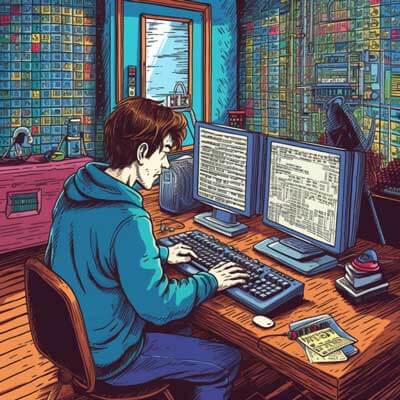
Table of Contents
Fetching Data in ReactJS
Fetching data is a common task in web development, and ReactJS provides several ways to accomplish this. One popular method is using the built-in fetch
function, which allows you to make HTTP requests and retrieve data from a server.
Here's an example of how you can fetch data in ReactJS using the fetch
function:
fetch('https://api.example.com/data') .then(response => response.json()) .then(data => { // Handle the retrieved data console.log(data); }) .catch(error => { // Handle any errors console.error(error); });
In the example above, we make a GET request to the https://api.example.com/data
endpoint. We use the then
method to handle the response and convert it to JSON format. Finally, we log the retrieved data to the console. If there are any errors during the request, we catch them and log them to the console as well.
You can customize the request by passing additional parameters to the fetch
function, such as headers or request methods. For example, you can make a POST request by specifying the method
parameter as 'POST'
and including the data in the request body.
Related Article: How to Integrate UseHistory from the React Router DOM
Using Axios in ReactJS
While the fetch
function is built-in to modern browsers, some developers prefer to use a third-party library like Axios for data fetching in ReactJS. Axios provides additional features and a more user-friendly API compared to fetch
.
To use Axios in your ReactJS project, you first need to install it using a package manager like npm or yarn:
npm install axios
Once installed, you can import Axios and use it to make HTTP requests. Here's an example:
import axios from 'axios'; axios.get('https://api.example.com/data') .then(response => { // Handle the retrieved data console.log(response.data); }) .catch(error => { // Handle any errors console.error(error); });
In this example, we import Axios and use the get
method to make a GET request to the https://api.example.com/data
endpoint. The response object contains the retrieved data, which we log to the console. Any errors during the request are caught and logged as well.
Axios also supports other HTTP methods like POST, PUT, and DELETE, which you can use to send data to the server or perform other operations.
Sending Requests to Server
ReactJS provides various ways to send requests to a server. As mentioned earlier, you can use the fetch
function or a library like Axios. Additionally, ReactJS also supports the use of AJAX for sending requests.
AJAX (Asynchronous JavaScript and XML) allows you to update parts of a web page without reloading the entire page. It is commonly used for sending and retrieving data from a server asynchronously.
To send a request using AJAX in ReactJS, you can use the XMLHttpRequest
object. Here's an example:
const xhr = new XMLHttpRequest(); xhr.open('GET', 'https://api.example.com/data'); xhr.onreadystatechange = function() { if (xhr.readyState === XMLHttpRequest.DONE) { // Request completed if (xhr.status === 200) { // Request successful console.log(xhr.responseText); } else { // Request failed console.error(xhr.statusText); } } }; xhr.send();
In this example, we create a new XMLHttpRequest
object and open a GET request to the https://api.example.com/data
endpoint. We set the onreadystatechange
event handler to handle the response. Once the request is completed (readyState
is XMLHttpRequest.DONE
), we check the status of the response. If the status is 200, the request was successful and we log the response text to the console. Otherwise, we log the status text as an error.
While AJAX is a useful and flexible way to send requests in ReactJS, it requires more manual handling compared to the fetch
function or Axios. You need to handle different states of the request and parse the response manually.
Posting Data to Server in ReactJS
Posting data to a server is a common task in web development, especially when dealing with forms or user input. ReactJS provides multiple options for posting data, including using the fetch
function, Axios, or AJAX.
