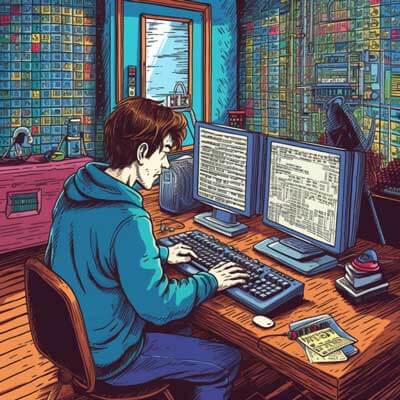
Table of Contents
To display memory usage in a bash script, you can make use of various Linux commands and utilities. One such command is free
, which provides information about the system's memory usage. Here's an example of how you can use free
within a bash script to display memory usage:
#!/bin/bash # Get memory usage memory=$(free -m | awk 'NR==2{printf "%.2f%%", $3*100/$2 }') # Print memory usage echo "Memory Usage: $memory"
In this example, the free
command is used to retrieve memory information. The output of free
is then piped to awk
, which extracts the memory usage percentage. Finally, the memory usage is printed using the echo
command.
The Top Command
To check memory usage in Linux, you can make use of various commands and utilities. One commonly used command is top
, which provides real-time information about system resources, including memory usage. Here's an example of how you can use top
to check memory usage in Linux:
top -n 1 | grep "KiB Mem"
This command will display the current memory usage in kilobytes. You can modify the command to display memory usage in different units, such as megabytes (MB
) or gigabytes (GB
), by using appropriate flags or conversion factors.
Related Article: Should You Use Numbers in Bash Script Names?
The Vmstat Command
In addition to top
, Linux provides several other commands to check memory usage. One such command is vmstat
, which provides information about virtual memory statistics. Here's an example of how you can use vmstat
to check memory usage in Linux:
vmstat -s
This command will display detailed information about memory usage, including total memory, free memory, used memory, and more. You can further customize the output by using different flags or options with the vmstat
command.
The Sar Command
Monitoring memory usage is crucial for ensuring optimal system performance. In a bash script, you can use tools like sar
(System Activity Reporter) to monitor memory usage over time. Here's an example of how you can use sar
to monitor memory usage in Linux:
sar -r 1 10
This command will display memory usage statistics every second for a duration of 10 seconds. You can adjust the duration and frequency of monitoring as per your requirements. The output of sar
includes information like free memory, used memory, buffers, cache, and more.
The Ps Command
To show memory usage in a bash script, you can use the ps
command to retrieve information about processes and their memory usage. Here's an example of how you can use ps
to show memory usage in Linux:
#!/bin/bash # Get memory usage of a specific process pid=$(pgrep -f "your_process_name") memory=$(ps -p $pid -o %mem) # Print memory usage echo "Memory Usage: $memory%"
In this example, the pgrep
command is used to retrieve the process ID (pid
) of a specific process using its name. The ps
command is then used to retrieve the memory usage (%mem
) of the process identified by the pid
. Finally, the memory usage is printed using the echo
command.
Related Article: How to Use an And Operator in an If Statement in Bash
Combining multiple Commands and Utilities
To get memory usage in a bash script, you can combine multiple commands and utilities to extract the desired information. Here's an example of a bash script that gets memory usage in Linux:
#!/bin/bash # Get total memory total_memory=$(grep "MemTotal" /proc/meminfo | awk '{print $2}') # Get available memory available_memory=$(grep "MemAvailable" /proc/meminfo | awk '{print $2}') # Calculate used memory used_memory=$((total_memory - available_memory)) # Calculate memory usage percentage memory_usage=$(awk "BEGIN {printf \"%.2f\", ($used_memory / $total_memory) * 100}") # Print memory usage echo "Memory Usage: $memory_usage%"
In this example, the script uses grep
and awk
to extract the total memory and available memory from the /proc/meminfo
file. It then calculates the used memory by subtracting the available memory from the total memory. Finally, it calculates the memory usage percentage and prints the result.
Summary of the Methods to Get Memory Usage
There are multiple methods to get memory usage in a bash script in Linux. Some of the commonly used methods include using commands like free
, top
, vmstat
, and parsing the /proc/meminfo
file. Here are a few examples:
Method 1: Using free
command
#!/bin/bash # Get memory usage memory_usage=$(free -m | awk 'NR==2{printf "%.2f%%", $3*100/$2 }') # Print memory usage echo "Memory Usage: $memory_usage"
Method 2: Using top
command
#!/bin/bash # Get memory usage memory_usage=$(top -b -n 1 | grep "KiB Mem" | awk '{print $8}') # Print memory usage echo "Memory Usage: $memory_usage"
Method 3: Using vmstat
command
#!/bin/bash # Get memory usage memory_usage=$(vmstat -s | grep "total memory" | awk '{printf "%.2f%%", ($1-$2)*100/$1}') # Print memory usage echo "Memory Usage: $memory_usage"
Method 4: Parsing /proc/meminfo
file
#!/bin/bash # Get total memory total_memory=$(grep "MemTotal" /proc/meminfo | awk '{print $2}') # Get available memory available_memory=$(grep "MemAvailable" /proc/meminfo | awk '{print $2}') # Calculate used memory used_memory=$((total_memory - available_memory)) # Calculate memory usage percentage memory_usage=$(awk "BEGIN {printf \"%.2f\", ($used_memory / $total_memory) * 100}") # Print memory usage echo "Memory Usage: $memory_usage%"
These methods provide different ways to retrieve memory usage in a bash script. You can choose the method that best suits your requirements and incorporate it into your scripts accordingly.
Additional Resources
- Is there a specific command to monitor memory usage in Linux?