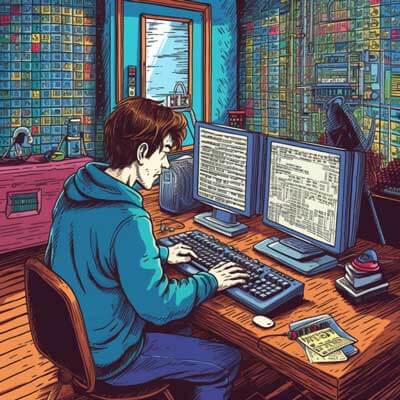
Table of Contents
Introduction
Real-time communication has become an essential feature in modern web applications. Users expect instant updates, live notifications, and dynamic content without the need for manual refreshing. To achieve this, web developers have turned to technologies like WebSockets to enable real-time communication between clients and servers. In this article, we will explore how to enhance real-time communication in Beego, a popular Go web framework, using WebSockets. We will delve into the inner workings of WebSockets, as well as other related concepts and technologies such as Goroutines, channels, HTTP/2, JSON-RPC, WebRTC, long polling, server-sent events, message queuing, and microservices architecture.
Related Article: Beego Integration with Bootstrap, Elasticsearch & Databases
How do Goroutines work?
Goroutines are a key feature of Go programming that allows concurrent execution of functions. They provide a lightweight and efficient mechanism for handling concurrent tasks in a Go program. Goroutines are not tied to the operating system threads, and the Go runtime manages their execution. This allows for easy scalability and efficient utilization of system resources.
To create a Goroutine, simply prefix a function call with the keyword "go". The function will then be executed concurrently in its own Goroutine. Here's an example:
package main import ( "fmt" "time" ) func main() { go printHello() time.Sleep(1 * time.Second) } func printHello() { fmt.Println("Hello, world!") }
In this example, the main function spawns a Goroutine to execute the printHello function concurrently. The sleep function is used to pause the main Goroutine for a second to allow the printHello Goroutine to complete its execution.
What are channels and how are they used?
Channels are a fundamental feature of Go that enable communication and synchronization between Goroutines. They are used to safely pass data between Goroutines without the need for explicit locks or condition variables.
A channel can be thought of as a conduit through which values can be sent and received. It has a specific type, which determines the type of values that can be sent and received on the channel. Channels are created using the built-in make function, and the <- operator is used to send and receive values on a channel.
Here's an example that demonstrates the usage of channels:
package main import "fmt" func main() { ch := make(chan int) go square(4, ch) result := <-ch fmt.Println(result) } func square(num int, ch chan int) { ch <- num * num }
In this example, the main function creates a channel of type int using the make function. It then spawns a Goroutine to calculate the square of the number 4 and send the result on the channel. The result is received from the channel and printed in the main Goroutine.
What is HTTP/2 and its relation to real-time communication?
HTTP/2 is the latest version of the Hypertext Transfer Protocol, which is widely used for transmitting data over the web. It is designed to improve the performance of web applications by introducing features such as multiplexing, header compression, and server push.
Real-time communication can benefit from the features of HTTP/2. Multiplexing allows multiple requests and responses to be sent and received concurrently over a single TCP connection, reducing latency and improving efficiency. Header compression reduces the overhead of transmitting HTTP headers, further improving performance. Server push enables the server to proactively send data to the client without waiting for a request, which is particularly useful for real-time updates.
To enable HTTP/2 in Beego, you need to configure the server to use the HTTP/2 protocol. Here's an example:
package main import ( "github.com/astaxie/beego" "github.com/astaxie/beego/plugins/cors" ) func main() { beego.BConfig.Listen.EnableHTTP2 = true beego.InsertFilter("*", beego.BeforeRouter, cors.Allow(&cors.Options{ AllowOrigins: []string{"*"}, AllowMethods: []string{"GET", "POST", "PUT", "DELETE", "OPTIONS"}, AllowHeaders: []string{"Origin", "Authorization", "Access-Control-Allow-Origin"}, ExposeHeaders: []string{"Content-Length", "Access-Control-Allow-Origin"}, AllowCredentials: true, })) beego.Run() }
In this example, the EnableHTTP2 field of the beego.BConfig struct is set to true to enable HTTP/2. The CORS plugin is also configured to allow cross-origin requests.
Related Article: Design Patterns with Beego & Golang
What is JSON-RPC and its use for real-time features?
JSON-RPC is a remote procedure call (RPC) protocol encoded in JSON. It allows a client to invoke methods on a remote server using a simple and lightweight message format. JSON-RPC is commonly used for real-time features as it provides an efficient way to communicate between clients and servers.
