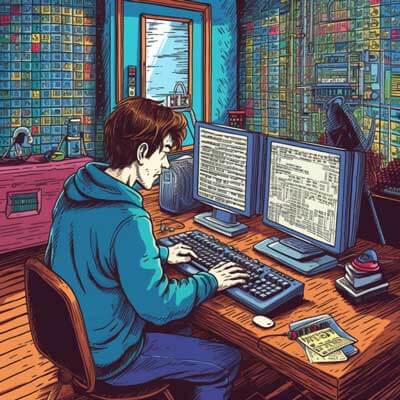
Table of Contents
In ReactJS, displaying arrays with onclick events involves handling click events to trigger the display of an array, updating the state to render the array, and using the map function to iterate over the array and render its elements. This allows for dynamic rendering of arrays based on user interaction.
How to Initialize an Array in ReactJS
To initialize an array in ReactJS, you can make use of the useState hook. The useState hook allows you to declare a state variable and initialize it with a default value, in this case, an array.
import React, { useState } from 'react';function MyComponent() { const [myArray, setMyArray] = useState([]); // Rest of the component code}
In the example above, we have imported the useState hook from the 'react' package and declared a state variable called myArray with an initial value of an empty array. We have also declared a setter function setMyArray to update the value of myArray.
Related Article: Comparing Reactivity in ReactJS and VueJS Variables
How to Handle Click Events in ReactJS
To handle click events in ReactJS, you can make use of the onClick event handler attribute. This attribute allows you to specify a function that will be called when the associated element is clicked.
import React from 'react';function handleClick() { console.log('Button clicked!');}function MyComponent() { return ( <button onClick={handleClick}>Click me</button> );}
In the example above, we have defined a handleClick function that logs a message to the console when called. We have then used the onClick attribute in the button element to associate the handleClick function with the click event of the button.
How to Update State in ReactJS
To update the state in ReactJS, you can make use of the setter function provided by the useState hook. This function allows you to update the value of the associated state variable.
import React, { useState } from 'react';function MyComponent() { const [count, setCount] = useState(0); function handleClick() { setCount(count + 1); } return ( <div> <p>Count: {count}</p> <button onClick={handleClick}>Increment</button> </div> );}
In the example above, we have declared a state variable count and its associated setter function setCount using the useState hook. We have also defined a handleClick function that updates the value of count by incrementing it. The updated count value is then displayed in the component.
How to Render an Array in ReactJS
To render an array in ReactJS, you can make use of the map function. The map function allows you to iterate over each element in the array and return a new array with modified values.
import React from 'react';function MyComponent() { const names = ['John', 'Jane', 'Bob']; return ( <ul> {names.map((name, index) => ( <li key={index}>{name}</li> ))} </ul> );}
In the example above, we have declared an array of names and used the map function to iterate over each name and return a new array of list items. Each list item is then displayed within an unordered list.
Related Article: How to Install Webpack in ReactJS: A Step by Step Process
How to Use onClick Event in ReactJS
To use the onClick event in ReactJS, you can associate it with a function that will be called when the associated element is clicked.
import React from 'react';function handleClick() { console.log('Button clicked!');}function MyComponent() { return ( <button onClick={handleClick}>Click me</button> );}
In the example above, we have defined a handleClick function that logs a message to the console when called. We have then used the onClick attribute in the button element to associate the handleClick function with the click event of the button.
What is the useState Hook in ReactJS
The useState hook is a built-in hook in ReactJS that allows functional components to have state. It provides a way to declare state variables and their associated setter functions, allowing you to manage and update state within functional components.
import React, { useState } from 'react';function MyComponent() { const [count, setCount] = useState(0); return ( <div> <p>Count: {count}</p> <button onClick={() => setCount(count + 1)}>Increment</button> </div> );}
In the example above, we have imported the useState hook from the 'react' package and declared a state variable count with an initial value of 0. We have also declared a setter function setCount to update the value of count. The updated count value is then displayed in the component and can be incremented by clicking the button.
How to Use the Map Function in ReactJS
The map function is a built-in function in JavaScript that allows you to iterate over each element in an array and perform a specified operation on each element. In ReactJS, the map function is commonly used to render arrays by returning a new array of JSX elements.
import React from 'react';function MyComponent() { const names = ['John', 'Jane', 'Bob']; return ( <ul> {names.map((name, index) => ( <li key={index}>{name}</li> ))} </ul> );}
In the example above, we have declared an array of names and used the map function to iterate over each name and return a new array of list items. Each list item is then displayed within an unordered list.
How to Conditionally Render an Array in ReactJS
To conditionally render an array in ReactJS, you can make use of conditional statements such as if statements or ternary operators to determine whether the array should be rendered or not.
import React from 'react';function MyComponent() { const showArray = true; const names = ['John', 'Jane', 'Bob']; return ( <div> {showArray && ( <ul> {names.map((name, index) => ( <li key={index}>{name}</li> ))} </ul> )} </div> );}
In the example above, we have declared a boolean variable showArray and set it to true. We have also declared an array of names. Using the conditional statement {showArray && ...}, we conditionally render the array only if showArray is true. If showArray is false, the array will not be rendered.
Related Article: How to Use a For Loop Inside Render in ReactJS
How to Show an Array on Click in ReactJS
To show an array on click in ReactJS, you can make use of state variables and click event handlers. By updating the state when the click event occurs, you can conditionally render the array based on the state value.
import React, { useState } from 'react';function MyComponent() { const [showArray, setShowArray] = useState(false); const names = ['John', 'Jane', 'Bob']; function handleClick() { setShowArray(true); } return ( <div> <button onClick={handleClick}>Show Array</button> {showArray && ( <ul> {names.map((name, index) => ( <li key={index}>{name}</li> ))} </ul> )} </div> );}
In the example above, we have declared a state variable showArray with an initial value of false. We have also declared an array of names. When the button is clicked, the handleClick function is called and updates the value of showArray to true. This triggers the conditional rendering of the array.
How to Update an Array on Click in ReactJS
To update an array on click in ReactJS, you can make use of state variables and click event handlers. By updating the state when the click event occurs, you can modify the array and trigger a re-render of the component.
import React, { useState } from 'react';function MyComponent() { const [names, setNames] = useState(['John', 'Jane', 'Bob']); function handleClick() { const updatedNames = [...names, 'Alice']; setNames(updatedNames); } return ( <div> <button onClick={handleClick}>Add Name</button> <ul> {names.map((name, index) => ( <li key={index}>{name}</li> ))} </ul> </div> );}
In the example above, we have declared a state variable names and its associated setter function setNames using the useState hook. We have also defined a handleClick function that updates the value of names by adding a new name to the array. The updated names array is then rendered in the component.
Additional Resources
- ReactJS - Passing Data Between Components