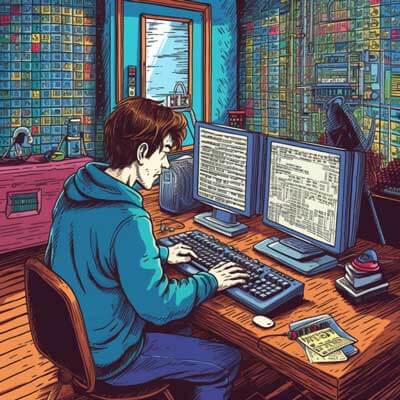
Table of Contents
Overview of File Not Found Errors
When working with file operations in Python, it is common to encounter a "File Not Found" error. This error occurs when the code attempts to access a file that does not exist in the specified path. It is important to understand the causes of this error and how to handle it effectively.
Related Article: How to Check If Something Is Not In A Python List
Handling File Not Found Errors with Exception Handling
Python provides a built-in exception handling mechanism to catch and handle errors, including the "File Not Found" error. By using the try-except block, we can handle the error gracefully and prevent our program from crashing.
Here is an example of how to handle the "File Not Found" error using exception handling:
try: file = open("myfile.txt", "r") # Perform file operations here except FileNotFoundError: print("File not found!")
In this example, we try to open a file named "myfile.txt" in read mode. If the file is not found, the code will raise a FileNotFoundError
exception, which we catch in the except block and display an appropriate error message.
Debugging File Not Found Error
When encountering a "File Not Found" error, it is essential to debug and identify the root cause of the issue. There are several steps you can take to debug this error effectively:
1. Verify the file path: Double-check the file path to ensure it is correct. Pay attention to any typos or missing directories in the path.
2. Check file permissions: Ensure that the file has the necessary read or write permissions. If the file is read-only or inaccessible due to permissions, it can result in a "File Not Found" error.
3. Test with a different file: Try accessing a different file in the same directory or location to determine if the issue is specific to a particular file or the entire directory.
4. Use print statements: Insert print statements in your code to track the file path or any variables that may impact the file access.
File Paths in File Not Found Error
Understanding file paths is crucial when encountering a "File Not Found" error. A file path is a string that represents the location of a file in the file system. In Python, file paths can be specified using relative or absolute paths.
- Relative paths: Relative paths are defined relative to the current working directory. For example, if the current working directory is /home/user/
, a relative path myfile.txt
will refer to /home/user/myfile.txt
.
- Absolute paths: Absolute paths specify the complete path from the root directory. For instance, /home/user/myfile.txt
is an absolute path that points directly to the file.
It is important to ensure the accuracy of file paths to avoid "File Not Found" errors. Verify the correctness of the file path, including spelling, capitalization, and the presence of any special characters.
Related Article: How to Handle Cookies and Cookie Outputs in Python
File Access and File Not Found Error
The "File Not Found" error can occur due to various reasons related to file access. Here are some scenarios that can lead to this error:
1. File deletion: If the file you are trying to access has been deleted from the file system, you will encounter a "File Not Found" error.
2. Incorrect file name: Make sure the file name you are using is correct. Even a single character difference in the file name can result in the error.
3. File moved or renamed: If the file has been moved to a different location or renamed, the previous file path will no longer be valid, resulting in a "File Not Found" error.
4. File permissions: If the file is located in a directory with restricted permissions, you may encounter a "File Not Found" error if you do not have the necessary access rights.
Best Practices
To avoid "File Not Found" errors and ensure smooth file handling in Python, it is recommended to follow these best practices:
1. Check file existence: Before performing any file operations, check if the file exists using the os.path.exists()
function. This helps to prevent "File Not Found" errors.
2. Use absolute file paths: Whenever possible, use absolute file paths to avoid any ambiguity in file locations.
3. Handle exceptions gracefully: Implement exception handling to catch and handle errors, including the "File Not Found" error. This prevents your program from crashing and allows for better error handling.
4. Close files after use: Always close files using the file.close()
method or by using the with
statement. This ensures that system resources are released properly and prevents potential file access issues.