To implement real-time features using JSON-RPC in Beego, you can use the built-in support for JSON-RPC provided by the Beego framework. Here's an example:
package main import ( "github.com/astaxie/beego" "github.com/astaxie/beego/logs" ) type MathService struct {} func (m *MathService) Add(args *struct { A int `json:"a"` B int `json:"b"` }, result *int) error { *result = args.A + args.B return nil } func main() { beego.JSONRPCMapping("/math", &MathService{}) logs.SetLogger(logs.AdapterConsole) logs.EnableFuncCallDepth(true) beego.Run() }
In this example, a MathService struct is defined with an Add method that takes two integers as arguments and returns their sum. The beego.JSONRPCMapping function is used to map the MathService to the "/math" endpoint. The logs package is used to enable logging for debugging purposes.
What is WebRTC and its implementation in Golang?
WebRTC (Web Real-Time Communication) is a free, open-source project that enables real-time communication between web browsers and mobile applications. It provides a set of APIs and protocols for building real-time audio, video, and data applications.
In Golang, WebRTC can be implemented using the Pion WebRTC library. Pion is a pure Go implementation of the WebRTC API. It allows developers to create WebRTC applications entirely in Go, without the need for external dependencies.
Here's an example of using Pion to implement a simple video chat application:
package main import ( "github.com/pion/webrtc/v3" "github.com/pion/webrtc/v3/examples/internal/signal" "github.com/pion/webrtc/v3/pkg/media/ivfwriter" "github.com/pion/webrtc/v3/pkg/media/oggwriter" "github.com/pion/webrtc/v3/pkg/media/samplebuilder" "github.com/pion/webrtc/v3/pkg/media/oggreader" "github.com/pion/webrtc/v3/pkg/media/ivfreader" "github.com/pion/webrtc/v3/pkg/media/ivfwriter" ) func main() { // Implement your WebRTC application using Pion }
In this example, we import the necessary packages from the Pion WebRTC library to handle signaling, media formats, and building WebRTC applications. The main function is left empty as it is the starting point for implementing your WebRTC application.
What is long polling and how does it differ from WebSockets?
Long polling is a technique used in web development to achieve real-time communication between clients and servers. It involves the client sending a request to the server, and the server holding the request open until new data is available or a timeout occurs. Once new data is available, the server responds to the client with the updated information.
Long polling differs from WebSockets in that it uses the HTTP protocol and relies on the client repeatedly sending requests to the server. This can lead to increased network overhead and latency compared to WebSockets, which establish a persistent, bidirectional connection between the client and server.
Here's an example of implementing long polling in Beego:
package main import ( "github.com/astaxie/beego" "time" ) func main() { beego.Get("/updates", func(ctx *context.Context) { ch := make(chan string) go func() { time.Sleep(5 * time.Second) ch <- "New update!" }() select { case update := <-ch: ctx.WriteString(update) case <-time.After(10 * time.Second): ctx.WriteString("No updates") } }) beego.Run() }
In this example, a route is defined for "/updates" using the beego.Get function. When a client requests this route, a Goroutine is spawned to simulate a delay of 5 seconds before sending a new update on the channel. The select statement is used to wait for either the update or a timeout of 10 seconds. The response is then written to the client.
How do server-sent events work?
Server-sent events (SSE) is a technology that allows servers to send updates to clients over a single HTTP connection. It provides a unidirectional, persistent connection from the server to the client, enabling real-time updates without the need for polling or long-lived connections.
To implement server-sent events in Beego, you can use the built-in support for SSE provided by the Beego framework. Here's an example:
package main import ( "github.com/astaxie/beego" "github.com/astaxie/beego/context" "time" ) func main() { beego.Get("/updates", func(ctx *context.Context) { ctx.ResponseWriter.Header().Set("Content-Type", "text/event-stream") ctx.ResponseWriter.Header().Set("Cache-Control", "no-cache") ctx.ResponseWriter.Header().Set("Connection", "keep-alive") ctx.ResponseWriter.Header().Set("Access-Control-Allow-Origin", "*") for { ctx.WriteString("data: New update!\n\n") ctx.ResponseWriter.(http.Flusher).Flush() time.Sleep(5 * time.Second) } }) beego.Run() }
In this example, a route is defined for "/updates" using the beego.Get function. When a client requests this route, the response writer's headers are set to indicate that it is an SSE stream. The server then enters an infinite loop, continuously sending updates to the client every 5 seconds. The response writer's Flush method is called to ensure that the updates are immediately sent to the client.
Related Article: Optimizing and Benchmarking Beego ORM in Golang
Techniques for real-time data streaming
Real-time data streaming is a critical aspect of building applications that require instant updates and live data. There are several techniques and technologies available to achieve real-time data streaming in Beego:
1. WebSockets: WebSockets provide a bidirectional communication channel between the client and server, enabling real-time data streaming. The server can push updates to the client, and the client can send data to the server without the need for manual refreshing or repeated requests. WebSockets offer low latency and efficient data transfer.
