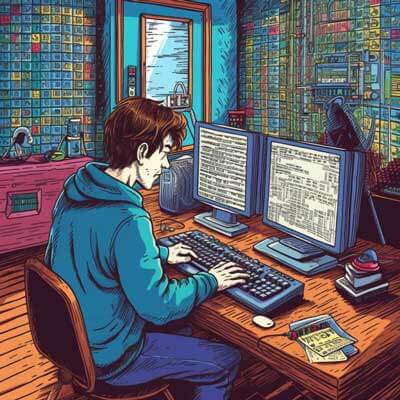
- Understanding ReactJS Component Styling
- How to Style a Button in ReactJS using Styled Components
- Advantages of Using Styled Components in ReactJS
- Event Handling in ReactJS
- Handling onClick Events in ReactJS
- Function Components vs Class Components in ReactJS
- Styling a Button in ReactJS with Inline CSS
- Compatibility of Styled Components with CSS Frameworks
- Do Styled Component Buttons in ReactJS Require an Onclick?
- Best Practices for Event Handling in ReactJS
- Passing Arguments to onClick Event Handler Function in ReactJS
Understanding ReactJS Component Styling
ReactJS is a popular JavaScript library for building user interfaces. One of the key aspects of building a user interface is styling the components. In ReactJS, there are several ways to style components, including using CSS classes, inline styles, CSS modules, and third-party libraries. One of the most useful and flexible ways to style components in ReactJS is by using Styled Components.
Related Article: Exploring Differences in Rendering Components in ReactJS
How to Style a Button in ReactJS using Styled Components
Styled Components is a library that allows you to write CSS code within your JavaScript code. It provides a way to define reusable styled components that can be used throughout your application. Let’s see how to style a button using Styled Components in ReactJS.
First, we need to install the Styled Components library:
npm install styled-components
Once installed, we can create a styled button component by importing the necessary functions from the Styled Components library:
import styled from 'styled-components';
Next, we can define our styled button component by using the styled function:
const Button = styled.button` background-color: blue; color: white; padding: 10px 20px; border: none; border-radius: 5px; cursor: pointer; `;
In the above code, we define a styled button component by using the styled function and passing a template literal as an argument. Inside the template literal, we write our CSS code to style the button. In this example, we set the background color to blue, the text color to white, and add some padding and border radius to the button. We also set the cursor to pointer to indicate that the button is clickable.
Now, we can use our styled button component in our ReactJS application:
import React from 'react'; function App() { return ( <div> <Button>Click me</Button> </div> ); } export default App;
In the above code, we import our styled button component and use it inside our App component. We simply render the styled button component and pass the text “Click me” as its child.
Advantages of Using Styled Components in ReactJS
Styled Components offer several advantages over traditional CSS styling in ReactJS. Here are some of the key advantages:
1. Encapsulation: Styled Components encapsulate the styles within the component, preventing styles from leaking out and affecting other components. This makes it easier to manage and reason about the styles in your application.
2. Reusability: With Styled Components, you can define reusable styled components that can be used throughout your application. This promotes code reuse and makes it easier to maintain a consistent look and feel across your application.
3. Dynamic styling: Styled Components allow you to dynamically style components based on props or state. This makes it easy to create responsive designs or apply different styles based on user interactions.
4. Interpolation: Styled Components support interpolation, which allows you to dynamically generate styles based on JavaScript expressions. This gives you greater flexibility and control over your styles.
5. Theming: Styled Components make it easy to create themes for your application. You can define theme variables and use them in your styled components, allowing you to easily change the look and feel of your application by changing the theme.
Event Handling in ReactJS
Event handling is an important aspect of building interactive user interfaces in ReactJS. React provides a synthetic event system that abstracts away the differences between different browsers and provides a consistent API for handling events.
In React, events are triggered by user actions, such as clicking a button, typing in an input field, or scrolling the page. When an event is triggered, React creates a synthetic event object and passes it to the event handler function.
To handle events in React, you can use the onEventName
props on the corresponding DOM element. For example, to handle the onClick
event on a button, you can use the onClick
prop:
function handleClick() { console.log('Button clicked'); } function App() { return ( <div> <button onClick={handleClick}>Click me</button> </div> ); }
In the above code, we define a function handleClick
that logs a message to the console when the button is clicked. We then pass this function as the onClick
prop to the button element.
