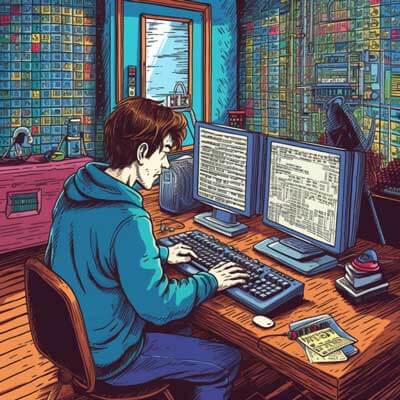
Table of Contents
Third-Party Services and Their Role in Enhancing React Applications
Third-party integrations play a crucial role in enhancing React applications by providing additional functionality, services, and libraries that can be easily integrated into the application. These integrations enable developers to extend the capabilities of their React applications without having to reinvent the wheel or build everything from scratch.
One of the key benefits of third-party integrations is the ability to leverage existing tools and services that are already well-established and widely used in the industry. This not only saves development time and effort but also ensures that the application benefits from the expertise and continuous improvement of these third-party providers.
Furthermore, third-party integrations can help address specific needs or requirements that are not directly supported by React itself. Whether it's integrating with a CSS framework for styling, integrating with a CMS for content management, or utilizing cloud functions and services, third-party integrations provide developers with a wide range of options to enhance their React applications.
Related Article: How To Convert A String To An Integer In Javascript
Exploring CSS Frameworks for Styling React Apps
CSS frameworks are a popular choice for styling React applications due to their pre-defined styles and components, which can significantly speed up the development process. One of the most widely used CSS frameworks is Bootstrap. Let's take a look at how we can integrate Bootstrap into a React application.
First, we need to install Bootstrap as a dependency in our project:
npm install bootstrap
Once installed, we can import the Bootstrap CSS file into our React component:
import 'bootstrap/dist/css/bootstrap.min.css';
Now, we can start using Bootstrap classes and components in our React component's JSX code. For example, we can use the container
class to create a responsive container:
import React from 'react';function App() { return ( <div className="container"> <h1>Hello, React!</h1> <button className="btn btn-primary">Click Me</button> </div> );}export default App;
Integrating CMS Systems with React for Content Management
Content Management Systems (CMS) are widely used for managing and organizing digital content. Integrating CMS systems with React allows developers to easily manage and update content without having to manually edit HTML or JSX files. Let's explore three popular CMS systems for React integration: Contentful, Sanity, and Strapi.
Understanding Contentful: A Useful CMS for React Integration
Contentful is a headless CMS that provides a flexible and scalable content infrastructure. It allows developers to create and manage content models using a web-based interface, and provides a useful API for retrieving and updating content programmatically.
To integrate Contentful with a React application, we first need to install the Contentful JavaScript SDK:
npm install contentful
Next, we can import the necessary modules and configure the SDK with our Contentful credentials:
import { createClient } from 'contentful';const client = createClient({ space: 'YOUR_SPACE_ID', accessToken: 'YOUR_ACCESS_TOKEN',});
Once the client is configured, we can make API calls to fetch content from Contentful and render it in our React components. For example, we can fetch and render a list of blog posts:
import React, { useEffect, useState } from 'react';function BlogList() { const [posts, setPosts] = useState([]); useEffect(() => { client.getEntries().then((response) => { setPosts(response.items); }); }, []); return ( <div> {posts.map((post) => ( <div key={post.sys.id}> <h2>{post.fields.title}</h2> <p>{post.fields.content}</p> </div> ))} </div> );}export default BlogList;
Enhancing React Apps with Sanity: A Headless CMS Solution
Sanity is another popular headless CMS that offers a flexible content modeling system and a real-time collaborative editing interface. It provides a fully customizable content API that can be easily integrated into React applications.
To integrate Sanity with a React application, we first need to install the Sanity client library:
npm install @sanity/client
Next, we can configure the Sanity client with our project's API credentials:
import sanityClient from '@sanity/client';const client = sanityClient({ projectId: 'YOUR_PROJECT_ID', dataset: 'YOUR_DATASET', apiVersion: 'YYYY-MM-DD', // Optional, defaults to latest token: 'YOUR_TOKEN', // Optional, if using private datasets useCdn: false, // Optional, use CDN for faster response times});
Once the client is configured, we can make API calls to fetch and update content in Sanity. For example, we can fetch and render a list of products:
import React, { useEffect, useState } from 'react';function ProductList() { const [products, setProducts] = useState([]); useEffect(() => { client.fetch('*[_type == "product"]').then((response) => { setProducts(response); }); }, []); return ( <div> {products.map((product) => ( <div key={product._id}> <h2>{product.title}</h2> <p>{product.description}</p> </div> ))} </div> );}export default ProductList;
Integrating Strapi with React for Dynamic Content Management
Strapi is a useful open-source headless CMS that provides developers with a highly customizable and extensible content management solution. It offers an easy-to-use admin panel for managing content and a RESTful API for retrieving and updating content programmatically.