2. Server-Sent Events (SSE): SSE is a unidirectional, persistent connection from the server to the client, allowing the server to send updates to the client over a single HTTP connection. SSE is well-suited for scenarios where the server needs to push updates to multiple clients simultaneously.
Here's an example of using SSE for real-time data streaming in Beego:
package main import ( "github.com/astaxie/beego" "github.com/astaxie/beego/context" "time" ) func main() { beego.Get("/stream", func(ctx *context.Context) { ctx.ResponseWriter.Header().Set("Content-Type", "text/event-stream") ctx.ResponseWriter.Header().Set("Cache-Control", "no-cache") ctx.ResponseWriter.Header().Set("Connection", "keep-alive") ctx.ResponseWriter.Header().Set("Access-Control-Allow-Origin", "*") for { ctx.WriteString("data: New update!\n\n") ctx.ResponseWriter.(http.Flusher).Flush() time.Sleep(5 * time.Second) } }) beego.Run() }
In this example, a route is defined for "/stream" using the beego.Get function. When a client requests this route, the response writer's headers are set to indicate that it is an SSE stream. The server then enters an infinite loop, continuously sending updates to the client every 5 seconds. The response writer's Flush method is called to ensure that the updates are immediately sent to the client.
3. Long Polling: Long polling is a technique where the client sends a request to the server and waits for a response. The server holds the request open until new data is available or a timeout occurs. Once new data is available, the server responds to the client with the updated information. Long polling can be an effective way to achieve real-time data streaming, although it may result in increased network overhead and latency compared to WebSockets.
4. Message Queuing: Message queuing systems like RabbitMQ or Apache Kafka can be utilized for real-time data streaming. They provide a robust and scalable way to distribute messages between different components of a system. Messages can be published to a broker and consumed by multiple clients or services, enabling real-time updates and data synchronization.
What is message queuing and its use for real-time communication?
Message queuing is a method of asynchronous communication between different components of a distributed system. It involves the use of a message broker, which acts as an intermediary for sending and receiving messages. Messages are placed into a queue by the sender and are then retrieved from the queue by the receiver.
Message queuing is commonly used for real-time communication as it provides a reliable and scalable way to distribute messages between different components. It enables decoupling of components, allowing them to communicate asynchronously without being directly dependent on each other. This is particularly useful in scenarios where real-time updates and data synchronization are required.
In Beego, you can use message queuing systems like RabbitMQ or Apache Kafka to implement real-time communication. These systems provide libraries and APIs that allow you to send and receive messages between components of your Beego application.
Here's an example of using RabbitMQ for real-time communication in Beego:
package main import ( "github.com/astaxie/beego" "github.com/streadway/amqp" ) func main() { conn, err := amqp.Dial("amqp://guest:guest@localhost:5672/") if err != nil { panic(err) } defer conn.Close() ch, err := conn.Channel() if err != nil { panic(err) } defer ch.Close() q, err := ch.QueueDeclare( "my_queue", // queue name false, // durable false, // delete when unused false, // exclusive false, // no-wait nil, // arguments ) if err != nil { panic(err) } // Send a message to the queue err = ch.Publish( "", // exchange q.Name, // routing key false, // mandatory false, // immediate amqp.Publishing{ ContentType: "text/plain", Body: []byte("Hello, RabbitMQ!"), }, ) if err != nil { panic(err) } beego.Run() }
In this example, we connect to a RabbitMQ server using the amqp.Dial function. We then create a channel and declare a queue using the ch.QueueDeclare function. Finally, we publish a message to the queue using the ch.Publish function.
Leveraging microservices architecture for real-time features
Microservices architecture is an architectural style that structures an application as a collection of loosely coupled services. Each service is responsible for a specific business capability and can be developed, deployed, and scaled independently. Microservices architecture provides several benefits for real-time features:
1. Scalability: With microservices, you can scale individual services independently based on their specific resource requirements. This allows you to handle the increased load of real-time communication without impacting other parts of the system.
2. Flexibility: Microservices architecture allows you to choose the most appropriate technology stack for each service. This means you can select the best-suited technology for implementing real-time features, such as WebSockets, SSE, or message queuing, without being limited by a monolithic architecture.
3. Resilience: Microservices architecture promotes fault isolation, meaning that failures in one service do not affect the overall system. This is crucial for real-time features, as any downtime or failures in the communication layer can result in a poor user experience.
4. Agility: Microservices architecture enables faster development and deployment cycles. This allows you to quickly iterate and improve real-time features based on user feedback, ensuring that you can deliver the most up-to-date and responsive user experience.
To leverage microservices architecture for real-time features in Beego, you can decompose your application into smaller, independent services that handle different aspects of real-time communication. Each service can be developed and deployed independently, allowing for faster iteration and scaling. Additionally, you can use inter-service communication mechanisms, such as message queuing or HTTP APIs, to enable real-time communication between services.