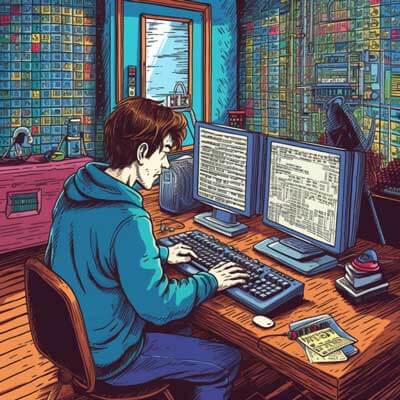
Table of Contents
When it comes to deploying Phoenix apps, there are several strategies and techniques that can be used depending on the specific needs of your application. In this section, we will explore some of the most common deployment strategies and discuss when and how to use them.
Using Releases and Hot Upgrades to Deploy Phoenix Apps
One popular deployment strategy for Phoenix apps is using releases and hot upgrades. Releases allow you to package your application along with all its dependencies into a single executable file. This makes it easy to deploy your application to different environments without worrying about dependencies or configuration issues.
To create a release for your Phoenix app, you can use the mix release command. This command will generate a release package that can then be deployed to your target environment. Here is an example of how to create a release for a Phoenix app:
mix release
Once you have created a release, you can deploy it to your target environment using various deployment tools and techniques. One popular approach is to use a tool like Distillery, which provides additional functionality for managing releases and deployments.
Hot upgrades, on the other hand, allow you to deploy updates to your Phoenix app without any downtime. With hot upgrades, you can apply changes to your application code while it is still running, ensuring that your users don't experience any interruption in service.
To perform a hot upgrade, you can use tools like the Erlang VM's built-in support for hot code swapping. This allows you to update your application code on the fly, without restarting the entire application. Here is an example of how to perform a hot upgrade for a Phoenix app:
defmodule MyApp.Release do def upgrade() do # Perform necessary upgrade tasks here :ok end end
Related Article: Optimizing Database Queries with Elixir & Phoenix
Scaling Techniques for Phoenix Apps
Scaling Phoenix apps is crucial to ensure that your application can handle increasing traffic and user demand. In this section, we will explore some scaling techniques that can be used to horizontally scale Phoenix apps and handle high traffic loads.
The Benefits of Horizontal Scaling for Phoenix Apps
Horizontal scaling involves adding more instances of your application to distribute the incoming traffic load. It offers several benefits, including improved performance, increased reliability, and better fault tolerance. By horizontally scaling your Phoenix app, you can handle more concurrent requests and ensure that your application remains responsive and available.
To horizontally scale a Phoenix app, you can use distributed Elixir, a useful feature of the Elixir programming language. Distributed Elixir allows you to distribute your application across multiple nodes, each running on a separate machine. This enables you to handle more traffic by leveraging the processing power of multiple machines.
To enable distributed Elixir in your Phoenix app, you need to configure the :name
and :cookie
options in your config.exs
file. Here is an example:
config :my_app, MyApp.Endpoint, http: [port: 4000], server: true, code_reloader: false, check_origin: false, watchers: []
Once you have configured distributed Elixir, you can start additional nodes by running the following command:
iex --name node2@localhost --cookie secret_cookie -S mix phx.server
Scaling Phoenix Apps with Distributed Elixir
Distributed Elixir allows you to scale your Phoenix app horizontally by distributing the workload across multiple nodes. Each node can handle a subset of the incoming requests, resulting in improved performance and scalability.
To distribute the workload across multiple nodes, you can use a load balancer to evenly distribute the incoming requests. The load balancer acts as a proxy and forwards the requests to the available nodes based on various algorithms, such as round-robin or least connections.
There are several load balancing solutions available, both open-source and commercial. One popular open-source load balancer is HAProxy. Here is an example configuration for HAProxy:
frontend http-in bind *:80 default_backend servers backend servers balance roundrobin server node1 127.0.0.1:4000 check server node2 127.0.0.1:4001 check
In this example, HAProxy is configured to forward incoming requests to two Phoenix app nodes running on ports 4000 and 4001.
