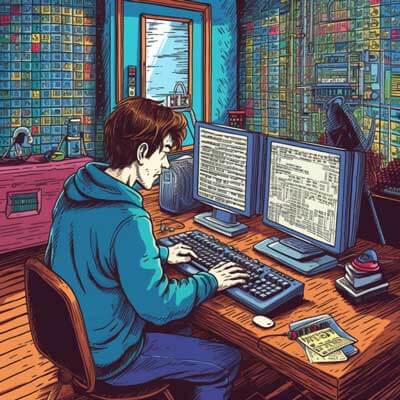
Table of Contents
What are React components?
React components are the building blocks of a React application. They are reusable pieces of code that encapsulate both the UI and the logic of a specific part of the user interface. Each component can be thought of as an independent, self-contained unit that can be combined with other components to create complex user interfaces. Components can be either functional or class-based.
Functional components are simple JavaScript functions that take in props (more on that later) as arguments and return JSX (JavaScript XML) to describe what should be rendered on the screen. Here's an example of a functional component:
function Welcome(props) { return <h1>Hello, {props.name}!</h1>;}
Class-based components are JavaScript classes that extend the React.Component
class. They use the render()
method to define what should be rendered on the screen. Class-based components also have access to additional features such as lifecycle methods and state. Here's an example of a class-based component:
class Welcome extends React.Component { render() { return <h1>Hello, {this.props.name}!</h1>; }}
Both functional and class-based components can be used interchangeably in a React application, depending on the complexity and requirements of the component.
Related Article: How to Use the Render Method in ReactJS
What is the Virtual DOM in React?
The Virtual DOM (VDOM) is a concept in React that allows for efficient rendering of components. It is a lightweight copy of the actual DOM (Document Object Model) that React uses to keep track of the state and structure of the UI. Instead of directly manipulating the actual DOM, React makes changes to the Virtual DOM and then reconciles those changes with the actual DOM.
The Virtual DOM works by creating a tree-like structure of React elements that represent the UI components. When there are changes to the state or props of a component, React updates the Virtual DOM tree accordingly. It then compares the new Virtual DOM tree with the previous one to determine the minimal set of changes needed to update the actual DOM.
This process of comparing and updating the Virtual DOM is called reconciliation. React uses a diffing algorithm to efficiently update only the parts of the DOM that have changed, reducing the overall performance impact and making UI updates faster.
Here's an example of how the Virtual DOM works:
// Initial stateconst state = { count: 0};// Render the component based on the stateconst element = <div>{state.count}</div>;// Update the statestate.count = 1;// Render the component again and compare with the previous Virtual DOM treeconst newElement = <div>{state.count}</div>;// Update only the changed parts of the actual DOM
In this example, when the state changes from 0 to 1, React updates only the part of the actual DOM that displays the count, instead of re-rendering the entire page.
How is JSX used in React?
JSX (JavaScript XML) is a syntax extension for JavaScript that allows you to write HTML-like code directly in your JavaScript files. It is a way to describe the structure and appearance of the UI in a declarative and concise manner.
JSX looks similar to HTML, but it is not valid JavaScript. However, React provides a tool called Babel that can transpile JSX code into regular JavaScript that browsers can understand.
Here's an example of how JSX is used in React:
const element = <h1>Hello, world!</h1>;
In this example, the variable element
contains JSX code that represents a heading element with the text "Hello, world!". This JSX code will be transpiled into JavaScript code that creates a React element.
JSX can also contain JavaScript expressions by wrapping them in curly braces {}
. This allows you to dynamically generate content and perform calculations within the JSX code. Here's an example:
const name = 'John Doe';const element = <h1>Hello, {name}!</h1>;
In this example, the JSX code includes a JavaScript expression {name}
that will be replaced with the value of the name
variable when the code is transpiled.
JSX is not required to use React, but it is a recommended and widely used syntax for writing React components due to its readability and expressiveness.
What is ReactDOM?
ReactDOM is a package in React that provides the necessary methods for rendering React components to the actual DOM. It serves as the bridge between the Virtual DOM and the browser's DOM.
ReactDOM contains a variety of methods, but the most commonly used method is ReactDOM.render()
. This method is responsible for rendering a React element or component to a specified container in the DOM.
Here's an example of how ReactDOM.render()
is used:
const element = <h1>Hello, world!</h1>;ReactDOM.render(element, document.getElementById('root'));
In this example, the ReactDOM.render()
method takes two arguments. The first argument is the React element or component that you want to render, and the second argument is the DOM element where you want to render it. In this case, the element <h1>Hello, world!</h1>
will be rendered inside the DOM element with the ID 'root'.
