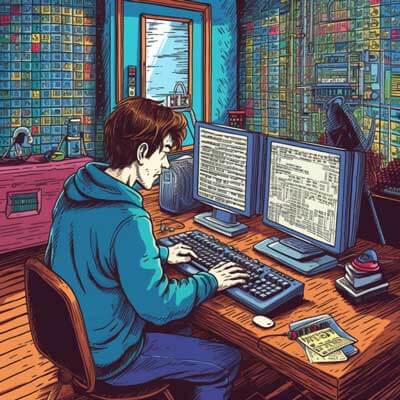
Table of Contents
Configuring ReactJS
When working with ReactJS, it is important to properly configure your project to ensure smooth and efficient file rendering. Configuring ReactJS involves setting up the necessary dependencies, optimizing the build process, and configuring any additional tools or libraries that may be required.
To get started, you will need to have Node.js installed on your machine. Node.js comes with npm (Node Package Manager), which allows you to easily install and manage dependencies for your ReactJS project.
Once you have Node.js installed, you can create a new ReactJS project by running the following command in your terminal:
npx create-react-app my-app
This will create a new directory called "my-app" with all the necessary configuration files and a basic ReactJS template.
Next, navigate to the project directory by running:
cd my-app
Now, you can start the development server by running:
npm start
This will launch your ReactJS application in your browser at http://localhost:3000
. You can now make changes to your code and see the changes reflected in real-time.
Related Article: How to Add Navbar Components for Different Pages in ReactJS
Installing Additional Dependencies
In addition to the default dependencies installed by Create React App, you may need to install additional dependencies depending on your specific requirements. For example, if you need to render files from a configuration file, you may need to install libraries that support file rendering, such as react-file-renderer
.
To install a new dependency, you can use the following command:
npm install <dependency-name>
For example, to install react-file-renderer
, you can run:
npm install react-file-renderer
Understanding File Rendering in ReactJS
File rendering in ReactJS refers to the process of displaying files, such as images, videos, or documents, within a React component. React offers several mechanisms for rendering files, including using static file paths or dynamically rendering files based on a configuration file.
When rendering files in ReactJS, it is important to consider performance, scalability, and maintainability. Rendering large files or a large number of files can impact the performance of your application. Therefore, it is important to optimize file rendering to ensure a smooth user experience.
Static File Rendering
One way to render files in ReactJS is by using static file paths. This involves specifying the file path directly within your component, using the appropriate HTML element to display the file.
For example, to render an image file, you can use the <img>
element and set the src
attribute to the file path:
import React from 'react'; function ImageComponent() { return ( <img src="/path/to/image.jpg" alt="Image" /> ); } export default ImageComponent;
Similarly, you can use other HTML elements, such as <video>
or <iframe>
, to render different types of files.
While static file rendering is simple and straightforward, it can become cumbersome if you have a large number of files or if you need to dynamically render files based on a configuration file.
Related Article: Exploring Buffer Usage in ReactJS
Rendering Files from Config in ReactJS
Rendering files from a configuration file in ReactJS allows you to dynamically render files based on a set of predefined rules or configurations. This approach offers more flexibility and scalability compared to static file rendering.
To render files from a configuration file, you will need to define the configuration file and write the necessary code to parse the file and render the files accordingly.
Creating a Configuration File
First, let's create a configuration file that defines the files to be rendered. You can use a JSON file or any other format that is easy to parse and understand.
For example, let's create a files.json
file with the following content:
{ "files": [ { "type": "image", "path": "/path/to/image.jpg" }, { "type": "video", "path": "/path/to/video.mp4" } ] }
This configuration file defines two files: an image and a video file, along with their respective paths.
Rendering Files from the Configuration
Next, let's write the code to parse the configuration file and render the files accordingly.
import React from 'react'; import files from './files.json'; function FileRenderer() { return ( <div> {files.map((file, index) => { if (file.type === 'image') { return <img key={index} src={file.path} alt="Image" />; } else if (file.type === 'video') { return <video key={index} src={file.path} controls />; } else { return null; } })} </div> ); } export default FileRenderer;
In this example, we import the files
array from the files.json
file. We then use the map
function to iterate over each file in the array and render the corresponding file based on its type.
If the file type is "image", we render an <img>
element with the specified file path. If the file type is "video", we render a <video>
element with the specified file path and the controls
attribute to display the video controls.
Best Practices for File Rendering in ReactJS
When rendering files in ReactJS, it is important to follow best practices to ensure optimal performance and maintainability. Here are some best practices to consider:
Related Article: How to Render ReactJS Code with NPM
Lazy Loading
Lazy loading is a technique that defers the loading of non-critical assets, such as images or videos, until they are needed. This can significantly improve the initial loading time of your application and reduce the amount of data transferred.
React offers several libraries, such as react-lazy-load
, that make it easy to implement lazy loading in your application. By lazy loading files, you can improve the overall performance and user experience.
Optimizing File Sizes
To ensure fast loading times, it is important to optimize the file sizes of your assets. This can be achieved by compressing images, converting videos to more efficient formats, or using techniques like minification and gzip compression.
