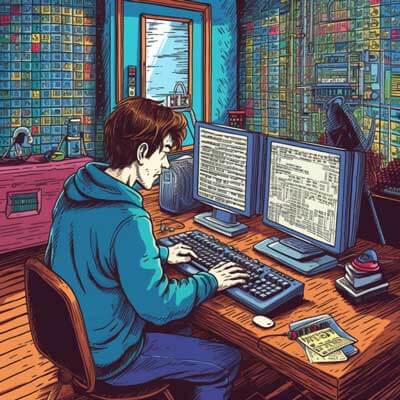
Table of Contents
Overview of Exporting Data Frame to SQL File
Exporting a Python data frame to an SQL file allows you to store and manipulate your data using the useful querying capabilities of SQL. Whether you need to share your data with a colleague, import it into a database, or simply keep a backup, exporting to an SQL file is a convenient and efficient option.
In this guide, we will explore how to export a Python data frame to an SQL file using the pandas and SQLAlchemy libraries. We will cover the installation process, creating a data frame, connecting to an SQL database, and the steps involved in exporting the data frame to an SQL file. We will also discuss important considerations such as managing data types, handling existing tables and indexes, and best practices for exporting data frames to SQL files.
Related Article: How to Replace Strings in Python using re.sub
Installing pandas and SQLAlchemy Libraries
Before we begin, we need to install two essential libraries: pandas and SQLAlchemy. Pandas is a useful data manipulation and analysis library, while SQLAlchemy provides a Pythonic way to interact with SQL databases.
To install pandas, open your terminal and run the following command:
pip install pandas
Next, we will install SQLAlchemy using the same method:
pip install sqlalchemy
With both libraries installed, we are now ready to start exporting our data frame to an SQL file.
Creating a Data Frame in pandas
To demonstrate the process of exporting a data frame to an SQL file, let's first create a simple data frame using pandas. Open your Python IDE or Jupyter Notebook and import the pandas library:
import pandas as pd
Next, let's create a data frame with some sample data. For this example, we will create a data frame representing sales data:
data = {'Product': ['A', 'B', 'C'], 'Price': [10, 20, 30], 'Quantity': [5, 10, 15]} df = pd.DataFrame(data)
Our data frame, df
, now contains three columns: 'Product', 'Price', and 'Quantity'. This serves as our starting point for exporting to an SQL file.
Connecting to an SQL Database with SQLAlchemy
Before we can export our data frame to an SQL file, we need to establish a connection to an SQL database using SQLAlchemy. SQLAlchemy provides a consistent and intuitive way to interact with various database engines.
To connect to an SQL database, we need to specify the database engine and the connection string. The connection string contains the necessary information to establish a connection, such as the database type, username, password, and host.
Here is an example of connecting to a SQLite database using SQLAlchemy:
from sqlalchemy import create_engine # Replace 'database_name.db' with the name of your SQLite database file engine = create_engine('sqlite:///database_name.db')
In this example, we create an engine object using the create_engine()
function from the SQLAlchemy library. We pass in the connection string, which specifies the SQLite database file we want to connect to. Replace 'database_name.db'
with the actual name of your SQLite database file.
If you are connecting to a different type of database, such as MySQL or PostgreSQL, you will need to modify the connection string accordingly. Refer to the SQLAlchemy documentation for the correct syntax.
Related Article: How to Add New Keys to a Python Dictionary
Code Snippet: Exporting Data Frame to SQL File using to_sql Method
Now that we have a data frame and a connection to an SQL database, we can proceed to export the data frame to an SQL file. The to_sql
method in pandas allows us to write the contents of a data frame to an SQL database.
Here is an example code snippet that demonstrates how to export a data frame to an SQL file:
# Export the data frame to an SQL file df.to_sql('sales', con=engine, if_exists='replace', index=False)
In this example, we call the to_sql
method on our data frame, df
. We pass in the following parameters:
- 'sales'
: The name of the table to be created in the SQL database.
- con=engine
: The connection object we created using SQLAlchemy's create_engine
function.
- if_exists='replace'
: Specifies what action to take if the table already exists in the database. In this case, we replace the existing table with the new data.
