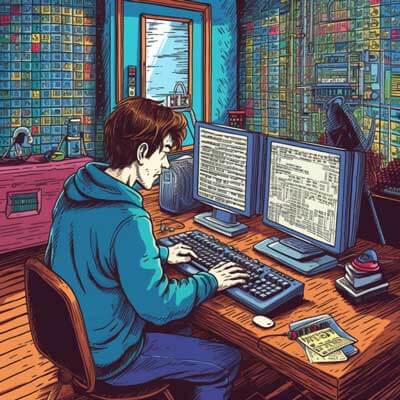
Table of Contents
API Integration
API integration is the process of combining two or more separate APIs to create a unified functionality. In the context of FastAPI, API integration refers to the ability to call one API from another API. This allows developers to leverage existing APIs and build more complex and useful applications.
To execute API calls within FastAPI, you can use the requests
library, which is a popular HTTP library for Python. It allows you to send HTTP requests and handle the responses easily.
Here's an example of how you can integrate an external API into your FastAPI application:
import requests from fastapi import FastAPI app = FastAPI() @app.get("/pokemon/{name}") async def get_pokemon(name: str): # Call the PokeAPI to get information about a specific Pokemon response = requests.get(f"https://pokeapi.co/api/v2/pokemon/{name}") if response.status_code == 200: pokemon_data = response.json() return pokemon_data else: return {"message": "Pokemon not found"}
In this example, we define a route /pokemon/{name}
that takes a name
parameter representing the name of a Pokemon. We then make an API call to the PokeAPI using the requests.get()
method, passing in the URL of the API endpoint. We handle the response and return the data if the API call is successful, or return an error message if the Pokemon is not found.
Related Article: Tutorial: i18n in FastAPI with Pydantic & Handling Encoding
API Chaining
API chaining refers to the process of calling multiple APIs in a sequential manner, where the output of one API call serves as the input for the next API call. This allows developers to build a chain of API calls to achieve a specific goal or retrieve complex data.
Here's an example of how you can chain multiple API calls within FastAPI:
import requests from fastapi import FastAPI app = FastAPI() @app.get("/weather/{city}") async def get_weather(city: str): # Call the OpenWeatherMap API to get the current weather of a specific city weather_response = requests.get(f"https://api.openweathermap.org/data/2.5/weather?q={city}&appid=YOUR_API_KEY") if weather_response.status_code == 200: weather_data = weather_response.json() # Extract the latitude and longitude from the weather data lat = weather_data["coord"]["lat"] lon = weather_data["coord"]["lon"] # Call the OpenCage Geocoding API to get the address of the city based on the latitude and longitude geocoding_response = requests.get(f"https://api.opencagedata.com/geocode/v1/json?q={lat}+{lon}&key=YOUR_API_KEY") if geocoding_response.status_code == 200: geocoding_data = geocoding_response.json() address = geocoding_data["results"][0]["formatted"] return {"city": city, "weather": weather_data["weather"][0]["description"], "address": address} else: return {"message": "Geocoding API call failed"} else: return {"message": "Weather API call failed"}
In this example, we define a route /weather/{city}
that takes a city
parameter representing the name of a city. We first make an API call to the OpenWeatherMap API to get the current weather of the city. We then extract the latitude and longitude from the weather data and use them to make another API call to the OpenCage Geocoding API to get the address of the city. Finally, we return the weather data and the address as the response.
API Composition
API composition involves combining multiple APIs to create a new API that provides a higher-level functionality. It allows developers to create APIs that are tailored to specific use cases or business requirements by aggregating and transforming data from multiple sources.
In FastAPI, you can compose APIs by defining new routes that call multiple existing APIs and combine their responses. This can be done using the requests
library to make the API calls and manipulating the data as needed.
Here's an example of how you can compose APIs in FastAPI:
import requests from fastapi import FastAPI app = FastAPI() @app.get("/pokemon/{name}/description") async def get_pokemon_description(name: str): # Call the PokeAPI to get information about a specific Pokemon response = requests.get(f"https://pokeapi.co/api/v2/pokemon/{name}") if response.status_code == 200: pokemon_data = response.json() # Extract the species URL from the Pokemon data species_url = pokemon_data["species"]["url"] # Call the species API to get the species data of the Pokemon species_response = requests.get(species_url) if species_response.status_code == 200: species_data = species_response.json() # Extract the flavor text from the species data flavor_text_entries = species_data["flavor_text_entries"] flavor_text = "" for entry in flavor_text_entries: if entry["language"]["name"] == "en": flavor_text = entry["flavor_text"] break return {"pokemon": name, "description": flavor_text} else: return {"message": "Species API call failed"} else: return {"message": "Pokemon API call failed"}
In this example, we define a route /pokemon/{name}/description
that takes a name
parameter representing the name of a Pokemon. We first make an API call to the PokeAPI to get information about the Pokemon. We then extract the species URL from the Pokemon data and make another API call to the species API to get the species data. Finally, we extract the flavor text from the species data and return it as the response.
