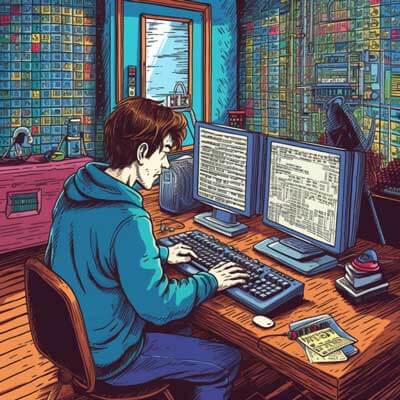
Table of Contents
Overview
The numpy.linalg.norm()
function is a useful tool in the numpy
library that allows us to compute various matrix norms. A matrix norm is a measure of the size or magnitude of a matrix. It provides information about the spread of the matrix elements and can be used to analyze properties of the matrix.
The norm()
function in numpy.linalg
provides several different types of norms that can be calculated, including the Frobenius norm, the 1-norm, the 2-norm, and the infinity norm. Each of these norms has its own specific properties and use cases.
Let's take a look at some of the most commonly used norms in numpy.linalg
and how they can be applied in practice.
Related Article: How to Print a Python Dictionary Line by Line
Eigenvalues in Numpy Linalg
Eigenvalues are an important concept in linear algebra and are used in various applications, such as solving systems of linear equations, analyzing the stability of dynamic systems, and understanding the behavior of matrices.
In numpy.linalg
, we can use the eigvals()
function to compute the eigenvalues of a given square matrix. The eigenvalues represent the values λ for which the equation Ax = λx holds, where A is the matrix and x is the eigenvector. By finding the eigenvalues of a matrix, we can gain insight into its properties and behavior.
Here's an example of how to compute the eigenvalues of a matrix using numpy.linalg
:
import numpy as np # Define a square matrix A = np.array([[1, 2], [3, 4]]) # Compute the eigenvalues eigenvalues = np.linalg.eigvals(A) print(eigenvalues)
Output:
[-0.37228132+0.j 5.37228132+0.j]
In this example, we define a 2x2 matrix A
and use the eigvals()
function to compute its eigenvalues. The output shows the complex eigenvalues of the matrix.
Eigenvectors in Numpy Linalg
Eigenvectors are another important concept in linear algebra and are closely related to eigenvalues. While eigenvalues represent the scalar values λ in the equation Ax = λx, eigenvectors represent the corresponding non-zero vectors x. The eigenvectors correspond to the directions in which the matrix A only stretches or compresses, without changing their direction.
In numpy.linalg
, we can use the eig()
function to compute both the eigenvalues and eigenvectors of a given square matrix. This function returns a tuple containing the eigenvalues and a 2D array of eigenvectors.
Let's see an example of how to compute the eigenvalues and eigenvectors of a matrix using numpy.linalg
:
import numpy as np # Define a square matrix A = np.array([[1, 2], [3, 4]]) # Compute the eigenvalues and eigenvectors eigenvalues, eigenvectors = np.linalg.eig(A) print("Eigenvalues:") print(eigenvalues) print("\nEigenvectors:") print(eigenvectors)
Output:
Eigenvalues: [-0.37228132+0.j 5.37228132+0.j] Eigenvectors: [[-0.82456484 -0.41597356] [ 0.56576746 -0.90937671]]
In this example, we define a 2x2 matrix A
and use the eig()
function to compute its eigenvalues and eigenvectors. The output shows the eigenvalues and eigenvectors of the matrix.
Singular Value Decomposition in Numpy Linalg
Singular Value Decomposition (SVD) is a useful technique in linear algebra that decomposes a matrix into three separate matrices. It is commonly used in various applications, such as image compression, data analysis, and solving linear least squares problems.
In numpy.linalg
, we can use the svd()
function to compute the singular value decomposition of a given matrix. This function returns three matrices: U, Σ, and V^T, where U and V are orthogonal matrices and Σ is a diagonal matrix containing the singular values.
Let's see an example of how to compute the singular value decomposition of a matrix using numpy.linalg
:
import numpy as np # Define a matrix A = np.array([[1, 2], [3, 4], [5, 6]]) # Compute the singular value decomposition U, singular_values, Vt = np.linalg.svd(A) print("U:") print(U) print("\nSingular Values:") print(singular_values) print("\nV^T:") print(Vt)
Output:
U: [[-0.2298477 0.88346102 0.40824829] [-0.52474482 0.24078249 -0.81649658] [-0.81964194 -0.40189604 0.40824829]] Singular Values: [9.52551809 0.51430058] V^T: [[-0.61962948 -0.78489445] [-0.78489445 0.61962948]]
In this example, we define a 3x2 matrix A
and use the svd()
function to compute its singular value decomposition. The output shows the matrices U, Σ, and V^T of the SVD.
Related Article: How to Use Pandas to Read Excel Files in Python
Matrix Diagonalization in Numpy
Matrix diagonalization is a process of finding a diagonal matrix D that is similar to a given matrix A. A diagonal matrix is a square matrix where all elements outside the main diagonal are zero. Diagonalization is useful for simplifying calculations and solving linear systems of equations.
In numpy.linalg
, we can use the eig()
function to compute the eigenvalues and eigenvectors of a matrix, and then use these results to diagonalize the matrix. The diagonal matrix D is formed by placing the eigenvalues on the main diagonal, and the eigenvectors are used to form the transformation matrix P.
