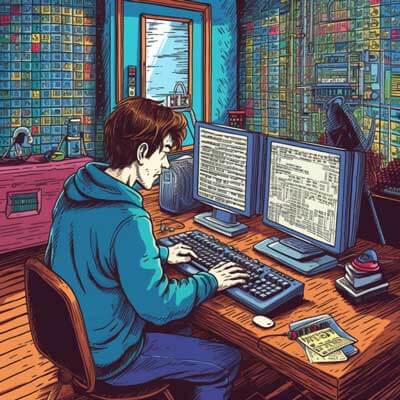
Table of Contents
Overview of GPU Acceleration with PyTorch
PyTorch is a popular open-source deep learning framework that allows developers to build and train neural networks. One of the key advantages of PyTorch is its ability to leverage the power of GPUs to accelerate computations, making it an excellent choice for training deep neural networks.
GPU acceleration refers to the use of a graphics processing unit (GPU) to perform computations in parallel, which can significantly speed up the training process for deep learning models. GPUs are designed to handle large amounts of data and perform parallel computations efficiently, making them ideal for the computationally intensive tasks involved in training neural networks.
PyTorch provides seamless integration with GPUs, allowing developers to easily take advantage of their computational power. By utilizing GPUs, developers can train models faster, enabling them to iterate and experiment more quickly, leading to faster development cycles and better results.
Related Article: Creating Custom Datasets and Dataloaders in PyTorch
Efficient Tensor Operations with GPU Acceleration
In PyTorch, tensors are the fundamental data structure used to store and manipulate data. Tensors can be thought of as multi-dimensional arrays, similar to matrices, but with support for higher dimensions. GPU acceleration in PyTorch enables efficient execution of tensor operations, such as matrix multiplication, element-wise operations, and reduction operations.
To perform tensor operations on a GPU, you first need to ensure that your data is stored on the GPU. This can be done by explicitly moving tensors to the GPU using the .to()
method. For example, to move a tensor x
to the GPU, you can use the following code:
import torch # Create a tensor on the CPU x = torch.tensor([1, 2, 3]) # Move the tensor to the GPU x = x.to('cuda')
Once the tensor is on the GPU, you can perform operations on it as you would with CPU tensors. PyTorch will automatically use the GPU to execute these operations, resulting in faster computation times.
It's important to note that not all operations are supported on the GPU. PyTorch provides a comprehensive list of supported operations in its documentation. If an operation is not supported on the GPU, PyTorch will automatically move the data back to the CPU to perform the operation and then move it back to the GPU.
Using Parallel Processing with PyTorch for GPU Acceleration
Parallel processing is a technique that involves dividing a task into smaller subtasks that can be executed simultaneously on multiple processors or cores. PyTorch allows developers to leverage parallel processing to further accelerate computations on GPUs.
PyTorch provides a feature called DataParallel, which allows you to parallelize the execution of a model across multiple GPUs. With DataParallel, you can split your input data across multiple GPUs, process them in parallel, and then combine the results. This can significantly speed up the training process, especially for large models or datasets.
To use DataParallel, you first need to wrap your model with the torch.nn.DataParallel
module. This module automatically splits the input data across available GPUs and executes the model in parallel. Here's an example of how to use DataParallel:
import torch import torch.nn as nn # Create a model model = nn.Linear(10, 1) # Wrap the model with DataParallel model = nn.DataParallel(model) # Move the model to the GPU model = model.to('cuda') # Perform forward pass on the model input_data = torch.randn(32, 10).to('cuda') output = model(input_data)
Managing GPU Memory for Deep Learning Tasks
When working with GPUs, it's important to manage GPU memory efficiently to avoid running out of memory and potential performance issues. PyTorch provides several mechanisms to help manage GPU memory effectively.
First, you can use the torch.cuda.empty_cache()
function to release all unused memory held by the GPU cache. This can be useful when you want to clear the GPU memory after completing a specific task or when you encounter an out-of-memory error.
import torch # Clear the GPU memory torch.cuda.empty_cache()
Additionally, PyTorch provides the torch.cuda.max_memory_allocated()
and torch.cuda.max_memory_cached()
functions to track the maximum amount of memory allocated and cached on the GPU, respectively. These functions can be useful for monitoring memory usage during training or debugging memory-related issues.
import torch # Track maximum memory allocated max_allocated = torch.cuda.max_memory_allocated() # Track maximum memory cached max_cached = torch.cuda.max_memory_cached()
You can also control the memory allocation behavior of PyTorch by using the torch.cuda.set_device()
function to specify the GPU device to be used or the torch.cuda.set_enabled_lms()
function to enable or disable the use of the GPU.
import torch # Set the GPU device torch.cuda.set_device(0) # Enable or disable the use of the GPU torch.cuda.set_enabled_lms(True)
Related Article: An Introduction to PyTorch
Neural Network Training with GPU Acceleration
PyTorch provides useful capabilities for training neural networks on GPUs, enabling faster and more efficient training. GPU acceleration allows you to take full advantage of the parallel processing capabilities of GPUs, speeding up the computation of forward and backward passes during training.