To integrate Strapi with a React application, we can make HTTP requests to the Strapi API endpoints. For example, we can fetch and render a list of blog posts:
import React, { useEffect, useState } from 'react';function BlogList() { const [posts, setPosts] = useState([]); useEffect(() => { fetch('https://api.example.com/posts') .then((response) => response.json()) .then((data) => { setPosts(data); }); }, []); return ( <div> {posts.map((post) => ( <div key={post.id}> <h2>{post.title}</h2> <p>{post.content}</p> </div> ))} </div> );}export default BlogList;
Utilizing Cloud Functions in React Applications
Cloud functions provide a way to run server-side code in the cloud, enabling developers to offload computationally intensive or time-consuming tasks from the client-side to the server-side. This can greatly enhance the functionality and performance of React applications. Let's explore two popular cloud function solutions: AWS Lambda and Firebase.
An Introduction to AWS Lambda and Its Integration with React
AWS Lambda is a serverless computing service provided by Amazon Web Services. It allows developers to run code without provisioning or managing servers. AWS Lambda functions can be used to perform various tasks, such as processing data, performing calculations, or interacting with other AWS services.
To integrate AWS Lambda with a React application, we can create a Lambda function that performs a specific task and invoke it from our client-side code. For example, let's create a Lambda function that calculates the square of a given number:
1. Create a new Lambda function in the AWS Management Console and write the code to calculate the square:
exports.handler = async (event) => { const number = parseInt(event.number); const square = number * number; return { statusCode: 200, body: JSON.stringify({ square }), };};
2. Deploy the Lambda function and note down its ARN (Amazon Resource Name).
3. In our React component, we can make an HTTP request to the Lambda function using the AWS SDK for JavaScript:
import React, { useState } from 'react';import AWS from 'aws-sdk';AWS.config.update({ region: 'YOUR_REGION',});const lambda = new AWS.Lambda({ apiVersion: '2015-03-31' });function SquareCalculator() { const [number, setNumber] = useState(''); const [square, setSquare] = useState(''); const calculateSquare = () => { const params = { FunctionName: 'YOUR_LAMBDA_FUNCTION_NAME', Payload: JSON.stringify({ number }), }; lambda.invoke(params, (err, data) => { if (err) { console.error(err); } else { const { square } = JSON.parse(data.Payload); setSquare(square); } }); }; return ( <div> <input type="number" value={number} onChange={(e) => setNumber(e.target.value)} /> <button onClick={calculateSquare}>Calculate Square</button> <p>Square: {square}</p> </div> );}export default SquareCalculator;
Leveraging Firebase for Enhanced Functionality in React Apps
Firebase is a popular platform provided by Google that offers a suite of cloud-based services for building and scaling web and mobile applications. One of the key features of Firebase is its support for cloud functions, which allow developers to write server-side code that can be triggered by various events.
To integrate Firebase with a React application, we first need to set up a Firebase project and enable the cloud functions feature. Then, we can write and deploy cloud functions that interact with our React application.
For example, let's create a cloud function that sends a welcome email to a user when they sign up:
1. Set up a Firebase project and enable the cloud functions feature.
2. Write the code for the cloud function that sends the welcome email:
const functions = require('firebase-functions');const admin = require('firebase-admin');const nodemailer = require('nodemailer');admin.initializeApp();exports.sendWelcomeEmail = functions.auth.user().onCreate((user) => { const transporter = nodemailer.createTransport({ service: 'gmail', auth: { user: 'YOUR_EMAIL', pass: 'YOUR_PASSWORD', }, }); const mailOptions = { from: 'YOUR_EMAIL', to: user.email, subject: 'Welcome to our app!', text: 'Thank you for signing up. We hope you enjoy using our app!', }; return transporter.sendMail(mailOptions);});
3. Deploy the cloud function using the Firebase CLI:
firebase deploy --only functions
4. In our React component, we can call the cloud function when a user signs up:
import React, { useState } from 'react';import firebase from 'firebase/app';import 'firebase/auth';firebase.initializeApp({ // Firebase configuration});function SignupForm() { const [email, setEmail] = useState(''); const [password, setPassword] = useState(''); const handleSignup = () => { firebase .auth() .createUserWithEmailAndPassword(email, password) .then(() => { // Cloud function will be triggered and send the welcome email console.log('User signed up successfully!'); }) .catch((error) => { console.error(error); }); }; return ( <div> <input type="email" value={email} onChange={(e) => setEmail(e.target.value)} /> <input type="password" value={password} onChange={(e) => setPassword(e.target.value)} /> <button onClick={handleSignup}>Sign Up</button> </div> );}export default SignupForm;
Related Article: How to Add Navbar Components for Different Pages in ReactJS
Styling React Apps with Bootstrap and Other CSS Frameworks
Styling React applications can be a time-consuming task, especially when it comes to creating responsive layouts and styling individual components. CSS frameworks like Bootstrap provide a set of pre-designed components and styles that can be easily integrated into React applications, saving developers both time and effort.
