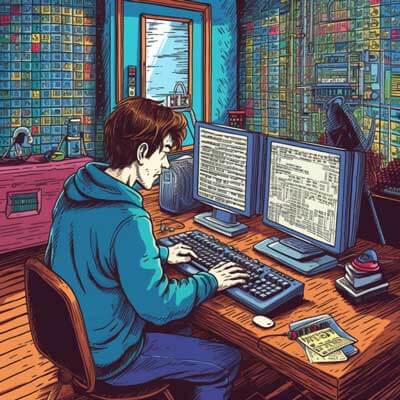
Table of Contents
Understanding the concept of URL parameters
URL parameters, also known as route parameters or path parameters, are a way to pass data to a web page or application through the URL. They are used to customize the content or behavior of a page based on the values provided in the URL.
URL parameters are typically denoted by a colon (:) followed by the parameter name in the URL path. For example, in the URL /users/:id, ":id" is a URL parameter that can be replaced with a specific value when accessing the page.
URL parameters are commonly used in web applications to provide dynamic content or to navigate to specific pages. For example, in an e-commerce website, the URL /products/:productId could be used to display detailed information about a specific product.
Let's take a closer look at how URL parameters can be handled in a ReactJS component using the React Router library.
Related Article: How to Style Components in ReactJS
Exploring the React Router library for handling URL parameters
React Router is a popular library for handling routing in React applications. It provides a declarative way to define routes and navigate between different components based on the current URL.
React Router offers several features for handling URL parameters, including the ability to define dynamic routes with parameters and access those parameters within React components.
To get started with React Router, you first need to install it in your project. You can do this by running the following command:
npm install react-router-dom
Once React Router is installed, you can import the necessary components and functions from the library to define your routes and handle URL parameters.
Example 1: Defining routes with URL parameters
To define a route with a URL parameter, you can use the Route
component from React Router and specify the parameter name in the path. Here's an example:
import { Route } from 'react-router-dom';function App() { return ( <div> <Route path="/users/:id" component={UserDetails} /> </div> );}
In this example, the Route
component is used to define a route for the path "/users/:id". The ":id" part is the URL parameter, which can be any value.
Example 2: Accessing URL parameters in a React component
Once you have defined a route with a URL parameter, you can access the value of the parameter within the corresponding React component. React Router provides different methods for accessing URL parameters, depending on the version of React Router you are using.
In React Router v5, you can use the useParams
hook to retrieve URL parameters. Here's an example:
import { useParams } from 'react-router-dom';function UserDetails() { const { id } = useParams(); return ( <div> <h2>User Details</h2> <p>ID: {id}</p> </div> );}
In this example, the useParams
hook is used to retrieve the value of the "id" URL parameter. The value is then displayed in the component.
It's important to note that the useParams
hook can only be used within a component that is rendered inside a Route
component.
Related Article: How to Build Forms in React
Differentiating between URL parameters and query parameters in React
In addition to URL parameters, there is another type of parameter that can be passed through a URL called query parameters. While URL parameters are used to customize the content or behavior of a page, query parameters are used to provide additional information to a page or application.
Query parameters are typically denoted by a question mark (?) followed by key-value pairs in the URL. For example, in the URL /search?q=react, "q" is the query parameter key and "react" is the value.
In React, URL parameters and query parameters can be handled differently. URL parameters are part of the URL path and can be accessed using React Router's useParams
hook, as shown in the previous example.
On the other hand, query parameters are separate from the URL path and can be accessed using the URLSearchParams
API or a third-party library like query-string
.
Example 1: Accessing query parameters using URLSearchParams
The URLSearchParams
API provides a convenient way to access and manipulate query parameters in JavaScript. Here's an example:
import { useLocation } from 'react-router-dom';function SearchResults() { const location = useLocation(); const searchParams = new URLSearchParams(location.search); const query = searchParams.get('q'); return ( <div> <h2>Search Results</h2> <p>Query: {query}</p> </div> );}
In this example, the useLocation
hook is used to get the current location object, which contains the query string. The URLSearchParams
constructor is then used to create a new instance of URLSearchParams
with the query string. Finally, the get
method is used to retrieve the value of the "q" query parameter.
