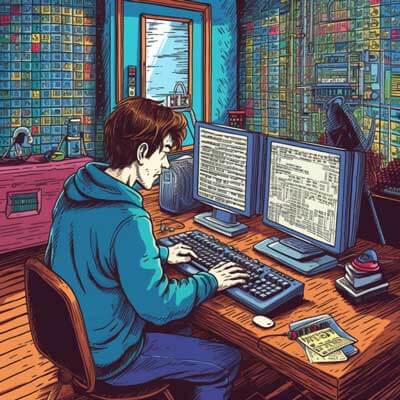
Table of Contents
Overview of Custom Datasets and Dataloaders
In the world of machine learning, having access to high-quality datasets is crucial for model training and evaluation. While PyTorch provides built-in datasets for common tasks, such as image classification and natural language processing, there may be cases where you need to create custom datasets to fit your specific needs.
Custom datasets allow you to work with your own data, whether it's images, text, audio, or any other type of data. By creating custom datasets, you can preprocess and transform your data in a way that is most suitable for your machine learning task.
Dataloaders, on the other hand, are responsible for loading data from datasets and feeding it to your model in batches. Dataloaders provide functionalities like shuffling, batching, and parallel data loading, making it easier to train your models efficiently.
In this article, we will explore how to create custom datasets and implement custom dataloaders in PyTorch. We will also discuss data augmentation techniques and the benefits of using custom dataloaders.
Related Article: GPU Acceleration Implementation with PyTorch
Creating Custom Datasets
Creating a custom dataset in PyTorch involves creating a class that inherits from the torch.utils.data.Dataset
class and implementing two key methods: __len__
and __getitem__
.
The __len__
method should return the size of the dataset, while the __getitem__
method should return the data item at a given index. This allows PyTorch to access and retrieve individual data items from your dataset.
Let's say we want to create a custom dataset for image classification. Here's an example of how you can create a custom dataset class:
import torch from torch.utils.data import Dataset class CustomImageDataset(Dataset): def __init__(self, file_paths, labels, transform=None): self.file_paths = file_paths self.labels = labels self.transform = transform def __len__(self): return len(self.file_paths) def __getitem__(self, index): image = Image.open(self.file_paths[index]) label = self.labels[index] if self.transform: image = self.transform(image) return image, label
In this example, we initialize the class with file paths and corresponding labels. The __len__
method returns the total number of data items in the dataset, and the __getitem__
method loads the image from the file path, applies any transformations specified by the transform
parameter, and returns the image and label.
Implementing Custom Dataloaders
Once you have created a custom dataset, you can use it with a custom dataloader to efficiently load and process your data. The torch.utils.data.DataLoader
class provides functionalities like shuffling, batching, and parallel data loading.
Here's an example of how you can implement a custom dataloader for our custom image dataset:
from torch.utils.data import DataLoader # Create an instance of the custom dataset dataset = CustomImageDataset(file_paths, labels, transform) # Create a dataloader with batch size and other options dataloader = DataLoader(dataset, batch_size=32, shuffle=True, num_workers=4)
In this example, we create an instance of the custom image dataset with the file paths, labels, and any desired transformations. We then create a dataloader, specifying the batch size, shuffle option, and the number of workers for parallel data loading.
The dataloader can be used in a training loop to iterate over batches of data, like this:
for batch in dataloader: images, labels = batch # Perform training or evaluation on the batch of data
Data Augmentation Techniques
Data augmentation is a technique used to artificially increase the size and diversity of your dataset by applying random transformations to your data. This can help improve the generalization and robustness of your models.
PyTorch provides a variety of data augmentation techniques through the torchvision.transforms
module. Some commonly used data augmentation techniques include:
- Random horizontal flipping
- Random vertical flipping
- Random rotation
- Random cropping
- Color jittering
Here's an example of how you can apply data augmentation to your custom dataset:
from torchvision import transforms # Define a set of transformation functions transform = transforms.Compose([ transforms.RandomHorizontalFlip(), transforms.RandomVerticalFlip(), transforms.RandomRotation(30), transforms.RandomCrop(224), transforms.ColorJitter(brightness=0.2, contrast=0.2, saturation=0.2, hue=0.2) ]) # Create an instance of the custom dataset with data augmentation dataset = CustomImageDataset(file_paths, labels, transform)
In this example, we define a set of transformation functions using the torchvision.transforms
module. We then create an instance of the custom image dataset, passing in the file paths, labels, and the transformation object.
When the __getitem__
method is called, the transformation functions will be applied to the image before returning it. This allows you to easily incorporate data augmentation into your custom datasets.
Related Article: Data Loading and Preprocessing in PyTorch
Image Loading in PyTorch
When working with image datasets, PyTorch provides several ways to load and preprocess images. The most common method is to use the PIL
library to load images, which can then be converted to tensors using the torchvision.transforms.ToTensor
transformation.
Here's an example of how you can load and preprocess images in PyTorch:
from PIL import Image from torchvision.transforms import ToTensor # Load an image using PIL image = Image.open('image.jpg') # Convert the image to a tensor tensor_image = ToTensor()(image)
In this example, we use the Image.open
function from the PIL
library to load an image file. We then apply the ToTensor
transformation from the torchvision.transforms
module to convert the image to a tensor.
Once the image is converted to a tensor, it can be easily processed and fed into your models for training or evaluation.
Benefits of Custom Dataloaders
Using custom dataloaders in PyTorch offers several benefits:
1. Flexibility: Custom dataloaders allow you to preprocess and transform your data in a way that is most suitable for your specific machine learning task. You have full control over how your data is loaded, shuffled, and batched.
2. Efficiency: Dataloaders provide functionalities like parallel data loading, which can significantly speed up the data loading process, especially for large datasets. They also allow you to load and process data in batches, reducing memory usage and improving overall efficiency.
3. Integration: Custom dataloaders seamlessly integrate with the rest of the PyTorch ecosystem, including models, loss functions, and optimizers. This makes it easier to build end-to-end machine learning pipelines and experiment with different combinations of datasets and models.
4. Reproducibility: By creating custom dataloaders, you can ensure that your data preprocessing and transformation steps are consistent across different experiments and runs. This improves reproducibility and makes it easier to compare and analyze your results.
Data Augmentation with Custom Datasets
Data augmentation can be particularly useful when working with custom datasets. By applying random transformations to your data, you can increase its diversity and make your models more robust to variations and noise in the real-world data.
When creating custom datasets, you can incorporate data augmentation techniques directly into the __getitem__
method. This allows you to apply different transformations to each data item, effectively creating a larger and more varied dataset.
Here's an example of how you can apply data augmentation to a custom dataset:
import random class CustomImageDataset(Dataset): def __init__(self, file_paths, labels): self.file_paths = file_paths self.labels = labels def __len__(self): return len(self.file_paths) def __getitem__(self, index): image = Image.open(self.file_paths[index]) label = self.labels[index] # Apply random data augmentation if random.random() < 0.5: image = transforms.RandomHorizontalFlip()(image) if random.random() < 0.5: image = transforms.RandomRotation(30)(image) # Apply other transformations if needed image = transforms.ToTensor()(image) return image, label
In this example, we add random horizontal flipping and random rotation as data augmentation techniques. The probability of applying each transformation is controlled by the random.random()
function.
Additional Resources
- Data Augmentation with Custom Datasets in PyTorch