Here's an example of how you can post data to a server using the fetch
function:
fetch('https://api.example.com/data', { method: 'POST', headers: { 'Content-Type': 'application/json' }, body: JSON.stringify({ name: 'John Doe', age: 30 }) }) .then(response => response.json()) .then(data => { // Handle the response data console.log(data); }) .catch(error => { // Handle any errors console.error(error); });
In this example, we use the fetch
function with the POST
method to send data to the https://api.example.com/data
endpoint. We include the data in the request body as a JSON string using the JSON.stringify
method. We also set the Content-Type
header to specify that the request body is in JSON format.
After sending the request, we handle the response by parsing it as JSON and logging the response data to the console. Any errors during the request are caught and logged as well.
Similarly, you can use Axios or AJAX to post data to a server. Here's an example using Axios:
import axios from 'axios'; axios.post('https://api.example.com/data', { name: 'John Doe', age: 30 }) .then(response => { // Handle the response data console.log(response.data); }) .catch(error => { // Handle any errors console.error(error); });
In this example, we use the post
method of the Axios library to send a POST request to the https://api.example.com/data
endpoint. We include the data as an object in the second parameter of the post
method. The response data is logged to the console, and any errors are caught and logged.
Related Article: How to Use the Render Method in ReactJS
Working with AJAX in ReactJS
AJAX (Asynchronous JavaScript and XML) allows you to send and retrieve data from a server asynchronously. It is a useful technique for updating parts of a web page without reloading the entire page. ReactJS provides support for working with AJAX through the use of the XMLHttpRequest
object or libraries like Axios.
Here's an example of how you can use AJAX in ReactJS to fetch data from a server:
const xhr = new XMLHttpRequest(); xhr.open('GET', 'https://api.example.com/data'); xhr.onreadystatechange = function() { if (xhr.readyState === XMLHttpRequest.DONE) { // Request completed if (xhr.status === 200) { // Request successful console.log(xhr.responseText); } else { // Request failed console.error(xhr.statusText); } } }; xhr.send();
In this example, we create a new XMLHttpRequest
object and open a GET request to the https://api.example.com/data
endpoint. We set the onreadystatechange
event handler to handle the response. Once the request is completed (readyState
is XMLHttpRequest.DONE
), we check the status of the response. If the status is 200, the request was successful and we log the response text to the console. Otherwise, we log the status text as an error.
AJAX can also be used to send data to a server. Here's an example of how you can use AJAX to post data:
const xhr = new XMLHttpRequest(); xhr.open('POST', 'https://api.example.com/data'); xhr.setRequestHeader('Content-Type', 'application/json'); xhr.onreadystatechange = function() { if (xhr.readyState === XMLHttpRequest.DONE) { // Request completed if (xhr.status === 201) { // Request successful console.log('Data posted successfully'); } else { // Request failed console.error(xhr.statusText); } } }; xhr.send(JSON.stringify({ name: 'John Doe', age: 30 }));
In this example, we create a new XMLHttpRequest
object and open a POST request to the https://api.example.com/data
endpoint. We set the Content-Type
header to specify that the request body is in JSON format. We also set the onreadystatechange
event handler to handle the response. Once the request is completed, we check the status of the response. If the status is 201, the request was successful and we log a success message to the console. Otherwise, we log the status text as an error.
While working with AJAX in ReactJS provides more control and flexibility, it requires more manual handling compared to using libraries like Axios. You need to handle different states of the request, set headers, parse the response, and handle errors manually.
Handling API Calls in ReactJS
API calls allow your application to interact with external services or retrieve data from a server. ReactJS provides various methods and techniques for handling API calls effectively.
One common approach is to use the fetch
function or a library like Axios to make API calls. These methods allow you to send HTTP requests, retrieve data, and handle errors in a structured and convenient way.