There are several tools and libraries available that can help you optimize file sizes, such as imagemin
for image compression or ffmpeg
for video conversion. By optimizing file sizes, you can reduce the amount of data transferred and improve the performance of your application.
Libraries and Tools for File Rendering in ReactJS
When rendering files in ReactJS, there are several libraries and tools available that can simplify the process and provide additional features. Here are some popular libraries and tools for file rendering in ReactJS:
react-file-viewer
react-file-viewer is a useful library that allows you to render various types of files, including images, videos, documents, and more. It provides a simple and intuitive API for rendering files and supports a wide range of file formats.
To use react-file-viewer
, you can install it via npm:
npm install react-file-viewer
Then, you can import and use it in your React components:
import React from 'react'; import { FileViewer } from 'react-file-viewer'; function MyComponent() { const file = '/path/to/my/file.pdf'; const type = 'pdf'; return ( <FileViewer fileType={type} filePath={file} /> ); } export default MyComponent;
Related Article: How to Build Forms in React
react-pdf
react-pdf is a library specifically designed for rendering PDF files in ReactJS. It provides a React component that can render PDF files with high performance and smooth scrolling.
To use react-pdf
, you can install it via npm:
npm install react-pdf
Then, you can import and use it in your React components:
import React from 'react'; import { Document, Page } from 'react-pdf'; function PDFViewer() { const file = '/path/to/my/file.pdf'; return ( <Document file={file}> <Page pageNumber={1} /> </Document> ); } export default PDFViewer;
Rendering Different File Types in ReactJS
ReactJS provides various mechanisms for rendering different file types. Here are some examples:
Rendering Images
To render images in ReactJS, you can use the <img>
element and set the src
attribute to the file path. React will automatically load and display the image.
import React from 'react'; function ImageComponent() { return ( <img src="/path/to/image.jpg" alt="Image" /> ); } export default ImageComponent;
Rendering Videos
To render videos in ReactJS, you can use the <video>
element and set the src
attribute to the video file path. You can also use the controls
attribute to display the video controls.
import React from 'react'; function VideoComponent() { return ( <video src="/path/to/video.mp4" controls /> ); } export default VideoComponent;
Related Article: Handling Routing in React Apps with React Router
Rendering PDF Files
To render PDF files in ReactJS, you can use libraries such as react-pdf
or react-file-viewer
. These libraries provide React components specifically designed for rendering PDF files.
import React from 'react'; import { Document, Page } from 'react-pdf'; function PDFViewer() { const file = '/path/to/my/file.pdf'; return ( <Document file={file}> <Page pageNumber={1} /> </Document> ); } export default PDFViewer;
ReactJS File Rendering Mechanisms
ReactJS provides several mechanisms for rendering files. These mechanisms include:
Static File Paths
Static file paths involve specifying the file path directly within your component. This approach is simple and straightforward but can become cumbersome for large numbers of files or dynamically rendering files.
import React from 'react'; function ImageComponent() { return ( <img src="/path/to/image.jpg" alt="Image" /> ); } export default ImageComponent;
Rendering Files from Config
Rendering files from a configuration file allows you to dynamically render files based on a set of predefined rules or configurations. This approach offers more flexibility and scalability compared to static file rendering.
import React from 'react'; import files from './files.json'; function FileRenderer() { return ( <div> {files.map((file, index) => { if (file.type === 'image') { return <img key={index} src={file.path} alt="Image" />; } else if (file.type === 'video') { return <video key={index} src={file.path} controls />; } else { return null; } })} </div> ); } export default FileRenderer;
Related Article: Implementing HTML Templates in ReactJS
Advantages of Rendering Files from Config in ReactJS
Rendering files from a configuration file in ReactJS offers several advantages:
- Flexibility: By defining files in a configuration file, you can easily add, remove, or modify files without changing the code. This makes it easier to manage and update your files.
- Scalability: With a configuration file, you can easily handle a large number of files and render them dynamically based on different conditions or rules. This allows for more scalable file rendering in your ReactJS application.
- Maintainability: Separating file configurations from the code improves the maintainability of your ReactJS application. It allows for easier updates and reduces the risk of introducing errors.
Limitations and Considerations for File Rendering in ReactJS
While rendering files in ReactJS offers great flexibility and scalability, there are some limitations and considerations to keep in mind:
- Performance: Rendering large files or a large number of files can impact the performance of your ReactJS application. It is important to optimize file rendering to ensure a smooth user experience.
- File Types: ReactJS supports rendering a wide range of file types, but there may be some file types that require additional configuration or specialized libraries. Make sure to research the specific requirements for rendering different file types in your ReactJS application.
- Security: When rendering files from a configuration file, it is important to validate and sanitize user input to prevent any potential security vulnerabilities. Always ensure that the file paths are trusted and properly validated before rendering them.
- Accessibility: When rendering files, it is important to consider accessibility. Provide alternative text for images and ensure that videos have captions or transcripts available. Also, make sure that your file rendering components are accessible to users who rely on assistive technologies.
Additional Resources
- Understanding ReactJS - A Beginner's Guide