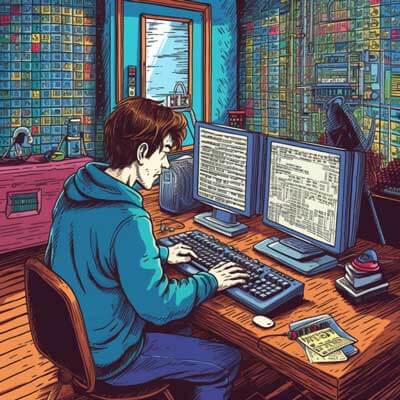
Table of Contents
What is Golang?
Golang, also known as Go, is an open-source programming language developed by Google. It was designed to be simple, efficient, and scalable, making it an ideal choice for building large-scale, high-performance applications. Go combines the ease of use of interpreted languages with the performance of compiled languages, making it a popular choice for systems programming, web development, and cloud computing.
Go's key features include:
- Strong typing: Go is statically typed, meaning that variables must be declared with their specific types. This helps catch errors at compile-time and improves code reliability.
- Garbage collection: Go has an efficient garbage collector that automatically manages memory allocation and deallocation. This relieves developers from the burden of manual memory management.
- Concurrency: Go has built-in support for concurrent programming, making it easy to write efficient and scalable concurrent applications. Goroutines, lightweight threads, and channels are the key constructs used for concurrency in Go.
- Extensive standard library: Go comes with a rich standard library that provides a wide range of functionalities, including networking, file I/O, cryptography, and more. This allows developers to build robust applications without relying on third-party libraries.
Related Article: Beego Integration with Bootstrap, Elasticsearch & Databases
What is Beego?
Beego is a useful and flexible web framework for building web applications and APIs in Go. It follows the model-view-controller (MVC) architectural pattern and provides a comprehensive set of features and tools to streamline the development process. Beego aims to simplify the development of web applications by providing a clear structure and conventions, allowing developers to focus on writing application logic rather than dealing with repetitive tasks.
Some key features of Beego include:
- Routing: Beego provides a flexible and easy-to-use routing mechanism, allowing developers to define URL patterns and map them to specific controller actions.
- ORM (Object-Relational Mapping): Beego's built-in ORM simplifies database operations by providing a high-level interface for interacting with databases. It supports a wide range of database drivers and provides features like automatic table creation, query generation, and transaction management.
- Middleware: Beego allows developers to define custom middleware functions that can be used to intercept and modify requests and responses. This provides a convenient way to implement cross-cutting concerns such as authentication, logging, and error handling.
- Templating: Beego includes a useful templating engine that allows developers to generate dynamic HTML content. It supports features like template inheritance, variable substitution, and control structures.
How does Beego's ORM work?
Beego's ORM (Object-Relational Mapping) provides a high-level interface for working with databases in Go. It simplifies database operations by abstracting away the complexities of SQL queries and providing a more intuitive API for interacting with the database.
Under the hood, Beego's ORM uses a combination of reflection and code generation to map Go structs to database tables. It automatically generates SQL queries based on the struct definition and executes them against the database.
To use Beego's ORM, you need to define a struct that represents a database table. Each field in the struct corresponds to a column in the table. You can use struct tags to specify additional information such as column names, data types, and primary keys.
Here's an example of a simple struct definition for a user table:
type User struct { Id int Username string `orm:"size(100)"` Email string `orm:"size(100)"` }
To perform database operations, you can use Beego's ORM methods such as Insert
, Read
, Update
, and Delete
. These methods generate the necessary SQL queries based on the struct definition and execute them against the database.
Here's an example of inserting a new user into the database using Beego's ORM:
user := User{ Username: "john", Email: "john@example.com", } orm := beego.NewOrm() _, err := orm.Insert(&user) if err != nil { // handle error }
Beego's ORM also provides advanced query techniques that allow you to perform complex queries using a fluent API. These techniques include filtering, ordering, joining tables, and aggregating data.
Advanced Query Techniques
Beego's ORM provides several advanced query techniques that allow you to perform complex queries using a fluent API. These techniques include filtering, ordering, joining tables, and aggregating data.