Bootstrap is one of the most popular CSS frameworks and offers a wide range of components, such as buttons, forms, and navigation bars, as well as a responsive grid system. To style a React application with Bootstrap, we can follow these steps:
1. Install Bootstrap as a dependency in our project:
npm install bootstrap
2. Import the Bootstrap CSS file into our React component:
import 'bootstrap/dist/css/bootstrap.min.css';
3. Start using Bootstrap classes and components in our JSX code. For example, we can use the container
class to create a responsive container and the btn
class to style a button:
import React from 'react';function App() { return ( <div className="container"> <h1>Hello, React!</h1> <button className="btn btn-primary">Click Me</button> </div> );}export default App;
In addition to Bootstrap, there are other CSS frameworks available that can be used to style React applications. Some popular alternatives include:
- Tailwind CSS: A utility-first CSS framework that provides a set of utility classes for building custom designs.
- Material-UI: A React component library that implements the Material Design guidelines and offers a wide range of pre-designed components.
- Bulma: A lightweight CSS framework that focuses on simplicity and ease of use, providing a responsive grid system and a set of customizable components.
These frameworks can be installed and integrated into React applications in a similar manner to Bootstrap, providing developers with additional options for styling their applications.
Comparing Contentful, Sanity, and Strapi: CMS Solutions for React
Content Management Systems (CMS) play a crucial role in managing and organizing digital content in React applications. There are several CMS solutions available that can be integrated with React, each with its own set of features and capabilities. In this section, we will compare three popular CMS solutions: Contentful, Sanity, and Strapi.
Contentful
Contentful is a headless CMS that provides a flexible and scalable content infrastructure. It offers a web-based interface for creating and managing content models, as well as a useful API for retrieving and updating content programmatically.
Key features of Contentful include:
- Content modeling: Contentful allows developers to define content models using a flexible and intuitive interface. This makes it easy to organize and structure content according to specific requirements.
- Content delivery API: Contentful provides a useful API that allows developers to fetch content from the CMS and integrate it into their React applications. The API supports various query parameters and filtering options, making it easy to retrieve specific content.
- Webhooks and web app integrations: Contentful supports webhooks, which allow developers to trigger custom actions or workflows when specific events occur in the CMS. It also offers integrations with popular web apps and services, such as Slack and Salesforce.
Sanity
Sanity is a headless CMS that offers a flexible content modeling system and a real-time collaborative editing interface. It provides a fully customizable content API that can be easily integrated into React applications.
Key features of Sanity include:
- Real-time collaboration: Sanity allows multiple users to collaboratively edit content in real-time, making it ideal for teams working on content creation and management.
- Portable text field type: Sanity provides a portable text field type that supports rich text editing and can be used to create complex content structures, such as blog posts or product descriptions.
- Customizable content schemas: Sanity allows developers to define custom content schemas using JavaScript. This provides flexibility and control over the content structure and allows for easy updates and modifications.
Strapi
Strapi is an open-source headless CMS that provides developers with a highly customizable and extensible content management solution. It offers an easy-to-use admin panel for managing content and a RESTful API for retrieving and updating content programmatically.
Key features of Strapi include:
- Customizable content types: Strapi allows developers to define custom content types using a visual interface or by writing code. This provides flexibility and control over the content structure and allows for easy updates and modifications.
- Authentication and user management: Strapi provides built-in authentication and user management features, making it easy to secure content and manage user permissions.
- Plugin system: Strapi offers a plugin system that allows developers to extend the CMS's functionality and add custom features. This makes it possible to integrate Strapi with other services or customize the CMS to fit specific requirements.
Overall, Contentful, Sanity, and Strapi are all useful CMS solutions that can be integrated with React applications. The choice between them depends on factors such as the project requirements, the desired level of customization, and the specific features and capabilities needed.
Choosing the Right CMS System for React Integration
When choosing a CMS system for React integration, there are several factors to consider to ensure the best fit for your project. Here are some key factors to consider when making your decision:
1. Flexibility and customization: Consider the level of flexibility and customization offered by the CMS. Does it allow you to define custom content models and schemas? Can you easily modify and update the content structure as your project evolves?
2. Ease of use and user interface: Evaluate the user interface and ease of use of the CMS. Is the content management interface intuitive and easy to navigate? Does it provide features like real-time collaboration or a visual content editor?
3. API and integration capabilities: Examine the API and integration capabilities of the CMS. Does it provide a useful and well-documented API for fetching and updating content? Does it support webhooks or integrations with other services?
4. Performance and scalability: Consider the performance and scalability of the CMS. Does it have a reliable and fast infrastructure? Can it handle high traffic and large amounts of content?
5. Community and support: Assess the community and support around the CMS. Is there an active community of developers using the CMS? Are there resources and documentation available to help with implementation and troubleshooting?
6. Cost and pricing model: Evaluate the cost and pricing model of the CMS. Is it a subscription-based service? Does it offer different pricing tiers or plans? Consider the long-term cost implications of using the CMS.
Additional Resources
- 10 Popular React Components