Related Article: Elixir’s Phoenix Security: Token Auth & CSRF Prevention
Monitoring Phoenix Applications
Monitoring Phoenix applications is essential to ensure that your app is performing optimally and to identify any potential issues or bottlenecks. In this section, we will explore some tools and techniques for monitoring Phoenix apps.
Using Telemetry to Monitor Phoenix Apps
Telemetry is a useful monitoring and observability framework for Elixir applications, including Phoenix apps. It allows you to collect, process, and visualize metrics and events from your application in real-time.
To use Telemetry in your Phoenix app, you need to add the telemetry
package as a dependency in your mix.exs
file:
defp deps do [ {:telemetry, "~> 0.4"} ] end
Once you have added the dependency, you can start instrumenting your application code to emit telemetry events. Telemetry provides hooks that you can use to instrument various parts of your application, such as controllers, views, and database queries.
Here is an example of how to instrument a Phoenix controller action using Telemetry:
defmodule MyAppWeb.MyController do use MyAppWeb, :controller def action(conn, _params) do :telemetry.execute([:my_app, :controller, :action], %{conn: conn}, &do_action/1) end defp do_action(_args) do # Perform your action here end end
AppSignal for Monitoring Phoenix Apps
AppSignal is a comprehensive application monitoring tool that provides real-time insights into the performance and health of your Phoenix app. It offers features such as error tracking, performance monitoring, and alerting.
To use AppSignal in your Phoenix app, you need to add the appsignal
package as a dependency in your mix.exs
file:
defp deps do [ {:appsignal, "~> 1.6"} ] end
Once you have added the dependency, you need to configure AppSignal with your API key. You can do this by adding the following configuration to your config.exs
file:
config :appsignal, api_key: "your_api_key"
After configuring AppSignal, you can start tracking errors and monitoring performance by instrumenting your application code. AppSignal provides an Elixir library that you can use to track errors, measure performance, and add custom instrumentation.
Here is an example of how to track an error using AppSignal:
defmodule MyAppWeb.MyController do use MyAppWeb, :controller def action(conn, _params) do try do # Perform your action here rescue exception -> Appsignal.send_error(exception) raise exception end end end
Best Practices for Deploying Phoenix Apps
When deploying Phoenix apps, it is important to follow best practices to ensure a smooth and successful deployment. In this section, we will discuss some best practices for deploying Phoenix apps.
Related Article: Internationalization & Encoding in Elixir Phoenix
Ensuring High Availability when Scaling Phoenix Apps
When scaling Phoenix apps horizontally, it is important to ensure high availability to minimize downtime and provide a seamless experience for your users. Here are some best practices to ensure high availability when scaling Phoenix apps:
- Use a load balancer: As mentioned earlier, using a load balancer is essential when scaling Phoenix apps. A load balancer distributes incoming requests across multiple app nodes, ensuring that the workload is evenly distributed and providing fault tolerance in case of node failures.
- Implement health checks: To ensure that your app nodes are healthy and able to handle requests, implement health checks that periodically monitor the state of each node. If a node is unresponsive or experiencing issues, the load balancer can automatically remove it from the pool of available nodes, preventing it from serving requests until it is back to a healthy state.
- Use a distributed database: When scaling Phoenix apps, it is important to ensure that your database can handle the increased load. Using a distributed database, such as Apache Cassandra or CockroachDB, allows you to distribute the data across multiple nodes, providing better performance and fault tolerance.
- Implement graceful shutdowns: When deploying updates or scaling down your app nodes, it is important to gracefully shut down the nodes to ensure that there is no interruption in service. Implement a mechanism to notify the load balancer that a node is being taken down, allowing it to stop sending requests to that node before shutting it down.