ReactDOM also provides other methods for more advanced rendering scenarios, such as ReactDOM.hydrate()
for server-side rendering and ReactDOM.createPortal()
for rendering components outside of the normal React component tree.
Related Article: Exploring Key Features of ReactJS
How does the render method work in React?
In React, the render()
method is a required method in a class-based component that defines what should be rendered on the screen. It is responsible for returning a React element or a tree of React elements that represent the UI of the component.
Here's an example of a class-based component with a render()
method:
class MyComponent extends React.Component { render() { return <h1>Hello, world!</h1>; }}
In this example, the render()
method returns a JSX element <h1>Hello, world!</h1>
. This element will be rendered to the screen when the component is mounted.
The render()
method can also return an array of elements, a string, a number, or a boolean, but it is most commonly used to return a single JSX element or a tree of elements.
It's important to note that the render()
method should be pure, meaning it should not modify the component's state or have any side effects. It should only be used to describe what the UI should look like based on the component's props and state.
React calls the render()
method whenever the component's props or state change, or when the parent component re-renders. This allows React to efficiently update only the parts of the UI that have changed, thanks to the Virtual DOM and the diffing algorithm.
What is the concept of Component hierarchy in React?
In React, component hierarchy refers to the structure and organization of components within a React application. It defines how components are nested inside one another and how they interact with each other.
Component hierarchy is important in React because it allows for a modular and reusable architecture. By breaking down the UI into smaller, independent components, you can easily manage and update different parts of the UI without affecting the rest of the application.
Here's an example of a component hierarchy in a simple React application:
function App() { return ( <div> <Header /> <MainContent /> <Footer /> </div> );}
In this example, the App
component is the top-level component that represents the entire application. It contains three child components: Header
, MainContent
, and Footer
. Each of these child components can have their own child components, creating a hierarchical structure.
Component hierarchy also plays a role in component reusability. By breaking down the UI into smaller components, you can reuse those components in different parts of the application, reducing code duplication and improving maintainability.
What are props in React?
In React, props (short for properties) are a way to pass data from a parent component to its child component. Props are read-only and cannot be modified by the child component.
Props are a fundamental concept in React and are used to create dynamic and reusable components. They allow you to customize the behavior and appearance of a component by passing different values to its props.
Here's an example of how props are used in React:
function Greeting(props) { return <h1>Hello, {props.name}!</h1>;}const App = () => { return <Greeting name="John" />;}
In this example, the Greeting
component accepts a prop called name
and renders a personalized greeting. The App
component then renders the Greeting
component and passes the value "John" to the name
prop.
Props can also be passed to class-based components using the this.props
syntax:
class Greeting extends React.Component { render() { return <h1>Hello, {this.props.name}!</h1>; }}const App = () => { return <Greeting name="John" />;}
In this example, the this.props.name
expression is used to access the name
prop inside the render()
method of the Greeting
component.
Props can be of any data type, including strings, numbers, booleans, objects, or even functions. They can also be used to pass down event handlers or other callback functions to child components, allowing for interactive and dynamic behavior.
What is state in React?
In React, state is a way to store and manage data within a component. Unlike props, which are passed from a parent component and are read-only, state is internal to a component and can be modified using the setState()
method.
State is useful for storing data that can change over time, such as user input, API responses, or the result of an asynchronous operation. By updating the state, React can automatically re-render the component and update the UI to reflect the new state.
Here's an example of how state is used in React:
class Counter extends React.Component { constructor(props) { super(props); this.state = { count: 0 }; } increment() { this.setState({ count: this.state.count + 1 }); } render() { return ( <div> <p>Count: {this.state.count}</p> <button onClick={() => this.increment()}>Increment</button> </div> ); }}
In this example, the Counter
component has an initial state with a count
property set to 0. The increment()
method is used to update the state by incrementing the count
property. The updated state is then reflected in the UI by using this.state.count
inside the render()
method.
It's important to note that you should never modify the state directly. Instead, use the setState()
method provided by React to update the state. This ensures that React can properly track and update the component's state.
State can also be passed down to child components as props, allowing for data flow between components. This allows you to create complex and interactive UIs by managing state at the appropriate level of the component hierarchy.
Related Article: How to Set Up Your First ReactJS Project
What are lifecycle methods in React?
Lifecycle methods are special methods that are automatically called at different stages of a component's lifecycle in React. They allow you to hook into the component's lifecycle events and perform actions at specific times, such as initializing the component, updating the component, or cleaning up resources.
React provides several lifecycle methods that you can override in class-based components:
- componentDidMount(): This method is called after the component has been rendered to the DOM. It is commonly used to fetch data from an API, set up event listeners, or perform other side effects.
- componentDidUpdate(prevProps, prevState): This method is called after the component has been updated. It receives the previous props and state as arguments, allowing you to compare them with the current props and state and perform actions based on the changes.