Here's an example of how you can handle API calls using the fetch
function in ReactJS:
fetch('https://api.example.com/data') .then(response => { if (response.ok) { return response.json(); } else { throw new Error('Request failed'); } }) .then(data => { // Handle the retrieved data console.log(data); }) .catch(error => { // Handle any errors console.error(error); });
In this example, we use the fetch
function to make a GET request to the https://api.example.com/data
endpoint. We check if the response is successful (response.ok
) and return the parsed JSON data. If the response is not successful, we throw an error.
After handling the response, we can further process the retrieved data or update the state of our React components. Any errors during the API call are caught and logged to the console.
It is also important to handle loading and error states while making API calls. You can use React's state and lifecycle methods to manage the state of your components during API calls. For example, you can display a loading spinner while the API call is in progress and show an error message if the API call fails.
Async/Await in ReactJS
Async/await is a modern JavaScript feature that allows you to write asynchronous code in a synchronous manner. It provides a more readable and structured way to handle promises and make asynchronous API calls.
ReactJS is compatible with async/await, and you can use it to handle asynchronous tasks, including data transmission to a server.
Here's an example of how you can use async/await to fetch data in ReactJS:
async function fetchData() { try { const response = await fetch('https://api.example.com/data'); const data = await response.json(); // Handle the retrieved data console.log(data); } catch (error) { // Handle any errors console.error(error); } } fetchData();
In this example, we define an async
function called fetchData
that fetches data from the https://api.example.com/data
endpoint. We use the await
keyword to wait for the fetch
function to complete and the response to be returned. We then parse the response as JSON and log the retrieved data to the console. Any errors during the API call are caught and logged.
Using async/await in ReactJS can make your code more readable and reduce the complexity of handling promises and callbacks. It allows you to write asynchronous code in a way that looks similar to synchronous code, making it easier to understand and maintain.
Sending Form Data to a Server in ReactJS
ReactJS provides several ways to send form data, including using the fetch
function, libraries like Axios, or AJAX.
Here's an example of how you can send form data to a server using the fetch
function in ReactJS:
const formData = new FormData(); formData.append('name', 'John Doe'); formData.append('age', 30); fetch('https://api.example.com/data', { method: 'POST', body: formData }) .then(response => response.json()) .then(data => { // Handle the response data console.log(data); }) .catch(error => { // Handle any errors console.error(error); });
In this example, we create a new FormData
object and append the form fields and their values. We then use the fetch
function with the POST
method to send the form data to the https://api.example.com/data
endpoint. We include the FormData
object as the request body.
After sending the request, we handle the response by parsing it as JSON and logging the response data to the console. Any errors during the request are caught and logged as well.
You can also send form data using libraries like Axios or AJAX. Here's an example using Axios:
import axios from 'axios'; const formData = new FormData(); formData.append('name', 'John Doe'); formData.append('age', 30); axios.post('https://api.example.com/data', formData) .then(response => { // Handle the response data console.log(response.data); }) .catch(error => { // Handle any errors console.error(error); });
In this example, we use the post
method of the Axios library to send a POST request to the https://api.example.com/data
endpoint. We include the FormData
object as the second parameter of the post
method. The response data is logged to the console, and any errors are caught and logged.
Sending form data is a crucial part of web development, and ReactJS provides flexible options to handle this task efficiently.
Related Article: How to Style Components in ReactJS
Best Practices for Data Transmission in ReactJS
When it comes to data transmission in ReactJS, there are several best practices to follow to ensure efficient and secure communication with the server. Here are some key recommendations:
1. Use HTTPS
Always use HTTPS for data transmission to ensure secure communication between the client and the server. HTTPS encrypts the data and protects it from unauthorized access.
2. Validate User Input
Before sending data to the server, validate user input to prevent any potential security vulnerabilities or data integrity issues. Use client-side validation techniques and server-side validation to ensure that only valid and safe data is transmitted.