Example 2: Accessing query parameters using query-string library
If you prefer to use a third-party library, you can use query-string
to parse and stringify query parameters in React. Here's an example:
import queryString from 'query-string';import { useLocation } from 'react-router-dom';function SearchResults() { const location = useLocation(); const queryParams = queryString.parse(location.search); const query = queryParams.q; return ( <div> <h2>Search Results</h2> <p>Query: {query}</p> </div> );}
In this example, the useLocation
hook is used to get the current location object. The queryString.parse
method from the query-string
library is then used to parse the query string into an object. Finally, the value of the "q" query parameter is accessed from the parsed object.
Handling URL parameters in React Router
React Router provides various features for handling and manipulating URL parameters within your application. These features include setting default values for URL parameters, specifying parameter types, and handling optional parameters.
Let's explore some of the ways you can handle URL parameters in React Router.
Related Article: How to Implement Reactstrap in ReactJS
Example 1: Setting default values for URL parameters
import { Route } from 'react-router-dom';function App() { return ( <div> <Route path="/users/:id?" component={UserDetails} /> </div> );}
In this example, the ?
character after the URL parameter name makes it optional. If the URL parameter is not present in the URL, React Router will still match the route and pass an empty string as the parameter value.
Example 2: Specifying parameter types in URL parameters
You can specify the type of a URL parameter by using regular expressions in the route path. This allows you to enforce specific patterns or constraints on the parameter value. Here's an example:
import { Route } from 'react-router-dom';function App() { return ( <div> <Route path="/users/:id(\d+)" component={UserDetails} /> </div> );}
In this example, the (\d+)
part of the route path specifies that the "id" parameter must be a numeric value. React Router will only match the route if the URL parameter matches the specified pattern.
Retrieving the value of a specific URL parameter in React
To retrieve the value of a specific URL parameter in React, you can use the useParams
hook from React Router. The useParams
hook returns an object containing all the URL parameters defined in the route.
To access a specific URL parameter, you can use dot notation or bracket notation. Here's an example:
import { useParams } from 'react-router-dom';function UserDetails() { const { id } = useParams(); return ( <div> <h2>User Details</h2> <p>ID: {id}</p> </div> );}
In this example, the useParams
hook is used to retrieve all the URL parameters defined in the route. Then, the value of the "id" parameter is accessed using dot notation ({id}
).
It's important to note that the parameter name used to access the value must match the name specified in the URL parameter definition.
Exploring built-in functions and hooks for accessing URL parameters in React
In addition to the useParams
hook, React Router provides other built-in functions and hooks for accessing and manipulating URL parameters in React components.
Related Article: How Rendering Works in ReactJS
Example 1: useLocation hook
The useLocation
hook from React Router returns the current location object, which contains information about the current URL. This can be useful for accessing the query string or other properties of the URL.
import { useLocation } from 'react-router-dom';function UserDetails() { const location = useLocation(); return ( <div> <h2>User Details</h2> <p>Current URL: {location.pathname}</p> </div> );}
In this example, the useLocation
hook is used to get the current location object. The pathname
property of the location object is then accessed to display the current URL path.
Example 2: useHistory hook
The useHistory
hook from React Router provides access to the browser's history object, which can be used to navigate programmatically or modify the browser's URL.
import { useHistory } from 'react-router-dom';function UserDetails() { const history = useHistory(); const handleClick = () => { history.push('/users'); }; return ( <div> <h2>User Details</h2> <button onClick={handleClick}>Go Back</button> </div> );}
In this example, the useHistory
hook is used to get the history object. The push
method of the history object is then used to navigate to the "/users" route when the button is clicked.
Directly accessing URL parameters in a React component
While React Router provides convenient hooks and functions for accessing URL parameters, there may be cases where you need to directly access the URL parameters without using React Router.
To directly access URL parameters in a React component, you can use the window.location
object and parse the URL manually.
Example 1: Directly accessing a URL parameter
import React from 'react';function UserDetails() { const url = window.location.href; const params = new URL(url).searchParams; const id = params.get('id'); return ( <div> <h2>User Details</h2> <p>ID: {id}</p> </div> );}
In this example, the window.location.href
property is used to get the current URL. Then, the URL
constructor is used to create a new URL object from the URL string. Finally, the searchParams
property of the URL object is used to access the query parameters, and the get
method is used to retrieve the value of the "id" parameter.
