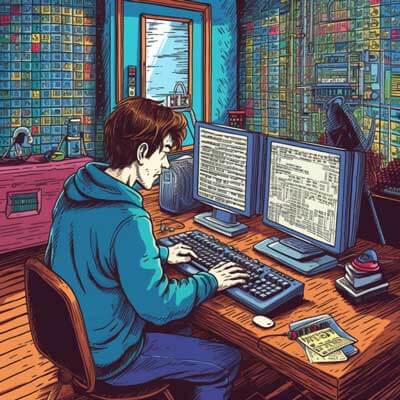
Table of Contents
Authentication
Authentication is the process of verifying the identity of a user or a system. It ensures that the user or system accessing a particular resource is indeed who they claim to be. In the context of enterprise applications, authentication is a critical functionality that allows users to securely access various resources and perform authorized actions.
In Python Flask, there are several ways to implement authentication. One popular approach is to use Single Sign-On (SSO) solutions like Flask-OAuthlib and Flask-OIDC. These libraries provide support for implementing authentication using OAuth and OpenID Connect protocols, which are widely used in enterprise applications.
Related Article: Python Data Types & Data Modeling
Implementing Single Sign-On (SSO) using Flask-OAuthlib or Flask-OIDC
Flask-OAuthlib and Flask-OIDC are two popular Flask extensions that provide support for implementing Single Sign-On (SSO) using OAuth and OpenID Connect protocols, respectively. These protocols allow users to authenticate themselves with a third-party identity provider (IdP) and then use the obtained authentication token to access protected resources in your Flask application.
Here's an example of how to implement SSO using Flask-OAuthlib:
from flask import Flask from flask_oauthlib.client import OAuth app = Flask(__name__) app.secret_key = 'your_secret_key' oauth = OAuth(app) oauth_provider = oauth.remote_app( 'provider_name', consumer_key='your_consumer_key', consumer_secret='your_consumer_secret', request_token_params={'scope': 'email'}, base_url='https://provider.com/api/', request_token_url=None, access_token_method='POST', access_token_url='https://provider.com/oauth/access_token', authorize_url='https://provider.com/oauth/authorize' ) @app.route('/login') def login(): return oauth_provider.authorize(callback='http://yourapp.com/callback') @app.route('/callback') def callback(): resp = oauth_provider.authorized_response() if resp is None or isinstance(resp, OAuthException): # Handle authentication failure return 'Authentication failed' else: # Store the access token and perform further actions access_token = resp['access_token'] # Access protected resources using the access token return 'Successfully authenticated' if __name__ == '__main__': app.run()
In this example, we create an instance of the OAuth
class and configure it with the necessary parameters for the OAuth provider. We define the /login
route, which redirects the user to the OAuth provider's authorization page. After successful authentication, the user is redirected to the /callback
route, where we can obtain the access token and perform further actions.
Integration with enterprise identity providers and Active Directory
Enterprise applications often need to integrate with existing identity providers, such as Active Directory, to leverage the existing user base and authentication mechanisms. Flask provides various extensions and libraries to facilitate this integration.
One common approach is to use Lightweight Directory Access Protocol (LDAP) for enterprise identity provider integration. Flask-LDAP3 is a Flask extension that provides a convenient way to interact with LDAP servers. With Flask-LDAP3, you can authenticate users against an LDAP server, retrieve user attributes, and perform other LDAP operations.
Here's an example of how to integrate Flask with LDAP for enterprise identity provider integration:
from flask import Flask from flask_ldap3_login import LDAP3LoginManager app = Flask(__name__) app.secret_key = 'your_secret_key' ldap_manager = LDAP3LoginManager(app) ldap_manager.init_config({ 'LDAP_HOST': 'ldap://your_ldap_server', 'LDAP_PORT': 389, 'LDAP_BASE_DN': 'dc=example,dc=com', 'LDAP_USER_DN': 'ou=users', 'LDAP_USER_LOGIN_ATTR': 'username', 'LDAP_ALWAYS_BIND': True }) @app.route('/login') def login(): if ldap_manager.authenticate(): # Successful authentication return 'Successfully authenticated' else: # Authentication failed return 'Authentication failed' if __name__ == '__main__': app.run()
In this example, we create an instance of the LDAP3LoginManager
class and configure it with the necessary parameters for the LDAP server. We define the /login
route, where we call the authenticate()
method to perform the LDAP authentication. If the authentication is successful, we return a success message; otherwise, we return an authentication failure message.
Another common integration in enterprise applications is with Active Directory Federation Services (ADFS). Flask-SSO is a Flask extension that provides support for integrating Flask applications with ADFS. With Flask-SSO, you can authenticate users against an ADFS server and obtain user attributes for further processing.