3. Sanitize and Escape User Input
When processing user input, make sure to sanitize and escape the data to prevent any potential security risks, such as cross-site scripting (XSS) attacks. Use libraries or built-in functions to handle input sanitization and escaping.
4. Use Proper Authentication and Authorization
Implement proper authentication and authorization mechanisms to ensure that only authorized users can access and transmit data to the server. Use secure token-based authentication or other authentication methods recommended for your application.
5. Implement Rate Limiting and Throttling
To prevent abuse or denial-of-service attacks, implement rate limiting and throttling mechanisms to control the number of requests that can be made within a certain time period. This helps protect your server from excessive traffic and ensures fair resource allocation.
6. Handle Errors and Provide Feedback
When sending data to the server, handle any errors that may occur and provide appropriate feedback to the user. Display error messages or notifications to inform the user about the status of their data transmission and guide them on how to resolve any issues.
7. Use Optimized Requests
Optimize your data transmission by minimizing the size of the requests and responses. Use compression techniques, such as gzip or brotli, to reduce the payload size. Avoid sending unnecessary data and only transmit the required information.
8. Implement Caching
Implement caching mechanisms to reduce the load on the server and improve the performance of data transmission. Use appropriate caching headers and techniques to store and retrieve data from the client's cache whenever possible.
9. Monitor and Analyze Performance
Regularly monitor and analyze the performance of your data transmission to identify any bottlenecks or areas for improvement. Use tools like browser developer tools, network analyzers, or performance monitoring services to gain insights into the performance of your application.
10. Stay Up-to-Date with Security Practices
Keep yourself updated with the latest security practices and vulnerabilities related to data transmission. Follow security blogs, subscribe to security mailing lists, and participate in security communities to stay informed about potential risks and best practices.
Fetch API vs. Axios for Data Transmission in ReactJS
When it comes to data transmission in ReactJS, you have the option to use the built-in fetch
function or a third-party library like Axios. Both options have their advantages and can be used effectively depending on your requirements.
Fetch API
The Fetch API is a built-in JavaScript function that allows you to make HTTP requests and retrieve data from a server. It is supported by modern browsers and provides a simple and lightweight way to handle data transmission.
Advantages of using the Fetch API include:
- Built-in support: The Fetch API is built into modern browsers, so you don't need to install any additional libraries or dependencies.
- Simplicity: The Fetch API has a simple and straightforward API that is easy to understand and use.
- Promises-based: The Fetch API uses promises, allowing you to handle asynchronous operations in a more structured and convenient way.
- Wide browser support: The Fetch API is supported by most modern browsers, making it a reliable choice for cross-browser compatibility.
However, the Fetch API has some limitations:
- Lack of older browser support: The Fetch API is not supported in older browsers like Internet Explorer. If you need to support these browsers, you may need to use a polyfill or fallback to other methods.
- Less feature-rich: The Fetch API provides basic functionality for making HTTP requests, but it may lack some advanced features provided by libraries like Axios, such as automatic JSON parsing, request cancellation, or interceptors.
Axios
Axios is a popular third-party library for making HTTP requests in JavaScript. It provides a more feature-rich API compared to the Fetch API and offers additional functionalities for handling data transmission.
Advantages of using Axios include:
- Easy integration: Axios can be easily integrated into your ReactJS project using a package manager like npm or yarn.
- Enhanced features: Axios provides additional features like automatic JSON parsing, request cancellation, request interceptors, and more, which can simplify the development process.
- Cross-browser compatibility: Axios is designed to work in all modern browsers and provides a consistent API across different environments.
- Community support: Axios has a large user community, which means you can find plenty of resources, examples, and support if you encounter any issues.
However, Axios also has some considerations:
- Additional dependency: Using Axios requires you to install and manage an additional library in your project, which may increase the bundle size and add complexity.
- Learning curve: If you are not familiar with Axios, there may be a learning curve to understand its API and features. However, the Axios API is well-documented and easy to understand.