- componentWillUnmount(): This method is called right before the component is removed from the DOM. It is commonly used to clean up any resources, such as event listeners or timers, that were set up in the componentDidMount()
method.
- shouldComponentUpdate(nextProps, nextState): This method is called before the component is updated. It receives the next props and state as arguments and allows you to control whether the component should re-render or not. By default, React re-renders the component whenever the props or state change, but you can optimize performance by implementing custom logic in this method.
- getDerivedStateFromProps(nextProps, prevState): This method is called before the component is rendered, both on the initial mount and on subsequent updates. It receives the next props and the previous state as arguments and returns an object that represents the updated state. This method is rarely used and should be used with caution.
- render(): This method is required and is responsible for returning a React element or a tree of elements that represent the UI of the component. It is called whenever the component needs to be re-rendered.
Here's an example of how lifecycle methods are used in a class-based component:
class MyComponent extends React.Component { componentDidMount() { console.log('Component mounted'); } componentDidUpdate(prevProps, prevState) { console.log('Component updated'); } componentWillUnmount() { console.log('Component unmounted'); } render() { return <h1>Hello, world!</h1>; }}
In this example, the componentDidMount()
method logs a message to the console when the component is mounted, the componentDidUpdate()
method logs a message when the component is updated, and the componentWillUnmount()
method logs a message when the component is unmounted.
Lifecycle methods provide a way to handle common scenarios in a React component's lifecycle, such as fetching data, updating the UI, or cleaning up resources. By using these methods effectively, you can create components that are responsive, efficient, and maintainable.
What is the process of reconciliation in React?
The process of reconciliation in React is the algorithm used by React to efficiently update the actual DOM based on the changes in the Virtual DOM. It is a key part of React's performance optimization strategy.
When a component's state or props change, React updates the Virtual DOM by creating a new tree of React elements that represents the updated UI. It then compares this new Virtual DOM tree with the previous one to determine the minimal set of changes needed to update the actual DOM.
The reconciliation process works by performing a diffing algorithm between the new and previous Virtual DOM trees. React compares each element in the new tree with its corresponding element in the previous tree and determines whether they are the same or different.
When React finds a difference between two elements, it updates the corresponding part of the actual DOM to reflect the new element. React uses efficient algorithms and data structures to minimize the number of DOM operations and optimize the update process.
Here's an example of how the reconciliation process works:
// Initial stateconst state = { count: 0};// Render the component based on the stateconst element = <div>{state.count}</div>;// Update the statestate.count = 1;// Render the component again and compare with the previous Virtual DOM treeconst newElement = <div>{state.count}</div>;// Update only the changed parts of the actual DOM
In this example, when the state changes from 0 to 1, React updates only the part of the actual DOM that displays the count, instead of re-rendering the entire page.
React's reconciliation process is efficient because it only updates the necessary parts of the DOM, reducing the overall performance impact. It also allows for a more declarative programming model, where you describe the desired UI state and React takes care of updating the actual DOM accordingly.
However, it's important to note that the reconciliation process has a cost, especially for complex components or components with large amounts of data. To optimize performance, React provides several techniques, such as keying, memoization, and shouldComponentUpdate(), that allow you to control and fine-tune the reconciliation process. By applying these techniques, you can ensure that your React application remains fast and responsive even as it grows in complexity.
Code Snippet: Creating a React component
import React from 'react';function MyComponent() { return <h1>Hello, world!</h1>;}
In this code snippet, we import the React
package and define a functional component called MyComponent
. The component returns a JSX element <h1>Hello, world!</h1>
, which will be rendered to the screen.
To use this component, you can simply include it in the JSX code of another component or in the ReactDOM.render()
method.
Code Snippet: Using the Virtual DOM in React
// Initial stateconst state = { count: 0};// Render the component based on the stateconst element = <div>{state.count}</div>;
In this code snippet, we define an initial state object with a count
property set to 0. We then create a JSX element <div>{state.count}</div>
that will render the value of state.count
to the screen. This JSX code represents a part of the Virtual DOM.
Related Article: How to Render ReactJS Code with NPM
Code Snippet: Writing JSX
const name = 'John Doe';const element = <h1>Hello, {name}!</h1>;
In this code snippet, we define a variable name
with the value 'John Doe'
. We then create a JSX element <h1>Hello, {name}!</h1>
that includes a JavaScript expression {name}
to insert the value of name
into the JSX code.
JSX allows you to write HTML-like code directly in your JavaScript files, making it easier to describe the structure and appearance of the UI. JSX code can also contain JavaScript expressions wrapped in curly braces {}
, allowing for dynamic and reusable components.
