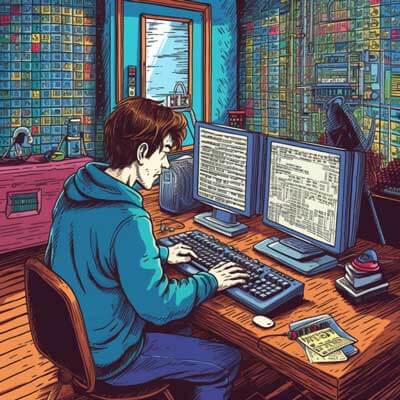
Table of Contents
Introduction
Redis has long been a popular choice for data caching and fast retrieval. However, as the complexity and scale of modern applications continue to grow, it's important to explore alternative data stores that can better meet the specific needs of your project. In this practical guide, we will discuss different alternatives to Redis, their use cases, performance considerations, implementation best practices, real-world examples, error handling techniques, advanced techniques, and provide code snippet ideas to help you evaluate and utilize these alternatives effectively.
Related Article: Tutorial on installing and using redis-cli in Redis
Use Cases for Alternative Data Stores
Alternative data stores can be valuable in various scenarios where Redis may not be the optimal choice. Here are two common use cases for considering alternatives:
1. Large Dataset Handling
While Redis provides excellent performance for small to medium-sized datasets, it may struggle with handling larger datasets efficiently. In such cases, alternative data stores like Apache Cassandra or Apache HBase, which are designed to handle massive amounts of data and provide distributed storage, can be a better fit.
Example 1: Using Apache Cassandra for large-scale data storage
import com.datastax.oss.driver.api.core.CqlSession;public class CassandraExample { public static void main(String[] args) { try (CqlSession session = CqlSession.builder().build()) { session.execute("CREATE KEYSPACE IF NOT EXISTS mykeyspace WITH replication = {'class': 'SimpleStrategy', 'replication_factor': 1}"); session.execute("CREATE TABLE IF NOT EXISTS mykeyspace.mytable (id UUID PRIMARY KEY, name TEXT)"); session.execute("INSERT INTO mykeyspace.mytable (id, name) VALUES (uuid(), 'John')"); session.execute("INSERT INTO mykeyspace.mytable (id, name) VALUES (uuid(), 'Jane')"); } }}
2. Complex Data Structures and Queries
Redis provides a limited set of data structures and querying capabilities. If your application requires more complex data structures or advanced querying capabilities, alternative data stores like MongoDB or Apache Solr can be more suitable.
Example 2: Using MongoDB for document storage and querying
from pymongo import MongoClient# Connect to the MongoDB serverclient = MongoClient("mongodb://localhost:27017/")# Access the database and collectiondb = client["mydatabase"]collection = db["mycollection"]# Insert a documentdocument = {"name": "John", "age": 30, "city": "New York"}collection.insert_one(document)# Query documentsquery = {"city": "New York"}results = collection.find(query)for result in results: print(result)
Related Article: Tutorial: Kafka vs Redis
Evaluating Performance Considerations
When considering alternative data stores, it's crucial to evaluate their performance characteristics. Here are two important performance considerations to keep in mind:
1. Latency and Throughput
Different data stores have varying latency and throughput capabilities. For example, if your application requires low-latency operations, you may consider alternatives like Apache Ignite or Memcached.
Example 3: Using Memcached for fast key-value caching
<?php// Connect to the Memcached server$memcached = new Memcached();$memcached->addServer("localhost", 11211);// Set a value$memcached->set("key", "value");// Retrieve a value$value = $memcached->get("key");echo $value;
2. Scalability and Replication
As your application grows, the ability to scale horizontally and replicate data becomes crucial. Data stores like Apache Kafka or Apache Pulsar provide scalable and fault-tolerant distributed messaging systems that can handle large volumes of data.
Example 4: Using Apache Kafka for distributed messaging
import org.apache.kafka.clients.producer.KafkaProducer;import org.apache.kafka.clients.producer.ProducerRecord;import java.util.Properties;public class KafkaExample { public static void main(String[] args) { Properties props = new Properties(); props.put("bootstrap.servers", "localhost:9092"); props.put("key.serializer", "org.apache.kafka.common.serialization.StringSerializer"); props.put("value.serializer", "org.apache.kafka.common.serialization.StringSerializer"); try (KafkaProducer<String, String> producer = new KafkaProducer<>(props)) { ProducerRecord<String, String> record = new ProducerRecord<>("mytopic", "key", "value"); producer.send(record); } }}
Best Practices for Implementing Alternative Data Stores
Implementing alternative data stores requires careful consideration and adherence to best practices. Here are two key practices to follow:
Related Article: Tutorial: Redis vs RabbitMQ Comparison
1. Data Modeling
Proper data modeling is essential for efficiently utilizing alternative data stores. Understand the strengths and limitations of the chosen data store's data model and design your schema accordingly.
Example 5: Data modeling in Apache Cassandra
CREATE TABLE IF NOT EXISTS mykeyspace.mytable ( id UUID PRIMARY KEY, name TEXT, age INT);
2. Consistency and Durability
Ensure that your chosen alternative data store provides the desired level of consistency and durability for your application. Configure replication and durability settings based on your requirements.
Example 6: Configuring replication in MongoDB
rs.initiate( { _id: "myreplicaset", members: [ { _id: 0, host: "mongodb1:27017" }, { _id: 1, host: "mongodb2:27017" }, { _id: 2, host: "mongodb3:27017" } ] });
Real World Examples of Alternative Data Stores
To further illustrate the usage of alternative data stores, let's explore two real-world examples:
1. TimescaleDB for Time-Series Data
TimescaleDB is a time-series database built on top of PostgreSQL, designed to handle massive volumes of time-series data efficiently.