Using Async/Await for Server Communication in ReactJS
Async/await is a modern JavaScript feature that allows you to write asynchronous code in a synchronous manner. It provides a more readable and structured way to handle promises and make asynchronous server communications in ReactJS.
Using async/await in ReactJS can simplify the code and make it easier to understand and maintain, especially when dealing with multiple asynchronous operations or complex data transmissions.
Here's an example of how you can use async/await for server communication in ReactJS:
async function fetchData() { try { const response = await fetch('https://api.example.com/data'); const data = await response.json(); // Handle the retrieved data console.log(data); } catch (error) { // Handle any errors console.error(error); } } fetchData();
In this example, we define an async
function called fetchData
that fetches data from the https://api.example.com/data
endpoint. We use the await
keyword to wait for the fetch
function to complete and the response to be returned. We then parse the response as JSON and log the retrieved data to the console. Any errors during the server communication are caught and logged.
Using async/await can make your server communication code more readable and structured compared to using callbacks or promises directly. It allows you to write asynchronous code in a way that looks similar to synchronous code, making it easier to understand and maintain.
However, keep in mind that async/await is a modern JavaScript feature and may not be supported in older browsers or environments. Make sure to check the compatibility of async/await with your target browsers or use a transpiler like Babel to ensure broader compatibility.
Advantages of Using Axios for Server Communication in ReactJS
Axios is a popular JavaScript library for making HTTP requests in browsers and Node.js. It provides a simple and intuitive API for server communication in ReactJS, with several advantages over other options.
Here are some advantages of using Axios for server communication in ReactJS:
1. Easy integration and setup
Axios can be easily integrated into your ReactJS project using a package manager like npm or yarn. Simply install the Axios package and import it into your project. Axios has a straightforward setup process and minimal configuration requirements, making it easy to get started.
2. Flexibility and functionality
Axios offers a wide range of features and functionalities that simplify server communication in ReactJS. It supports various HTTP methods, including GET, POST, PUT, and DELETE, allowing you to perform different operations on the server. Axios also provides features like request cancellation, automatic JSON parsing, request interceptors, and more, which can enhance the development process and improve code quality.
3. Promises-based API
Axios uses promises, which are a useful feature of JavaScript, to handle asynchronous operations and server communication. Promises provide a more structured and convenient way to handle asynchronous code, allowing you to chain multiple requests, handle errors, and perform other operations easily.
4. Cross-browser compatibility
Axios is designed to work in all modern browsers and provides a consistent API across different environments. It handles browser inconsistencies and ensures cross-browser compatibility, making it a reliable choice for server communication in ReactJS. Axios also supports server-side rendering (SSR) in Node.js, allowing you to use the same codebase for both client-side and server-side rendering.
5. Community support and documentation
Axios has a large and active community of developers who use and contribute to the library. This means you can find plenty of resources, examples, and support if you encounter any issues or have questions. Axios also has comprehensive documentation, including detailed API references and guides, making it easy to learn and use.
6. Interoperability with other libraries
Axios is widely used and well-supported by other libraries and frameworks, making it easy to integrate with other tools in your ReactJS project. For example, Axios can be used alongside Redux or React Query to manage state and caching effectively. It also supports interceptors, which allow you to modify requests or responses before they are sent or received, making it highly customizable and adaptable to your specific needs.
Related Article: How to Solve “_ is not defined” Errors in ReactJS
Libraries for Data Transmission in ReactJS
When it comes to data transmission in ReactJS, there are several libraries available that can simplify the process and provide additional features and functionalities. These libraries offer various options for making HTTP requests, handling responses, and managing data transmission in a more convenient way.
Here are some popular libraries for data transmission in ReactJS:
1. Axios
Axios is a widely-used JavaScript library for making HTTP requests in browsers and Node.js. It provides a simple and intuitive API for data transmission, with features like automatic JSON parsing, request cancellation, request interceptors, and more. Axios is easy to integrate into your ReactJS project and offers cross-browser compatibility and extensive community support.