Here are some examples of advanced query techniques in Beego's ORM:
- Filtering: You can use the Filter
method to apply filters to your query. The Filter
method takes a field name and a value, and it generates a SQL condition that filters the records based on the specified field and value.
orm.QueryTable("user").Filter("username__contains", "john").All(&users)
- Ordering: You can use the OrderBy
method to specify the order in which the records should be returned. The OrderBy
method takes a list of field names and generates a SQL clause that orders the records based on the specified fields.
orm.QueryTable("user").OrderBy("username", "-email").All(&users)
- Joining tables: You can use the RelatedSel
method to join related tables in your query. The RelatedSel
method takes a field name and generates a SQL join clause that joins the specified related table.
orm.QueryTable("user").RelatedSel("profile").All(&users)
- Aggregating data: You can use the Sum
, Count
, Max
, and Min
methods to perform aggregations on your query. These methods generate SQL clauses that calculate the sum, count, maximum, and minimum values of the specified field.
var count int64 orm.QueryTable("user").Count(&count)
These advanced query techniques allow you to perform complex database operations using Beego's ORM in a simple and concise way.
Related Article: Golang Tutorial for Backend Development
How to use custom middleware in Beego?
Middleware is a useful concept in web development that allows you to intercept and modify requests and responses before they reach the main application logic. Beego provides a flexible and easy-to-use middleware mechanism that allows you to define custom middleware functions and apply them to specific routes or the entire application.
To use custom middleware in Beego, you need to define a function that takes http.ResponseWriter
and *http.Request
as parameters. This function will be called for every request that matches the specified route. Inside the middleware function, you can perform any custom logic and modify the request or response as needed.
Here's an example of a simple middleware function that logs the request URL and duration:
func LoggerMiddleware(ctx *context.Context) { start := time.Now() // Call the next middleware or controller ctx.Next() duration := time.Since(start) beego.Info(ctx.Request.URL.Path, " - ", duration) }
To apply the middleware to a specific route, you can use the Filter
method of the beego.Router
object:
beego.Router("/users", &controllers.UserController{}, "get:GetAllUsers;post:CreateUser").Filter("before", LoggerMiddleware)
In this example, the LoggerMiddleware
function will be called before the GetAllUsers
and CreateUser
methods of the UserController
are executed.
You can also apply the middleware to the entire application by using the beego.InsertFilter
function:
beego.InsertFilter("*", beego.BeforeRouter, LoggerMiddleware)
This will apply the LoggerMiddleware
function to all routes in the application.
Beego also provides a set of built-in middleware functions that you can use out of the box. These include authentication middleware, session middleware, CSRF protection middleware, and more. You can find more information about these built-in middleware functions in the Beego documentation.
What are filters in Beego?
In Beego, filters are a way to intercept and modify requests and responses at different stages of the request processing pipeline. Filters provide a convenient mechanism for implementing cross-cutting concerns such as authentication, authorization, logging, and error handling.
Filters in Beego are similar to middleware, but they provide more fine-grained control over the request processing pipeline. Filters can be applied to specific routes or the entire application, and they can be executed before or after the main controller logic.
To define a filter in Beego, you need to implement the beego.Filter
interface. The beego.Filter
interface defines two methods: BeforeExec
and AfterExec
. The BeforeExec
method is called before the main controller logic is executed, and the AfterExec
method is called after the main controller logic is executed.
Here's an example of a simple filter that logs the request URL and duration:
type LoggerFilter struct { beego.Filter } func (f *LoggerFilter) BeforeExec(ctx *context.Context) { start := time.Now() ctx.Input.SetData("start", start) } func (f *LoggerFilter) AfterExec(ctx *context.Context) { start := ctx.Input.GetData("start").(time.Time) duration := time.Since(start) beego.Info(ctx.Request.URL.Path, " - ", duration) }
To apply the filter to a specific route, you can use the Filter
method of the beego.Router
object:
beego.Router("/users", &controllers.UserController{}, "get:GetAllUsers;post:CreateUser").Filter(&LoggerFilter{})
In this example, the LoggerFilter
filter will be applied to the GetAllUsers
and CreateUser
methods of the UserController
.
