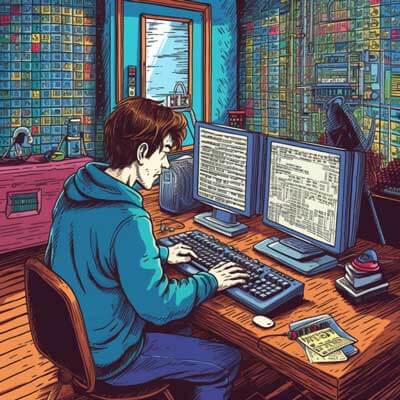
Table of Contents
Overview of the Max Function
The max
function in Python is a built-in function that allows us to find the maximum value among a set of values. It takes either an iterable or a series of arguments and returns the largest item. The max
function can be used with different data types, including numbers, strings, and even custom objects.
Let's start by looking at some basic examples of how the max
function works:
numbers = [5, 2, 9, 10, 3] max_number = max(numbers) print(max_number) # Output: 10 words = ['apple', 'banana', 'cherry', 'date'] max_word = max(words) print(max_word) # Output: cherry
In the first example, we have a list of numbers, and the max
function returns the largest number in the list, which is 10. In the second example, we have a list of words, and the max
function returns the word that comes last alphabetically, which is "cherry".
Related Article: How To Use Matplotlib Inline In Python
Handling Function Arguments in Python
When using the max
function, we can pass either an iterable or a series of arguments. If we pass an iterable, such as a list or a tuple, the max
function will iterate over the elements and return the maximum value. If we pass multiple arguments, the max
function will compare all the arguments and return the maximum value.
Let's see some examples to understand how the max
function handles different types of arguments:
numbers = [5, 2, 9, 10, 3] max_number = max(*numbers) print(max_number) # Output: 10 max_number = max(5, 2, 9, 10, 3) print(max_number) # Output: 10 words = ('apple', 'banana', 'cherry', 'date') max_word = max(*words) print(max_word) # Output: cherry max_word = max('apple', 'banana', 'cherry', 'date') print(max_word) # Output: cherry
In the first example, we pass the numbers
list as arguments by unpacking it using the *
operator. The max
function treats each element of the list as a separate argument and returns the maximum value. The same result is obtained when passing the list directly as an argument.
In the second set of examples, we pass the words
tuple as arguments by unpacking it using the *
operator. Again, the max
function treats each element as a separate argument and returns the maximum value. The same result is obtained when passing the tuple directly as an argument.
Function Return Value in Python
The max
function in Python returns the maximum value found among the given arguments or iterable. If all the elements are comparable and have the same type, the max
function will return the maximum value of that type. If the elements are of different types, the max
function will raise a TypeError
with a message indicating that the elements are not comparable.
Let's see some examples to understand the return value of the max
function:
numbers = [5, 2, 9, 10, 3] max_number = max(numbers) print(max_number) # Output: 10 print(type(max_number)) # Output: <class 'int'> words = ['apple', 'banana', 'cherry', 'date'] max_word = max(words) print(max_word) # Output: cherry print(type(max_word)) # Output: <class 'str'> mixed = [5, 'banana', 9.8, 'date'] max_value = max(mixed) # Raises TypeError
In the first example, the numbers
list consists of integers, so the max
function returns the maximum integer value, which is 10. The same applies to the second example with the words
list.
In the third example, the mixed
list contains elements of different types, including integers, strings, and floats. Since these types are not comparable, the max
function raises a TypeError
with a message indicating that the elements are not comparable.
Measuring Function Performance in Python
Measuring the performance of a function is essential to understand its efficiency and to identify any potential bottlenecks. In Python, we can use the time
module to measure the execution time of a function.
Let's see an example of how to measure the performance of the max
function:
import time numbers = [5, 2, 9, 10, 3] start_time = time.time() max_number = max(numbers) end_time = time.time() execution_time = end_time - start_time print(f"Execution time: {execution_time} seconds")
In this example, we import the time
module and store the current time in the start_time
variable before calling the max
function. After the function call, we store the current time in the end_time
variable. By subtracting the start_time
from the end_time
, we get the execution time of the max
function.
Related Article: How to Do Numeric Operations in Python
Assessing Function Complexity in Python
The complexity of a function refers to how its execution time or resource usage grows as the input size increases. Assessing the complexity of a function helps us understand its scalability and efficiency.
For the max
function, the complexity is O(n), where n is the number of elements in the input. This means that as the number of elements increases, the execution time of the max
function will increase linearly.
Optimizing Python Functions for Performance
To optimize the performance of a Python function, we can make use of various techniques. Here are some tips to optimize the max
function:
1. Use the key
parameter: The max
function allows us to specify a key
parameter, which is a function that takes an element as input and returns a value used for comparison. By using the key
parameter, we can optimize the comparison process and make the max
function more efficient.
numbers = [5, 2, 9, 10, 3] max_number = max(numbers, key=lambda x: -x) print(max_number) # Output: 2
In this example, we use a lambda function as the key
parameter to reverse the comparison order. This allows us to find the minimum value instead of the maximum value.
2. Sort the input: If the input is already sorted, we can optimize the max
function by accessing the last element directly. This eliminates the need for iterating over all the elements to find the maximum value.
numbers = [2, 3, 5, 9, 10] max_number = numbers[-1] print(max_number) # Output: 10
In this example, the numbers
list is already sorted in ascending order. By accessing the last element of the list directly, we can find the maximum value without iterating over the entire list.
3. Use a custom comparison function: If the elements are complex objects and the default comparison behavior is not suitable, we can define a custom comparison function to optimize the max
function.
class Person: def __init__(self, name, age): self.name = name self.age = age people = [Person('Alice', 25), Person('Bob', 30), Person('Charlie', 20)] max_person = max(people, key=lambda p: p.age) print(max_person.name) # Output: Bob
In this example, we have a list of Person
objects, and we want to find the person with the highest age. By using a lambda function as the key
parameter, we can define a custom comparison based on the age
attribute of each Person
object.
Best Practices for Handling Function Arguments in Python
When handling function arguments in Python, it is important to follow some best practices to ensure code readability and maintainability:
1. Use meaningful variable names: Choose descriptive variable names that accurately represent the purpose of each argument. This makes the code more readable and easier to understand.
2. Use default values for optional arguments: If a function has optional arguments, provide sensible default values for them. This allows users of the function to omit those arguments if they are not needed.
3. Document the function signature: Use docstrings to document the purpose, arguments, and return value of the function. This helps other developers understand how to use the function correctly.
4. Use type hints: Python 3.5 introduced type hints, which allow you to annotate the types of function arguments and return values. Type hints improve code readability and can help catch type-related bugs early.
Additional Resources
- Optimizing Python code