2. Superagent
Superagent is another popular library for making HTTP requests in JavaScript. It provides a fluent API for data transmission and supports features like request and response interception, promise-based API, and support for different data formats. Superagent is lightweight and versatile, making it a good choice for data transmission in ReactJS.
3. Fetch API
The Fetch API is a built-in JavaScript function that allows you to make HTTP requests and retrieve data from a server. It is supported by modern browsers and provides a simple and lightweight way to handle data transmission. The Fetch API is easy to use and does not require any additional dependencies.
4. jQuery.ajax
jQuery.ajax is a part of the jQuery library and provides a versatile way to make AJAX requests in JavaScript. It supports various HTTP methods, data serialization, and error handling. While jQuery is not specifically designed for ReactJS, you can still use the jQuery.ajax function in your React components if you are already using jQuery in your project.
5. Axios-mock-adapter
Axios-mock-adapter is a library that allows you to mock HTTP requests made with Axios. It provides an easy way to simulate server responses and test your React components without the need for a real server. Axios-mock-adapter is useful for writing unit tests or when developing locally without a backend API.
These are just a few examples of libraries available for data transmission in ReactJS. Depending on your specific requirements and preferences, you can choose the library that best fits your needs. It's important to consider factors like ease of integration, features, community support, and compatibility with other tools in your project.
Sending Form Data without Libraries in ReactJS
While using libraries like Axios or the Fetch API can simplify the process of sending form data in ReactJS, it is also possible to send form data without relying on any external libraries. ReactJS provides built-in features and techniques that allow you to handle form data transmission effectively.
Here's an example of how you can send form data without libraries in ReactJS:
import React, { useState } from 'react'; function MyForm() { const [name, setName] = useState(''); const [age, setAge] = useState(''); const handleSubmit = (event) => { event.preventDefault(); // Create a new FormData object const formData = new FormData(); formData.append('name', name); formData.append('age', age); // Make a POST request to the server fetch('https://api.example.com/data', { method: 'POST', body: formData }) .then(response => response.json()) .then(data => { // Handle the response data console.log(data); }) .catch(error => { // Handle any errors console.error(error); }); }; return ( <form onSubmit={handleSubmit}> <label> Name: <input type="text" value={name} onChange={event => setName(event.target.value)} /> </label> <br /> <label> Age: <input type="number" value={age} onChange={event => setAge(event.target.value)} /> </label> <br /> <button type="submit">Submit</button> </form> ); } export default MyForm;
In this example, we define a functional component called MyForm
that represents a form with two input fields: name and age. We use the useState
hook to manage the form state. When the form is submitted, we create a new FormData
object and append the form fields and their values. We then make a POST request to the server using the fetch
function, sending the form data as the request body.
Note that we use the event.preventDefault()
method to prevent the default form submission behavior, which would cause a page reload. Instead, we handle the form submission manually and make the AJAX request.
Recommended Way to Send Data to Server in ReactJS
When it comes to sending data to a server in ReactJS, there are multiple options available, including using the fetch
function, libraries like Axios, or AJAX. Each option has its advantages and can be used effectively depending on your specific requirements and preferences.
However, the recommended way to send data to a server in ReactJS is to use the Axios library. Axios offers a user-friendly API, additional features, and extensive community support, making it a popular and reliable choice for server communication.
Here are some reasons why using Axios is recommended for sending data to a server in ReactJS:
1. Ease of use and integration
Axios provides a simple and intuitive API that is easy to understand and use. It offers a consistent interface for making HTTP requests and handling responses, regardless of the browser or environment. Axios can be easily integrated into your ReactJS project using a package manager like npm or yarn.