React also provides synthetic event objects that contain additional information about the event, such as the target element, the key that was pressed, or the mouse coordinates. You can access this information in the event handler function by accepting the event object as a parameter:
function handleClick(event) { console.log('Button clicked'); console.log('Target element:', event.target); }
In the above code, we log the target element of the event to the console. This can be useful if you need to access information about the element that triggered the event.
Related Article: How Component Interaction Works in ReactJS
Handling onClick Events in ReactJS
The onClick
event is one of the most commonly used events in ReactJS. It is triggered when a user clicks on an element, such as a button or a link. In this section, we will explore how to handle onClick
events in ReactJS.
To handle onClick
events in ReactJS, you can define an event handler function and pass it as the onClick
prop to the element. The event handler function will be called when the element is clicked.
Here is an example of how to handle onClick
events in ReactJS:
import React from 'react'; function handleClick() { console.log('Button clicked'); } function App() { return ( <div> <button onClick={handleClick}>Click me</button> </div> ); } export default App;
In the above code, we define a function handleClick
that logs a message to the console when the button is clicked. We then pass this function as the onClick
prop to the button element.
You can also pass arguments to the event handler function by using arrow functions or by using the bind
method. Here is an example of how to pass arguments to the event handler function:
import React from 'react'; function handleClick(name) { console.log(`Hello, ${name}!`); } function App() { return ( <div> <button onClick={() => handleClick('John')}>Click me</button> </div> ); } export default App;
In the above code, we define a function handleClick
that takes a name
parameter and logs a message to the console using the name
parameter. We pass an arrow function as the onClick
prop to the button element, which calls the handleClick
function with the argument 'John'
.
Function Components vs Class Components in ReactJS
In ReactJS, there are two types of components: function components and class components. Both types of components serve the same purpose of rendering UI elements, but they have some differences in terms of syntax and features.
Function components are the simpler and newer way to write components in ReactJS. They are defined as regular JavaScript functions that return JSX elements. Here is an example of a function component:
import React from 'react'; function Welcome(props) { return <h1>Hello, {props.name}!</h1>; } export default Welcome;
In the above code, we define a function component called Welcome
that takes a name
prop and returns a JSX element. The JSX element is a heading element that displays a greeting message with the value of the name
prop.
Class components, on the other hand, are defined as ES6 classes that extend the React.Component
class. They have more features than function components, such as lifecycle methods and state. Here is an example of a class component:
import React from 'react'; class Welcome extends React.Component { render() { return <h1>Hello, {this.props.name}!</h1>; } } export default Welcome;
In the above code, we define a class component called Welcome
that extends the React.Component
class. The render
method of the class component returns a JSX element, similar to the function component.
Function components are recommended for most cases, as they are simpler, easier to read, and perform better. However, there are some cases where class components are necessary, such as when using lifecycle methods or managing state.
Styling a Button in ReactJS with Inline CSS
In addition to using Styled Components, ReactJS also provides the option to style a button using inline CSS. Inline CSS is a way to define styles directly within the JSX code, using the style
prop.
Here is an example of how to style a button using inline CSS in ReactJS:
import React from 'react'; function App() { const buttonStyle = { backgroundColor: 'blue', color: 'white', padding: '10px 20px', border: 'none', borderRadius: '5px', cursor: 'pointer', }; return ( <div> <button style={buttonStyle}>Click me</button> </div> ); } export default App;
In the above code, we define an object buttonStyle
that contains the CSS properties and values for styling the button. We then pass this object as the style
prop to the button element.
Inline CSS can also accept dynamic values by using JavaScript expressions. Here is an example of how to use dynamic values in inline CSS:
import React from 'react'; function App() { const buttonColor = 'blue'; const buttonStyle = { backgroundColor: buttonColor, color: 'white', padding: '10px 20px', border: 'none', borderRadius: '5px', cursor: 'pointer', }; return ( <div> <button style={buttonStyle}>Click me</button> </div> ); } export default App;
In the above code, we define a variable buttonColor
that holds the value ‘blue’. We then use this variable as the value for the backgroundColor
property in the buttonStyle
object.
Using inline CSS can be convenient for small-scale styling, but it can become cumbersome for larger projects. It can also make it harder to maintain a consistent look and feel across your application. In such cases, using Styled Components or other CSS-in-JS libraries may be a better choice.
Related Article: How To Pass Parameters to Components in ReactJS & TypeScript
Compatibility of Styled Components with CSS Frameworks
Styled Components are compatible with CSS frameworks such as Bootstrap, Material-UI, and Tailwind CSS. You can use Styled Components alongside these frameworks to style your components in a more modular and flexible way.