5. Use context managers: Utilize context managers, such as the with
statement, for file operations. This ensures that files are automatically closed even if an exception occurs.
Interpreting Error Messages for File Not Found Exception
When encountering a "File Not Found" error, Python provides detailed error messages to help pinpoint the issue. These error messages can provide valuable information for debugging and resolving the error.
Here is an example of a typical error message for a "File Not Found" exception:
FileNotFoundError: [Errno 2] No such file or directory: 'myfile.txt'
Let's break down this error message:
- FileNotFoundError
is the specific exception class raised when a file is not found.
- [Errno 2]
indicates the error number associated with the exception. In this case, it represents a "No such file or directory" error.
- 'myfile.txt'
is the file name or path that could not be found.
Strategies for Dealing with File Not Found Error
When dealing with a "File Not Found" error, there are several strategies you can employ to handle the issue effectively:
1. Error handling: Implement exception handling to catch the "File Not Found" error and provide a graceful error message to the user. This prevents the program from crashing and allows for better error handling.
2. User input validation: If the file path is provided by user input, perform validation to ensure the path is correct and the file exists before attempting to access it.
3. Default file handling: If a file is expected to exist but is not found, consider providing a default file or a fallback mechanism to handle the situation gracefully.
4. Logging and error tracking: Implement logging and error tracking mechanisms to record the occurrence of "File Not Found" errors. This helps in identifying patterns and resolving recurring issues.
Related Article: How to Detect Duplicates in a Python List
Functionality of 'os' Module in Handling File Not Found Error
The os
module in Python provides various functions and methods to handle file operations, including the "File Not Found" error. Here are some key functionalities of the os
module in relation to handling this error:
1. os.path.exists()
: This function checks if a file or directory exists at the specified path. By using this function, you can verify the existence of a file before attempting to access it, thus avoiding the "File Not Found" error.
2. os.getcwd()
: This function returns the current working directory as a string. It can be useful for determining the base path for relative file paths.
3. os.path.join()
: This function joins one or more path components together to create a valid file path. It handles the correct formatting of the path based on the operating system.
4. os.makedirs()
: This function creates directories recursively, including any missing intermediate directories. It can be helpful when handling file paths that include directories that do not exist yet.
Common Mistakes Leading to File Not Found Errors
While working with file operations in Python, certain common mistakes can lead to "File Not Found" errors. It is important to be aware of these mistakes to avoid them. Here are some common mistakes that can cause this error:
1. Incorrect file path: Providing an incorrect file path is a common mistake that can result in a "File Not Found" error. Double-check the path, including the spelling, capitalization, and the presence of special characters.
2. Missing file extension: For files that require specific extensions (e.g., .txt
, .csv
), forgetting to include the extension in the file path can lead to a "File Not Found" error.
3. Incorrect directory: If the file is located in a different directory than the one specified in the code, it will result in a "File Not Found" error. Ensure that the directory path is accurate.
4. File not closed properly: Failing to close a file after performing file operations can cause issues with subsequent file access. Make sure to close files using the file.close()
method or by utilizing the with
statement.
Best Practices for Error Handling in File Access
Effective error handling is crucial when working with file access in Python. Here are some best practices to follow when handling errors related to file access:
1. Implement exception handling: Use try-except blocks to catch and handle specific exceptions, such as the "File Not Found" error. This allows you to handle errors gracefully and provide meaningful error messages to the user.
2. Provide informative error messages: When an error occurs, provide clear and informative error messages that describe the issue and suggest possible solutions. This helps users understand the problem and take appropriate action.
3. Log errors: Implement logging mechanisms to record errors encountered during file access. This helps in troubleshooting and identifying recurring issues.
4. Use context managers: Utilize context managers, such as the with
statement, for file operations. Context managers automatically handle resource management, including file closing, even in the presence of exceptions.
5. Test error scenarios: When developing file access code, include test cases that simulate error scenarios, such as file not found or permission denied. This helps to identify and address potential issues before deployment.
Additional Resources
- Understanding the FileNotFoundError Exception in Python