2. Additional features and functionalities
Axios offers a wide range of features and functionalities that simplify server communication in ReactJS. It supports various HTTP methods, automatic JSON parsing, request cancellation, request interceptors, and more. These features can enhance the development process, improve code quality, and provide more control over data transmission.
3. Promises-based API
Axios uses promises, a useful feature of JavaScript, to handle asynchronous operations and server communication. Promises provide a more structured and convenient way to handle asynchronous code, allowing you to chain multiple requests, handle errors, and perform other operations easily. Promises also align well with modern JavaScript features like async/await.
4. Cross-browser compatibility
Axios is designed to work in all modern browsers and provides a consistent API across different environments. It handles browser inconsistencies and ensures cross-browser compatibility, making it a reliable choice for server communication in ReactJS. Axios also supports server-side rendering (SSR) in Node.js, allowing you to use the same codebase for both client-side and server-side rendering.
5. Community support and documentation
Axios has a large and active community of developers who use and contribute to the library. This means you can find plenty of resources, examples, and support if you encounter any issues or have questions. Axios also has comprehensive documentation, including detailed API references and guides, making it easy to learn and use.
While the fetch
function and AJAX are viable alternatives for sending data to a server in ReactJS, using Axios provides a more user-friendly and feature-rich experience. It simplifies the server communication process, improves code readability, and offers additional functionalities that can enhance your ReactJS applications.
Best Practices for Sending Data to Server in ReactJS
When sending data to a server in ReactJS, following best practices ensures efficient, secure, and reliable data transmission. These best practices help you handle various aspects of data transmission, including validation, security, performance, and error handling.
Here are some recommended best practices for sending data to a server in ReactJS:
1. Validate user input
Before sending data to the server, validate user input to prevent any potential security vulnerabilities or data integrity issues. Use client-side validation techniques and server-side validation to ensure that only valid and safe data is transmitted.
2. Sanitize and escape user input
When processing user input, make sure to sanitize and escape the data to prevent any potential security risks, such as cross-site scripting (XSS) attacks. Use libraries or built-in functions to handle input sanitization and escaping.
3. Use HTTPS for secure communication
Always use HTTPS for data transmission to ensure secure communication between the client and the server. HTTPS encrypts the data and protects it from unauthorized access. Obtain and install a valid SSL certificate for your server to enable HTTPS.
4. Implement proper authentication and authorization
Implement proper authentication and authorization mechanisms to ensure that only authorized users can access and transmit data to the server. Use secure token-based authentication or other authentication methods recommended for your application.
5. Implement rate limiting and throttling
To prevent abuse or denial-of-service attacks, implement rate limiting and throttling mechanisms to control the number of requests that can be made within a certain time period. This helps protect your server from excessive traffic and ensures fair resource allocation.
6. Handle errors and provide feedback
When sending data to the server, handle any errors that may occur and provide appropriate feedback to the user. Display error messages or notifications to inform the user about the status of their data transmission and guide them on how to resolve any issues.
7. Use optimized requests
Optimize your data transmission by minimizing the size of the requests and responses. Use compression techniques, such as gzip or brotli, to reduce the payload size. Avoid sending unnecessary data and only transmit the required information.
8. Implement caching
Implement caching mechanisms to reduce the load on the server and improve the performance of data transmission. Use appropriate caching headers and techniques to store and retrieve data from the client's cache whenever possible.
9. Monitor and analyze performance
Regularly monitor and analyze the performance of your data transmission to identify any bottlenecks or areas for improvement. Use tools like browser developer tools, network analyzers, or performance monitoring services to gain insights into the performance of your application.
10. Stay up-to-date with security practices
Keep yourself updated with the latest security practices and vulnerabilities related to data transmission. Follow security blogs, subscribe to security mailing lists, and participate in security communities to stay informed about potential risks and best practices.
Related Article: Exploring Differences in Rendering Components in ReactJS
Additional Resources
- Role of the Content-Type header when sending data in ReactJS