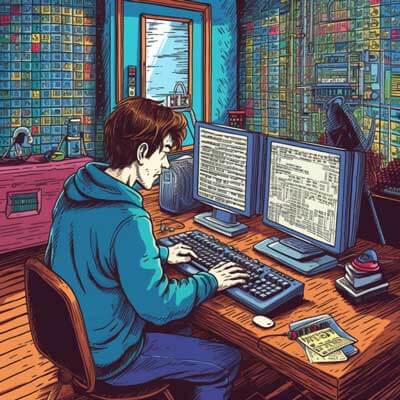
Table of Contents
MongoDB is a popular NoSQL database that allows for flexible and efficient querying of data. One common type of query is the range query, which allows you to retrieve documents that fall within a specified range of values. Range queries can be performed on various data types, including numbers, strings, and dates.
Performing Range Queries with MongoDB Find
The find()
method is used to query documents in a collection. To perform a range query, you can use the $gte
and $lte
operators to specify the lower and upper bounds of the range, respectively.
Here's an example of a range query using the find()
method to retrieve all documents with a field age
greater than or equal to 25:
db.users.find({ age: { $gte: 25 } })
This query will return all documents in the users
collection where the age
field is greater than or equal to 25.
You can also combine multiple range queries using the $and
operator. For example, to retrieve documents with a field age
between 25 and 30, you can use the following query:
db.users.find({ $and: [{ age: { $gte: 25 } }, { age: { $lte: 30 } }] })
This query will return all documents in the users
collection where the age
field is greater than or equal to 25 and less than or equal to 30.
Related Article: Comparing Databases: MongoDB, Scylla, and Snowflake
Exploring Range Queries with the $gte Operator
The $gte
operator allows you to match values that are greater than or equal to a specified value. It can be used in range queries to retrieve documents that fall within a specified range.
Here's an example of a range query using the $gte
operator to retrieve all documents with a field price
greater than or equal to 100:
db.products.find({ price: { $gte: 100 } })
This query will return all documents in the products
collection where the price
field is greater than or equal to 100.
You can also use the $gte
operator with other query operators, such as $and
and $or
, to create more complex range queries. For example, to retrieve documents with a field price
greater than or equal to 100 and less than or equal to 200, you can use the following query:
db.products.find({ $and: [{ price: { $gte: 100 } }, { price: { $lte: 200 } }] })
This query will return all documents in the products
collection where the price
field is greater than or equal to 100 and less than or equal to 200.
The $lte Operator
The $lte
operator is used to match values that are less than or equal to a specified value. It can be used in range queries to retrieve documents that fall within a specified range.
Here's an example of a range query using the $lte
operator to retrieve all documents with a field quantity
less than or equal to 10:
db.products.find({ quantity: { $lte: 10 } })
This query will return all documents in the products
collection where the quantity
field is less than or equal to 10.
You can also use the $lte
operator with other query operators, such as $and
and $or
, to create more complex range queries. For example, to retrieve documents with a field quantity
greater than or equal to 5 and less than or equal to 10, you can use the following query:
db.products.find({ $and: [{ quantity: { $gte: 5 } }, { quantity: { $lte: 10 } }] })
This query will return all documents in the products
collection where the quantity
field is greater than or equal to 5 and less than or equal to 10.
Querying MongoDB for Values within a Specific Range
You can also query for values within a specific range by combining the $gte
and $lte
operators. This allows you to retrieve documents that fall within a specified range of values.
Here's an example of a range query to retrieve all documents with a field score
between 80 and 90:
db.students.find({ score: { $gte: 80, $lte: 90 } })
This query will return all documents in the students
collection where the score
field is greater than or equal to 80 and less than or equal to 90.
You can also use the $and
operator to combine multiple range queries. For example, to retrieve documents with a field price
between 100 and 200 and a field quantity
between 5 and 10, you can use the following query:
db.products.find({ $and: [{ price: { $gte: 100, $lte: 200 } }, { quantity: { $gte: 5, $lte: 10 } }] })
This query will return all documents in the products
collection where the price
field is between 100 and 200, and the quantity
field is between 5 and 10.
Related Article: How to Improve the Speed of MongoDB Queries
Using Range Queries on Dates
MongoDB supports range queries on dates, allowing you to retrieve documents that fall within a specified range of dates. Date range queries can be useful when working with time-based data, such as event logs or sensor readings.
Here's an example of a range query to retrieve all documents with a field date
between two specific dates:
db.events.find({ date: { $gte: ISODate("2022-01-01"), $lte: ISODate("2022-01-31") } })
This query will return all documents in the events
collection where the date
field is greater than or equal to January 1, 2022, and less than or equal to January 31, 2022.
You can also use other date operators, such as $gt
and $lt
, to create more specific date range queries. For example, to retrieve documents with a field date
greater than January 1, 2022, and less than January 31, 2022, you can use the following query:
db.events.find({ date: { $gt: ISODate("2022-01-01"), $lt: ISODate("2022-01-31") } })
This query will return all documents in the events
collection where the date
field is greater than January 1, 2022, and less than January 31, 2022.
Performing Range Queries on Multiple Fields
You can perform range queries on multiple fields by combining the $and
operator with multiple range conditions. This allows you to retrieve documents that fall within a specified range of values for multiple fields.
Here's an example of a range query to retrieve all documents with a field price
between 100 and 200 and a field quantity
between 5 and 10:
db.products.find({ $and: [{ price: { $gte: 100, $lte: 200 } }, { quantity: { $gte: 5, $lte: 10 } }] })
This query will return all documents in the products
collection where the price
field is between 100 and 200, and the quantity
field is between 5 and 10.
You can combine multiple range queries on different fields using the $and
operator to create more complex range conditions. For example, to retrieve documents with a field age
between 18 and 30 and a field income
between 50000 and 100000, you can use the following query:
db.users.find({ $and: [{ age: { $gte: 18, $lte: 30 } }, { income: { $gte: 50000, $lte: 100000 } }] })
This query will return all documents in the users
collection where the age
field is between 18 and 30, and the income
field is between 50000 and 100000.
Additional Resources
- MongoDB