API Aggregation
API aggregation involves combining the responses from multiple APIs into a single response. It allows developers to retrieve data from multiple sources and present it in a unified format.
To aggregate API responses in FastAPI, you can use the requests
library to make the API calls and combine the data as needed.
Here's an example of how you can aggregate API responses in FastAPI:
import requests from fastapi import FastAPI app = FastAPI() @app.get("/pokemon/{name}/details") async def get_pokemon_details(name: str): # Call the PokeAPI to get information about a specific Pokemon pokemon_response = requests.get(f"https://pokeapi.co/api/v2/pokemon/{name}") if pokemon_response.status_code == 200: pokemon_data = pokemon_response.json() # Call the PokeAPI to get the species data of the Pokemon species_response = requests.get(pokemon_data["species"]["url"]) if species_response.status_code == 200: species_data = species_response.json() # Call the PokeAPI to get the evolution chain data of the Pokemon evolution_response = requests.get(species_data["evolution_chain"]["url"]) if evolution_response.status_code == 200: evolution_data = evolution_response.json() return {"pokemon": pokemon_data["name"], "height": pokemon_data["height"], "weight": pokemon_data["weight"], "evolution_chain": evolution_data} else: return {"message": "Evolution API call failed"} else: return {"message": "Species API call failed"} else: return {"message": "Pokemon API call failed"}
In this example, we define a route /pokemon/{name}/details
that takes a name
parameter representing the name of a Pokemon. We first make an API call to the PokeAPI to get information about the Pokemon. We then extract the species URL from the Pokemon data and make another API call to get the species data. Finally, we extract the evolution chain URL from the species data and make another API call to get the evolution chain data. We combine all the data and return it as the response.
Related Article: FastAPI Integration: Bootstrap Templates, Elasticsearch and Databases
Nested API Calls
Nested API calls involve making an API call within the response handler of another API call. It allows developers to retrieve additional data or perform additional actions based on the response of the initial API call.
In FastAPI, you can make nested API calls by invoking the requests
library within the response handler of another API call.
Here's an example of how you can make nested API calls in FastAPI:
import requests from fastapi import FastAPI app = FastAPI() @app.get("/pokemon/{name}/species") async def get_pokemon_species(name: str): # Call the PokeAPI to get information about a specific Pokemon pokemon_response = requests.get(f"https://pokeapi.co/api/v2/pokemon/{name}") if pokemon_response.status_code == 200: pokemon_data = pokemon_response.json() # Make a nested API call to the PokeAPI to get the species data of the Pokemon species_response = requests.get(pokemon_data["species"]["url"]) if species_response.status_code == 200: species_data = species_response.json() return {"pokemon": pokemon_data["name"], "species": species_data["name"]} else: return {"message": "Species API call failed"} else: return {"message": "Pokemon API call failed"}
In this example, we define a route /pokemon/{name}/species
that takes a name
parameter representing the name of a Pokemon. We first make an API call to the PokeAPI to get information about the Pokemon. Within the response handler of the initial API call, we make a nested API call to get the species data of the Pokemon. We combine the data from both API calls and return it as the response.
API Cascading
API cascading involves making API calls in a cascading manner, where the output of one API call serves as the input for the next API call. It allows developers to retrieve data from multiple APIs in a sequential manner, building on the results of the previous API calls.
To cascade API calls in FastAPI, you can use the requests
library to make the API calls and process the responses accordingly.