Example 7: Creating a hypertable in TimescaleDB
CREATE TABLE sensor_data ( time TIMESTAMPTZ NOT NULL, sensor_id INT NOT NULL, temperature DOUBLE PRECISION, humidity DOUBLE PRECISION, PRIMARY KEY (time, sensor_id));SELECT create_hypertable('sensor_data', 'time');
Related Article: How to Configure a Redis Cluster
2. Elasticsearch for Full-Text Search
Elasticsearch is a powerful search engine that excels in full-text search and real-time analytics.
Example 8: Indexing and searching documents in Elasticsearch
from elasticsearch import Elasticsearch# Connect to the Elasticsearch clusteres = Elasticsearch(["localhost:9200"])# Index a documentdocument = {"title": "Introduction to Elasticsearch", "content": "Elasticsearch is a distributed search engine."}es.index(index="myindex", id=1, body=document)# Search documentsquery = {"query": {"match": {"content": "search engine"}}}results = es.search(index="myindex", body=query)for hit in results["hits"]["hits"]: print(hit["_source"])
Error Handling in Alternative Data Stores
Error handling is an important aspect of utilizing alternative data stores effectively. Here are two approaches to consider:
1. Handling Connection Errors
Alternative data stores may encounter connection errors, especially when dealing with distributed systems. Implement robust error handling to gracefully handle connection failures and retries.
Example 9: Handling connection errors in Apache Cassandra
import com.datastax.oss.driver.api.core.CqlSession;import com.datastax.oss.driver.api.core.connection.ConnectionException;public class CassandraErrorHandlingExample { public static void main(String[] args) { try { CqlSession session = CqlSession.builder().build(); // Perform operations on the session } catch (ConnectionException e) { // Handle connection errors System.err.println("Connection error: " + e.getMessage()); } }}
2. Gracefully Handling Data Integrity Errors
Alternative data stores may have different constraints and error conditions. Implement appropriate error handling mechanisms to handle data integrity errors and ensure consistency.
Example 10: Handling data integrity errors in MongoDB
from pymongo import MongoClient, errors# Connect to the MongoDB servertry: client = MongoClient("mongodb://localhost:27017/")except errors.ConnectionFailure: print("Failed to connect to MongoDB.")# Access the database and collectiondb = client["mydatabase"]collection = db["mycollection"]# Insert a document with duplicate keytry: collection.insert_one({"_id": 123, "name": "John"}) collection.insert_one({"_id": 123, "name": "Jane"})except errors.DuplicateKeyError: print("Duplicate key error.")
Related Article: How to Use Redis Streams
Advanced Techniques for Alternative Data Stores
To leverage the full potential of alternative data stores, consider exploring advanced techniques. Here are two examples:
1. Geospatial Queries in PostGIS
PostGIS is an extension to PostgreSQL that provides support for geospatial data and queries.
Example 11: Performing geospatial queries in PostGIS
-- Create a table with geospatial dataCREATE TABLE places ( name TEXT, location GEOMETRY(POINT, 4326));-- Insert a pointINSERT INTO places (name, location) VALUES ('New York', ST_Point(-74.0060, 40.7128));-- Find places within a radiusSELECT nameFROM placesWHERE ST_DWithin(location, ST_MakePoint(-74.0060, 40.7128)::geography, 10000);
2. Graph Queries in Neo4j
Neo4j is a graph database that allows you to model and query complex relationships between entities.
Example 12: Modeling and querying a social graph in Neo4j
// Create nodesCREATE (alice:User {name: 'Alice'})CREATE (bob:User {name: 'Bob'})CREATE (carol:User {name: 'Carol'})// Create relationshipsCREATE (alice)-[:FOLLOWS]->(bob)CREATE (bob)-[:FOLLOWS]->(carol)// Find friends of friendsMATCH (user:User {name: 'Alice'})-[:FOLLOWS]->()-[:FOLLOWS]->(fof:User)RETURN DISTINCT fof.name
Code Snippet Ideas for Alternative Data Stores
Here are a few code snippet ideas to help you get started with implementing alternative data stores:
Related Article: How to use Redis with Laravel and PHP
1. Using Apache Kafka for Event Streaming
import org.apache.kafka.clients.producer.KafkaProducer;import org.apache.kafka.clients.producer.ProducerRecord;import java.util.Properties;public class KafkaEventProducer { public static void main(String[] args) { Properties props = new Properties(); props.put("bootstrap.servers", "localhost:9092"); props.put("key.serializer", "org.apache.kafka.common.serialization.StringSerializer"); props.put("value.serializer", "org.apache.kafka.common.serialization.StringSerializer"); try (KafkaProducer<String, String> producer = new KafkaProducer<>(props)) { ProducerRecord<String, String> record = new ProducerRecord<>("events-topic", "event-key", "event-data"); producer.send(record); } }}
2. Using Apache Solr for Search Functionality
import org.apache.solr.client.solrj.SolrClient;import org.apache.solr.client.solrj.impl.HttpSolrClient;import org.apache.solr.client.solrj.SolrQuery;import org.apache.solr.client.solrj.response.QueryResponse;import org.apache.solr.common.SolrDocumentList;public class SolrSearchClient { public static void main(String[] args) throws Exception { String solrUrl = "http://localhost:8983/solr/mycollection"; SolrClient solrClient = new HttpSolrClient.Builder(solrUrl).build(); SolrQuery query = new SolrQuery("search keyword"); QueryResponse response = solrClient.query(query); SolrDocumentList results = response.getResults(); for (int i = 0; i < results.size(); i++) { System.out.println(results.get(i)); } solrClient.close(); }}