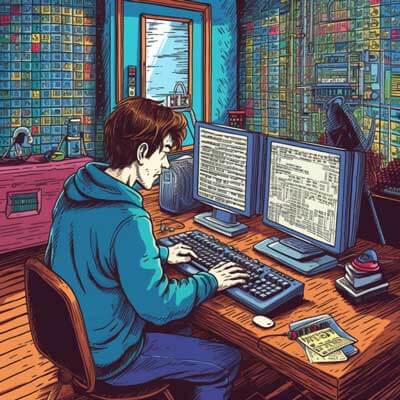
Table of Contents
Syntax Error and Its Impact on Code Execution
Syntax errors occur when the code violates the rules of the Python programming language. These errors prevent the code from being executed and can result in unexpected behavior or program crashes. One common type of syntax error in Python is the indentation error.
Related Article: Implementing a cURL command in Python
Code Formatting Best Practices
Proper code formatting is essential for writing clean and maintainable code. It not only improves code readability but also helps in preventing syntax errors. Here are some best practices for code formatting in Python:
1. Use consistent indentation: Python relies on indentation to define blocks of code. It is important to use a consistent indentation style, such as using four spaces or a tab, to make the code more readable.
2. Limit line length: PEP 8, the official style guide for Python code, recommends keeping lines of code up to 79 characters long. This makes the code more readable and prevents horizontal scrolling.
3. Use meaningful variable and function names: Descriptive names for variables and functions make the code easier to understand. Avoid using single-letter variable names and choose names that accurately describe the purpose of the variable or function.
4. Add comments: Comments help explain the logic and purpose of the code. Use comments to document complex or tricky parts of the code to make it easier for others to understand and maintain.
Deciphering Error Messages in Python
When encountering an error in Python, it is important to understand the error message to diagnose and fix the issue. Error messages in Python provide valuable information about the type of error and its location in the code.
Here's an example of an indentation error and its corresponding error message:
def print_hello(): print("Hello, World!")
Error message:
IndentationError: expected an indented block
The error message indicates that an indented block was expected after the line with the print_hello()
function definition. This error usually occurs when the indentation of the code is incorrect.
Using the Python Interpreter for Troubleshooting
The Python interpreter can be a useful tool for troubleshooting and debugging code. It allows you to interactively execute Python code and quickly test different scenarios. To use the Python interpreter, open the terminal or command prompt and type python
to start the interactive mode.
Here's an example of using the Python interpreter to troubleshoot an indentation error:
def print_hello(): print("Hello, World!")
In the Python interpreter, you would see the following error message:
File "<stdin>", line 2 print("Hello, World!") ^ IndentationError: expected an indented block
The error message indicates the line number and the type of error. By entering the code in the interpreter, you can quickly identify the issue and fix the indentation error.
Related Article: Python Operators Tutorial & Advanced Examples
Whitespace in Python Code
Whitespace refers to spaces, tabs, and line breaks in Python code. In Python, whitespace is significant and is used to define the structure and readability of the code. Indentation, which is a form of whitespace, is particularly important in Python as it determines the scope and hierarchy of code blocks.
Here are some key points to remember about whitespace in Python:
- Indentation: Python uses indentation to define blocks of code. A consistent indentation style, such as using four spaces, is recommended for readability.
- Tabs vs Spaces: While both tabs and spaces can be used for indentation, it is generally recommended to use spaces for consistency and to avoid any potential issues with different text editors or IDEs.
- Trailing Whitespace: Trailing whitespace, which refers to spaces or tabs at the end of a line, should be avoided as it can cause syntax errors.
- Line Breaks: Line breaks are used to separate statements and improve readability. Python allows line breaks within parentheses, brackets, or braces, which can be useful for long or complex expressions.
Strategies for Resolving Indentation Errors in Python
Indentation errors can be frustrating to deal with, but with a systematic approach, they can be easily resolved. Here are some strategies to fix indentation errors in Python:
1. Check for consistent indentation: Make sure that the indentation is consistent throughout the code. In Python, a common convention is to use four spaces for indentation. Avoid mixing spaces and tabs for indentation as it can lead to errors.
2. Use an IDE or text editor with automatic indentation: IDEs and text editors often have built-in features that automatically handle indentation. Use these features to ensure consistent and correct indentation.
3. Review the error message and traceback: When encountering an indentation error, carefully read the error message and traceback to identify the line of code where the error occurred. This can help pinpoint the location and cause of the error.
4. Compare with working code: If you have a working version of the code, compare it with the code that is causing the indentation error. Look for any differences in indentation and make the necessary adjustments.
5. Use code linters and formatters: Code linters and formatters, such as Pylint or Black, can automatically check and fix indentation errors. Integrate these tools into your development workflow to catch and correct indentation issues early on.
Identifying Causes of Indentation Errors in Python
Indentation errors can be caused by various factors. Here are some common causes to watch out for:
1. Mixing spaces and tabs: Mixing spaces and tabs for indentation can lead to indentation errors. Stick to one consistent style, such as using four spaces, to avoid this issue.
2. Incorrect nesting: Indentation errors can occur when the nesting of code blocks is incorrect. Make sure that each block is properly indented within its parent block.
3. Missing or extra indentation: Missing or extra indentation can cause indentation errors. Check for any missing or extra spaces or tabs at the beginning of lines to ensure proper indentation.
4. Mismatched indentation levels: Indentation errors can also occur when the indentation levels do not match. For example, if a line of code is indented more or less than expected, it can result in an indentation error.
Python's Handling of Indentation Errors
Python's handling of indentation errors is strict. Unlike some other programming languages, Python relies on proper indentation to define the structure and hierarchy of code blocks. Indentation errors in Python code will result in a syntax error and prevent the code from being executed.
When encountering an indentation error, Python will raise an IndentationError
and provide an error message indicating the line number and the type of error. This helps in identifying and resolving the indentation error quickly.
Related Article: How to Append to a Dict in Python
Additional Resources
- Understanding Indentation in Python