Here's an example of how you can cascade API calls in FastAPI:
import requests from fastapi import FastAPI app = FastAPI() @app.get("/pokemon/{name}/evolution") async def get_pokemon_evolution(name: str): # Call the PokeAPI to get information about a specific Pokemon pokemon_response = requests.get(f"https://pokeapi.co/api/v2/pokemon/{name}") if pokemon_response.status_code == 200: pokemon_data = pokemon_response.json() # Make a cascading API call to the PokeAPI to get the evolution chain data of the Pokemon species_url = pokemon_data["species"]["url"] species_response = requests.get(species_url) if species_response.status_code == 200: species_data = species_response.json() evolution_url = species_data["evolution_chain"]["url"] evolution_response = requests.get(evolution_url) if evolution_response.status_code == 200: evolution_data = evolution_response.json() return {"pokemon": pokemon_data["name"], "evolution_chain": evolution_data} else: return {"message": "Evolution API call failed"} else: return {"message": "Species API call failed"} else: return {"message": "Pokemon API call failed"}
In this example, we define a route /pokemon/{name}/evolution
that takes a name
parameter representing the name of a Pokemon. We first make an API call to the PokeAPI to get information about the Pokemon. Within the response handler of the initial API call, we make a cascading API call to get the evolution chain data of the Pokemon. We combine the data from all the API calls and return it as the response.
API Orchestration
API orchestration involves coordinating and executing multiple API calls in a specific order or sequence to achieve a larger goal or perform a complex task. It allows developers to control the flow of API calls and handle dependencies between them.
In FastAPI, you can orchestrate API calls by defining routes that call multiple APIs and coordinate their execution. This can be done using the requests
library to make the API calls and handle the responses accordingly.
Here's an example of how you can orchestrate multiple API calls in FastAPI:
import requests from fastapi import FastAPI app = FastAPI() @app.get("/pokemon/{name}/details") async def get_pokemon_details(name: str): # Call the PokeAPI to get information about a specific Pokemon pokemon_response = requests.get(f"https://pokeapi.co/api/v2/pokemon/{name}") if pokemon_response.status_code == 200: pokemon_data = pokemon_response.json() # Make a cascading API call to the PokeAPI to get the species data of the Pokemon species_url = pokemon_data["species"]["url"] species_response = requests.get(species_url) if species_response.status_code == 200: species_data = species_response.json() # Make a nested API call to the PokeAPI to get the evolution chain data of the Pokemon evolution_url = species_data["evolution_chain"]["url"] evolution_response = requests.get(evolution_url) if evolution_response.status_code == 200: evolution_data = evolution_response.json() # Make an API call to the PokeAPI to get the abilities data of the Pokemon abilities_urls = [ability["ability"]["url"] for ability in pokemon_data["abilities"]] abilities_data = [] for url in abilities_urls: ability_response = requests.get(url) if ability_response.status_code == 200: ability_data = ability_response.json() abilities_data.append(ability_data) return {"pokemon": pokemon_data["name"], "evolution_chain": evolution_data, "abilities": abilities_data} else: return {"message": "Evolution API call failed"} else: return {"message": "Species API call failed"} else: return {"message": "Pokemon API call failed"}
In this example, we define a route /pokemon/{name}/details
that takes a name
parameter representing the name of a Pokemon. We first make an API call to the PokeAPI to get information about the Pokemon. Within the response handler of the initial API call, we make a cascading API call to get the species data of the Pokemon. We then make a nested API call to get the evolution chain data of the Pokemon. Finally, we make an API call for each ability of the Pokemon to get the abilities data. We combine all the data and return it as the response.
API Forwarding
API forwarding involves receiving an API request and forwarding it to another API endpoint without modifying or processing the request. It allows developers to create proxy-like functionality where the FastAPI application acts as an intermediary between the client and the target API.
In FastAPI, you can forward API requests by using the requests
library to send the request to the target API endpoint and returning the response as is.
Here's an example of how you can forward API requests in FastAPI:
import requests from fastapi import FastAPI, Request app = FastAPI() @app.get("/forward") async def forward_request(request: Request): # Get the URL query parameters from the request query_params = request.query_params # Get the target URL from the query parameters target_url = query_params.get("url") if target_url: # Forward the request to the target URL response = requests.get(target_url) # Return the response from the target API return response.json() else: return {"message": "Target URL not provided"}
In this example, we define a route /forward
that receives a request object representing the incoming API request. We extract the target URL from the query parameters of the request and use the requests.get()
method to forward the request to the target API endpoint. We return the response from the target API as the response of the FastAPI application.
Related Article: FastAPI Enterprise Basics: SSO, RBAC, and Auditing
API Proxying
API proxying involves receiving an API request and forwarding it to another API endpoint while potentially modifying or processing the request and response. It allows developers to create a layer of abstraction between the client and the target API, enabling additional functionality such as request/response transformation, caching, rate limiting, and more.
To implement API proxying in FastAPI, you can use the requests
library to send requests to the target API endpoint and manipulate the request and response as needed.
