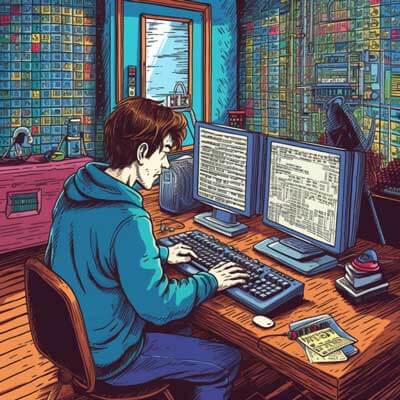
Table of Contents
Overview of Named Tuples
Named tuples are a convenient and efficient way to work with structured data in Python. They are similar to regular tuples, but with the added advantage of being able to access fields by name instead of index. Named tuples are immutable, meaning that once created, their values cannot be modified. This makes them ideal for representing data that should not be changed, such as coordinates, points, or any other type of data that needs to be treated as a single entity.
Related Article: How to Generate Equidistant Numeric Sequences with Python
Immutable Data with Named Tuples
Immutability refers to the property of an object whose state cannot be modified after it is created. Named tuples are immutable, which means that once a named tuple is created, its fields cannot be modified. This can be advantageous in certain situations, such as when you want to ensure that a specific set of data remains constant throughout your program.
For example, let's say you have a named tuple representing a point in a two-dimensional space:
from collections import namedtuple Point = namedtuple('Point', ['x', 'y']) p = Point(3, 4)
Once the point is created, you cannot modify its fields:
p.x = 5 # This will raise an AttributeError
Defining Fields in Named Tuples
When defining a named tuple, you need to specify the name of the tuple type and the names of its fields. The field names can be specified as a list of strings or as a single string with field names separated by spaces or commas.
from collections import namedtuple Person = namedtuple('Person', ['name', 'age'])
or
Person = namedtuple('Person', 'name age')
Working with Collections of Named Tuples
Named tuples can be used as elements of other data structures such as lists, dictionaries, or sets. This allows you to work with collections of named tuples in a convenient and efficient manner.
For example, let's say you have a list of named tuples representing students:
Student = namedtuple('Student', ['name', 'age', 'grade']) students = [ Student('Alice', 18, 'A'), Student('Bob', 17, 'B'), Student('Charlie', 16, 'C') ]
You can access the fields of each student by name:
for student in students: print(student.name, student.age, student.grade)
Related Article: PHP vs Python: How to Choose the Right Language
Attributes of Named Tuples
Named tuples have several attributes that provide useful information about the tuple type and its fields.
- __name__
: The name of the named tuple type.
- _fields
: A tuple containing the names of the fields.
- _asdict()
: Returns an ordered dictionary representation of the named tuple.
- _replace()
: Returns a new named tuple with specified fields replaced with new values.
For example, let's say you have a named tuple representing a person:
Person = namedtuple('Person', ['name', 'age']) p = Person('Alice', 25)
You can access the attributes of the named tuple as follows:
print(p.__name__) # Person print(p._fields) # ('name', 'age') print(p._asdict()) # OrderedDict([('name', 'Alice'), ('age', 25)])
Creating Named Tuples
To create a named tuple instance, you can use the named tuple type as a constructor and pass the values for each field.
from collections import namedtuple Person = namedtuple('Person', ['name', 'age']) p = Person('Alice', 25)
Alternatively, you can also create a named tuple instance by passing the values as arguments to the named tuple type.
p = Person(name='Alice', age=25)
Differences Between Named Tuples and Regular Tuples
While named tuples and regular tuples share some similarities, there are a few key differences between them.
1. Accessing Fields: Named tuples allow you to access fields by name, whereas regular tuples require you to access fields by index.
2. Immutability: Named tuples are immutable, meaning that their fields cannot be modified once created. Regular tuples are also immutable, but they can contain mutable objects.
3. Named tuples have additional attributes such as __name__
, _fields
, _asdict()
, and _replace()
.
Advantages of Named Tuples in Python
Named tuples offer several advantages over regular tuples and other data structures in Python.
1. Readability: Named tuples provide more descriptive field names, making your code more readable and self-explanatory.
2. Accessibility: Named tuples allow you to access fields by name, which makes your code more maintainable and less error-prone.
3. Immutability: Named tuples are immutable, ensuring that the data they represent remains constant throughout your program.
4. Performance: Named tuples are implemented as lightweight Python classes, providing efficient access to their fields.
Related Article: Python Bitwise Operators Tutorial
Using Named Tuples to Create Data Structures
Named tuples can be used to create custom data structures that are both easy to work with and efficient.
For example, let's say you want to represent a rectangle with its width and height:
Rectangle = namedtuple('Rectangle', ['width', 'height']) r = Rectangle(5, 3)
You can then perform operations on the rectangle, such as calculating its area:
area = r.width * r.height print(area) # 15
Additional Resources
- Namedtuple - A more serious approach to Python dictionaries | PyMOTW
- Namedtuples in Python: How to Use Them and Why - Real Python