You can also apply the filter to the entire application by using the beego.InsertFilter
function:
beego.InsertFilter("*", beego.BeforeRouter, &LoggerFilter{})
This will apply the LoggerFilter
filter to all routes in the application.
Filters in Beego provide a useful mechanism for implementing cross-cutting concerns in a modular and reusable way.
How does Beego handle routing?
Routing is a fundamental aspect of any web framework, including Beego. Beego provides a flexible and useful routing mechanism that allows you to define URL patterns and map them to specific controller actions.
In Beego, routing is typically defined in the routers
package of your application. The routers
package contains a set of functions that define the URL patterns and map them to the appropriate controller actions.
Here's an example of a simple routing configuration in Beego:
package routers import ( "github.com/astaxie/beego" "yourapp/controllers" ) func init() { beego.Router("/", &controllers.MainController{}) beego.Router("/users", &controllers.UserController{}, "get:GetAllUsers") beego.Router("/users/:id:int", &controllers.UserController{}, "get:GetUserById") beego.Router("/users", &controllers.UserController{}, "post:CreateUser") }
In this example, we define three routes:
- The root URL ("/") is mapped to the MainController
and the default Get
method.
- The "/users" URL is mapped to the UserController
and the GetAllUsers
method.
- The "/users/:id:int" URL is mapped to the UserController
and the GetUserById
method. The ":id" parameter is specified as an integer.
In addition to mapping URLs to controller actions, Beego also supports regular expressions in URL patterns and parameter matching. This allows you to define more complex URL patterns and extract parameters from the URL.
Here's an example of a routing configuration that uses regular expressions and parameter matching:
beego.Router("/users/:id(\\d+)", &controllers.UserController{}, "get:GetUserById")
In this example, the ":id" parameter is specified as a regular expression that matches one or more digits.
Beego also supports HTTP method-based routing, allowing you to map different HTTP methods (GET, POST, PUT, DELETE, etc.) to different controller actions.
beego.Router("/users", &controllers.UserController{}, "get:GetAllUsers;post:CreateUser")
In this example, the "GET" method is mapped to the GetAllUsers
method of the UserController
, and the "POST" method is mapped to the CreateUser
method.
Beego's routing mechanism provides a flexible and intuitive way to define URL patterns and map them to controller actions, allowing you to build robust and scalable web applications.
What are advanced routing techniques in Beego?
In addition to the basic routing capabilities, Beego provides several advanced routing techniques that allow you to handle more complex URL patterns and customize the routing behavior.
Related Article: Golang & Gin Security: JWT Auth, Middleware, and Cryptography
Custom URL Patterns
Beego allows you to define custom URL patterns using regular expressions. This gives you the flexibility to handle dynamic URLs and extract parameters from the URL.
Here's an example of a custom URL pattern:
beego.Router("/users/:id(\\d+)", &controllers.UserController{}, "get:GetUserById")
In this example, the ":id" parameter is specified as a regular expression that matches one or more digits. This allows you to handle URLs like "/users/123" and extract the "123" as a parameter in the GetUserById
method of the UserController
.
Namespace Routing
Beego supports namespace routing, which allows you to group related routes under a common prefix. This is useful for organizing your routes and avoiding conflicts between different parts of your application.
Here's an example of namespace routing:
ns := beego.NewNamespace("/api", beego.NSNamespace("/v1", beego.NSRouter("/users", &controllers.UserController{}, "get:GetAllUsers"), beego.NSRouter("/users/:id:int", &controllers.UserController{}, "get:GetUserById"), ), beego.NSNamespace("/v2", beego.NSRouter("/users", &controllers.UserController{}, "get:GetAllUsersV2"), beego.NSRouter("/users/:id:int", &controllers.UserController{}, "get:GetUserByIdV2"), ), ) beego.AddNamespace(ns)
In this example, we define two namespaces ("/api/v1" and "/api/v2") and group related routes under each namespace. This allows us to have separate versions of the "/users" route with different controller actions.
