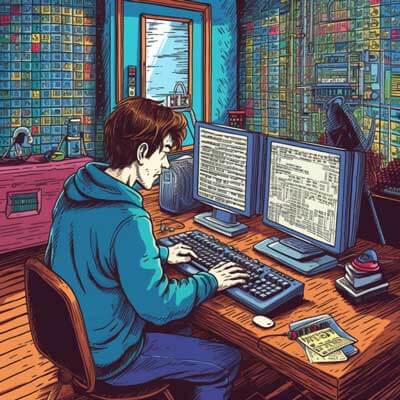
Table of Contents
Overview of Fetch Calls in ReactJS
Fetch is a built-in web API in JavaScript that allows making network requests to retrieve resources from a server. It is commonly used in ReactJS applications to fetch data from APIs and render it on the user interface. Fetch calls in ReactJS are asynchronous, meaning they do not block the execution of other code while waiting for a response.
To make a fetch call in ReactJS, you need to use the fetch()
function. This function takes a URL as an argument and returns a Promise that resolves to the response from the server. The response contains information such as the status code, headers, and the actual data.
Here's an example of making a simple fetch call in ReactJS:
fetch('https://api.example.com/data') .then(response => response.json()) .then(data => { // Do something with the data }) .catch(error => { // Handle any errors });
In this example, we make a GET request to the https://api.example.com/data
endpoint. We chain the .json()
method to the response to parse the response body as JSON. Finally, we handle the parsed data in the second .then()
block and catch any errors in the .catch()
block.
Related Article: How to Implement Hover State in ReactJS with Inline Styles
Fetching Multiple Requests in ReactJS
There may be scenarios where you need to fetch multiple requests in ReactJS. For example, you might need to fetch data from multiple APIs or fetch data from different endpoints of the same API. In such cases, you can use the Promise.all()
method to fetch multiple requests in parallel.
The Promise.all()
method takes an array of Promises as an argument and returns a new Promise that resolves when all the promises in the array have resolved. This allows you to wait for all the fetch requests to complete before proceeding with further processing.
Here's an example of fetching multiple requests in ReactJS using Promise.all()
:
const fetchRequest1 = fetch('https://api.example.com/data1'); const fetchRequest2 = fetch('https://api.example.com/data2'); Promise.all([fetchRequest1, fetchRequest2]) .then(responses => { const [response1, response2] = responses; // Process the responses }) .catch(error => { // Handle any errors });
In this example, we create two fetch requests, fetchRequest1
and fetchRequest2
, for different endpoints. We pass these requests as an array to the Promise.all()
method. The resulting Promise resolves to an array of responses, which we can destructure and process accordingly.
Combining Fetch Calls
Sometimes, you may need to combine the results of multiple fetch calls in ReactJS. For example, you might want to fetch data from one endpoint and use that data to fetch data from another endpoint. In such cases, you can chain multiple fetch calls using the .then()
method.
Here's an example of combining fetch calls in ReactJS:
fetch('https://api.example.com/data1') .then(response1 => { // Process response1 return fetch('https://api.example.com/data2'); }) .then(response2 => { // Process response2 }) .catch(error => { // Handle any errors });
In this example, we first fetch data from the https://api.example.com/data1
endpoint. Once we have the response from data1
, we can process it and then make another fetch call to the https://api.example.com/data2
endpoint. We handle the second response in the next .then()
block.
Merging Fetch Calls
Merging fetch calls in ReactJS refers to combining the responses of multiple fetch calls into a single response. This can be useful when you want to aggregate data from different endpoints into a single object or when you want to perform some operations on the combined data.
To merge fetch calls in ReactJS, you can use the Promise.all()
method along with the Array.map()
method to fetch data from multiple endpoints and merge the responses.
Here's an example of merging fetch calls in ReactJS:
const endpoints = ['https://api.example.com/data1', 'https://api.example.com/data2']; const fetchPromises = endpoints.map(endpoint => fetch(endpoint)); Promise.all(fetchPromises) .then(responses => Promise.all(responses.map(response => response.json()))) .then(data => { // Merge and process the data }) .catch(error => { // Handle any errors });
In this example, we have an array of endpoints that we want to fetch data from. We use the Array.map()
method to create an array of fetch promises. We then pass this array to the Promise.all()
method to fetch data from all the endpoints simultaneously. Finally, we use another Promise.all()
to parse the response bodies as JSON and merge the data.
Related Article: How to to Deploy a ReactJS Application to Production
Making Multiple Fetch Requests
Making multiple fetch requests in ReactJS can be achieved by using a loop or an array of URLs. This approach allows you to dynamically generate fetch requests based on the number of URLs or the content of an array.
Here's an example of making multiple fetch requests in ReactJS:
const urls = ['https://api.example.com/data1', 'https://api.example.com/data2']; const fetchPromises = urls.map(url => fetch(url)); Promise.all(fetchPromises) .then(responses => Promise.all(responses.map(response => response.json()))) .then(data => { // Process the data }) .catch(error => { // Handle any errors });
In this example, we have an array of URLs that we want to fetch data from. We use the Array.map()
method to create an array of fetch promises by iterating over the URLs. We then pass this array of promises to the Promise.all()
method to fetch data from all the URLs simultaneously. Finally, we use another Promise.all()
to parse the response bodies as JSON and process the data.
Calling Multiple Fetch Requests in One Call
Calling multiple fetch requests in one call in ReactJS can be achieved by using the Promise.all()
method to fetch data from multiple endpoints simultaneously. This allows you to make multiple fetch requests in a single call and wait for all the responses to be received before further processing.
Here's an example of calling multiple fetch requests in one call in ReactJS:
const endpoints = ['https://api.example.com/data1', 'https://api.example.com/data2']; const fetchPromises = endpoints.map(endpoint => fetch(endpoint)); Promise.all(fetchPromises) .then(responses => { // Process the responses }) .catch(error => { // Handle any errors });
In this example, we have an array of endpoints that we want to fetch data from. We use the Array.map()
method to create an array of fetch promises. We then pass this array to the Promise.all()
method to fetch data from all the endpoints simultaneously. The resulting Promise resolves to an array of responses, which we can process accordingly.
Best Practices for Combining Fetch Calls
When combining fetch calls in ReactJS, there are a few best practices to keep in mind:
1. Use the Promise.all()
method to fetch data from multiple endpoints simultaneously. This allows you to make efficient use of network resources and reduces the overall time required to fetch the data.
2. Handle errors appropriately by using the .catch()
block to catch any errors that may occur during the fetch calls. You can display error messages to the user or take other appropriate actions based on the specific requirements of your application.
3. Use appropriate error handling and error messages to provide meaningful feedback to the user. This can help in troubleshooting and resolving any issues that may occur during the fetch calls.
4. Consider using async/await syntax for cleaner and more readable code. Async/await allows you to write asynchronous code in a synchronous manner, making it easier to understand and maintain.
5. Modularize your code by separating fetch calls into separate functions or components. This improves code readability and maintainability, as well as allows for easier testing and debugging.
Additional Resources
- Best practices for managing state in React