Authorization
Authorization is the process of granting or denying access to specific resources or actions based on the authenticated user's privileges. It ensures that users can only access the resources they are authorized to access. In enterprise applications, authorization is a crucial functionality that helps enforce security policies and protect sensitive information.
Flask provides several mechanisms for implementing authorization in your applications. One common approach is to use role-based access control (RBAC), where users are assigned roles, and permissions are associated with these roles. Flask-Principal is a Flask extension that provides support for implementing RBAC and managing user roles and permissions.
Related Article: Python Keywords Identifiers: Tutorial and Examples
Implementing Role-Based Access Control in Flask
Flask-Principal simplifies the implementation of role-based access control in Flask applications. It provides decorators and context managers to specify the required roles or permissions for accessing specific routes or resources.
Here's an example of how to implement role-based access control in Flask using Flask-Principal:
from flask import Flask, render_template from flask_principal import Principal, Identity, AnonymousIdentity, Permission, RoleNeed app = Flask(__name__) app.secret_key = 'your_secret_key' principal = Principal(app) @app.route('/') def index(): return 'Welcome to the homepage' @app.route('/admin') @Permission(RoleNeed('admin')) def admin(): return 'Welcome to the admin panel' if __name__ == '__main__': app.run()
In this example, we create an instance of the Principal
class and initialize it with the Flask application. We define the /
route for the homepage and the /admin
route for the admin panel. We use the @Permission
decorator to associate the admin
role with the /admin
route.
When a user tries to access the /admin
route, Flask-Principal checks if the user has the admin
role. If the user has the role, the access is granted; otherwise, the user is redirected to a forbidden page or an error message.
User Management
User management is an essential functionality of enterprise applications that involves creating, updating, and deleting user accounts, as well as managing user roles, permissions, and authentication credentials. Flask provides various extensions and libraries to simplify user management in your applications.
Implementing User Management in Flask with Python
Flask-User is a Flask extension that provides a complete user management solution for Flask applications. It allows you to easily add user registration, login, and profile management features to your application.
Here's an example of how to implement user management in Flask using Flask-User:
from flask import Flask from flask_sqlalchemy import SQLAlchemy from flask_user import UserManager, UserMixin app = Flask(__name__) app.secret_key = 'your_secret_key' app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///your_database.db' db = SQLAlchemy(app) class User(db.Model, UserMixin): id = db.Column(db.Integer, primary_key=True) email = db.Column(db.String(120), unique=True) password = db.Column(db.String(120)) db.create_all() user_manager = UserManager(app, db, User) if __name__ == '__main__': app.run()
In this example, we create a User
model class that inherits from db.Model
and UserMixin
. We define the necessary attributes for user management, such as email
and password
. We create the necessary database tables using db.create_all()
.
We initialize the UserManager
with the Flask application, the SQLAlchemy database, and the User
model. Flask-User automatically adds routes for user registration, login, and profile management. It also provides various user management features, such as password hashing, email confirmation, and password reset.
Token-Based Authentication
Token-based authentication is a popular approach for securing APIs and web applications. It involves issuing a token to the user after successful authentication, which the user includes in subsequent requests to access protected resources. Flask provides several extensions and libraries for implementing token-based authentication in your applications.
Related Article: How to Use Python Import Math GCD
Understanding Token-Based Authentication and Its Working
Token-based authentication works by issuing a token to the user after successful authentication. The token is typically a long string of characters that is generated based on the user's credentials. The user includes this token in subsequent requests as an authorization header or as a query parameter to access protected resources.
Token-based authentication has several advantages over traditional session-based authentication. It eliminates the need for server-side session storage, allows for stateless API authentication, and enables cross-domain requests.
Here's an example of how to implement token-based authentication in Flask:
from flask import Flask, request from flask_jwt_extended import JWTManager, create_access_token, jwt_required, get_jwt_identity app = Flask(__name__) app.secret_key = 'your_secret_key' jwt = JWTManager(app) @app.route('/login', methods=['POST']) def login(): username = request.json.get('username') password = request.json.get('password') if username == 'admin' and password == 'password': access_token = create_access_token(identity=username) return {'access_token': access_token} else: return {'error': 'Invalid credentials'}, 401 @app.route('/protected', methods=['GET']) @jwt_required def protected(): current_user = get_jwt_identity() return {'message': f'Hello, {current_user}'} if __name__ == '__main__': app.run()
In this example, we use the Flask-JWT-Extended
extension to implement token-based authentication. We define the /login
route, where we authenticate the user's credentials and generate an access token using create_access_token()
. We return the access token to the user.