To train a neural network on a GPU, you need to ensure that both the model parameters and the input data are on the GPU. This can be done by moving the model to the GPU using the .to()
method and moving the input data to the GPU using the .to()
method or by passing the device
argument when creating the tensors.
Here's an example of how to train a neural network on a GPU:
import torch import torch.nn as nn import torch.optim as optim # Create a model model = nn.Linear(10, 1) # Move the model to the GPU model = model.to('cuda') # Create a loss function and an optimizer criterion = nn.MSELoss() optimizer = optim.SGD(model.parameters(), lr=0.01) # Move the loss function to the GPU criterion = criterion.to('cuda') # Move the optimizer to the GPU optimizer = optimizer.to('cuda') # Train the model for epoch in range(10): # Move the input data to the GPU input_data = torch.randn(32, 10).to('cuda') target = torch.randn(32, 1).to('cuda') # Zero the gradients optimizer.zero_grad() # Forward pass output = model(input_data) # Compute the loss loss = criterion(output, target) # Backward pass loss.backward() # Update the weights optimizer.step()
Implementation of GPU Acceleration in PyTorch for Tensor Operations
PyTorch provides a straightforward implementation of GPU acceleration for tensor operations. By moving tensors to the GPU and performing operations on them, you can take advantage of the parallel processing capabilities of GPUs and speed up computations.
Here's an example of how to implement GPU acceleration in PyTorch for tensor operations:
import torch # Create tensors on the CPU x = torch.tensor([1, 2, 3]) y = torch.tensor([4, 5, 6]) # Move tensors to the GPU x = x.to('cuda') y = y.to('cuda') # Perform tensor operations on the GPU z = x + y
In this example, we create tensors x
and y
on the CPU and then move them to the GPU using the .to()
method. We then perform the tensor operation x + y
on the GPU, which effectively adds the corresponding elements of x
and y
together. The result z
is also stored on the GPU.
Advantages of GPU Acceleration for Neural Network Training in PyTorch
GPU acceleration offers several advantages for neural network training in PyTorch:
1. Faster Training: GPUs are designed for parallel processing and can perform multiple computations simultaneously. By utilizing GPU acceleration, you can speed up the training process, enabling you to train larger models and process larger datasets in less time.
2. Improved Efficiency: GPUs are highly efficient in performing complex computations, making them well-suited for the computationally intensive tasks involved in training deep neural networks. GPU acceleration allows you to take full advantage of the parallel processing capabilities of GPUs, maximizing the efficiency of your training process.
3. Better Model Iteration: With faster training times, you can iterate and experiment more quickly, enabling you to try out different architectures, hyperparameters, and training strategies. This iterative process can lead to faster development cycles and better results.
4. Support for Large Models and Datasets: Deep learning models are becoming increasingly complex and require significant computational resources. GPUs provide the necessary power to handle large models and process large datasets efficiently. With GPU acceleration, you can tackle more challenging deep learning tasks that may not be feasible on CPUs alone.
Overall, GPU acceleration in PyTorch provides a significant advantage for neural network training, allowing you to train models faster, improve efficiency, and tackle more complex deep learning tasks.
Efficient GPU Memory Management for Deep Learning Tasks in PyTorch
Efficient GPU memory management is crucial for deep learning tasks to prevent running out of memory and to optimize the utilization of GPU resources. PyTorch provides several techniques to manage GPU memory effectively.
1. Batch Processing: Processing data in smaller batches instead of processing the entire dataset at once can help reduce memory consumption. By using techniques such as mini-batch gradient descent, you can process a subset of the data in each iteration, allowing you to work with smaller tensors and reduce memory usage.
2. Data Parallelism: As mentioned earlier, PyTorch's DataParallel feature allows you to parallelize the execution of a model across multiple GPUs. This not only speeds up computations but also reduces memory usage by splitting the input data across GPUs.
3. Gradient Accumulation: Instead of updating the model weights after each batch, you can accumulate gradients over multiple batches and update the weights once. This can help reduce memory usage, especially when working with limited GPU memory.
4. Memory Clearing: PyTorch provides the torch.cuda.empty_cache()
function to release unused memory held by the GPU cache. Calling this function at appropriate times, such as after completing a specific task or encountering an out-of-memory error, can help free up GPU memory.
5. Memory Usage Tracking: PyTorch offers functions like torch.cuda.max_memory_allocated()
and torch.cuda.max_memory_cached()
to track the maximum amount of memory allocated and cached on the GPU, respectively. These functions can be used to monitor memory usage during training and identify any memory leaks or inefficiencies.