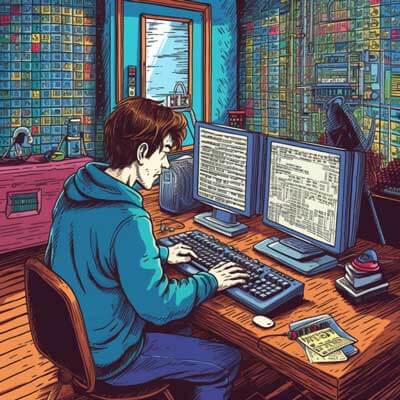
Table of Contents
Integrating Golang with Third-party and Open Source Tools
Golang, or Go, is a programming language developed by Google that has gained popularity for its simplicity, efficiency, and built-in support for concurrency. One of the strengths of Go is its ability to easily integrate with third-party and open source tools, allowing developers to leverage existing libraries and frameworks to enhance their applications.
When it comes to integrating Golang with third-party and open source tools, there are several key considerations to keep in mind. First, you'll need to identify the specific tool or library that you want to integrate with your Go application. This could be a CSS framework like Bootstrap, a search engine like Elasticsearch, or a database system like MySQL, PostgreSQL, or MongoDB. Once you've identified the tool or library, you'll need to understand how to interface with it using Go's native features or available Go clients.
In the following sections, we'll explore three specific areas of integration with third-party and open source tools: styling Beego front-ends using Bootstrap and CSS frameworks, integrating Beego with Elasticsearch using Go clients, and setting up a database with Beego's ORM.
Related Article: Deployment and Monitoring Strategies for Gin Apps
Styling Beego Front-ends using Bootstrap and CSS Frameworks
Beego is a popular web framework for building web applications in Go. It provides a robust set of features and tools that make it easy to develop scalable and efficient web applications. One aspect of web application development is the front-end design and styling, which can be enhanced using CSS frameworks like Bootstrap.
Bootstrap is a widely-used CSS framework that provides a collection of pre-built components and styles to help developers create responsive and visually appealing web interfaces. Integrating Bootstrap with Beego allows you to leverage these pre-built components and styles in your Beego applications.
To integrate Bootstrap with Beego, follow these steps:
1. Download Bootstrap CSS and JavaScript files from the official Bootstrap website or include them using a CDN.
2. Place the downloaded CSS and JavaScript files in your Beego project's static directory.
3. Import the CSS and JavaScript files in your Beego templates using the link
and script
tags.
4. Use the Bootstrap classes and components in your Beego templates to style your front-end elements.
Here's an example of how you can include Bootstrap in a Beego template:
<!DOCTYPE html> <html> <head> <title>My Beego Application</title> <link rel="stylesheet" href="/static/css/bootstrap.min.css"> <script src="/static/js/bootstrap.min.js"></script> </head> <body> <div class="container"> <h1>Welcome to My Beego Application</h1> <button class="btn btn-primary">Click me!</button> </div> </body> </html>
In this example, we include the Bootstrap CSS file using the link
tag and the Bootstrap JavaScript file using the script
tag. We then use the Bootstrap classes container
, h1
, and btn btn-primary
to style the elements of our Beego application.
Integrating Beego with Elasticsearch using Go Clients
Elasticsearch is a useful search engine that provides fast and scalable search capabilities for your applications. If you want to integrate Elasticsearch with your Beego application, you can use Go clients that provide an interface to interact with Elasticsearch's REST API.
One popular Go client for Elasticsearch is olivere/elastic
. It provides a high-level API for indexing, searching, and managing documents in Elasticsearch. To integrate Beego with Elasticsearch using olivere/elastic
, follow these steps:
1. Install the olivere/elastic
package using go get
:
go get gopkg.in/olivere/elastic.v7
2. Import the olivere/elastic
package in your Beego application:
import "gopkg.in/olivere/elastic.v7"
3. Create a new Elasticsearch client using the elastic.NewClient
function:
client, err := elastic.NewClient(elastic.SetURL("http://localhost:9200")) if err != nil { // Handle error } defer client.Stop()
4. Use the Elasticsearch client to perform operations like indexing documents, searching for documents, and managing the Elasticsearch cluster.
Here's an example of indexing a document in Elasticsearch using olivere/elastic
:
type Product struct { Name string `json:"name"` Price float64 `json:"price"` } product := Product{Name: "iPhone 12", Price: 999.99} _, err := client.Index(). Index("products"). BodyJson(product). Do(ctx) if err != nil { // Handle error }
In this example, we create a Product
struct and index it in Elasticsearch using the Index
function provided by olivere/elastic
. We specify the index name as "products" and pass the Product
struct as the document to be indexed.
Setting up a Database with Beego's ORM
Beego's ORM (Object-Relational Mapping) provides a convenient way to interact with databases in your Beego applications. It supports multiple databases, including MySQL, PostgreSQL, and MongoDB. In this section, we'll explore how to set up a database with Beego's ORM and perform basic database operations.
To set up a database with Beego's ORM, follow these steps:
1. Install the required database drivers for Beego's ORM. For MySQL, use the github.com/go-sql-driver/mysql
package. For PostgreSQL, use the github.com/lib/pq
package. For MongoDB, use the gopkg.in/mgo.v2
package.
2. Configure the database connection in your Beego application's configuration file. For MySQL and PostgreSQL, specify the database driver, database name, username, password, and host. For MongoDB, specify the database driver, database name, and URL.
# MySQL configuration db.driver = mysql db.name = mydatabase db.user = myuser db.password = mypassword db.host = localhost # PostgreSQL configuration db.driver = postgres db.name = mydatabase db.user = myuser db.password = mypassword db.host = localhost # MongoDB configuration db.driver = mongodb db.name = mydatabase db.url = mongodb://localhost:27017
3. Import the required database driver package in your Beego application:
import ( _ "github.com/go-sql-driver/mysql" // MySQL driver _ "github.com/lib/pq" // PostgreSQL driver _ "gopkg.in/mgo.v2" // MongoDB driver )
4. Define your database models using Beego's ORM tags. These tags specify the table name, column names, and other database-related information.
type User struct { Id int Name string `orm:"column(username)"` Age int }
5. Use Beego's ORM functions to perform database operations like creating tables, inserting records, updating records, and querying records.
Here's an example of inserting a record into a MySQL database using Beego's ORM:
o := orm.NewOrm() user := User{Name: "John Doe", Age: 25} _, err := o.Insert(&user) if err != nil { // Handle error }
In this example, we create a new User
struct and insert it into the database using the Insert
function provided by Beego's ORM. We pass a pointer to the User
struct to the Insert
function.
Related Article: Building Gin Backends for React.js and Vue.js
Supported Databases for Beego's ORM: MySQL, PostgreSQL, and MongoDB
Beego's ORM supports multiple databases, including MySQL, PostgreSQL, and MongoDB. These databases are widely used in the industry and offer different features and capabilities.
To use MySQL with Beego's ORM, you'll need to install the MySQL driver for Go by running the following command:
go get github.com/go-sql-driver/mysql
To use PostgreSQL with Beego's ORM, you'll need to install the PostgreSQL driver for Go by running the following command:
go get github.com/lib/pq
To use MongoDB with Beego's ORM, you'll need to install the MongoDB driver for Go by running the following command:
go get gopkg.in/mgo.v2
Once you've installed the required database drivers, you can configure the database connection in your Beego application's configuration file, as mentioned in the previous section.
Beego's ORM provides a unified API for interacting with these databases, allowing you to write database-agnostic code. This means that you can switch between different databases without changing your application's code.
Additional Resources
- Beego GitHub Repository
- Bulma