- index=False
: Specifies whether to include the row index as a separate column in the table. In this example, we exclude the index.
The Parameters of to_sql Method
The to_sql
method in pandas provides several parameters that allow us to customize the export process. Here are some important parameters to be aware of:
- name
: Specifies the name of the table to be created in the SQL database.
- con
: Specifies the connection object to the SQL database.
- if_exists
: Specifies what action to take if the table already exists in the database. Possible values are 'fail', 'replace', and 'append'.
- index
: Specifies whether to include the row index as a separate column in the table.
- dtype
: Specifies the data types for the columns in the SQL table. This parameter accepts a dictionary mapping column names to SQL data types.
It is important to note that the to_sql
method infers the data types of the columns in the data frame and attempts to map them to the closest SQL data types. However, there may be cases where the inferred data types do not match the desired SQL data types. We will discuss managing data types during export in the next section.
Managing Data Types During Export
When exporting a data frame to an SQL file, it is crucial to ensure that the data types of the columns in the data frame are compatible with the corresponding columns in the SQL table. Mismatched data types can lead to data loss or unexpected behavior when querying the SQL table.
Here is an example of specifying the data types for the columns during export:
# Define the desired data types for the columns dtype = {'Product': sqlalchemy.String, 'Price': sqlalchemy.Float, 'Quantity': sqlalchemy.Integer} # Export the data frame to an SQL file with the specified data types df.to_sql('sales', con=engine, if_exists='replace', index=False, dtype=dtype)
In this example, we define a dictionary called dtype
that maps column names to the desired SQL data types. We then pass this dictionary as the value for the dtype
parameter of the to_sql
method.
Handling Existing Tables and Indexes
When exporting a data frame to an SQL file, it is important to consider the presence of existing tables and indexes in the SQL database. The if_exists
parameter of the to_sql
method allows us to define the behavior when encountering an existing table with the same name.
The if_exists
parameter accepts three possible values:
- 'fail'
: Raises an error if the table already exists.
- 'replace'
: Drops the existing table and creates a new one with the same name.
- 'append'
: Appends the data frame to the existing table.
Here is an example of using the if_exists
parameter to handle existing tables:
# Export the data frame to an SQL file, appending the data to the existing table df.to_sql('sales', con=engine, if_exists='append', index=False)
In this example, we set if_exists='append'
, which appends the data from the data frame to the existing table named 'sales'. This is useful when you want to add new data to an existing table without overwriting the existing data.
It is important to note that when appending data to an existing table, the column names and data types of the data frame must match the corresponding columns in the table. Any mismatch can result in errors or unexpected behavior.
Related Article: How to use the Python Random Module: Use Cases and Advanced Techniques
Best Practices for Exporting Data Frame to SQL File
Here are some best practices to keep in mind when exporting a data frame to an SQL file:
1. Check and manage data types: Ensure that the data types of the columns in the data frame align with the desired data types in the SQL table. Use the dtype
parameter of the to_sql
method to specify the data types explicitly if needed.
2. Handle existing tables: Consider the presence of existing tables in the SQL database. Choose the appropriate value for the if_exists
parameter of the to_sql
method to handle existing tables. Use 'replace'
to overwrite the existing table, 'append'
to add data to the existing table, or 'fail'
to raise an error if the table already exists.
3. Optimize performance: Depending on the size of your data frame, exporting to an SQL file can be a time-consuming process. To optimize performance, you can use SQLAlchemy's bulk insert functionality or consider using other tools specifically designed for large-scale data exports.
4. Test and validate: Before relying on the exported SQL file, it is essential to test and validate the data. Run queries on the SQL table to ensure that the data was exported correctly and that the desired transformations, such as data type conversions and handling of existing tables, were applied as expected.
In this guide, we have covered the steps involved in exporting a Python data frame to an SQL file using the pandas and SQLAlchemy libraries. We explored the installation process, creating a data frame, connecting to an SQL database, and the code snippet for exporting the data frame to an SQL file. We also discussed important considerations such as managing data types, handling existing tables and indexes, and best practices for exporting data frames to SQL files.
Additional Resources
- Saving a Pandas DataFrame to a SQL Database using SQLAlchemy