To use Styled Components with a CSS framework, you can create a styled component that extends the styles of a component from the framework. Here is an example of how to use Styled Components with Bootstrap:
import React from 'react'; import styled from 'styled-components'; import { Button as BootstrapButton } from 'react-bootstrap'; const StyledButton = styled(BootstrapButton)` background-color: blue; color: white; padding: 10px 20px; border: none; border-radius: 5px; cursor: pointer; `; function App() { return ( <div> <StyledButton>Click me</StyledButton> </div> ); } export default App;
In the above code, we import the Button
component from the react-bootstrap
library and create a styled component called StyledButton
that extends the styles of the Button
component. We then use the StyledButton
component in our app.
Do Styled Component Buttons in ReactJS Require an Onclick?
In ReactJS, the onClick
event is not strictly necessary for button components. However, it is highly recommended to include an onClick
event handler for button components that are meant to be interactive.
The onClick
event allows you to define a function that will be called when the button is clicked. This function can perform any action or update the state of your application. Without an onClick
event handler, the button component would be static and not respond to user interactions.
Here is an example of a button component without an onClick
event handler:
import React from 'react'; function Button() { return <button>Click me</button>; } export default Button;
In the above code, we define a simple button component that does not have an onClick
event handler. This button component will be rendered as a static button that does not respond to user interactions.
On the other hand, here is an example of a button component with an onClick
event handler:
import React from 'react'; function handleClick() { console.log('Button clicked'); } function Button() { return <button onClick={handleClick}>Click me</button>; } export default Button;
In the above code, we define a function handleClick
that logs a message to the console when the button is clicked. We then pass this function as the onClick
prop to the button element. This button component will respond to user interactions by calling the handleClick
function.
Including an onClick
event handler for button components allows you to add interactivity and functionality to your application. It gives users the ability to perform actions and trigger events by clicking on the button.
Best Practices for Event Handling in ReactJS
When handling events in ReactJS, it is important to follow some best practices to ensure clean and maintainable code. Here are some best practices for event handling in ReactJS:
1. Use arrow functions or bind the event handler function: When passing an event handler function as a prop, it is recommended to use arrow functions or bind the function to the correct context. This ensures that the function is called with the correct this
value and any necessary arguments.
// Using arrow function <button onClick={() => handleClick()}>Click me</button> // Binding the function <button onClick={handleClick.bind(this)}>Click me</button>
2. Avoid using inline arrow functions: Inline arrow functions can be convenient, but they can also cause unnecessary re-renders of components. It is generally better to define the event handler function outside of the render function and pass it as a prop.
3. Use synthetic event pooling: By default, React reuses synthetic event objects for performance reasons. However, if you need to access the event object asynchronously, it may be nullified. To avoid this issue, you can call the event.persist()
method to remove the event from the pool and ensure that it is not nullified.
function handleClick(event) { event.persist(); setTimeout(() => { console.log(event.target); }, 1000); }
4. Use event delegation when handling events for multiple elements: If you have multiple elements that need to handle the same event, it is more efficient to use event delegation instead of attaching individual event handlers to each element. You can use the event.target
property to determine which element triggered the event.
5. Consider using a state management library: If your application has complex state management requirements, you may benefit from using a state management library such as Redux or MobX. These libraries provide a predictable way to manage and update the state of your application, including handling events.
Related Article: Extracting URL Parameters in Your ReactJS Component
Passing Arguments to onClick Event Handler Function in ReactJS
In ReactJS, you can pass arguments to the onClick
event handler function by using arrow functions or by using the bind
method. This allows you to pass dynamic values to the event handler based on the context or user interactions.
Here is an example of how to pass arguments to the onClick
event handler function using arrow functions:
import React from 'react'; function handleClick(name) { console.log(`Hello, ${name}!`); } function App() { return ( <div> <button onClick={() => handleClick('John')}>Click me</button> </div> ); } export default App;
In the above code, we define a function handleClick
that takes a name
parameter and logs a message to the console using the name
parameter. We pass an arrow function as the onClick
prop to the button element, which calls the handleClick
function with the argument 'John'
.
Alternatively, you can use the bind
method to pass arguments to the onClick
event handler function:
import React from 'react'; function handleClick(name) { console.log(`Hello, ${name}!`); } function App() { return ( <div> <button onClick={handleClick.bind(null, 'John')}>Click me</button> </div> ); } export default App;
In the above code, we use the bind
method to bind the handleClick
function to the null
context and pass the argument 'John'
. This creates a new function that, when called, will call the handleClick
function with the provided argument.