Code Snippet: Rendering a component with ReactDOM
import React from 'react';import ReactDOM from 'react-dom';function MyComponent() { return <h1>Hello, world!</h1>;}ReactDOM.render(<MyComponent />, document.getElementById('root'));
In this code snippet, we import the React
and ReactDOM
packages. We then define a functional component called MyComponent
that returns a JSX element <h1>Hello, world!</h1>
.
To render this component to the actual DOM, we use the ReactDOM.render()
method. We pass the JSX element <MyComponent />
as the first argument, which represents the component we want to render. We also specify the DOM element with the ID 'root' as the second argument, which is the container where the component will be rendered.
Code Snippet: Understanding the render method in React
class MyComponent extends React.Component { render() { return <h1>Hello, world!</h1>; }}
In this code snippet, we define a class-based component called MyComponent
that extends the React.Component
class. The component overrides the render()
method to define what should be rendered on the screen.
The render()
method returns a JSX element <h1>Hello, world!</h1>
, which will be rendered to the screen when the component is mounted.
The render()
method is required in class-based components and is responsible for describing the UI based on the component's props and state. It is called whenever the component needs to be re-rendered, such as when the props or state change, or when the parent component re-renders.
Code Snippet: Structuring component hierarchy
function App() { return ( <div> <Header /> <MainContent /> <Footer /> </div> );}
In this code snippet, we define a functional component called App
that represents the top-level component of our application. The component returns a JSX element <div>
that contains three child components: Header
, MainContent
, and Footer
.
Related Article: How to Add If Statements in a ReactJS Render Method
Code Snippet: Passing props to a React component
function Greeting(props) { return <h1>Hello, {props.name}!</h1>;}const App = () => { return <Greeting name="John" />;}
In this code snippet, we define a functional component called Greeting
that accepts a prop called name
. The component renders a personalized greeting by including the value of props.name
in the JSX code.
In the App
component, we render the Greeting
component and pass the value "John" to the name
prop. This allows us to customize the behavior of the Greeting
component by passing different values to its props.
Props can be of any data type and can also be used to pass down event handlers or other callback functions to child components, allowing for interactive and dynamic behavior.
Code Snippet: Managing state in React
class Counter extends React.Component { constructor(props) { super(props); this.state = { count: 0 }; } increment() { this.setState({ count: this.state.count + 1 }); } render() { return ( <div> <p>Count: {this.state.count}</p> <button onClick={() => this.increment()}>Increment</button> </div> ); }}
In this code snippet, we define a class-based component called Counter
that manages a state property called count
. The initial value of count
is set to 0 in the constructor.
The component also defines an increment()
method that updates the state by incrementing the value of count
by 1. The updated state is then reflected in the UI by using this.state.count
inside the render()
method.
Code Snippet: Utilizing lifecycle methods in React
class MyComponent extends React.Component { componentDidMount() { console.log('Component mounted'); } componentDidUpdate(prevProps, prevState) { console.log('Component updated'); } componentWillUnmount() { console.log('Component unmounted'); } render() { return <h1>Hello, world!</h1>; }}
In this code snippet, we define a class-based component called MyComponent
that overrides several lifecycle methods provided by React.
The componentDidMount()
method is called after the component has been rendered to the DOM. In this example, it logs a message to the console when the component is mounted.
The componentDidUpdate()
method is called after the component has been updated. It receives the previous props and state as arguments, allowing you to compare them with the current props and state and perform actions based on the changes. In this example, it logs a message to the console when the component is updated.
The componentWillUnmount()
method is called right before the component is removed from the DOM. In this example, it logs a message to the console when the component is unmounted.
These lifecycle methods allow you to hook into different stages of the component's lifecycle and perform actions at specific times, such as fetching data, updating the UI, or cleaning up resources.
Code Snippet: Exploring React's reconciliation process
// Initial stateconst state = { count: 0};// Render the component based on the stateconst element = <div>{state.count}</div>;// Update the statestate.count = 1;// Render the component again and compare with the previous Virtual DOM treeconst newElement = <div>{state.count}</div>;// Update only the changed parts of the actual DOM
In this code snippet, we demonstrate how React's reconciliation process works by updating a component's state and re-rendering it.
We start with an initial state object that contains a count
property set to 0. We then create a JSX element <div>{state.count}</div>
that represents a part of the Virtual DOM and renders the value of state.count
.
When the state changes from 0 to 1, we update the state.count
property and re-render the component. React compares the new Virtual DOM tree with the previous one and determines the minimal set of changes needed to update the actual DOM. In this case, only the part of the actual DOM that displays the count will be updated.
React's reconciliation process is efficient because it only updates the necessary parts of the DOM, reducing the overall performance impact. This allows for fast and responsive UI updates, even in large and complex React applications.