Here's an example of how you can implement API proxying in FastAPI:
import requests from fastapi import FastAPI, Request app = FastAPI() @app.get("/proxy") async def proxy_request(request: Request): # Get the URL query parameters from the request query_params = request.query_params # Get the target URL from the query parameters target_url = query_params.get("url") if target_url: # Modify the request, if needed headers = dict(request.headers) headers["X-Custom-Header"] = "Custom Value" # Forward the modified request to the target URL response = requests.get(target_url, headers=headers) # Modify the response, if needed response.headers["X-Custom-Header"] = "Custom Value" # Return the modified response return response.json() else: return {"message": "Target URL not provided"}
In this example, we define a route /proxy
that receives a request object representing the incoming API request. We extract the target URL from the query parameters of the request and modify the request headers by adding a custom header. We then use the requests.get()
method to forward the modified request to the target API endpoint. After receiving the response from the target API, we modify the response headers by adding a custom header. We return the modified response as the response of the FastAPI application.
API Tunneling
API tunneling involves establishing a secure and encrypted connection between the client and the target API endpoint by encapsulating API requests within another protocol. It allows developers to bypass network restrictions, firewall rules, or other limitations that may prevent direct access to the target API.
To implement API tunneling in FastAPI, you can use tools or methods such as ngrok, localtunnel, or ngrok.io to create a tunnel between the client and the target API endpoint.
Here's an example of how you can implement API tunneling in FastAPI using ngrok:
from fastapi import FastAPI app = FastAPI() @app.get("/hello") async def hello(): return {"message": "Hello, world!"}
In this example, we define a route /hello
that returns a simple JSON response with a greeting message.
To create a tunnel using ngrok, you can follow these steps:
1. Download ngrok from the official website (https://ngrok.com/) and install it on your system.
2. Run the following command in your terminal to start a tunnel to your FastAPI application:
ngrok http 8000
3. Once the tunnel is established, ngrok will provide you with a public URL that you can use to access your FastAPI application from anywhere.
4. Test the tunnel by accessing the /hello
route using the ngrok URL. For example, if the ngrok URL is http://12345678.ngrok.io
, you can access the /hello
route by visiting http://12345678.ngrok.io/hello
.
Best Practices for Calling an API from Another API
When calling an API from another API, it's important to follow some best practices to ensure the reliability, performance, and security of your application. Here are some best practices to consider:
1. Use Asynchronous Requests: When making API calls from FastAPI, consider using asynchronous requests instead of synchronous requests. Asynchronous requests allow your application to handle multiple API calls concurrently, improving the overall performance and responsiveness of your application.
2. Handle Errors and Failures: When calling an API from another API, ensure that you handle errors and failures gracefully. Implement proper error handling mechanisms to handle scenarios such as API timeouts, network errors, and invalid responses. This will help prevent your application from crashing or returning incorrect data in case of API failures.
3. Implement Caching: If the API you are calling has data that is relatively static or changes infrequently, consider implementing caching in your application. Caching can significantly improve the performance of your application by reducing the number of API calls and the associated latency.
4. Implement Rate Limiting: To prevent abuse and ensure fair usage of the APIs you are calling, consider implementing rate limiting in your application. Rate limiting helps control the number of API requests made within a specific time period and protects both your application and the target API from excessive traffic.
5. Secure API Calls: When making API calls from FastAPI, ensure that you use secure protocols such as HTTPS to protect the data being transmitted. If the target API requires authentication, handle the authentication process securely and store any access tokens or credentials securely.
6. Monitor API Performance: Monitor the performance of the APIs you are calling to identify any bottlenecks or issues. This can help you optimize your application and ensure that your API calls are efficient and performant.
7. Use API Documentation: Always refer to the documentation provided by the API provider before making API calls. The documentation will provide information on the API endpoints, request/response formats, authentication requirements, rate limits, and any other relevant details.
Following these best practices will help you build robust, performant, and secure applications when calling APIs from FastAPI.
Libraries or Frameworks for API Aggregation in FastAPI
There are several libraries and frameworks available for API aggregation in FastAPI. These libraries and frameworks provide higher-level abstractions and features for aggregating data from multiple APIs and composing complex workflows.
Here are some popular libraries and frameworks for API aggregation in FastAPI:
1. GraphQL: GraphQL is a query language and runtime for APIs that allows you to define the shape and structure of the data you need. It provides a flexible and efficient way to retrieve data from multiple APIs in a single request. There are several Python libraries available for integrating GraphQL with FastAPI, such as graphene and Ariadne.
