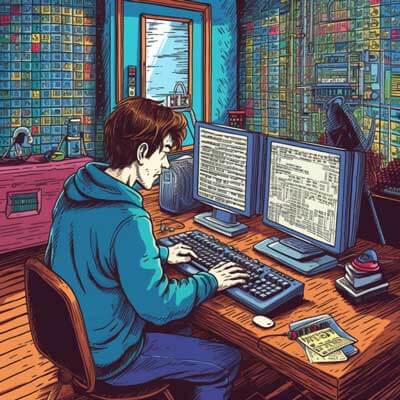
Table of Contents
Directus is an open-source headless CMS (Content Management System) that provides a useful interface for managing and delivering content to various channels. With the introduction of Directus GraphQL, developers have a new way to interact with and consume data from Directus APIs. In this article, we will explore the various aspects of Directus GraphQL and how it can be leveraged in programming.
Schema Stitching in Directus GraphQL
Schema stitching is a technique that allows multiple GraphQL schemas to be combined into a single schema. In the context of Directus GraphQL, schema stitching can be used to integrate Directus with other GraphQL APIs or to combine multiple Directus projects into a unified schema.
Here is an example of how schema stitching can be implemented in Directus GraphQL using the graphql-tools
library:
const { stitchSchemas } = require('@graphql-tools/stitch'); const schema1 = require('./schema1'); const schema2 = require('./schema2'); const stitchedSchema = stitchSchemas({ schemas: [schema1, schema2], });
In this example, schema1
and schema2
represent the individual schemas that need to be stitched together. The stitchSchemas
function from the @graphql-tools/stitch
library is used to combine these schemas into a single schema called stitchedSchema
.
Related Article: Implementing TypeORM with GraphQL and NestJS
The Role of Apollo Client in Directus GraphQL
Apollo Client is a useful GraphQL client that provides a seamless way to interact with GraphQL APIs. In the context of Directus GraphQL, Apollo Client can be used to fetch data from Directus APIs, perform mutations, and subscribe to real-time updates.
To use Apollo Client with Directus GraphQL, you need to create an instance of Apollo Client and configure it to connect to your Directus GraphQL endpoint. Here is an example of how to set up Apollo Client with Directus GraphQL:
import { ApolloClient, InMemoryCache } from '@apollo/client'; const client = new ApolloClient({ uri: 'https://directus.example.com/graphql', cache: new InMemoryCache(), });
In this example, the ApolloClient
class is imported from the @apollo/client
package. The uri
option is set to the Directus GraphQL endpoint, and the cache
option is set to an instance of InMemoryCache
, which is the default cache implementation provided by Apollo Client.
How GraphQL Subscriptions Work in Directus GraphQL
GraphQL subscriptions allow clients to receive real-time updates from a GraphQL API. In the context of Directus GraphQL, subscriptions can be used to listen for changes to data in the Directus database and receive updates in real-time.
Directus GraphQL uses the PubSub
class from the graphql-subscriptions
library to implement subscriptions. Here is an example of how to set up a subscription in Directus GraphQL:
import { PubSub } from 'graphql-subscriptions'; const pubsub = new PubSub(); const Subscription = { commentAdded: { subscribe: () => pubsub.asyncIterator('COMMENT_ADDED'), }, }; const resolvers = { Subscription, };
In this example, the PubSub
class is imported from the graphql-subscriptions
package. An instance of PubSub
is created, and a subscription resolver called commentAdded
is defined. The subscribe
function returns an AsyncIterator
that listens for events with the COMMENT_ADDED
topic.
Writing GraphQL Mutations in Directus
GraphQL mutations allow clients to modify data in a GraphQL API. In the context of Directus GraphQL, mutations can be used to create, update, and delete records in the Directus database.
Here is an example of how to write a mutation in Directus GraphQL:
mutation { createPost(input: { title: "New Post", content: "Lorem ipsum dolor sit amet" }) { id title content } }
In this example, a createPost
mutation is defined with an input
argument that contains the data for creating a new post. The mutation returns the id
, title
, and content
fields of the newly created post.
Related Article: Working with GraphQL Enums: Values Explained
Defining Resolvers in Directus GraphQL
Resolvers are functions that resolve the data for a GraphQL query or mutation. In the context of Directus GraphQL, resolvers can be used to fetch data from the Directus database, perform transformations, and return the requested data to the client.
Here is an example of how to define resolvers in Directus GraphQL:
const resolvers = { Query: { posts: async () => { // Fetch posts from the Directus database const response = await fetch('https://directus.example.com/api/1.1/tables/posts/rows'); const data = await response.json(); // Return the posts return data; }, }, };
In this example, a resolver is defined for the posts
field in the Query
type. The resolver uses the fetch
function to fetch the posts from the Directus database and returns the fetched data.
Understanding GraphQL Introspection in Directus
GraphQL introspection allows clients to fetch the schema of a GraphQL API. In the context of Directus GraphQL, introspection can be used to discover the available types, fields, and directives in the Directus schema.
To enable introspection in Directus GraphQL, you need to set the introspection
option to true
in the Directus configuration file. Here is an example of how to enable introspection in Directus:
module.exports = { // Other Directus configuration options introspection: true, };
With introspection enabled, clients can use tools like GraphiQL or GraphQL Playground to explore the Directus schema and execute queries against the API.
Using GraphQL Directives in Directus
GraphQL directives are used to add additional functionality to the schema. In the context of Directus GraphQL, directives can be used to apply custom logic or transformations to fields, arguments, or entire queries/mutations.
Here is an example of how to define and use a directive in Directus GraphQL:
directive @uppercase on FIELD_DEFINITION type Query { hello: String @uppercase } schema { query: Query }
In this example, a custom directive called uppercase
is defined using the @uppercase
syntax. The directive is applied to the hello
field in the Query
type, which transforms the returned string to uppercase.
Leveraging GraphQL Fragments in Directus
GraphQL fragments allow clients to define reusable selections of fields that can be included in multiple queries or mutations. In the context of Directus GraphQL, fragments can be used to define common sets of fields that are frequently requested.
Here is an example of how to define and use a fragment in Directus GraphQL:
fragment PostFields on Post { id title content } query { post(id: 1) { ...PostFields } }
In this example, a fragment called PostFields
is defined with the id
, title
, and content
fields of the Post
type. The fragment is then included in a query for a specific post, which allows the selected fields to be reused in multiple queries.
Related Article: Implementing Upsert Operation in GraphQL
Defining a GraphQL Schema in Directus
A GraphQL schema defines the types, fields, and directives available in a GraphQL API. In the context of Directus GraphQL, a schema can be defined using the Directus schema builder or by writing the schema definition language (SDL) directly.
Here is an example of how to define a schema in Directus using the Directus schema builder:
const schema = new SchemaBuilder() .createObjectType('Post') .addFields({ id: 'ID', title: 'String', content: 'String', }) .build();
In this example, a schema builder instance is created using the SchemaBuilder
class. An object type called Post
is defined with the id
, title
, and content
fields. The schema is then built using the build
method.
Understanding GraphQL Scalars in Directus
GraphQL scalars are the basic building blocks of a GraphQL schema and represent primitive data types like strings, integers, booleans, and floats. In the context of Directus GraphQL, scalars can be used to define the types of fields in the Directus schema.
Here is an example of how to define a scalar in Directus GraphQL:
scalar Date type Post { id: ID title: String createdAt: Date }
In this example, a scalar called Date
is defined. The Date
scalar can be used as the type for the createdAt
field in the Post
type, allowing dates to be represented in the Directus schema.
Additional Resources
- Directus Official Website