We define the /protected
route, which requires a valid access token. We use the @jwt_required
decorator to enforce token-based authentication. The get_jwt_identity()
function retrieves the current user's identity from the access token.
Authentication Protocols
Authentication protocols provide a standardized way for applications to authenticate users and obtain authorization to access protected resources. Flask provides support for various authentication protocols commonly used in enterprise applications, such as OAuth, OpenID Connect, and LDAP.
Commonly Used Authentication Protocols in Enterprise Applications
- OAuth: OAuth is an open standard for authorization that allows users to grant third-party applications access to their resources without sharing their credentials. It is widely used in enterprise applications for delegating access to protected resources.
- OpenID Connect: OpenID Connect is an authentication layer built on top of OAuth 2.0. It provides a standardized way for users to authenticate with an identity provider and obtain identity information in the form of JSON Web Tokens (JWTs). OpenID Connect is commonly used in enterprise applications for single sign-on (SSO) and user authentication.
- LDAP: Lightweight Directory Access Protocol (LDAP) is a protocol for accessing and maintaining directory services. It is commonly used in enterprise applications for user authentication, authorization, and directory management. LDAP provides a hierarchical structure for organizing user and group information and supports various authentication mechanisms.
OAuth and SSO Implementation with Flask-OAuthlib
Flask-OAuthlib is a Flask extension that provides support for implementing OAuth 1.0 and OAuth 2.0 authentication in your Flask applications. It simplifies the process of integrating with OAuth providers and allows you to easily implement single sign-on (SSO) functionality.
Here's an example of how to implement OAuth and SSO with Flask-OAuthlib:
from flask import Flask, redirect, url_for from flask_oauthlib.client import OAuth app = Flask(__name__) app.secret_key = 'your_secret_key' oauth = OAuth(app) oauth_provider = oauth.remote_app( 'provider_name', consumer_key='your_consumer_key', consumer_secret='your_consumer_secret', request_token_params={'scope': 'email'}, base_url='https://provider.com/api/', request_token_url=None, access_token_method='POST', access_token_url='https://provider.com/oauth/access_token', authorize_url='https://provider.com/oauth/authorize' ) @app.route('/login') def login(): return oauth_provider.authorize(callback=url_for('callback', _external=True)) @app.route('/callback') def callback(): resp = oauth_provider.authorized_response() if resp is None or isinstance(resp, OAuthException): # Handle authentication failure return 'Authentication failed' else: # Store the access token and perform further actions access_token = resp['access_token'] # Access protected resources using the access token return 'Successfully authenticated' if __name__ == '__main__': app.run()
In this example, we create an instance of the OAuth
class and configure it with the necessary parameters for the OAuth provider. We define the /login
route, which redirects the user to the OAuth provider's authorization page. After successful authentication, the user is redirected to the /callback
route, where we can obtain the access token and perform further actions.
Related Article: How to Create Multiline Comments in Python
OpenID Connect and SSO Implementation with Flask-OIDC
Flask-OIDC is a Flask extension that provides support for implementing OpenID Connect authentication in your Flask applications. It simplifies the process of integrating with OpenID Connect providers and allows you to easily implement single sign-on (SSO) functionality.
Here's an example of how to implement OpenID Connect and SSO with Flask-OIDC:
from flask import Flask, redirect, url_for from flask_oidc import OpenIDConnect app = Flask(__name__) app.secret_key = 'your_secret_key' oidc = OpenIDConnect(app) @app.route('/login') @oidc.require_login def login(): return redirect(url_for('protected')) @app.route('/protected') @oidc.require_login def protected(): return f'Hello, {oidc.user_getfield("preferred_username")}' if __name__ == '__main__': app.run()
In this example, we create an instance of the OpenIDConnect
class and initialize it with the Flask application. We define the /login
route, where we use the @oidc.require_login
decorator to enforce OpenID Connect authentication. If the user is not authenticated, they are redirected to the OpenID Connect provider's login page.
We define the /protected
route, which also requires OpenID Connect authentication. The oidc.user_getfield()
function retrieves user information from the OpenID Connect provider's response.
Integrating Flask with LDAP for Enterprise Identity Provider Integration
LDAP (Lightweight Directory Access Protocol) is a protocol for accessing and maintaining directory services. It is commonly used in enterprise applications for user authentication, authorization, and directory management. Flask-LDAP3 is a Flask extension that provides a convenient way to interact with LDAP servers.