2. aiohttp: aiohttp is an asynchronous HTTP client/server library for Python that provides a high-level API for making HTTP requests. It supports both client-side and server-side functionality, making it suitable for API aggregation in FastAPI. With aiohttp, you can easily make concurrent API calls and handle the responses asynchronously.
3. HTTPX: HTTPX is a fully featured HTTP client for Python that provides a simple and intuitive API for making HTTP requests. It supports both synchronous and asynchronous requests, making it suitable for API aggregation in FastAPI. HTTPX offers features such as connection pooling, request streaming, HTTP/2 support, and more.
4. Pydantic: Pydantic is a data validation and parsing library for Python that provides a declarative syntax for defining data models. It integrates seamlessly with FastAPI and can be used to validate and parse API responses from multiple APIs. Pydantic supports automatic validation, serialization, and deserialization of data based on the defined models.
5. FastAPI-utils: FastAPI-utils is a collection of utilities and tools for FastAPI that includes features for API composition and aggregation. It provides decorators and functions for composing routes, handling dependencies, and more. FastAPI-utils simplifies the process of building complex APIs in FastAPI by providing higher-level abstractions and utilities.
These libraries and frameworks can greatly simplify the process of API aggregation in FastAPI by providing high-level abstractions, utilities, and features. Choose the one that best fits your needs and requirements for your specific use case.
Related Article: Handling Large Volumes of Data in FastAPI
Tools or Methods for Proxying APIs in FastAPI
Proxying APIs in FastAPI involves receiving an API request, modifying or processing the request, forwarding it to another API endpoint, and potentially modifying or processing the response before returning it to the client. It allows you to create a layer of abstraction between the client and the target API, enabling additional functionality such as request/response transformation, caching, rate limiting, and more.
To proxy APIs in FastAPI, you can use tools or methods such as reverse proxies, API gateways, or libraries/frameworks that provide proxying functionality.
Here are some popular tools and methods for proxying APIs in FastAPI:
1. Nginx: Nginx is a high-performance web server and reverse proxy server that can be used to proxy API requests in FastAPI. It provides useful proxying capabilities, load balancing, caching, SSL/TLS termination, and more. You can configure Nginx to proxy requests to FastAPI application servers, handle SSL/TLS encryption, and perform request/response transformation.
2. Traefik: Traefik is a modern reverse proxy and load balancer that supports dynamic configuration and automatic discovery of services. It can be used to proxy API requests in FastAPI and provides features such as SSL/TLS termination, load balancing, circuit breaking, request/response transformation, and more. Traefik integrates seamlessly with containerized environments and cloud platforms.
3. FastAPI-Proxy: FastAPI-Proxy is a library specifically designed for proxying APIs in FastAPI. It provides a declarative syntax for defining proxy routes and allows you to easily modify or process the request and response using middleware functions. FastAPI-Proxy supports features such as request/response transformation, rate limiting, caching, authentication, and more.
4. Envoy: Envoy is a high-performance, extensible, and cloud-native proxy server that can be used to proxy API requests in FastAPI. It provides advanced features such as load balancing, circuit breaking, rate limiting, health checking, request/response transformation, and more. Envoy can be used as a standalone proxy server or as part of a service mesh architecture.
These tools and methods provide useful capabilities for proxying APIs in FastAPI and enable you to build scalable, performant, and secure API architectures.
Techniques for Tunneling API Requests in FastAPI
Tunneling API requests in FastAPI involves establishing a secure and encrypted connection between the client and the target API endpoint by encapsulating API requests within another protocol. It allows you to bypass network restrictions, firewall rules, or other limitations that may prevent direct access to the target API.
To tunnel API requests in FastAPI, you can use tools or methods such as ngrok, localtunnel, or VPNs.
Here's an example of how you can tunnel API requests in FastAPI using ngrok:
1. Download ngrok from the official website (https://ngrok.com/) and install it on your system.
2. Run the following command in your terminal to start a tunnel to your FastAPI application:
ngrok http 8000
3. Once the tunnel is established, ngrok will provide you with a public URL that you can use to access your FastAPI application from anywhere.
4. Test the tunnel by accessing your FastAPI routes using the ngrok URL. For example, if the ngrok URL is http://12345678.ngrok.io
, you can access your FastAPI routes by visiting http://12345678.ngrok.io/your-route
.
Additional Resources
- FastAPI documentation - Passing data between API calls