Auto Router
Beego provides an auto router feature that allows you to automatically generate routes based on the controller structure. This can be useful for quickly setting up routes for CRUD operations.
Here's an example of using the auto router:
beego.AutoRouter(&controllers.UserController{})
In this example, Beego will automatically generate routes for all the methods in the UserController
that follow the naming convention of "Verb" + "Resource". For example, the method GetAllUsers
will be mapped to the "/users" URL with the "GET" method.
These advanced routing techniques in Beego give you more flexibility and control over your application's URL patterns, allowing you to build complex and customizable routing structures.
How does Beego handle parameter handling?
Parameter handling is a critical aspect of web development, as it allows you to pass data to your application from the client side. Beego provides a convenient and flexible way to handle parameters in URLs, forms, and query strings.
Related Article: Intergrating Payment, Voice and Text with Gin & Golang
URL Parameters
In Beego, you can define URL parameters by specifying them in the route pattern. URL parameters are denoted by a colon followed by the parameter name.
Here's an example of defining a route with a URL parameter:
beego.Router("/users/:id", &controllers.UserController{}, "get:GetUserById")
In this example, the ":id" parameter is defined in the route pattern. When a request is made to "/users/123", Beego will automatically extract the value "123" and pass it as a parameter to the GetUserById
method of the UserController
.
You can also specify the data type of the URL parameter by appending the type to the parameter name. For example, ":id:int" specifies that the parameter is an integer.
Form Parameters
Beego provides a convenient way to handle form parameters submitted via HTTP POST requests. When a POST request is made with form data, Beego automatically parses the form data and maps it to the corresponding fields in the controller's struct.
Here's an example of handling form parameters in Beego:
type UserController struct { beego.Controller } func (c *UserController) CreateUser() { username := c.GetString("username") email := c.GetString("email") // handle form data }
In this example, the GetString
method is used to retrieve the values of the "username" and "email" form fields. Beego automatically parses the form data and makes it available via the GetString
method.
Query Parameters
Query parameters are parameters passed in the URL query string. Beego provides a simple and convenient way to handle query parameters in your controllers.
Here's an example of handling query parameters in Beego:
type UserController struct { beego.Controller } func (c *UserController) GetAllUsers() { page, _ := c.GetInt("page") limit, _ := c.GetInt("limit") // handle query parameters }
In this example, the GetInt
method is used to retrieve the values of the "page" and "limit" query parameters. Beego automatically parses the query string and makes it available via the GetInt
method.
Best practices for parameter handling in Beego
When handling parameters in Beego, it's important to follow best practices to ensure the security and reliability of your application. Here are some best practices for parameter handling in Beego:
- Validate input: Always validate input parameters to ensure that they meet your application's requirements. Beego provides validation functions such as IsEmail
, IsNumeric
, and IsURL
that you can use to validate input parameters.
username := c.GetString("username") if !beego.Valid.IsEmail(username) { // handle invalid email address }
- Sanitize input: Sanitize input parameters to prevent malicious code injection and other security vulnerabilities. Beego provides functions such as Html2str
and Htmlquote
that you can use to sanitize input parameters.
content := c.GetString("content") sanitizedContent := beego.Htmlquote(content)
- Use type conversion functions: Beego provides type conversion functions such as GetInt
, GetInt64
, GetFloat
, and GetBool
that you can use to convert parameter values to the desired types. Always use the appropriate type conversion function to avoid type-related errors.
page, _ := c.GetInt("page")
- Use default values: Beego provides functions such as GetString
, GetInt
, and GetBool
that allow you to specify a default value to be returned if the parameter is not found or is invalid. This can help prevent unexpected errors and improve the user experience.
limit := c.GetString("limit", "10")
- Secure sensitive information: If your application handles sensitive information such as passwords or API keys, make sure to handle them securely. Avoid storing sensitive information in plain text or exposing them in URLs or forms.
- Follow RESTful principles: When designing your API, follow RESTful principles to ensure a consistent and intuitive parameter handling mechanism. Use HTTP methods and URL patterns to represent different resources and actions.