Here's an example of how to integrate Flask with LDAP for enterprise identity provider integration:
from flask import Flask from flask_ldap3_login import LDAP3LoginManager app = Flask(__name__) app.secret_key = 'your_secret_key' ldap_manager = LDAP3LoginManager(app) ldap_manager.init_config({ 'LDAP_HOST': 'ldap://your_ldap_server', 'LDAP_PORT': 389, 'LDAP_BASE_DN': 'dc=example,dc=com', 'LDAP_USER_DN': 'ou=users', 'LDAP_USER_LOGIN_ATTR': 'username', 'LDAP_ALWAYS_BIND': True }) @app.route('/login') def login(): if ldap_manager.authenticate(): # Successful authentication return 'Successfully authenticated' else: # Authentication failed return 'Authentication failed' if __name__ == '__main__': app.run()
In this example, we create an instance of the LDAP3LoginManager
class and configure it with the necessary parameters for the LDAP server. We define the /login
route, where we call the authenticate()
method to perform the LDAP authentication. If the authentication is successful, we return a success message; otherwise, we return an authentication failure message.
Active Directory Federation Services Integration with Flask
Active Directory Federation Services (ADFS) is a component of Microsoft's Active Directory that provides single sign-on (SSO) capabilities across different applications and systems. Flask-SSO is a Flask extension that provides support for integrating Flask applications with ADFS.
Here's an example of how to integrate Flask with Active Directory Federation Services (ADFS) using Flask-SSO:
from flask import Flask, redirect, url_for from flask_sso import FlaskSSO app = Flask(__name__) app.secret_key = 'your_secret_key' sso = FlaskSSO(app) @app.route('/login') @sso.login_required def login(): return redirect(url_for('protected')) @app.route('/protected') @sso.login_required def protected(): return f'Hello, {sso.user.username}' if __name__ == '__main__': app.run()
In this example, we create an instance of the FlaskSSO
class and initialize it with the Flask application. We define the /login
route, where we use the @sso.login_required
decorator to enforce ADFS authentication. If the user is not authenticated, they are redirected to the ADFS login page.
We define the /protected
route, which also requires ADFS authentication. The sso.user
object provides access to user information obtained from ADFS.
Difference Between Authentication and Authorization
Authentication and authorization are two distinct concepts in the context of security and access control.
Authentication is the process of verifying the identity of a user or a system. It ensures that the user or system accessing a particular resource is indeed who they claim to be. Authentication typically involves presenting credentials, such as a username and password, to prove identity. It can also involve more advanced methods, such as biometric authentication or multi-factor authentication.
Authorization, on the other hand, is the process of granting or denying access to specific resources or actions based on the authenticated user's privileges. It determines what a user is allowed to do and what resources they can access. Authorization is typically based on roles, permissions, or access control lists (ACLs) associated with the user. It ensures that users can only access the resources they are authorized to access.
Related Article: How to Use Reduction with Python
Audit Logging and Implementation in Flask
Audit logging is the process of recording and monitoring events in a system to ensure accountability, traceability, and compliance. It involves capturing and storing relevant information about user activities, system actions, and security events. Flask provides various extensions and libraries to implement audit logging in your applications.
Audit Logging and Implementation in Flask
Flask-Login is a Flask extension that provides user session management and authentication functionalities. It simplifies the process of managing user sessions and provides hooks for implementing audit logging.
Here's an example of how to implement audit logging in Flask using Flask-Login:
from flask import Flask, request, redirect, url_for from flask_login import LoginManager, UserMixin, login_required, login_user, logout_user app = Flask(__name__) app.secret_key = 'your_secret_key' login_manager = LoginManager(app) class User(UserMixin): def __init__(self, id): self.id = id @login_manager.user_loader def load_user(user_id): return User(user_id) @app.route('/login', methods=['GET', 'POST']) def login(): if request.method == 'POST': user_id = request.form['user_id'] password = request.form['password'] if user_id == 'admin' and password == 'password': user = User(user_id) login_user(user) # Log successful login event return redirect(url_for('protected')) else: # Log failed login event return 'Invalid credentials' return ''' <br> <br> ''' @app.route('/protected') @login_required def protected(): # Log protected resource access event return 'Protected resource' @app.route('/logout') @login_required def logout(): # Log logout event logout_user() return redirect(url_for('login')) if __name__ == '__main__': app.run()
In this example, we use the Flask-Login
extension to manage user sessions and authentication. We define a User
class that inherits from UserMixin
to represent a user. We implement the load_user
function to load a user object based on the user ID.
We define the /login
route, where we handle the login form submission. If the credentials are valid, we create a user object and use login_user()
to log the user in. We log the successful login event and redirect the user to the protected resource. If the credentials are invalid, we log the failed login event and display an error message.
We define the /protected
route, which requires the user to be logged in. We log the access to the protected resource event.
We define the /logout
route, where we log the logout event and log the user out using logout_user()
.
Additional Resources
- Flask-OAuthlib Documentation