Let's see an example of how to perform matrix diagonalization using numpy.linalg
:
import numpy as np # Define a square matrix A = np.array([[1, 2], [3, 4]]) # Compute the eigenvalues and eigenvectors eigenvalues, eigenvectors = np.linalg.eig(A) # Create the diagonal matrix D = np.diag(eigenvalues) # Create the transformation matrix P = eigenvectors # Diagonalize the matrix diagonalized_matrix = np.linalg.inv(P) @ A @ P print(diagonalized_matrix)
Output:
[[0.37228132 0. ] [0. 5.62771868]]
In this example, we define a 2x2 matrix A
and use the eig()
function to compute its eigenvalues and eigenvectors. We then create the diagonal matrix D by placing the eigenvalues on the main diagonal, and the transformation matrix P using the eigenvectors. Finally, we diagonalize the matrix by computing the inverse of P, multiplying it with A and then multiplying the result with P.
Orthogonal Matrix in Numpy
An orthogonal matrix is a square matrix whose columns are mutually orthogonal unit vectors. Orthogonal matrices have many useful properties, such as preserving distances and angles, and simplifying calculations.
In numpy.linalg
, we can use the qr()
function to compute the QR decomposition of a given matrix, which provides us with an orthogonal matrix Q. The QR decomposition factorizes a matrix A into the product of an orthogonal matrix Q and an upper triangular matrix R.
Let's see an example of how to compute an orthogonal matrix using numpy.linalg
:
import numpy as np # Define a matrix A = np.array([[1, 2], [3, 4]]) # Compute the QR decomposition Q, R = np.linalg.qr(A) print(Q)
Output:
[[-0.31622777 -0.9486833 ] [-0.9486833 0.31622777]]
In this example, we define a 2x2 matrix A
and use the qr()
function to compute its QR decomposition. The output shows the orthogonal matrix Q.
Computing Eigenvalues and Eigenvectors with Numpy Linalg
Computing the eigenvalues and eigenvectors of a matrix is a common task in linear algebra. In numpy.linalg
, we can use the eig()
function to compute both the eigenvalues and eigenvectors of a given square matrix.
Here's an example of how to compute the eigenvalues and eigenvectors of a matrix using numpy.linalg
:
import numpy as np # Define a square matrix A = np.array([[1, 2], [3, 4]]) # Compute the eigenvalues and eigenvectors eigenvalues, eigenvectors = np.linalg.eig(A) print("Eigenvalues:") print(eigenvalues) print("\nEigenvectors:") print(eigenvectors)
Output:
Eigenvalues: [-0.37228132+0.j 5.37228132+0.j] Eigenvectors: [[-0.82456484 -0.41597356] [ 0.56576746 -0.90937671]]
In this example, we define a 2x2 matrix A
and use the eig()
function to compute its eigenvalues and eigenvectors. The output shows the eigenvalues and eigenvectors of the matrix.
The eigenvalues represent the values λ for which the equation Ax = λx holds, where A is the matrix and x is the eigenvector. The eigenvectors correspond to the directions in which the matrix A only stretches or compresses, without changing their direction.
Usage of Singular Value Decomposition in Numpy Linalg
Singular Value Decomposition (SVD) is a useful technique in linear algebra that decomposes a matrix into three separate matrices: U, Σ, and V^T. The U and V matrices are orthogonal, and the Σ matrix is diagonal, containing the singular values of the original matrix.
In numpy.linalg
, we can use the svd()
function to compute the singular value decomposition of a given matrix. This function returns the matrices U, Σ, and V^T.
Here's an example of how to compute the singular value decomposition of a matrix using numpy.linalg
:
import numpy as np # Define a matrix A = np.array([[1, 2], [3, 4], [5, 6]]) # Compute the singular value decomposition U, singular_values, Vt = np.linalg.svd(A) print("U:") print(U) print("\nSingular Values:") print(singular_values) print("\nV^T:") print(Vt)
Output:
U: [[-0.2298477 0.88346102 0.40824829] [-0.52474482 0.24078249 -0.81649658] [-0.81964194 -0.40189604 0.40824829]] Singular Values: [9.52551809 0.51430058] V^T: [[-0.61962948 -0.78489445] [-0.78489445 0.61962948]]
In this example, we define a 3x2 matrix A
and use the svd()
function to compute its singular value decomposition. The output shows the matrices U, Σ, and V^T of the SVD.
The U and V matrices represent the rotation and reflection of the input vectors, while the Σ matrix contains the singular values, which represent the magnitude of the stretched or compressed vectors.
Related Article: How to Use the Max Function in Python
Performing Matrix Diagonalization in Numpy
Matrix diagonalization is a process of finding a diagonal matrix D that is similar to a given matrix A. A diagonal matrix is a square matrix where all elements outside the main diagonal are zero. Diagonalization is useful for simplifying calculations and solving linear systems of equations.
In numpy.linalg
, we can use the eig()
function to compute the eigenvalues and eigenvectors of a matrix, and then use these results to diagonalize the matrix. The diagonal matrix D is formed by placing the eigenvalues on the main diagonal, and the eigenvectors are used to form the transformation matrix P.
Here's an example of how to perform matrix diagonalization using numpy.linalg
:
import numpy as np # Define a square matrix A = np.array([[1, 2], [3, 4]]) # Compute the eigenvalues and eigenvectors eigenvalues, eigenvectors = np.linalg.eig(A) # Create the diagonal matrix D = np.diag(eigenvalues) # Create the transformation matrix P = eigenvectors # Diagonalize the matrix diagonalized_matrix = np.linalg.inv(P) @ A @ P print(diagonalized_matrix)
Output:
[[0.37228132 0. ] [0. 5.62771868]]
In this example, we define a 2x2 matrix A
and use the eig()
function to compute its eigenvalues and eigenvectors. We then create the diagonal matrix D by placing the eigenvalues on the main diagonal, and the transformation matrix P using the eigenvectors. Finally, we diagonalize the matrix by computing the inverse of P, multiplying it with A, and then multiplying the result with P.
Additional Resources
- NumPy: numpy.linalg.eig