Related Article: How To Develop a Full Application with ReactJS
Using the URL query string to retrieve query parameters in ReactJS
In addition to URL parameters, query parameters can also be used to pass data to a web page or application. The query parameters are appended to the URL after a question mark (?) and can be accessed using the URLSearchParams
API or a third-party library like query-string
.
Example 1: Accessing query parameters using URLSearchParams
import React from 'react';function SearchResults() { const url = window.location.href; const params = new URL(url).searchParams; const query = params.get('q'); return ( <div> <h2>Search Results</h2> <p>Query: {query}</p> </div> );}
In this example, the window.location.href
property is used to get the current URL. Then, the URL
constructor is used to create a new URL object from the URL string. Finally, the searchParams
property of the URL object is used to access the query parameters, and the get
method is used to retrieve the value of the "q" query parameter.
Example 2: Accessing query parameters using query-string library
import React from 'react';import queryString from 'query-string';function SearchResults() { const url = window.location.href; const queryParams = queryString.parseUrl(url).query; const query = queryParams.q; return ( <div> <h2>Search Results</h2> <p>Query: {query}</p> </div> );}
In this example, the window.location.href
property is used to get the current URL. Then, the queryString.parseUrl
method from the query-string
library is used to parse the URL and extract the query parameters. Finally, the value of the "q" query parameter is accessed from the parsed object.
Leveraging the React Router library to get URL parameters
React Router provides a convenient way to handle URL parameters in React components. By using the useParams
hook, you can easily retrieve and use URL parameters in your components.
Related Article: How to Update a State Property in ReactJS
Example 1: Retrieving URL parameters using useParams hook
import React from 'react';import { useParams } from 'react-router-dom';function UserDetails() { const { id } = useParams(); return ( <div> <h2>User Details</h2> <p>ID: {id}</p> </div> );}
In this example, the useParams
hook from React Router is used to retrieve the value of the "id" URL parameter. The value is then displayed in the component.
Best practices for accessing and handling URL parameters in React
When working with URL parameters in React, it's important to follow some best practices to ensure a clean and maintainable codebase. Here are some best practices for accessing and handling URL parameters in React:
1. Use meaningful parameter names: Choose descriptive names for your URL parameters to make your code easier to understand and maintain.
2. Handle missing or invalid parameters: Check if the required URL parameters are present and handle cases where they are missing or invalid. You can display an error message or redirect the user to a default page.
3. Sanitize and validate parameter values: If the parameter values are used in database queries or API calls, make sure to sanitize and validate them to prevent security vulnerabilities such as SQL injection or cross-site scripting (XSS).
4. Use type checking: If your application relies on specific data types for URL parameters, consider using type-checking libraries like PropTypes or TypeScript to enforce type safety and prevent runtime errors.
5. Separate concerns: If your components have complex logic for handling URL parameters, consider extracting that logic into separate functions or hooks to keep your components clean and focused.
6. Test your code: Write unit tests to ensure that your code handles URL parameters correctly and that it behaves as expected in different scenarios.
Implementing code snippets to extract URL parameters in React components
Here are some code snippets that demonstrate how to extract URL parameters in React components using the React Router library:
import React from 'react';import { useParams, useLocation, useHistory } from 'react-router-dom';import queryString from 'query-string';function UserDetails() { const { id } = useParams(); const location = useLocation(); const searchParams = new URLSearchParams(location.search); const query = searchParams.get('q'); const history = useHistory(); const handleClick = () => { history.push('/users'); }; const url = window.location.href; const params = new URL(url).searchParams; const otherParam = params.get('other'); const queryParams = queryString.parseUrl(url).query; const otherQuery = queryParams.other; return ( <div> <h2>User Details</h2> <p>ID: {id}</p> <p>Query: {query}</p> <button onClick={handleClick}>Go Back</button> <p>Other Param: {otherParam}</p> <p>Other Query: {otherQuery}</p> </div> );}
In this example, the useParams
hook is used to retrieve the value of the "id" URL parameter. The useLocation
hook is used to get the current location object and extract the query parameter using the URLSearchParams
API. The useHistory
hook is used to navigate programmatically when the button is clicked. Finally, the window.location
object and the query-string
library are used to directly access the URL parameters and query parameters.
Additional Resources
- Retrieving URL Parameters in React Router